How Can I Resolve PHP Timezone Mismatch with Server Timezone?
In the world of web development, time is more than just a number; it’s a critical element that can affect everything from user experience to data integrity. When working with PHP, developers often encounter the perplexing issue of timezone mismatches between their server and application. This seemingly minor discrepancy can lead to a cascade of problems, including incorrect timestamps, scheduling errors, and even data inconsistencies. Understanding how to navigate these timezone challenges is essential for anyone looking to build robust, reliable applications that operate seamlessly across different regions and user bases.
Timezone mismatches can arise from various factors, including server configurations, PHP settings, and even user preferences. When a PHP application does not align with the server’s timezone, it can result in unexpected behavior, especially for time-sensitive functionalities like logging, scheduling, and displaying dates. Developers must be proactive in addressing these discrepancies to ensure that their applications run smoothly and provide accurate information to users, regardless of their geographic location.
To effectively manage timezone settings in PHP, it’s crucial to grasp how PHP handles timezones and the tools available for adjusting them. From utilizing built-in functions to configuring server settings, there are several strategies developers can employ to mitigate the risks associated with timezone mismatches. In the following sections, we will delve deeper into the causes
Understanding Timezone Mismatch in PHP
Timezone mismatch issues in PHP often arise when the server’s timezone configuration does not align with the application’s expected timezone. This discrepancy can lead to various problems, particularly with date and time functions, resulting in incorrect timestamps or unexpected behavior in applications.
To diagnose a timezone mismatch, it is essential to check both the server’s timezone settings and the timezone configuration within the PHP environment. You can retrieve the server’s timezone using the following command in a terminal:
“`bash
date
“`
This command will display the current system time along with the timezone. Additionally, you can check the PHP configuration for the timezone by using the `phpinfo()` function or checking the `php.ini` file.
Common PHP Timezone Settings
In PHP, the timezone can be set in several ways:
- php.ini Configuration: Modify the `date.timezone` directive.
Example:
“`ini
date.timezone = “America/New_York”
“`
- Runtime Configuration: Use the `date_default_timezone_set()` function in your scripts.
Example:
“`php
date_default_timezone_set(‘America/New_York’);
“`
- Environment Variable: Set the timezone in the server environment.
Each method serves different needs, but it’s critical to ensure consistency across the application.
Checking and Setting Timezones
When diagnosing and correcting timezone mismatches, follow these steps:
- Check Server Timezone:
- Use the terminal command mentioned earlier.
- Check PHP Timezone:
- Create a PHP file with the following content:
“`php
“`
- Access this file via a web browser to review the timezone settings.
- Set the Correct Timezone:
- Choose the appropriate method (php.ini, runtime, or environment variable) to set the desired timezone.
Timezone Database and Available Timezones
PHP uses the IANA Time Zone Database for timezone names. Here’s a brief list of common timezone identifiers:
Identifier | Description |
---|---|
America/New_York | Eastern Standard Time (EST) |
America/Los_Angeles | Pacific Standard Time (PST) |
Europe/London | Greenwich Mean Time (GMT) |
Asia/Tokyo | Japan Standard Time (JST) |
Australia/Sydney | Australian Eastern Daylight Time (AEDT) |
To get a full list of available timezones in PHP, you can use the following code snippet:
“`php
$timezones = DateTimeZone::listIdentifiers();
foreach ($timezones as $timezone) {
echo $timezone . “\n”;
}
“`
This will display all the available timezones that you can set in your PHP applications.
Handling Timezone in Applications
When working with time-sensitive applications, it is crucial to handle timezones properly. Here are some best practices:
- Always store timestamps in UTC in the database.
- Convert timestamps to the user’s timezone when displaying them.
- Use reliable libraries like DateTime or Carbon for managing dates and times effectively.
- Regularly review and update timezone settings as needed, especially during daylight saving time changes.
By following these guidelines, you can mitigate timezone-related issues and ensure that your PHP application operates smoothly across different regions.
Understanding Timezone Mismatches
Timezone mismatches occur when the timezone settings of the PHP application do not align with the server’s timezone settings. This discrepancy can lead to incorrect date and time information, impacting functionalities such as logging, scheduling, and user interactions.
Common sources of timezone mismatches include:
- Default timezone settings in PHP
- Server configuration settings
- Database timezone settings
- User-specific timezone configurations
Identifying Your Current PHP Timezone
To determine the current timezone set in your PHP environment, you can use the following code snippet:
“`php
echo date_default_timezone_get();
“`
This command will return the default timezone that PHP is currently using. It is essential to compare this with the server’s timezone to identify any mismatches.
Configuring PHP Timezone
You can set the timezone in PHP using the `date_default_timezone_set()` function. This can be done in the PHP configuration file (`php.ini`) or directly in your script.
Example of setting the timezone in a script:
“`php
date_default_timezone_set(‘America/New_York’);
“`
Alternatively, modify the `php.ini` file:
“`ini
date.timezone = “America/New_York”
“`
After making changes, ensure to restart your web server for the new settings to take effect.
