How Can You Pass and Store Information in Shadertoy?
In the ever-evolving world of computer graphics, ShaderToy stands out as a vibrant platform where creativity meets technical prowess. This online community allows artists and developers to craft stunning visual effects using GLSL (OpenGL Shading Language) in real-time, pushing the boundaries of what can be achieved on the web. However, as projects grow in complexity, the need for efficient data management becomes paramount. Understanding how to effectively pass and store information within ShaderToy not only enhances the functionality of your shaders but also unlocks new possibilities for dynamic visual storytelling.
Passing and storing information in ShaderToy is a fundamental aspect that enables developers to create intricate and interactive graphics. At its core, ShaderToy allows users to share data between different shader passes, facilitating the creation of layered visual effects. This capability is crucial for managing state and maintaining continuity across frames, especially in projects that require real-time interaction or complex animations. By leveraging various techniques, such as utilizing textures or uniforms, developers can effectively handle data flow and optimize performance.
Moreover, the art of data management in ShaderToy extends beyond mere storage. It involves understanding how to manipulate and retrieve information efficiently, ensuring that shaders remain responsive and visually compelling. Whether you’re aiming to create a mesmerizing particle system or a reactive environment, mastering these
Passing Data Between Shaders
In Shadertoy, passing data between shaders is accomplished through the use of textures and buffers. Each shader can write to a texture, which can then be read by another shader in the subsequent frame. This technique allows for complex interactions and the storage of information across frames.
- Textures: Used to store data that can be accessed by shaders.
- Buffers: Offer a more advanced way to manage and manipulate data, allowing for greater flexibility.
When working with textures, it is crucial to understand the following:
- The format of the texture (e.g., RGBA, RGB).
- The resolution of the texture, which can affect performance and detail.
- The method of sampling the texture to retrieve data in the next shader.
Storing Information Using Textures
Textures in Shadertoy can be utilized to store various types of information, such as colors, depth values, or even arbitrary data. To effectively store information, the following approaches can be employed:
- Render to Texture: Use the output of one shader as the input for another by rendering to a texture.
- Framebuffers: Create multiple textures to manage different types of data simultaneously.
The following table summarizes the key characteristics of textures in Shadertoy:
Texture Type | Description | Use Cases |
---|---|---|
Color Texture | Stores color data in RGBA format. | Rendering images, effects. |
Depth Texture | Stores depth information for 3D effects. | Shadow mapping, depth-based effects. |
Data Texture | Stores arbitrary data, often in a normalized format. | Physics simulations, procedural generation. |
To render to a texture, one must utilize the `mainImage` function to output the desired information. This output can then be directed into a texture by using the `iChannel` inputs in subsequent shaders.
Performance Considerations
When passing and storing information, performance is a critical factor. Key considerations include:
- Texture Resolution: Higher resolutions provide more detail but at the cost of performance.
- Sampling Frequency: Frequent sampling from textures can slow down execution.
- Memory Usage: Multiple textures can consume significant GPU memory, impacting performance.
Optimizing these factors can lead to smoother performance and more visually appealing results.
Passing Information Between Shaders
In Shadertoy, one can pass information between different shaders using various techniques, including uniforms, buffers, and textures. Each method has its own use cases and limitations.
- Uniforms: These are constant values that can be passed to a shader at render time. They are useful for values that do not change per frame.
- Example usage: `uniform float time;`
- Buffers: Shadertoy allows the use of image buffers to store and pass information. Buffers can be used to read data from one shader and write to another.
- Usage involves:
- Creating a buffer with the `buffer` keyword.
- Reading from it in a subsequent shader using `imageLoad()`.
- Textures: Textures can be used to store and retrieve data. They allow for more complex data structures compared to uniforms and buffers.
- Textures can be sampled using functions like `texture()`.
Storing Information in Shadertoy
Storing information effectively in Shadertoy is crucial for creating dynamic and interactive visual content. The following methods can be employed for storing data:
- Image Buffers: These are the primary method for storing intermediate results or state information across frames.
- Setup:
- Define a buffer in the shader.
- Use `fragColor` to output data to the buffer.
- Global Variables: While not persistent across frames, global variables can store temporary state information during a single frame render.
- Textures: Textures can also be utilized to hold data that needs to be accessed in different shaders. This is particularly useful for storing colors, normals, or other attributes.
