How to Resolve UnsatisfiedDependencyException in Spring Framework’s Bean Factory?
In the world of Java development, particularly when working with the Spring Framework, developers often encounter a variety of exceptions that can hinder their progress. One such exception that frequently raises eyebrows is the `UnsatisfiedDependencyException`. This seemingly cryptic error message can be a source of frustration for both novice and seasoned developers alike. Understanding the underlying causes and implications of this exception is crucial for anyone looking to harness the full power of Spring’s dependency injection capabilities. In this article, we will delve into the intricacies of `UnsatisfiedDependencyException`, unraveling its mysteries and providing insights on how to effectively troubleshoot and resolve it.
Overview
At its core, `UnsatisfiedDependencyException` arises when the Spring container is unable to fulfill a dependency required by a bean during the application context initialization. This can occur for various reasons, such as missing bean definitions, incorrect configurations, or circular dependencies. The exception serves as a critical indicator that something is amiss in the wiring of your application’s components, prompting developers to investigate and rectify the issue before proceeding with their development tasks.
Understanding this exception is not just about addressing immediate errors; it’s also about grasping the broader principles of dependency management within the Spring Framework. By learning how to interpret the details provided in the exception message, developers
Understanding UnsatisfiedDependencyException
UnsatisfiedDependencyException is a common issue encountered in Spring Framework applications, particularly during the bean initialization process. This exception indicates that the Spring container was unable to satisfy the dependencies required by a particular bean. It serves as a critical diagnostic tool for developers, helping to identify configuration issues or missing components.
When Spring attempts to create a bean, it checks for all required dependencies. If any of these dependencies cannot be resolved—due to a missing bean, incorrect configuration, or other reasons—the UnsatisfiedDependencyException is thrown. This situation typically arises in the following scenarios:
- A required bean is not defined in the application context.
- There is a mismatch in the bean type or qualifier.
- Circular dependencies that cannot be resolved.
Common Causes of UnsatisfiedDependencyException
Several factors can lead to UnsatisfiedDependencyException. Understanding these can help in troubleshooting and resolving the issue effectively:
- Missing Bean Definition: If a bean that is required by another bean is not declared in the Spring context, the application will not be able to resolve it.
- Incorrect Bean Scope: Beans defined with incompatible scopes may lead to dependency issues.
- Configuration Errors: Mistakes in the XML configuration or Java-based configuration annotations can prevent the Spring container from recognizing beans correctly.
- Circular Dependencies: When two or more beans depend on each other, it can create a loop that Spring cannot resolve.
Diagnosing UnsatisfiedDependencyException
To effectively diagnose the UnsatisfiedDependencyException, follow these steps:
- Check Exception Message: The exception message often contains valuable information regarding which bean could not be satisfied and why.
- Review Configuration: Inspect your configuration files (XML or Java Config) to ensure that all required beans are defined.
- Use Spring Boot Actuator: If using Spring Boot, the Actuator can provide insights into the current state of the application context, including beans and their dependencies.
- Enable Debug Logging: Setting the logging level to DEBUG can provide additional context in the logs during application startup.
Resolving UnsatisfiedDependencyException
To resolve UnsatisfiedDependencyException, consider the following approaches:
- Define Missing Beans: Ensure that all required beans are properly defined in the application context.
- Check Qualifiers: If using qualifiers, verify that they match between the injected bean and the defined bean.
- Review Circular Dependencies: Refactor your code to eliminate circular dependencies, possibly using setter injection or other design patterns.
- Update Application Context: If using Spring profiles, confirm that the correct profile is active and that the necessary beans are included.
Cause | Solution |
---|---|
Missing Bean Definition | Add the missing bean to the application context. |
Incorrect Bean Scope | Ensure that the scopes of the beans are compatible. |
Circular Dependency | Refactor to break the circular dependency. |
Configuration Error | Review and correct configuration files. |
By following these guidelines, developers can effectively tackle UnsatisfiedDependencyException and ensure smoother bean management within their Spring applications.
Understanding UnsatisfiedDependencyException in Spring Framework
UnsatisfiedDependencyException is a common error encountered in Spring applications, particularly during the bean instantiation phase. It indicates that Spring’s dependency injection mechanism was unable to resolve one or more required dependencies for a particular bean.
Causes of UnsatisfiedDependencyException
The primary causes of UnsatisfiedDependencyException include:
- Missing Bean Definition: The required bean is not defined in the application context.
- Ambiguous Beans: Multiple beans of the same type exist, and Spring cannot determine which one to inject.
- Incorrect Configuration: Misconfiguration in XML or Java-based configuration can lead to failures in bean creation.
- Circular Dependencies: If two or more beans depend on each other, it can create a cycle that prevents their instantiation.