Checking Server Timezone
To verify the server’s timezone, you can execute the following command in the terminal:
“`bash
date
“`
This command will display the current server time along with the timezone. Ensure that this matches your PHP configuration to avoid discrepancies.
Database Timezone Considerations
If your application interacts with a database, ensure that the database timezone is configured correctly. Common databases like MySQL allow you to set the timezone using:
“`sql
SET time_zone = ‘America/New_York’;
“`
Table: Common Timezone Settings
System | Configuration Method | Example Setting |
---|---|---|
PHP | `date_default_timezone_set()` or `php.ini` | `America/New_York` |
Server | Terminal command `date` | Output example: `Wed Sep 22 14:00:00 EDT 2023` |
MySQL | SQL command | `SET time_zone = ‘America/New_York’;` |
Handling User-Specific Timezones
In applications where users may be in different timezones, it is crucial to store user-specific timezone information. Use this data to convert timestamps when displaying date and time values.
- Store user timezones in the database.
- Convert server time to user timezone during data retrieval.
- Use PHP’s `DateTime` object for conversions:
“`php
$date = new DateTime(‘now’, new DateTimeZone(‘UTC’));
$date->setTimezone(new DateTimeZone(‘America/New_York’));
echo $date->format(‘Y-m-d H:i:s’);
“`
Best Practices for Avoiding Timezone Issues
To minimize timezone-related problems:
- Always use UTC for storing timestamps in databases.
- Convert to local time only when displaying data to users.
- Regularly check and synchronize server, PHP, and database timezones.
- Implement error handling to catch and log timezone-related issues.
Resolving PHP Timezone Mismatches: Expert Insights
Dr. Emily Carter (Senior PHP Developer, Tech Innovations Inc.). “A common issue developers face is the mismatch between PHP’s configured timezone and the server’s default timezone. This discrepancy can lead to unexpected behavior in date and time functions, causing confusion in applications. It is crucial to explicitly set the timezone in your PHP scripts using the date_default_timezone_set() function to ensure consistency across different environments.”
Mark Thompson (System Administrator, Cloud Solutions Group). “When deploying PHP applications, it is essential to align the server timezone with the application’s timezone settings. Failing to do so can result in incorrect timestamps in logs and databases. Always verify the server’s timezone configuration and adjust your PHP settings accordingly to prevent these issues from arising.”
Linda Garcia (Web Application Architect, Digital Dynamics). “Timezone mismatches can significantly impact user experience, especially in applications that rely on accurate time tracking. I recommend implementing a robust system for handling timezones, including storing all timestamps in UTC and converting them to the user’s local timezone as needed. This approach minimizes the risk of errors related to timezone discrepancies.”
Frequently Asked Questions (FAQs)
What is a PHP timezone mismatch?
A PHP timezone mismatch occurs when the timezone settings in a PHP application differ from the server’s timezone settings, leading to discrepancies in date and time calculations.
How can I check the current PHP timezone?
You can check the current PHP timezone by using the `date_default_timezone_get()` function in your PHP script, which will return the timezone currently set in your PHP configuration.
How do I set the correct timezone in PHP?
To set the correct timezone in PHP, use the `date_default_timezone_set(‘Your/Timezone’)` function at the beginning of your script, or configure the `date.timezone` directive in your `php.ini` file.
What are the consequences of a timezone mismatch?
Consequences of a timezone mismatch include incorrect timestamps in logs, inaccurate scheduling of events, and potential issues with user data that relies on accurate time representation.
How can I synchronize PHP timezone with server timezone?
To synchronize the PHP timezone with the server timezone, ensure both are set to the same value. This can be done by configuring the server’s timezone and then setting the same timezone in your PHP application using `date_default_timezone_set()`.
Can I override the server timezone in PHP?
Yes, you can override the server timezone in PHP by explicitly setting a different timezone using `date_default_timezone_set()`. However, this may lead to inconsistencies if not managed properly.
In the context of PHP development, a timezone mismatch between the server and the application can lead to significant issues, particularly when dealing with date and time functions. This discrepancy often arises when the server’s default timezone settings do not align with the application’s intended timezone. As a result, timestamps can be inaccurately recorded, leading to confusion in data processing and user interactions.
To address timezone mismatches, developers should prioritize setting the correct timezone within their PHP applications. This can be achieved by utilizing the `date_default_timezone_set()` function, which allows developers to specify the desired timezone explicitly. Additionally, it is crucial to verify the server’s timezone configuration, which can typically be found in the `php.ini` file or through server management tools. Ensuring both the server and application are synchronized in terms of timezone will help mitigate potential errors.
Another important consideration is the impact of timezone mismatches on database operations. When storing and retrieving datetime values, it is advisable to use a consistent timezone, preferably UTC, to avoid discrepancies. This practice not only simplifies the handling of datetime data across different systems but also enhances the reliability of data integrity and consistency.
addressing timezone mismatches is essential for maintaining accurate date and time handling
Author Profile
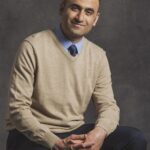
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?