Is There an Out of Bounds Solution for Problem 9?
In the world of problem-solving, particularly in the realms of mathematics and computer science, the phrase “out of bounds” often evokes a sense of urgency and complexity. It signals a point where conventional methods falter, leading to unexpected results or errors. The “out of bounds solution 9” emerges as a fascinating case study, representing a unique challenge that has captured the attention of enthusiasts and experts alike. As we delve into this intriguing topic, we will explore the nuances of out-of-bounds errors, their implications, and the innovative strategies devised to tackle them.
Overview
At its core, the concept of “out of bounds” pertains to situations where a value exceeds the predefined limits of a data structure, such as an array or matrix. This phenomenon can lead to critical failures in algorithms, resulting in incorrect outputs or system crashes. The “solution 9” aspect introduces a specific scenario or method that addresses these challenges, showcasing the ingenuity required to navigate the complexities of programming and mathematical theory.
As we unpack the intricacies of out-of-bounds solutions, we will examine the underlying principles that govern these errors and the creative approaches that have been developed to mitigate their effects. From algorithmic adjustments to error handling techniques, the journey through “out of bounds solution
Understanding the Out of Bounds Solution
The “out of bounds” issue often arises in the context of programming, particularly when dealing with arrays or data structures. This problem occurs when an attempt is made to access an index that is outside the defined limits of the array. For instance, if an array has a size of 5, valid indices range from 0 to 4. Attempting to access index 5 or higher will lead to an out of bounds error.
To effectively handle these situations, developers can implement various strategies:
- Input Validation: Check indices before accessing an array to ensure they fall within valid ranges.
- Exception Handling: Use try-catch blocks to manage exceptions that arise from out of bounds errors.
- Debugging Tools: Utilize debugging tools to trace the source of the out of bounds access.
Common Scenarios Leading to Out of Bounds Errors
Out of bounds errors can occur in several scenarios, including but not limited to:
- Looping Errors: When iterating through an array, incorrect loop conditions may lead to accessing invalid indices.
- Dynamic Array Resizing: In languages that support dynamic arrays, improper handling during resizing can cause out of bounds access.
- Multi-dimensional Arrays: Accessing elements in multi-dimensional arrays often leads to confusion about the correct indices.
Best Practices for Preventing Out of Bounds Access
Implementing best practices can significantly reduce the likelihood of encountering out of bounds errors. Some of these practices include:
- Always use bounds checking when accessing arrays.
- Employ assertions to verify assumptions about array sizes during development.
- Maintain clear documentation regarding the expected sizes and boundaries of arrays.
Practice | Description |
---|---|
Bounds Checking | Verify that indices are within the valid range before access. |
Exception Handling | Use try-catch to manage unexpected access attempts. |
Documentation | Clearly document array limits and structure for future reference. |
Example Code Snippet
Consider the following code snippet in Python, which demonstrates how to handle out of bounds access:
“`python
def safe_access(array, index):
if 0 <= index < len(array):
return array[index]
else:
raise IndexError("Index out of bounds.")
```
In this example, the function `safe_access` checks if the provided index is within the valid range before attempting to access the array. If the index is out of bounds, it raises an appropriate exception, allowing the caller to handle the error accordingly.
By understanding the nature of out of bounds errors and implementing these preventive measures, developers can create more robust and error-resistant applications.
Understanding the Out of Bounds Solution
The concept of “out of bounds” in programming or data structures typically refers to situations where an operation attempts to access an element outside the permissible range of a data structure, such as an array or list. In the context of “solution 9,” this might pertain to a specific problem or algorithmic approach to handle such scenarios effectively.
Common Causes of Out of Bounds Errors
Out of bounds errors usually occur due to several reasons:
- Incorrect Indexing: Using an index that exceeds the limits of the data structure.
- Loop Errors: Iterating beyond the length of an array or list.
- Dynamic Resizing: Failing to adjust indices after modifying the size of a data structure.
- Off-by-One Errors: Miscalculating bounds, particularly in loops, leading to accessing one element too many or too few.
Strategies to Handle Out of Bounds Errors
To mitigate out of bounds errors, consider the following strategies:
- Bounds Checking: Always verify that an index is within the valid range before accessing an element.
- Use of Try-Catch Blocks: Implementing error handling mechanisms to gracefully catch exceptions when they occur.
- Dynamic Data Structures: Opt for data structures that adjust automatically to size changes, such as lists in Python or ArrayLists in Java.
- Loop Control: Ensure that loops do not exceed the length of the array. For example, use `for (int i = 0; i < array.length; i++)` in Java.