Storage Method | Description | Use Case |
---|---|---|
Uniforms | Constant values for a shader | Static parameters like color or time |
Buffers | Dynamic data storage across frames | Particle systems or physics simulations |
Textures | Multi-dimensional data storage | Complex visual effects requiring sampling |
Example: Using Buffers to Store and Pass Data
To demonstrate how to use buffers for passing and storing information, consider the following example that implements a simple particle system.
- Shader 1 (Particle Generation):
- Create a buffer to store particle positions.
- Generate new particles based on some criteria and store their positions.
“`glsl
void mainImage( out vec4 fragColor, in vec2 fragCoord ) {
vec2 uv = fragCoord / iResolution.xy;
vec4 particle = vec4(uv, 0.0, 1.0);
fragColor = particle;
imageStore(iChannel0, ivec2(fragCoord), particle);
}
“`
- Shader 2 (Rendering Particles):
- Read from the buffer created in Shader 1 to visualize the particles.
“`glsl
void mainImage( out vec4 fragColor, in vec2 fragCoord ) {
vec4 particle = imageLoad(iChannel0, ivec2(fragCoord));
fragColor = particle; // Render the particle
}
“`
By utilizing these methods, Shadertoy users can create complex visual effects that require both passing and storing information across different shaders. This flexibility allows for a wide range of creative applications in real-time graphics.
Expert Insights on Passing and Storing Information in Shadertoy
Dr. Elena Torres (Graphics Programmer, Interactive Media Institute). “In Shadertoy, passing and storing information effectively relies on utilizing textures and buffers. By leveraging framebuffer objects (FBOs), users can store intermediate results and create complex visual effects that enhance the overall rendering pipeline.”
Marcus Chen (Shader Development Specialist, Visual Effects Studio). “To optimize performance in Shadertoy, it is essential to understand how to manage data flow between shaders. Using uniform variables for passing information allows for efficient updates without the need for redundant calculations, thereby streamlining the rendering process.”
Linda Patel (Computer Graphics Researcher, University of Technology). “The use of global variables in Shadertoy can simplify the process of storing state information across frames. However, developers should be cautious of the limitations in precision and scope, ensuring that their implementations do not lead to unintended side effects in the rendering output.”
Frequently Asked Questions (FAQs)
How can I pass information between different shaders in Shadertoy?
You can pass information between shaders in Shadertoy using texture buffers. By rendering data to a texture in one shader, you can sample that texture in another shader, allowing for data transfer.
What are the methods for storing data in Shadertoy?
Data can be stored in Shadertoy using various techniques, including using textures, uniform variables, or the built-in buffer system. Textures are commonly used for storing pixel data, while uniforms can hold scalar or vector values.
Can I use multiple textures to store different types of information?
Yes, you can utilize multiple textures to store various types of information. Each texture can represent different data, such as color, depth, or any custom information, which can then be accessed in your shaders.
What is the role of the buffer system in Shadertoy?
The buffer system in Shadertoy allows you to create and manipulate multiple render targets. It enables you to store intermediate results and use them in subsequent frames or passes, facilitating complex visual effects and data processing.
How do I read data from a texture in a shader?
To read data from a texture in a shader, you can use the `texture()` function. This function samples the texture at specified coordinates and returns the color or value stored at that location.
Is it possible to dynamically update stored information in Shadertoy?
Yes, it is possible to dynamically update stored information in Shadertoy. By rendering to a texture in one frame and using it in the next, you can create animations or effects that change over time based on user input or other parameters.
In the context of Shadertoy, passing and storing information is crucial for creating complex visual effects and interactive graphics. Shadertoy utilizes GLSL (OpenGL Shading Language) to manage data flow between different shader stages. Information can be passed using textures, uniforms, and varying variables, allowing for a flexible approach to rendering and computation. Textures serve as a primary means of storing data, enabling shaders to read and write information efficiently, which is essential for achieving dynamic visual outputs.
Moreover, the use of framebuffers in Shadertoy facilitates the storage of intermediate results, allowing shaders to render scenes in multiple passes. This technique is particularly useful for effects such as post-processing, where the output of one shader can be utilized as input for another. By leveraging these methods, developers can create intricate visual effects that respond to user interactions or procedural algorithms, enhancing the overall experience of the shader.
In summary, understanding the mechanisms of passing and storing information in Shadertoy is vital for developers aiming to create sophisticated graphics. Mastery of these concepts not only improves the efficiency of shader programs but also expands creative possibilities. As users experiment with different techniques for data management, they can unlock new dimensions in their visual creations,
Author Profile
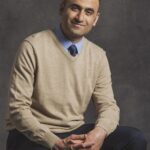
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?