How Can You Resolve OSError Errno 24: Too Many Open Files?
In the fast-paced world of computing, efficiency and resource management are paramount. However, even the most seasoned developers and system administrators can encounter perplexing errors that disrupt their workflow. One such error is the notorious `OSError: [Errno 24] Too many open files`, a message that can send chills down the spine of anyone managing a server or developing an application. This error signifies a critical threshold has been crossed, where the system can no longer handle additional file descriptors, leading to potential application crashes or degraded performance. Understanding this error is essential for maintaining robust systems and ensuring smooth operations.
At its core, the `OSError: [Errno 24] Too many open files` is a manifestation of resource limits within operating systems. Each process is allocated a finite number of file descriptors, which are used for various operations, including accessing files, sockets, and other I/O resources. When an application exceeds this limit, it can no longer open new files or connections, leading to unexpected behavior and frustration for developers and users alike. This error can arise in various scenarios, from web servers handling numerous simultaneous connections to applications that fail to close file handles properly.
To tackle this issue effectively, it’s crucial to understand both the underlying causes and the potential solutions. Whether it’s adjusting
Understanding OSError: Errno 24
When working with file systems in programming, encountering the error `OSError: [Errno 24] Too many open files` can be a common issue. This error arises when a process attempts to open more files than the limit set by the operating system. Each operating system has a predefined limit on the number of file descriptors that can be opened simultaneously by a single process or by the entire system.
The limit on open files can vary based on the operating system and its configuration. Here are some key points about this error:
- File Descriptor: A file descriptor is a unique identifier for an open file, socket, or other resources within a process.
- System Limit: Each operating system has a maximum limit for open file descriptors, which can usually be modified but defaults to a certain value.
- Process Limit: Individual processes have their own limits, which may be lower than the system-wide limit.
Causes of the Error
The `Errno 24` error can occur due to several reasons, including:
- Resource Leakage: Not properly closing file descriptors after their use can lead to resource leakage, causing the limit to be reached.
- High Concurrency: Applications that handle many simultaneous connections, such as web servers or database connections, can quickly exhaust available file descriptors.
- Misconfigured Limits: The system or user-defined limits may be set too low for the intended application’s needs.
Checking Current Limits
To troubleshoot the `OSError: Errno 24`, one must first check the current limits for open files. This can be done using the following command in the terminal:
“`bash
ulimit -n
“`
This command returns the number of open file descriptors allowed for the current user session.
Increasing Open File Limits
If the current limit is insufficient, it can be increased. The method to do this depends on the operating system. Here’s a brief overview:
- Linux:
- To temporarily change the limit for the current session, use:
“`bash
ulimit -n [new_limit]
“`
- To make the change permanent, edit `/etc/security/limits.conf` and add:
“`
username soft nofile [new_limit]
username hard nofile [new_limit]
“`
- macOS:
- Similar to Linux, use:
“`bash
ulimit -n [new_limit]
“`
- For permanent changes, edit the launchd configuration.
- Windows:
- Windows does not have a direct equivalent, but limits can be managed through the Windows Registry or by configuring specific application settings.
Best Practices to Avoid OSError: Errno 24
To prevent running into the `OSError: Errno 24`, consider the following best practices:
- Always Close File Descriptors: Ensure that files, sockets, and other resources are closed properly after use. Utilize context managers in Python:
“`python
with open(‘file.txt’) as f:
data = f.read()
“`
- Monitor Resource Usage: Use monitoring tools to keep an eye on file descriptor usage within applications to anticipate limits.
- Optimize Resource Handling: Consider techniques such as connection pooling for database access or using asynchronous I/O for network connections.
Operating System | Command to Check Limits | Command to Increase Limits |
---|---|---|
Linux | ulimit -n | ulimit -n [new_limit] |
macOS | ulimit -n | ulimit -n [new_limit] |
Windows | N/A | Registry or application settings |
Understanding the Error
The `OSError: [Errno 24] Too many open files` error indicates that a process has reached the limit of file descriptors it can open. File descriptors are used by the operating system to manage files, sockets, and other resources. When the limit is exceeded, the operating system prevents further file operations, leading to this error.
Common Causes
Several factors can lead to this error:
- Excessive File Operations: Opening too many files within a single process without closing them can quickly exhaust the available file descriptors.
- File Descriptor Leaks: Failing to close file descriptors after usage can result in a gradual increase in open files, eventually hitting the limit.
- High Concurrency: Applications that handle many concurrent connections (e.g., web servers) may require more file descriptors than the default limit.
- System-wide Limits: The operating system itself has limits that can be reached due to the cumulative number of open files across all processes.
Identifying the Limit
To check the current limits on your system, use the following commands depending on your operating system:
- Linux:
“`bash
ulimit -n
“`
- macOS:
“`bash
ulimit -n
“`
- Windows:
Windows does not have a direct equivalent, but the limits can be checked in the registry or through system settings.
Increasing the Limit
If the default limit is insufficient, you can increase it. Here’s how:
- Linux:
- Edit the `/etc/security/limits.conf` file and add:
“`plaintext
- soft nofile 4096
- hard nofile 8192
“`
- Apply the changes by logging out and back in, or by rebooting the system.
