How to Handle Out of Order Sequence Responses in Golang with HBase?
In the dynamic world of data management, ensuring the integrity and order of data transactions is paramount, especially when working with distributed systems. For developers using Go (Golang) in conjunction with HBase, a popular NoSQL database, the challenge of handling out-of-order sequences can be particularly daunting. As applications scale and the volume of data grows, the risk of encountering discrepancies in data order increases, leading to potential issues in data retrieval and processing. This article delves into the intricacies of managing out-of-order sequence responses in Golang when interfacing with HBase, providing insights and strategies to maintain data consistency and reliability.
At the heart of this discussion lies the fundamental nature of HBase as a distributed database designed for high-throughput and low-latency access to large datasets. However, the very characteristics that make HBase powerful can also introduce complexities when it comes to data sequencing. In a typical scenario, operations may not always execute in the order they were initiated, leading to challenges in maintaining the expected sequence of responses. Understanding how to effectively manage these out-of-order sequences is crucial for developers aiming to build robust applications that rely on accurate data processing.
This article will explore various techniques and best practices for handling out-of-order sequence responses in Golang applications using HBase.
Understanding Out of Order Sequences in HBase
In HBase, data is stored in a distributed manner, which can result in out of order sequences when data is being written or read. This can lead to inconsistencies and unexpected behaviors in applications that rely on strict ordering of records. Understanding how to handle these out of order sequences is crucial for maintaining data integrity and ensuring a reliable application.
To address this, developers often implement strategies to manage the order of data. Some common approaches include:
- Timestamping Records: Each record can be assigned a timestamp that reflects the time it was created. This allows clients to sort or filter records based on their temporal sequence.
- Versioning: HBase supports multiple versions of a cell. By utilizing versions, applications can retrieve the latest record and manage out of order sequences more effectively.
- Client-side Sorting: When fetching data, clients can sort the results based on the timestamp or an application-specific identifier.
Implementing Out of Order Handling in Go
When working with HBase in Go, developers can implement out of order handling using the Go HBase client. A common pattern is to fetch records and sort them based on a timestamp. Below is a simplified example of how this can be implemented:
“`go
package main
import (
“context”
“fmt”
“time”
“github.com/apache/hbase-client-go/hbase”
)
func main() {
client, err := hbase.NewClient(“localhost:2181”)
if err != nil {
fmt.Println(“Error creating HBase client:”, err)
return
}
ctx := context.Background()
table := client.Table(“my_table”)
// Fetch data
result, err := table.Get(ctx, []byte(“row_key”))
if err != nil {
fmt.Println(“Error fetching data:”, err)
return
}
// Example data processing
records := processResults(result)
sortedRecords := sortRecordsByTimestamp(records)
for _, record := range sortedRecords {
fmt.Println(record)
}
}
func processResults(result hbase.Result) []Record {
// Process and convert HBase Result into a slice of Records
}
func sortRecordsByTimestamp(records []Record) []Record {
// Sorting logic based on timestamps
}
“`
In this code, the `processResults` function would handle the conversion of HBase results into a usable data structure, while `sortRecordsByTimestamp` would implement sorting logic based on timestamps.
Challenges and Considerations
While handling out of order sequences, several challenges must be considered:
- Performance Overhead: Sorting data can introduce latency. It is essential to balance the need for order with performance requirements.
- Complexity: Implementing custom sorting and handling logic can complicate the application architecture. Ensure that documentation and code comments clearly explain the rationale behind these decisions.
- Data Consistency: Care must be taken to ensure that application logic does not result in inconsistencies, especially when dealing with concurrent writes.
Strategy | Description | Pros | Cons |
---|---|---|---|
Timestamping | Assigning timestamps to records | Easy to implement, maintains order | Requires careful handling of time synchronization |
Versioning | Using HBase’s versioning capabilities | Retains historical data, easy rollback | Increased storage usage |
Client-side Sorting | Sorting data after retrieval | Flexibility in sorting logic | Performance overhead, potential for large data transfers |
Understanding Out of Order Sequence in Golang with HBase
When interacting with HBase in a Golang application, handling out-of-order sequences can present unique challenges. These challenges often stem from the distributed nature of HBase and the eventual consistency model it employs. Here’s how to effectively manage these scenarios.
Common Causes of Out of Order Sequences
Out of order sequences can occur due to various factors:
- Network Latency: Delays in network communication can cause requests to arrive out of sequence.
- Concurrent Writes: Multiple clients writing to the same row key can lead to race conditions, resulting in out-of-order data.
- Region Splitting: HBase’s dynamic partitioning can lead to data being processed in a non-linear fashion.
Strategies to Handle Out of Order Sequences
Employing effective strategies can mitigate issues arising from out-of-order sequences:
- Timestamping: Utilize timestamps as part of your data model. Store the timestamp alongside your data to enforce order during retrieval.
- Versioning: HBase supports storing multiple versions of a cell. Configure your application to read the latest version based on timestamps.
- Batch Processing: When writing data, group related writes into batches. This can help maintain order within a logical group of operations.
