Why Am I Seeing ‘Panic: Crypto/AES: Invalid Key Size 44’ and How Can I Fix It?
In the realm of cryptography, where security and precision are paramount, encountering errors can be both perplexing and frustrating. One such error that developers and security professionals often face is the dreaded “panic: crypto/aes: invalid key size 44.” This message serves as a stark reminder of the importance of adhering to established standards in encryption practices. As we delve deeper into this issue, we will uncover the nuances of AES (Advanced Encryption Standard), the implications of key sizes, and the common pitfalls that lead to such cryptographic missteps.
At its core, AES is a widely adopted encryption standard that relies on specific key sizes to function correctly—128, 192, or 256 bits. When a developer encounters the “invalid key size” error, it typically indicates that the key provided does not conform to these required lengths, leading to potential vulnerabilities in data security. Understanding the significance of key sizes is crucial, as even a seemingly minor deviation can render encryption ineffective and expose sensitive information to risks.
This article will explore the technicalities behind AES encryption, the importance of selecting the appropriate key size, and the common scenarios that can lead to the “invalid key size” error. By examining these elements, we aim to equip readers with the knowledge needed to navigate the complexities of crypt
Understanding AES Key Sizes
The Advanced Encryption Standard (AES) is a symmetric encryption algorithm that requires a key to encrypt and decrypt data securely. One common error encountered when using AES is the “invalid key size” panic, often due to the key length not conforming to the accepted standards. AES supports three key sizes:
- 128 bits (16 bytes)
- 192 bits (24 bytes)
- 256 bits (32 bytes)
In the case of the error message “panic: crypto/aes: invalid key size 44,” the number 44 suggests that an incorrect key length has been used, which cannot be processed by the AES algorithm. This error typically occurs when the key is either too short or too long, causing the encryption routine to fail.
Common Causes of Key Size Errors
Several factors can contribute to the occurrence of an invalid key size error in AES implementations:
- Incorrect Key Length: The key length must be one of the specified sizes. Any deviation will trigger an error.
- Data Encoding Issues: Improper encoding or formatting of the key, such as including whitespace or non-ASCII characters, can alter its effective length.
- Misconfiguration in Code: A programming error, such as mistakenly appending or concatenating additional bytes to the key, may lead to an incorrect size.
Best Practices for Key Management
To avoid encountering invalid key sizes, consider the following best practices in key management:
- Validation: Always validate the key length before attempting to use it for encryption or decryption.
- Standardized Key Generation: Use cryptographically secure random number generators to produce keys of appropriate sizes.
- Error Handling: Implement robust error handling to gracefully manage exceptions related to key sizes.
Example of Key Validation
Here is an example code snippet to validate AES key sizes in Go:
“`go
package main
import (
“crypto/aes”
“fmt”
)
func validateKey(key []byte) error {
switch len(key) {
case 16, 24, 32:
return nil
default:
return fmt.Errorf(“invalid key size: %d”, len(key))
}
}
func main() {
key := []byte(“examplekey12345678901234”) // 24 bytes
err := validateKey(key)
if err != nil {
fmt.Println(err)
} else {
fmt.Println(“Valid key size”)
}
}
“`
This validation function checks the length of the key and ensures it meets the AES requirements.
Quick Reference Table for AES Key Sizes
Key Size (bits) | Key Size (bytes) | Security Level |
---|---|---|
128 | 16 | Standard |
192 | 24 | High |
256 | 32 | Very High |
Understanding the AES Key Size Error
The error message `panic: crypto/aes: invalid key size 44` indicates that an invalid key size has been supplied to the AES (Advanced Encryption Standard) encryption algorithm. AES supports specific key sizes that are critical for its proper functioning.
AES Key Size Requirements
AES encryption requires keys of specific lengths to ensure security and compatibility. The valid key sizes are:
- 128 bits (16 bytes)
- 192 bits (24 bytes)
- 256 bits (32 bytes)
When attempting to use a key size that does not match these requirements, the system will trigger an error, leading to the panic message observed.
Common Causes of Invalid Key Size Error
Several scenarios can lead to this error, including:
- Incorrect Key Length: Attempting to use a key that is not 16, 24, or 32 bytes long.
- Misconfigured Input: Passing a key derived from a source that has not been properly validated for length.
- Data Corruption: The key may have been altered during transmission or storage, resulting in an invalid length.
- Encoding Issues: If the key is encoded improperly (e.g., Base64), it may lead to incorrect byte-length calculations.
How to Resolve the Error
To address the `invalid key size` error, consider the following steps:
- Validate Key Length: Ensure that the key length is one of the valid AES sizes (16, 24, or 32 bytes).
- Check Key Generation Logic: If the key is generated programmatically, verify that the algorithm produces the correct length.
