How Can You Fix the OSError: [Errno 24] Too Many Open Files?
Have you ever encountered the frustrating `OSError: [Errno 24] Too many open files` message while working on a project? If so, you’re not alone. This error is a common hurdle faced by developers and system administrators alike, often appearing at the most inconvenient times. As our applications grow in complexity and the demand for resources increases, understanding the underlying causes of this error becomes crucial for maintaining smooth operations. In this article, we will delve into the intricacies of file handling in operating systems, explore the reasons behind this pesky error, and provide actionable solutions to help you overcome it.
The `OSError: [Errno 24]` is a signal that your application has exceeded the maximum number of file descriptors allowed by the operating system. This limit is in place to prevent resource exhaustion, which can lead to system instability. However, as applications become more resource-intensive, whether through increased user interactions or the need to manage numerous files simultaneously, hitting this limit can become a frequent occurrence. Understanding how file descriptors work and how they are managed by your system is essential for diagnosing and fixing this issue.
In addition to the technical aspects, we will also discuss best practices for file management, including how to properly close file handles and implement efficient resource allocation strategies
Understanding the Error
The error message `OSError: [Errno 24] Too many open files` typically indicates that a process has attempted to open more files than the operating system allows. Each operating system has a limit on the number of file descriptors that can be opened by a single process or the entire system. When this limit is exceeded, the error is raised, and the affected application may fail to operate as intended.
Common causes of this error include:
- Resource Leaks: Failing to close file descriptors after their use can lead to exhaustion of available file handles.
- High Concurrency: Applications designed to handle many simultaneous connections (e.g., web servers) can quickly reach open file limits.
- Configuration Settings: Default limits are often lower than necessary for high-traffic applications or services.
Identifying the Limits
To diagnose and address the issue, it’s crucial to understand the current limits set on your system. You can check the maximum number of open files allowed using the `ulimit` command in a Unix-like operating system:
“`bash
ulimit -n
“`
This command will return the current limit for the number of open files. To see the limits for the entire system, you can examine the `/proc/sys/fs/file-max` file:
“`bash
cat /proc/sys/fs/file-max
“`
The output will indicate the maximum number of file handles that the kernel will allocate.
Increasing the Limit
If the existing limits are insufficient, they can be adjusted. The method of increasing the limit will depend on whether you want to set it for a single session, a specific user, or system-wide.
For a single session: You can temporarily increase the limit in your terminal session using:
“`bash
ulimit -n [new_limit]
“`
For a specific user: You can modify the `limits.conf` file located at `/etc/security/limits.conf`. Add the following lines to set new soft and hard limits for a user:
“`
username soft nofile new_limit
username hard nofile new_limit
“`
For system-wide settings: You can adjust the value in the `/etc/sysctl.conf` file by adding or modifying the following line:
“`
fs.file-max = new_limit
“`
After editing this file, apply the changes with:
“`bash
sudo sysctl -p
“`
Best Practices to Avoid the Error
To mitigate the risk of encountering this error in the future, consider implementing the following best practices:
- Always Close File Descriptors: Ensure that files and network connections are explicitly closed after use. Utilizing context managers in Python can help manage this automatically.
- Monitor Resource Usage: Use tools like `lsof` to monitor open files and detect leaks:
“`bash
lsof -p [PID]
“`
- Optimize Application Design: Reduce the number of concurrently open files when possible. For example, use connection pooling for databases.
Summary Table of Commands
Command | Description |
---|---|
ulimit -n | Displays the current limit on open files. |
cat /proc/sys/fs/file-max | Shows the maximum file handles allocated by the kernel. |
lsof -p [PID] | Lists open files for a given process ID. |
Understanding the Error
The error `OSError: [Errno 24] Too many open files` indicates that a process has reached the limit on the number of file descriptors it can have open simultaneously. Each file, socket, or pipe that a process opens consumes a file descriptor, which is a unique identifier for that file within the operating system.
File descriptor limits are governed by operating system settings, which can be adjusted but have default values. The error typically arises in applications that open many files or network connections without properly closing them.
Common Causes
Several factors can lead to hitting the open files limit:
- Resource Leaks: Failing to close file descriptors after use, leading to gradual resource exhaustion.
- High Concurrency: Applications that spawn numerous threads or processes, each requiring file access.
- Configuration Limits: Default limits set by the operating system, which can vary between Unix-like systems and Windows.
Checking Current Limits
To diagnose the issue, it is important to check the current limits on open files. This can be done using various commands based on the operating system:
Operating System | Command |
---|---|
Linux | `ulimit -n` |
macOS | `ulimit -n` |
Windows | `Get-Item WSMan:\localhost\Shell\MaxShells` (PowerShell) |
Increasing File Descriptor Limits
If the default limits are too low for your application, consider increasing them. The method varies by operating system:
- Linux and macOS:
- Edit the `/etc/security/limits.conf` file.
