How Can You Check if a File Exists Using PowerShell?
In the world of automation and system administration, efficiency is key. PowerShell, a powerful scripting language from Microsoft, empowers users to streamline tasks and manage systems with remarkable ease. One fundamental operation that often arises in scripting is the need to verify the existence of a file. Whether you’re developing a complex script or simply trying to ensure that a necessary file is present before executing a command, knowing how to check if a file exists in PowerShell is an essential skill that can save you time and prevent errors.
Understanding how to check for file existence in PowerShell not only enhances your scripting capabilities but also helps you create more robust and error-resistant scripts. With a few simple commands, you can easily ascertain whether a file is available in a specified location, allowing you to make informed decisions based on its presence or absence. This functionality is particularly useful in scenarios where scripts depend on external resources, such as configuration files, logs, or data inputs, ensuring that your automation processes run smoothly without unexpected interruptions.
As we delve deeper into this topic, we will explore various methods and best practices for checking file existence in PowerShell. From basic commands to more advanced techniques, you’ll gain the insights needed to implement effective file checks in your scripts, enhancing your overall productivity and system management prowess. Whether you’re a novice
Using PowerShell to Check File Existence
PowerShell provides a straightforward approach to check if a file exists on the system. The primary cmdlet used for this purpose is `Test-Path`. This cmdlet returns a boolean value, indicating whether the specified path exists.
To use `Test-Path`, you simply provide the file path as an argument. Here is a basic example:
“`powershell
$path = “C:\example\file.txt”
$fileExists = Test-Path $path
“`
In this example, the variable `$fileExists` will contain `True` if the file exists and “ if it does not. This can be especially useful in scripts where conditional logic is required based on file existence.
Conditional Logic Based on File Existence
You can incorporate `Test-Path` into conditional statements to execute code based on whether a file exists. For instance:
“`powershell
if (Test-Path $path) {
Write-Host “The file exists.”
} else {
Write-Host “The file does not exist.”
}
“`
This script checks if the file specified in the `$path` variable exists and provides appropriate feedback.
Checking for Directories
The `Test-Path` cmdlet can also be used to check for the existence of directories. The syntax remains the same, but it is important to provide the directory path. For example:
“`powershell
$dirPath = “C:\example\folder”
$dirExists = Test-Path $dirPath
“`
The following table summarizes the usage of `Test-Path` for files and directories:
Type | Example Code | Returns |
---|---|---|
File | Test-Path “C:\example\file.txt” | True or |
Directory | Test-Path “C:\example\folder” | True or |
Using Wildcards with Test-Path
PowerShell’s `Test-Path` also supports wildcards, allowing you to check for the existence of multiple files that match a pattern. For example, to check for any `.txt` files in a directory:
“`powershell
$wildcardPath = “C:\example\*.txt”
$anyTxtFilesExist = Test-Path $wildcardPath
“`
This will return `True` if any `.txt` files exist in the specified directory, enabling more flexible file checking.
Verbose Output for Debugging
For debugging purposes, you may wish to see more detailed output when checking for file existence. You can use the `-Verbose` flag with `Test-Path` as follows:
“`powershell
Test-Path -Path $path -Verbose
“`
This will provide additional information about the operation, which can be helpful when troubleshooting scripts that depend on file existence.
Overall, leveraging PowerShell’s `Test-Path` cmdlet streamlines the process of file existence checks and integrates seamlessly into larger automation scripts.
Checking for File Existence in PowerShell
To determine if a file exists in PowerShell, you can utilize the `Test-Path` cmdlet. This cmdlet checks the existence of a path and returns a boolean value indicating whether the specified file or directory exists.
Using `Test-Path` Cmdlet
The syntax for the `Test-Path` cmdlet is straightforward:
“`powershell
Test-Path -Path “C:\path\to\your\file.txt”
“`
Example
To check if a specific file exists, you can run the following command:
“`powershell
$FilePath = “C:\Users\YourUsername\Documents\example.txt”
if (Test-Path -Path $FilePath) {
Write-Host “The file exists.”
} else {
Write-Host “The file does not exist.”
}
“`
Key Considerations
- Case Sensitivity: The file path is not case-sensitive on Windows systems.
- Network Paths: You can also use `Test-Path` to check for files on network shares, such as:
“`powershell
Test-Path -Path “\\NetworkShare\Folder\File.txt”
“`
- Wildcard Support: `Test-Path` supports wildcards, allowing you to check for the existence of multiple files:
“`powershell
Test-Path -Path “C:\path\to\your\*.txt”
“`
Alternative Methods
While `Test-Path` is the most common method, there are alternative ways to check for file existence, such as using the `.Exists` property of the `FileInfo` class.