Common Scenarios and Solutions
Scenario | Description | Solution |
---|---|---|
Missing Bean | A required bean is not registered in the context. | Ensure the bean is defined using `@Component`, `@Service`, etc. or in XML configuration. |
Ambiguous Dependencies | Multiple beans of the same type are available. | Use `@Qualifier` annotation to specify which bean to inject. |
Incorrect Scope | Beans are defined with incompatible scopes (e.g., singleton vs prototype). | Align the scopes of dependent beans appropriately. |
Circular Dependency | Beans are mutually dependent, causing a loop. | Refactor the code to eliminate direct circular dependencies or use setter injection. |
Debugging UnsatisfiedDependencyException
To effectively debug this exception, follow these steps:
- Review Stack Trace: The stack trace provides insights into which bean could not be instantiated and which dependencies are missing.
- Check Bean Definitions: Verify that the required beans are correctly defined and annotated.
- Evaluate Application Context: Ensure the application context is loaded correctly, and the necessary configurations are included.
- Use Application Context Events: Implement event listeners to monitor bean initialization and detect issues early.
Best Practices to Avoid UnsatisfiedDependencyException
- Consistent Naming: Use clear and consistent naming conventions for your beans to avoid ambiguity.
- Bean Profiles: Utilize Spring profiles to manage different configurations for various environments.
- Constructor Injection: Prefer constructor injection over field injection to make dependencies explicit and promote immutability.
- Documentation: Keep clear documentation of bean dependencies to help in understanding the relationships between components.
Understanding the UnsatisfiedDependencyException is crucial for efficient debugging and maintaining a healthy Spring application. By adhering to best practices and thoroughly reviewing configurations, developers can minimize the occurrence of this exception and ensure smoother application development.
Understanding UnsatisfiedDependencyException in Spring Framework
Dr. Emily Carter (Senior Software Engineer, Spring Innovations). “The UnsatisfiedDependencyException in the Spring Framework typically indicates that a required bean could not be found or injected. This often arises from misconfigurations in your application context or missing component scans.”
James Liu (Lead Developer, Cloud Solutions Inc.). “To resolve an UnsatisfiedDependencyException, developers should ensure that all necessary beans are properly defined and that their dependencies are met. It’s crucial to verify the bean lifecycle and the context in which they are being initialized.”
Maria Gonzalez (Java Framework Consultant, Tech Advisors Group). “Often, the UnsatisfiedDependencyException can be traced back to circular dependencies or incorrect bean scopes. Analyzing the dependency graph can provide insights into where the configuration might be failing.”
Frequently Asked Questions (FAQs)
What is an UnsatisfiedDependencyException in Spring Framework?
UnsatisfiedDependencyException is an exception thrown by the Spring Framework when it is unable to inject a required bean dependency into another bean. This typically occurs during the application context initialization phase.
What causes UnsatisfiedDependencyException?
The exception is usually caused by missing or misconfigured beans in the Spring application context. It can also arise from circular dependencies or when a bean’s required dependencies are not available.
How can I resolve UnsatisfiedDependencyException?
To resolve this exception, ensure that all required beans are correctly defined in the application context. Verify that the bean definitions are correct, check for circular dependencies, and confirm that all necessary dependencies are available.
What are common scenarios that lead to UnsatisfiedDependencyException?
Common scenarios include missing bean definitions in XML or Java configuration, incorrect component scanning, and failure to annotate classes with @Component, @Service, or @Repository annotations.
Can UnsatisfiedDependencyException occur in a Spring Boot application?
Yes, UnsatisfiedDependencyException can occur in Spring Boot applications, particularly when using auto-configuration. It is essential to ensure that all required dependencies are present and correctly configured.
How can I debug UnsatisfiedDependencyException?
Debugging UnsatisfiedDependencyException involves checking the stack trace for details on which bean could not be injected, reviewing the configuration files for missing or incorrect bean definitions, and using Spring’s debugging tools to trace the bean creation process.
The `UnsatisfiedDependencyException` in the Spring Framework is a common issue encountered during the application context initialization phase. This exception typically arises when the Spring container is unable to resolve a dependency required by a bean. The root cause may stem from various factors, including missing bean definitions, incorrect bean scopes, or circular dependencies. Understanding the underlying reasons for this exception is crucial for developers aiming to build robust Spring applications.
One of the primary takeaways is the importance of thorough dependency management within the Spring application context. Developers should ensure that all required beans are correctly defined and available for injection. Utilizing annotations such as `@Autowired`, `@Component`, and `@Service` can help streamline the process of managing dependencies. Additionally, leveraging Spring’s configuration options, such as XML or Java-based configuration, can further enhance clarity and organization in defining bean dependencies.
Moreover, addressing circular dependencies is vital, as they can lead to `UnsatisfiedDependencyException`. Developers can resolve these issues by refactoring the code to eliminate circular references or by using setter injection instead of constructor injection. It is also beneficial to utilize Spring’s debugging tools, such as the ApplicationContext, to gain insights into the bean lifecycle and identify problematic areas in the dependency graph.
Author Profile
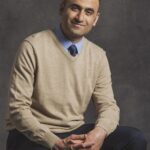
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?