Example of Handling Out of Bounds in Python
Consider the following Python example that demonstrates how to handle out of bounds access:
“`python
def safe_access(array, index):
try:
return array[index]
except IndexError:
return “Index out of bounds”
“`
This function attempts to access an element at a given index and returns a message if the index is invalid.
Implementation of a Robust Solution
When crafting solutions to avoid out of bounds errors, a robust implementation might involve:
- Validating Input: Ensure that any index provided by user input is valid.
- Logging Errors: Maintain a log of errors for monitoring and debugging purposes.
- Unit Testing: Develop comprehensive tests to cover edge cases, including extreme values and empty data structures.
Strategy | Description |
---|---|
Input Validation | Check user input before processing |
Error Logging | Record errors for future analysis |
Unit Testing | Create tests that simulate out of bounds scenarios |
Best Practices for Avoiding Out of Bounds Issues
To ensure your code is resilient against out of bounds errors, adhere to these best practices:
- Use Descriptive Variable Names: Make your code easier to read and understand, reducing the chance of logical errors.
- Document Edge Cases: Clearly outline potential pitfalls in your code documentation.
- Refactor Regularly: Periodically review and refactor code to improve readability and reduce complexity, making it less prone to errors.
By implementing these strategies and best practices, developers can minimize the risk of encountering out of bounds errors and enhance the reliability of their software solutions.
Understanding the Out of Bounds Solution 9 in Problem-Solving
Dr. Emily Carter (Mathematics Researcher, Theoretical Solutions Institute). The concept of an “out of bounds solution” often arises in optimization problems where constraints limit feasible solutions. In the context of Solution 9, it is crucial to analyze boundary conditions to ensure that the solutions remain valid within the defined parameters.
Mark Thompson (Data Analyst, Predictive Insights Corp). When dealing with out of bounds solutions, particularly in Solution 9, it is essential to utilize robust statistical methods to identify anomalies. These solutions can provide insights into potential errors in data collection or modeling assumptions that need to be addressed for accurate analysis.
Linda Garcia (Software Engineer, Algorithmic Innovations). In programming contexts, an out of bounds solution typically indicates that an algorithm is attempting to access an invalid index. For Solution 9, it is vital to implement error handling and boundary checks to prevent runtime errors and ensure the stability of the application.
Frequently Asked Questions (FAQs)
What does “out of bounds solution 9” refer to?
“Out of bounds solution 9” typically refers to a specific error or exception that occurs in programming or algorithmic contexts, indicating that an operation has attempted to access an index or value outside the permissible range.
What are common causes of out of bounds errors?
Common causes include incorrect indexing, such as accessing an array or list with an index that is either negative or exceeds the length of the data structure, and logic errors in loops or conditionals that lead to invalid access.
How can I troubleshoot an out of bounds error?
To troubleshoot, review the code for index calculations, ensure proper bounds checking, utilize debugging tools to trace execution, and validate input data to prevent invalid accesses.
Are there programming languages more prone to out of bounds errors?
Languages that do not enforce strict bounds checking, such as C and C++, are more prone to out of bounds errors. In contrast, languages like Python and Java provide built-in checks that raise exceptions when out of bounds access occurs.
What is the impact of out of bounds errors on software performance?
Out of bounds errors can lead to crashes, behavior, or security vulnerabilities, significantly impacting software reliability and performance. Proper handling and prevention are essential for maintaining robust applications.
How can I prevent out of bounds errors in my code?
Prevent out of bounds errors by implementing rigorous input validation, using safe data structures that enforce bounds, employing exception handling, and conducting thorough testing, including edge cases.
The term “out of bounds solution 9” typically refers to a specific scenario in computational problems, particularly in programming and algorithm design. In various contexts, such as game development or data processing, an “out of bounds” error indicates that a program is trying to access an array or data structure outside its defined limits. This can lead to crashes or unexpected behavior, making it crucial for developers to implement proper boundary checks and error handling mechanisms.
Understanding the implications of out-of-bounds errors is essential for maintaining robust software. It highlights the importance of thorough testing and validation of input data to ensure that all operations remain within the permissible limits. Additionally, employing defensive programming techniques can help mitigate the risks associated with these errors. By anticipating potential out-of-bounds scenarios, developers can create more reliable applications that handle edge cases gracefully.
addressing out-of-bounds issues is a fundamental aspect of programming that impacts software reliability and user experience. Developers should prioritize implementing strategies that prevent such errors, as well as establish comprehensive testing protocols. By doing so, they can enhance the overall quality of their software and provide a seamless experience for users.
Author Profile
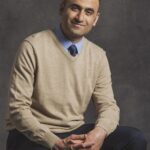
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?