Example Using `System.IO.FileInfo`
“`powershell
$FileInfo = New-Object System.IO.FileInfo(“C:\path\to\your\file.txt”)
if ($FileInfo.Exists) {
Write-Host “The file exists.”
} else {
Write-Host “The file does not exist.”
}
“`
Advantages of Using `FileInfo`
- Detailed Information: This method allows access to additional file properties without needing a separate command.
- Object-Oriented: It provides an object-oriented approach, which can be beneficial for more complex scripts.
Handling Errors
When checking for file existence, it’s crucial to handle potential errors, especially when dealing with paths that may not be accessible.
Example of Error Handling
“`powershell
try {
$FilePath = “C:\path\to\your\file.txt”
if (Test-Path -Path $FilePath) {
Write-Host “The file exists.”
} else {
Write-Host “The file does not exist.”
}
} catch {
Write-Host “An error occurred: $_”
}
“`
Error Handling Best Practices
- Utilize `try` and `catch` blocks to manage exceptions.
- Log errors for troubleshooting, especially in production scripts.
Summary of Common Commands
Command | Description |
---|---|
`Test-Path -Path “C:\file.txt”` | Checks if the specified file exists. |
`$FileInfo.Exists` | Checks existence using `FileInfo` object. |
`Try/Catch` | Error handling to manage exceptions. |
By using these methods, you can effectively verify the existence of files in PowerShell, ensuring robust script execution in various scenarios.
Expert Insights on Using PowerShell to Check File Existence
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “PowerShell provides a straightforward and efficient method to check if a file exists using the Test-Path cmdlet. This command not only simplifies the process but also enhances script readability, which is crucial for maintaining code in collaborative environments.”
Michael Thompson (IT Solutions Architect, CyberSafe Technologies). “Incorporating file existence checks in PowerShell scripts is essential for error handling and ensuring that subsequent commands operate on valid files. Using conditional statements with Test-Path allows developers to create robust scripts that can gracefully handle missing files.”
Linda Nguyen (DevOps Consultant, CloudOps Experts). “When automating tasks with PowerShell, checking for file existence is a best practice. It prevents runtime errors and ensures that your automation workflows are both reliable and efficient. Leveraging Test-Path in scripts can save time and reduce troubleshooting efforts.”
Frequently Asked Questions (FAQs)
How can I check if a file exists using PowerShell?
You can check if a file exists in PowerShell using the `Test-Path` cmdlet. For example, `Test-Path “C:\path\to\your\file.txt”` returns `True` if the file exists and “ otherwise.
What is the syntax for using Test-Path in PowerShell?
The syntax for using `Test-Path` is straightforward: `Test-Path
Can I use Test-Path to check for directories as well?
Yes, `Test-Path` can be used to check for both files and directories. It returns `True` if the specified directory exists and “ if it does not.
Is there a way to check for the existence of multiple files at once in PowerShell?
Yes, you can check for multiple files by using a loop or by passing an array of file paths to `Test-Path`. For example: `@(“file1.txt”, “file2.txt”) | ForEach-Object { Test-Path $_ }`.
What happens if the specified path is invalid when using Test-Path?
If the specified path is invalid or does not exist, `Test-Path` will return “. It does not throw an error for invalid paths.
Can I use Test-Path in conditional statements?
Yes, `Test-Path` can be used in conditional statements. For example, you can use it within an `if` statement: `if (Test-Path “C:\path\to\your\file.txt”) { “File exists” }`.
In summary, checking if a file exists in PowerShell is a straightforward task that can be accomplished using various methods. The most common approach is to utilize the `Test-Path` cmdlet, which returns a Boolean value indicating the presence of a specified file or directory. This cmdlet is efficient and easy to use, making it a preferred choice for many PowerShell users. Additionally, PowerShell provides other methods, such as using the `Get-Item` cmdlet, which can also be employed to determine file existence, although it may throw an error if the file does not exist.
Key takeaways from the discussion include the importance of understanding the context in which file existence checks are performed. For instance, when scripting, using `Test-Path` is often more appropriate due to its simplicity and error handling capabilities. Furthermore, incorporating file existence checks into scripts can enhance their robustness by preventing errors that arise from attempting to access non-existent files.
Ultimately, mastering file existence checks in PowerShell not only streamlines scripting processes but also contributes to more reliable and maintainable code. As users become more familiar with these techniques, they can leverage PowerShell’s capabilities to automate tasks effectively while minimizing potential disruptions caused by missing files.
Author Profile
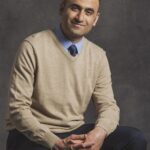
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?