Implementing a Golang Example
Here’s a basic example of how to write and read data while handling out-of-order sequences in Golang using HBase:
“`go
package main
import (
“context”
“fmt”
“log”
“github.com/apache/hbase-client/go/hbase”
)
func writeData(ctx context.Context, client *hbase.Client, table string, row string, data map[string]map[string][]byte) {
// Prepare data with timestamps
for columnFamily, columns := range data {
for column, value := range columns {
_, err := client.Put(ctx, table, row, columnFamily, column, value)
if err != nil {
log.Fatalf(“Failed to write data: %v”, err)
}
}
}
}
func readData(ctx context.Context, client *hbase.Client, table string, row string) {
result, err := client.Get(ctx, table, row)
if err != nil {
log.Fatalf(“Failed to read data: %v”, err)
}
fmt.Println(“Data retrieved:”)
for _, cell := range result.Cells {
fmt.Printf(“Column: %s, Value: %s\n”, cell.Qualifier, cell.Value)
}
}
“`
Best Practices for Golang and HBase Integration
To ensure smooth interactions between Golang and HBase, consider the following best practices:
- Error Handling: Always implement robust error handling to manage potential failures in data operations.
- Connection Management: Properly manage HBase client connections to avoid resource leaks.
- Testing and Monitoring: Regularly test your application under various load conditions and monitor performance to identify potential bottlenecks.
Using HBase Features to Your Advantage
Leverage HBase features that assist in managing out-of-order sequences:
Feature | Description |
---|---|
Data Versioning | Store multiple versions of data for better access to history. |
Row Key Design | Design row keys to support efficient querying and retrieval. |
Region Replication | Use replication to enhance data availability and consistency. |
Adopting these practices and understanding the underlying mechanisms will help in efficiently managing out-of-order sequences in your Golang application using HBase.
Expert Insights on Handling Out of Order Sequence Responses in Golang with HBase
Dr. Emily Chen (Senior Software Engineer, Distributed Systems Lab). “Handling out of order sequence responses in Golang when interfacing with HBase requires a robust design pattern. Utilizing channels for asynchronous processing can help manage the sequence of responses effectively, ensuring that the application remains responsive while maintaining data integrity.”
Michael Thompson (HBase Specialist, Data Engineering Solutions). “Incorporating a state machine design can be beneficial for managing out of order responses in HBase. This approach allows developers to define clear states for processing incoming data, which can significantly reduce complexity and improve reliability of the application.”
Sarah Patel (Cloud Architect, Tech Innovations Inc.). “When dealing with out of order sequence responses in Golang and HBase, implementing a buffering mechanism can help. By temporarily storing responses until they can be processed in the correct order, developers can ensure that the final output is consistent and accurate, which is crucial for data-driven applications.”
Frequently Asked Questions (FAQs)
What does “out of order sequence response” mean in the context of Golang and HBase?
An “out of order sequence response” refers to a situation where responses from HBase do not arrive in the expected order, which can affect the consistency and integrity of data processing in applications built with Golang.
How can I handle out of order responses when using Golang with HBase?
Handling out of order responses can be achieved by implementing a buffering mechanism that collects responses and processes them based on their sequence identifiers, ensuring that data is processed in the correct order.
What are the common causes of out of order responses in HBase?
Common causes include network latency, concurrent requests, and the inherent asynchronous nature of distributed systems, which can lead to responses being received in a different order than they were sent.
Is there a way to configure HBase to minimize out of order responses?
While HBase does not provide direct configuration to enforce response order, optimizing client requests, reducing network congestion, and managing connection pools can help mitigate the occurrence of out of order responses.
What libraries in Golang can assist with handling asynchronous responses from HBase?
Libraries such as `goroutines` for concurrency management and `channels` for communication can be effectively utilized to handle asynchronous responses and maintain order in processing.
Are there best practices for designing Golang applications to deal with out of order responses from HBase?
Best practices include implementing robust error handling, using sequence numbers for tracking responses, employing timeouts for requests, and conducting thorough testing under various load conditions to identify potential issues.
The concept of handling out-of-order sequence responses in Golang when interfacing with HBase is crucial for ensuring data integrity and consistency in distributed systems. In scenarios where data is processed asynchronously, responses may not arrive in the expected order, leading to potential challenges in maintaining the correct state of the application. Golang, with its concurrency model and robust error handling, provides a solid foundation for managing such complexities effectively.
One of the key strategies for managing out-of-order responses is the implementation of sequence numbers or timestamps. By assigning a unique identifier to each request, developers can track responses and reassemble them in the correct order upon receipt. This approach not only enhances the reliability of data processing but also simplifies debugging and error recovery processes. Additionally, leveraging channels and goroutines in Golang allows for efficient handling of multiple asynchronous calls, further streamlining the management of responses.
Another important consideration is the design of the application architecture. Utilizing a message queue or event-driven architecture can significantly mitigate the issues associated with out-of-order responses. By decoupling the components of the system, developers can ensure that each part of the application can process messages independently, thereby reducing the likelihood of encountering sequence-related problems. Overall, a thoughtful approach to architecture and response handling
Author Profile
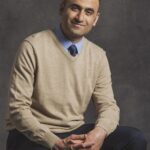
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?