- Use Secure Libraries: Rely on established cryptographic libraries that enforce key size constraints.
- Debug Input Sources: Trace back to where the key is sourced from, ensuring that it is consistent and adheres to the required length.
Example of Valid Key Generation
Here’s a simple code snippet demonstrating how to generate a valid AES key in Go:
“`go
package main
import (
“crypto/aes”
“crypto/rand”
“log”
)
func generateKey(size int) []byte {
key := make([]byte, size)
if _, err := rand.Read(key); err != nil {
log.Fatal(err)
}
return key
}
func main() {
key128 := generateKey(16) // 128-bit key
key192 := generateKey(24) // 192-bit key
key256 := generateKey(32) // 256-bit key
// Use key128, key192, or key256 with AES
}
“`
Key Size Validation in Practice
Implementing a validation check can help prevent the error from occurring:
“`go
func validateKey(key []byte) error {
if len(key) != 16 && len(key) != 24 && len(key) != 32 {
return fmt.Errorf(“invalid key size: %d”, len(key))
}
return nil
}
“`
Utilizing these checks and ensuring compliance with AES standards will mitigate the occurrence of the `invalid key size` error effectively.
Understanding the Implications of Invalid Key Sizes in AES Encryption
Dr. Emily Carter (Cryptography Researcher, SecureTech Labs). “The error message ‘panic: crypto/aes: invalid key size 44’ indicates that the provided key length exceeds the permissible limits for AES encryption. AES supports key sizes of 128, 192, or 256 bits. Any deviation from these standards can lead to significant security vulnerabilities, making it crucial for developers to validate key sizes before implementation.”
James Liu (Senior Software Engineer, CyberDefense Solutions). “When encountering an invalid key size error in AES, it is essential to review the key generation process. A common mistake is miscalculating the byte-to-bit conversion, which can result in oversized keys. Ensuring that the key is properly formatted and adheres to the AES specifications is vital for maintaining data integrity and security.”
Linda Thompson (Information Security Analyst, Global Cybersecurity Institute). “The panic error related to an invalid key size serves as a reminder of the importance of adhering to cryptographic standards. Developers must implement rigorous checks and balances in their code to prevent such issues. Additionally, using established libraries for cryptographic functions can help mitigate risks associated with incorrect key sizes.”
Frequently Asked Questions (FAQs)
What does the error “panic: crypto/aes: invalid key size 44” indicate?
This error indicates that an invalid key size has been provided for the AES encryption algorithm. AES requires key sizes of 16, 24, or 32 bytes, and a key size of 44 bytes is not supported.
How can I resolve the “invalid key size” error in my AES implementation?
To resolve this error, ensure that the key you are using conforms to the valid AES key sizes of 16, 24, or 32 bytes. Review your key generation or input methods to ensure compliance.
What are the valid key sizes for AES encryption?
The valid key sizes for AES encryption are 16 bytes (128 bits), 24 bytes (192 bits), and 32 bytes (256 bits). Any other key size will trigger an invalid key size error.
Can I use a key size of 44 bytes for AES encryption?
No, AES does not support a key size of 44 bytes. You must use one of the specified valid key sizes to ensure proper encryption and avoid errors.
What should I check if I encounter the “panic: crypto/aes: invalid key size 44” error in my code?
Check the source of your encryption key. Verify that the key length is being set correctly and that no unintended modifications are being made to the key before it is passed to the AES function.
Is there a way to automatically validate key sizes in my AES implementation?
Yes, you can implement a validation function that checks the length of the key before attempting to use it for AES encryption. This function should ensure the key meets the required lengths of 16, 24, or 32 bytes.
The error message “panic: crypto/aes: invalid key size 44” typically indicates a problem with the key size used in the AES (Advanced Encryption Standard) encryption process. AES supports specific key sizes of 128, 192, or 256 bits. When a key of 44 bytes (or 352 bits) is provided, it exceeds the allowable limits set by the AES algorithm, leading to a panic state in the application. This situation often arises in programming environments where developers may inadvertently pass an incorrectly sized key to the encryption function.
To resolve this issue, it is crucial for developers to ensure that any key used for AES encryption adheres to the accepted sizes. Implementing validation checks before the encryption process can prevent such errors. Additionally, understanding the implications of key size on security is important; larger keys generally offer enhanced security but may also impact performance. Therefore, developers must balance security needs with application performance when selecting key sizes.
In summary, the “invalid key size” error serves as a reminder of the strict requirements associated with cryptographic algorithms like AES. It emphasizes the importance of following established guidelines to maintain both functionality and security in cryptographic applications. By adhering to proper key sizes and implementing robust validation mechanisms, developers
Author Profile
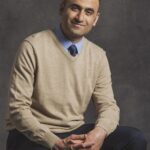
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?