How Can You Remove Rows with Conditions in Pandas?
In the world of data analysis, the ability to manipulate and clean datasets is crucial for deriving meaningful insights. One powerful tool in the Python ecosystem that has become synonymous with data manipulation is the Pandas library. With its intuitive syntax and robust functionality, Pandas allows analysts and data scientists to efficiently handle large datasets, making it easier to extract valuable information. Among the many tasks that Pandas can perform, removing rows based on specific conditions stands out as a fundamental operation that can significantly enhance the quality of your data.
When working with datasets, it’s not uncommon to encounter rows that contain irrelevant, erroneous, or incomplete information. These unwanted entries can skew your analysis and lead to misleading conclusions. Fortunately, Pandas provides several methods to filter out these problematic rows based on defined criteria. Whether you’re looking to remove duplicates, filter out outliers, or eliminate entries that do not meet certain conditions, mastering this aspect of Pandas will empower you to maintain the integrity of your data.
In this article, we will explore the various techniques available in Pandas for removing rows with specific conditions. From simple boolean indexing to more complex filtering functions, you’ll learn how to streamline your data cleaning process. By the end, you’ll be equipped with the knowledge to enhance your data preparation workflow, ensuring that your analyses are
Pandas DataFrame Row Removal Based on Conditions
To remove rows from a Pandas DataFrame based on specific conditions, you can utilize boolean indexing. This method allows you to filter the DataFrame by applying a condition that evaluates to `True` or “. The rows that evaluate to “ can be effectively removed from the DataFrame.
The basic syntax for removing rows is:
“`python
df = df[condition]
“`
Where `condition` is a boolean expression that determines which rows should be kept.
Examples of Row Removal
Here are some common scenarios where you might want to remove rows based on certain conditions:
– **Removing rows based on a single condition**: For instance, if you have a DataFrame containing student scores and wish to remove all students with scores below a certain threshold.
“`python
df = df[df[‘score’] >= 50]
“`
– **Removing rows based on multiple conditions**: You can also combine multiple conditions using the logical operators `&` (and) and `|` (or).
“`python
df = df[(df[‘score’] >= 50) & (df[‘attendance’] >= 75)]
“`
– **Removing rows using the `query` method**: This method provides a more readable way to apply conditions.
“`python
df = df.query(‘score >= 50 and attendance >= 75’)
“`
Using the `drop` Method
Another approach to remove rows is by using the `drop` method. This is particularly useful when you know the index of the rows you want to remove.
“`python
df = df.drop(index=rows_to_remove)
“`
You can identify `rows_to_remove` based on conditions. For example, to drop rows where the score is less than 50:
“`python
rows_to_remove = df[df[‘score’] < 50].index
df = df.drop(index=rows_to_remove)
```
Example DataFrame
Consider the following DataFrame as an example:
Name | Score | Attendance |
---|---|---|
Alice | 75 | 80 |
Bob | 45 | 90 |
Charlie | 60 | 70 |
David | 30 | 85 |
To remove students with scores less than 50, you can execute:
“`python
df = df[df[‘score’] >= 50]
“`
This will result in a DataFrame containing only Alice and Charlie.
Using these methods, you can efficiently manage and clean your DataFrame by removing unnecessary or irrelevant rows based on your criteria. Whether you use boolean indexing or the `drop` method, Pandas offers flexible options to suit your data manipulation needs.
Removing Rows Based on Conditions in Pandas
In Pandas, removing rows based on specific conditions can be efficiently accomplished using boolean indexing. This method allows users to filter DataFrames by evaluating conditions on one or more columns.
Basic Syntax for Removing Rows
To remove rows that meet a certain condition, you can use the `drop()` method in combination with boolean indexing. Here’s a general format:
“`python
df = df[condition]
“`
Where `condition` is a boolean expression that evaluates to `True` or “. Rows corresponding to “ will be kept in the DataFrame.
Examples of Removing Rows
- **Removing Rows Based on a Single Condition**
To remove rows where a specific column meets a condition, you can do the following:
“`python
import pandas as pd
Sample DataFrame
data = {‘A’: [1, 2, 3, 4], ‘B’: [5, 6, 7, 8]}
df = pd.DataFrame(data)
Remove rows where column ‘A’ is less than 3
df = df[df[‘A’] >= 3]
“`
- **Removing Rows Based on Multiple Conditions**
You can combine conditions using logical operators (`&` for AND, `|` for OR):
“`python
Remove rows where column ‘A’ is less than 3 or column ‘B’ is greater than 6
df = df[(df[‘A’] >= 3) & (df[‘B’] <= 6)]
```
- **Using the `query()` Method**
The `query()` method provides a more readable way to filter DataFrames:
“`python
df = df.query(‘A >= 3 and B <= 6')
```
Removing Rows with `drop()` Method
The `drop()` method can be employed to remove rows by their index. If you have identified the indices of the rows to drop, you can execute:
“`python
Dropping specific rows by index
df = df.drop(index=[0, 1]) Drops the first two rows
“`
For conditions that need to be evaluated before dropping, first filter the DataFrame to obtain the indices:
“`python
indices_to_drop = df[df[‘A’] < 3].index
df = df.drop(index=indices_to_drop)
```
In-Place Removal of Rows
If you wish to modify the original DataFrame without creating a new one, you can utilize the `inplace` parameter:
“`python
df.drop(index=indices_to_drop, inplace=True)
“`
This approach saves memory and can be more efficient when working with large DataFrames.
