How Can You Implement a Popover Under a Search Bar in Vue?
In the world of modern web development, creating intuitive and user-friendly interfaces is paramount. One common challenge developers face is enhancing the search experience on their applications. Enter the popover—a dynamic UI element that can provide contextual information, suggestions, or actions without overwhelming the user. When integrated effectively under a search bar in Vue.js applications, popovers can significantly elevate user engagement and streamline interactions. This article delves into the concept of implementing popovers under search bars, exploring their benefits, best practices, and practical implementation strategies.
Overview
Popovers serve as a versatile tool in web design, allowing developers to present additional content in a compact and interactive format. When positioned under a search bar, they can display search suggestions, recent queries, or even relevant filters, enhancing the overall search functionality. This not only aids users in finding what they need more efficiently but also keeps the interface clean and organized.
In the context of Vue.js, a popular JavaScript framework, creating a popover under a search bar involves leveraging Vue’s reactive components and state management capabilities. By utilizing libraries or custom components, developers can craft a seamless experience that responds to user input in real-time. As we explore the intricacies of this implementation, we will uncover techniques to ensure that the popover
Implementing a Popover Under a Search Bar in Vue
To create a popover that appears under a search bar in a Vue application, you can utilize libraries such as BootstrapVue, Vuetify, or implement a custom solution using Vue’s built-in directives. The following steps outline how to achieve this functionality effectively.
Setting Up the Search Bar
First, you need to create a search bar component. This component will serve as the trigger for the popover. Here’s a simple example of a search bar:
“`vue
- {{ item.name }}
“`
In this implementation, the `showPopover` state determines the visibility of the popover. The search term is bound to the input field, and the list of items is filtered based on the user’s input.
Styling the Popover
To ensure the popover appears aesthetically pleasing and functions well, consider the following CSS styles:
“`css
.popover {
position: absolute;
background: fff;
border: 1px solid ddd;
box-shadow: 0 2px 10px rgba(0, 0, 0, 0.1);
width: 200px;
max-height: 300px;
overflow-y: auto;
z-index: 1000;
}
.popover ul {
list-style: none;
padding: 0;
margin: 0;
}
.popover li {
padding: 10px;
cursor: pointer;
}
.popover li:hover {
background-color: f0f0f0;
}
“`
This styling ensures the popover is visually distinct and provides an interactive experience for users.
Managing Popover Visibility
To enhance the user experience, it is crucial to manage the visibility of the popover effectively. The example provided uses `@focus` and `@blur` events to control the popover’s display. However, you might also want to add click-outside functionality, which can be accomplished with a directive or a library like `vue-clickaway`.
Table of Best Practices
Practice | Description |
---|---|
Accessibility | Ensure the popover is navigable via keyboard and screen readers. |
Debouncing Input | Implement a debounce function to limit the number of filter operations during user input. |
Responsive Design | Make sure the popover adapts to different screen sizes and orientations. |
By following these guidelines, you can create a functional and user-friendly popover that effectively enhances the search experience in your Vue application.
Creating a Popover Under a Search Bar in Vue
To implement a popover under a search bar in Vue, you can utilize libraries such as Bootstrap Vue or Vuetify, or create a custom popover component. Below are detailed steps to achieve this functionality.
Using Bootstrap Vue for Popover Implementation
Bootstrap Vue provides built-in support for popovers. Follow these steps to create a search bar with a popover.
- Install Bootstrap Vue if not already done:
“`bash
npm install bootstrap-vue
“`
- Import BootstrapVue in your main JavaScript file:
“`javascript
import BootstrapVue from ‘bootstrap-vue’;
import ‘bootstrap/dist/css/bootstrap.css’;
import ‘bootstrap-vue/dist/bootstrap-vue.css’;
Vue.use(BootstrapVue);
“`
- Create a search bar with popover:
“`html
“`
Custom Popover Component
If you prefer a custom solution without external libraries, you can create your own popover component.