- Add or modify the lines:
“`
- soft nofile 65536
- hard nofile 65536
“`
- Save the file and log out and back in to apply changes.
- Windows:
Windows does not have a direct equivalent but can be managed through system settings and ensuring proper resource management in applications.
Best Practices for Resource Management
To avoid encountering this error, adopt the following best practices:
- Always Close File Descriptors: Use context managers in Python (e.g., `with open() as f:`) to ensure files are closed automatically.
- Limit Concurrent Connections: Use connection pooling for databases or APIs to manage the number of simultaneous connections.
- Monitor Resource Usage: Implement logging to track the number of open files and connections over time.
Debugging the Issue
If the error persists, debugging may be required:
- Use `lsof` Command: On Unix-like systems, `lsof` lists open files and their usage:
“`
lsof -p
“`
- Profile Your Application: Tools like `py-spy` or `objgraph` can help identify resource leaks in Python applications.
Addressing the `OSError: [Errno 24] Too many open files` error involves a combination of monitoring, configuration, and adherence to best coding practices. By understanding the underlying causes and implementing proactive resource management strategies, you can mitigate the risk of encountering this issue in your applications.
Understanding the Causes and Solutions for OSError: [Errno 24] Too Many Open Files
Dr. Emily Carter (Systems Architect, Tech Innovations Inc.). “The error ‘OSError: [Errno 24] too many open files’ typically arises when an application exceeds the limit of file descriptors it can open simultaneously. This limit is often set by the operating system and can be adjusted based on the application’s requirements and server capacity. Properly managing file handles and implementing efficient resource cleanup can mitigate this issue.”
James Liu (Senior Software Engineer, Cloud Solutions Corp.). “In my experience, encountering the ‘too many open files’ error is common in high-load environments. It is crucial to monitor and optimize file usage within applications. Tools like `lsof` can help track open files, while increasing the limits in configuration files, such as `/etc/security/limits.conf`, can provide a quick resolution.”
Linda Thompson (DevOps Specialist, NextGen Technologies). “Addressing the ‘OSError: [Errno 24]’ error requires a dual approach: first, diagnose the application to identify potential file leaks, and second, adjust the system’s file descriptor limits. Additionally, employing connection pooling for database interactions can significantly reduce the number of open files needed at any given time.”
Frequently Asked Questions (FAQs)
What does the error “oserror: [errno 24] too many open files” mean?
This error indicates that a process has attempted to open more files than the operating system allows, exceeding the limit set for file descriptors.
What causes the “too many open files” error?
The error typically arises from a program that opens files without properly closing them, or when a system-wide limit on file descriptors is reached due to high concurrent file usage.
How can I check the current limit for open files on my system?
You can check the limit by using the command `ulimit -n` in a terminal on Unix-based systems, which displays the maximum number of open file descriptors allowed for the current user session.
How can I increase the limit for open files?
To increase the limit, you can modify the `/etc/security/limits.conf` file on Linux systems by adding or editing entries for the desired user or group, and then restart the session or system for changes to take effect.
What are the potential risks of increasing the open files limit?
Increasing the limit can lead to higher resource consumption, which may affect system stability and performance if not managed properly. It is essential to monitor resource usage after making changes.
How can I troubleshoot and resolve the “too many open files” error?
To troubleshoot, identify the process causing the issue using commands like `lsof` to list open files. Ensure that files are being closed properly in your code, and consider optimizing file handling or increasing the limit if necessary.
The error message “OSError: [Errno 24] Too many open files” indicates that a process has exceeded the limit of file descriptors it can open simultaneously. This situation often arises in applications that handle numerous files or network connections, such as web servers, database applications, or data processing scripts. Operating systems impose a limit on the number of file descriptors to prevent resource exhaustion, and when this limit is reached, the application can no longer open new files or sockets, leading to potential disruptions in functionality.
To address this issue, it is essential to identify the root cause of the excessive file descriptors. Common reasons include improper file handling, such as failing to close files after use, or design flaws in the application that lead to resource leaks. Developers should implement best practices for file management, including utilizing context managers in Python or ensuring that all resources are explicitly closed when no longer needed. Additionally, monitoring tools can help track open file descriptors to diagnose and resolve issues before they escalate.
Another solution is to increase the file descriptor limit set by the operating system. This can typically be done by modifying system configuration files or using commands to adjust the limits for specific users or processes. However, this should be approached with caution, as merely increasing the
Author Profile
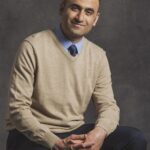
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?