- macOS:
- Edit `/etc/launchd.conf` and add:
“`plaintext
limit maxfiles 4096 8192
“`
- Restart the system for changes to take effect.
- Docker (if applicable):
Add the following to your Docker daemon configuration:
“`json
{
“default-ulimits”: {
“nofile”: {
“hard”: 8192,
“soft”: 4096
}
}
}
“`
Best Practices to Avoid the Error
Implementing best practices can help prevent hitting the open file limit:
- Always Close Files: Ensure that every file opened is closed after its use. Utilize context managers in Python (with `with` statements) to automatically handle file closure.
- Monitor Resource Usage: Use tools to monitor open files and connections, such as `lsof` on Linux.
- Optimize File Usage: Reduce the number of files opened simultaneously by batching operations or using file caching mechanisms.
- Adjust Application Logic: If your application inherently requires a large number of file descriptors, consider refactoring it to manage resources more efficiently.
Debugging the Issue
When the error occurs, consider these debugging steps:
- List Open Files: Use the command:
“`bash
lsof -p
Replace `
- Check for Leaks: Review your code for any missed file closures or excessive file operations.
- Log Resource Usage: Implement logging to track the number of open files throughout the application’s lifecycle.
By addressing these areas, you can effectively manage file descriptors and minimize the risk of encountering the `OSError: [Errno 24] Too many open files` error.
Understanding the ‘OSError Errno 24: Too Many Open Files’ Issue
Dr. Emily Tran (Systems Architect, Tech Innovations Inc.). “The ‘OSError Errno 24’ typically arises when a process attempts to open more file descriptors than the system allows. This can be particularly problematic in applications that require handling multiple file streams simultaneously, such as web servers or database applications. It is crucial to monitor and manage file descriptor usage to prevent this error.”
Mark Chen (DevOps Engineer, Cloud Solutions Co.). “To mitigate the ‘too many open files’ error, one should consider increasing the limit of open file descriptors at both the user and system levels. This can often be done by modifying the limits in the `/etc/security/limits.conf` file or using the `ulimit` command in Unix-based systems. However, it is equally important to analyze the application’s file handling logic to ensure it closes files properly.”
Sarah Patel (Software Development Manager, Innovative Software Ltd.). “In many cases, the occurrence of OSError Errno 24 indicates a memory leak or improper resource management within the application. Developers should implement proper error handling and resource cleanup mechanisms to ensure that all opened files are closed after use. Additionally, utilizing tools to monitor file descriptor usage can help identify bottlenecks and optimize performance.”
Frequently Asked Questions (FAQs)
What does the error “OSError Errno 24: Too many open files” mean?
This error indicates that a process has exceeded the limit of file descriptors it can open simultaneously. Each open file, socket, or other resource consumes a file descriptor, and when the limit is reached, the operating system prevents further file openings.
What causes “OSError Errno 24: Too many open files”?
This error typically arises from applications that do not properly close file descriptors after use, leading to resource leaks. It can also occur when a program attempts to open a large number of files concurrently, exceeding the system’s configured limits.
How can I check the current limit for open files on my system?
You can check the limit by running the command `ulimit -n` in the terminal on Unix-based systems. This command will display the maximum number of open file descriptors allowed for the current user session.
How can I increase the limit for open files?
To increase the limit, you can use the command `ulimit -n [new_limit]` in the terminal, where `[new_limit]` is the desired number of open files. For permanent changes, you may need to edit configuration files such as `/etc/security/limits.conf` or systemd service files.
What are the potential risks of increasing the open files limit?
Increasing the limit can lead to higher resource consumption, which may affect system stability and performance. It is important to ensure that the system has adequate resources to handle the increased load without adverse effects.
How can I troubleshoot applications that trigger this error?
To troubleshoot, review the application code for proper file handling practices, ensuring that all file descriptors are closed after use. Additionally, monitor the number of open files using tools like `lsof` to identify potential leaks or excessive usage patterns.
The error message “OSError: [Errno 24] Too many open files” typically indicates that a process has exceeded the limit of file descriptors it can open simultaneously. This limit is set by the operating system and can vary based on system configuration and user permissions. When a program attempts to open more files than allowed, it results in this error, which can disrupt the application’s functionality and lead to performance issues.
To address this issue, it is essential to identify the root cause, which may involve examining the application for potential file leaks or ensuring that files are properly closed after use. Additionally, system administrators can increase the limit of open files by modifying system settings, such as the `ulimit` command in Unix-based systems. However, increasing the limit should be approached with caution, as it may lead to resource exhaustion if not managed properly.
Key takeaways include the importance of efficient file management within applications to prevent reaching the open file limit. Regular monitoring and implementing best practices for resource handling can mitigate the occurrence of this error. Furthermore, understanding the system’s configuration and limits can empower developers and administrators to optimize performance and maintain stability in their applications.
Author Profile
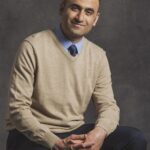
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?