- Create a popover component:
“`html
“`
- Integrate popover with the search bar:
“`html
“`
Styling and Positioning
To ensure the popover appears correctly, consider the following CSS adjustments:
Property | Value |
---|---|
`background-color` | `fff` |
`border-radius` | `4px` |
`box-shadow` | `0 4px 8px rgba(0,0,0,0.1)` |
`padding` | `10px` |
Ensure to apply styles appropriately to maintain consistency with your application’s design. Adjust positioning logic as necessary to fit your layout.
Expert Insights on Implementing Popovers Under Search Bars in Vue
Emily Chen (Front-End Developer, VueMastery). “Incorporating a popover under a search bar in Vue can significantly enhance user experience by providing contextual suggestions. Leveraging Vue’s reactive data binding allows for real-time updates, ensuring that users receive relevant information as they type.”
James Patel (UI/UX Designer, DesignLab). “When designing a popover for a search bar, it is crucial to focus on accessibility and usability. Ensuring that the popover is keyboard navigable and screen-reader friendly can make the interface more inclusive, ultimately improving engagement.”
Sarah Thompson (Software Engineer, CodeCraft). “Utilizing libraries such as BootstrapVue or Vuetify can streamline the process of implementing popovers in Vue applications. These libraries offer pre-built components that can save development time while ensuring a polished and professional look.”
Frequently Asked Questions (FAQs)
What is a popover in Vue?
A popover in Vue is a UI component that displays additional information or options when a user interacts with an element, such as clicking or hovering. It typically appears as a small overlay that can contain text, buttons, or other interactive elements.
How can I create a popover under a search bar in Vue?
To create a popover under a search bar in Vue, you can use a library like BootstrapVue or Vuetify, which provide built-in popover components. Alternatively, you can create a custom popover component using Vue’s transition and positioning techniques, ensuring it is rendered below the search bar based on its position.
What libraries can I use for implementing popovers in Vue?
Popular libraries for implementing popovers in Vue include BootstrapVue, Vuetify, Element UI, and Tippy.js. Each library offers different styles and functionalities, allowing you to choose one that best fits your project’s design requirements.
How do I position the popover correctly under the search bar?
To position the popover correctly under the search bar, you can use CSS for absolute positioning based on the search bar’s coordinates. You may also utilize JavaScript to dynamically calculate the position when the popover is displayed, ensuring it aligns perfectly beneath the search bar.
Can I customize the content of the popover?
Yes, you can customize the content of the popover in Vue. You can pass any HTML or Vue components as content, allowing for rich and interactive displays, such as lists, forms, or images.
Is it possible to control the visibility of the popover programmatically?
Yes, you can control the visibility of the popover programmatically in Vue. By using data properties or Vuex state management, you can toggle the popover’s visibility based on user interactions or other application events.
Incorporating a popover under a search bar in a Vue application can significantly enhance user experience by providing contextual assistance and relevant suggestions. This functionality often involves utilizing Vue’s reactive data properties and components to dynamically display the popover based on user input. By leveraging libraries such as BootstrapVue or Vuetify, developers can streamline the implementation process, ensuring that the popover is both visually appealing and responsive.
Furthermore, the integration of a popover can facilitate improved interaction by allowing users to quickly access additional information or options without navigating away from the search bar. This not only keeps the interface clean but also encourages users to engage more deeply with the content. It is essential to ensure that the popover is appropriately styled and positioned to avoid obstructing the search input, thereby maintaining usability.
implementing a popover under a search bar in Vue applications is a practical approach to enhance interactivity and user satisfaction. By focusing on the design and functionality of the popover, developers can create a seamless experience that supports users in their search endeavors. Ultimately, this feature can contribute to a more intuitive and efficient user interface, aligning with modern web development best practices.
Author Profile
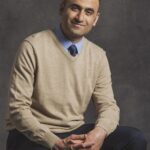
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?