Practical Considerations
When removing rows:
- Check for NaN values: Ensure that your conditions account for any missing values, which can affect filtering.
- Data Integrity: Always verify that the removal of rows does not compromise the integrity of your dataset, especially when working with time series data or related datasets.
- Performance: For very large DataFrames, consider using optimized methods or chunk processing to improve performance.
By leveraging these techniques, users can effectively manage and manipulate their DataFrames in Pandas, ensuring data cleanliness and relevance for subsequent analyses.
Expert Insights on Removing Rows with Conditions in Pandas
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “When working with large datasets, efficiently removing rows based on specific conditions is crucial. Utilizing the `DataFrame.drop` method in conjunction with boolean indexing allows for precise and effective data cleaning, ultimately enhancing the quality of your analysis.”
Michael Patel (Senior Data Analyst, Analytics Solutions Group). “In my experience, employing the `DataFrame.loc` method combined with conditions provides a straightforward approach to filter out unwanted rows. This technique not only maintains the integrity of the original dataset but also ensures that subsequent analyses are based on relevant data.”
Laura Kim (Machine Learning Engineer, Data Insights Lab). “Removing rows with specific conditions in Pandas is an essential step in preprocessing data for machine learning models. I recommend using the `query` method for its readability and efficiency, which can significantly streamline the workflow when handling complex datasets.”
Frequently Asked Questions (FAQs)
How can I remove rows from a Pandas DataFrame based on a condition?
You can use boolean indexing to filter out rows that meet a specific condition. For example, `df = df[df[‘column_name’] != value]` will remove rows where ‘column_name’ equals ‘value’.
What method can I use to drop rows with missing values in Pandas?
You can use the `dropna()` method to remove rows with any missing values. For instance, `df.dropna(inplace=True)` will remove all rows containing NaN values in the DataFrame.
Can I remove rows based on multiple conditions in Pandas?
Yes, you can combine multiple conditions using logical operators. For example, `df = df[(df[‘col1’] != value1) & (df[‘col2’] > value2)]` removes rows based on both conditions.
Is it possible to remove rows using the `query()` method in Pandas?
Yes, the `query()` method allows you to filter rows based on a string expression. For example, `df = df.query(‘column_name != value’)` will remove rows where ‘column_name’ equals ‘value’.
What happens to the index when I remove rows from a DataFrame?
When you remove rows, the original index is retained. To reset the index after dropping rows, use `df.reset_index(drop=True, inplace=True)`.
Can I remove rows with specific values in multiple columns simultaneously?
Yes, you can use the `isin()` method to remove rows with specific values in multiple columns. For instance, `df = df[~df[‘column_name’].isin([value1, value2])]` will drop rows where ‘column_name’ matches either value.
In the realm of data manipulation using the pandas library in Python, removing rows based on specific conditions is a fundamental operation. The ability to filter data effectively allows users to clean datasets and focus on relevant information. Common methods for achieving this include using boolean indexing, the `drop()` method, and the `query()` function. Each of these approaches offers flexibility and efficiency, catering to different use cases and preferences among data analysts and scientists.
One of the most straightforward methods is boolean indexing, where conditions are applied directly to the DataFrame to create a mask that filters out unwanted rows. This method is particularly intuitive and allows for complex conditions to be combined using logical operators. Alternatively, the `drop()` method can be employed, which is useful when you want to remove rows by index labels. The `query()` function provides a more readable syntax for filtering, especially when dealing with multiple conditions, making it an excellent choice for those who prefer a more SQL-like approach.
In summary, pandas offers a variety of methods for removing rows based on conditions, each with its own advantages. Understanding these methods enhances a user’s ability to manage and manipulate data effectively. By leveraging these techniques, data professionals can ensure their datasets are clean, relevant, and ready for
Author Profile
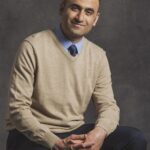
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?