How Can You Convert a String to an Integer in PowerShell?
In the world of PowerShell scripting, the ability to manipulate data types is crucial for effective programming. One common task that developers often encounter is the need to convert strings to integers. Whether you’re processing user input, reading from files, or handling data from APIs, understanding how to accurately transform a string representation of a number into an integer can streamline your scripts and enhance their functionality. This article will delve into the various methods available in PowerShell for converting strings to integers, providing you with the tools to tackle this essential task with confidence.
Converting a string to an integer in PowerShell is not just a simple matter of changing data types; it involves understanding the nuances of how PowerShell handles different formats and potential errors that may arise during conversion. From basic type casting to utilizing built-in cmdlets, there are several strategies that can be employed to achieve the desired outcome. Each method has its own advantages and considerations, making it important for users to choose the approach that best fits their specific needs.
As we explore the intricacies of string-to-integer conversion, you’ll discover practical examples and best practices that can help you avoid common pitfalls. By the end of this article, you’ll be equipped with the knowledge to confidently handle string conversions in your PowerShell scripts, ensuring that your code runs smoothly
Converting Strings to Integers in PowerShell
In PowerShell, converting a string to an integer can be achieved using several methods. The choice of method may depend on the specific context and requirements of your script. Below are some common approaches to perform this conversion effectively.
Using the [int] Type Accelerator
One of the simplest ways to convert a string to an integer in PowerShell is by using the type accelerator `[int]`. This method is straightforward and provides clear syntax.
“`powershell
$stringValue = “123”
$intValue = [int]$stringValue
“`
This conversion works seamlessly as long as the string contains a valid integer representation. If the string cannot be converted, an error will occur.
Using the `Convert` Class
The .NET `Convert` class provides methods to convert between different types, including strings to integers. The `ToInt32` method can be used as follows:
“`powershell
$stringValue = “456”
$intValue = [Convert]::ToInt32($stringValue)
“`
This method also handles the conversion process, throwing an exception if the string is not a valid integer.
Using TryParse for Safe Conversion
For scenarios where the input string may not always be a valid integer, using `int.TryParse` is recommended. This approach allows for error handling without exceptions.
“`powershell
$stringValue = “789”
[int]$intValue
$result = [int]::TryParse($stringValue, [ref]$intValue)
if ($result) {
Write-Host “Conversion successful: $intValue”
} else {
Write-Host “Conversion failed”
}
“`
Here, `TryParse` attempts to convert the string and returns a Boolean indicating success or failure, making it a robust option for user input.
Comparison of Methods
Method | Syntax Example | Exception on Failure | Recommended Usage |
---|---|---|---|
Type Accelerator `[int]` | `$intValue = [int]$stringValue` | Yes | Quick conversions, known data |
Convert Class | `$intValue = [Convert]::ToInt32($stringValue)` | Yes | General conversions |
TryParse | `$result = [int]::TryParse($stringValue, [ref]$intValue)` | No | User input, uncertain data |
Understanding the various methods available for converting strings to integers in PowerShell is essential for effective scripting. Each method has its use cases, and selecting the appropriate one can enhance the robustness and reliability of your scripts.
Converting a String to an Integer in PowerShell
In PowerShell, converting a string to an integer can be accomplished using several methods. The most common methods include casting, using the `[int]` type accelerator, or employing the `Convert` class.
Using Type Casting
Type casting is a straightforward method to convert a string to an integer. This method works well when the string represents a valid integer value.
“`powershell
$stringValue = “123”
$intValue = [int]$stringValue
“`
In this example, `$intValue` will hold the integer value `123`. If the string cannot be converted, PowerShell will throw an error.
Using the Convert Class
The `Convert` class provides a robust way to handle conversions, including from strings to integers. The `ToInt32` method can be utilized as follows:
“`powershell
$stringValue = “456”
$intValue = [Convert]::ToInt32($stringValue)
“`
This approach is useful for handling potential exceptions or when you need to specify different number styles.
Handling Non-Numeric Strings
When converting strings that may not represent valid integers, it is essential to handle exceptions to avoid runtime errors. The `Try-Catch` block can be employed effectively.
“`powershell
$stringValue = “abc”
try {
$intValue = [int]$stringValue
} catch {
Write-Host “Conversion failed: $_”
}
“`
This method allows the script to continue running while providing feedback when conversion fails.
Examples of Conversion with Different Scenarios
Below is a table illustrating different scenarios of string conversion and their outcomes:
Input String | Conversion Method | Outcome |
---|---|---|
“100” | `[int]` | 100 |
“200” | `[Convert]::ToInt32()` | 200 |
“3.14” | `[int]` | 3 (truncated) |
“xyz” | `[int]` | Error (non-numeric) |
” 42″ | `[int]` | 42 (whitespace ignored) |
Best Practices for String to Integer Conversion
When converting strings to integers in PowerShell, consider the following best practices:
- Validate Input: Ensure that the string is numeric before conversion.
- Use Try-Catch: Implement error handling to manage invalid conversions gracefully.
- Trim Whitespace: Use the `.Trim()` method on strings to remove leading or trailing whitespace.
- Check for Null Values: Always check if the string is null or empty before attempting conversion.
By adhering to these practices, you can minimize errors and ensure smooth conversions in your PowerShell scripts.
Expert Insights on Converting Strings to Integers in PowerShell
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When converting strings to integers in PowerShell, it is crucial to ensure that the string is a valid numeric representation. The use of the `[int]` type accelerator is a straightforward approach, but one must also handle potential exceptions that arise from invalid input.”
Michael Chen (PowerShell Expert, Scripting Solutions Group). “PowerShell’s ability to convert strings to integers seamlessly is one of its strengths. However, developers should be aware of the implications of data types and the need for validation, particularly when dealing with user input or external data sources.”
Lisa Tran (IT Consultant, CloudTech Advisors). “In practice, using `TryParse` methods can enhance the reliability of string-to-integer conversions in PowerShell scripts. This method prevents runtime errors and allows for graceful handling of invalid data, which is essential in production environments.”
Frequently Asked Questions (FAQs)
How can I convert a string to an integer in PowerShell?
You can convert a string to an integer in PowerShell using the `[int]` type accelerator. For example, use `$intValue = [int]$stringValue` where `$stringValue` is the string you want to convert.
What happens if the string cannot be converted to an integer?
If the string cannot be converted to an integer, PowerShell will throw an error indicating that the conversion failed. It is advisable to validate the string before conversion.
Is there a way to handle exceptions during conversion?
Yes, you can use a try-catch block to handle exceptions. For example:
“`powershell
try {
$intValue = [int]$stringValue
}
catch {
Write-Host “Conversion failed: $_”
}
“`
Can I convert a string with non-numeric characters?
No, attempting to convert a string containing non-numeric characters will result in an error. You should ensure the string is purely numeric before conversion.
Are there alternative methods to convert strings to integers in PowerShell?
Yes, you can also use the `Convert` class, specifically `Convert.ToInt32($stringValue)`, which provides additional options for handling different formats.
How do I convert a string to a different integer type, like long or byte?
You can specify the desired type by using the respective type accelerator. For example, use `[long]$stringValue` for long integers or `[byte]$stringValue` for byte values, ensuring the string is within the valid range for the target type.
In PowerShell, converting a string to an integer is a straightforward process that can be accomplished using various methods. The most common approach involves using the `[int]` type accelerator, which allows for a seamless transformation of string representations of numbers into their integer counterparts. For example, executing `[int]”123″` will yield the integer value `123`. This method is efficient and widely used for basic conversions.
Another method to consider is the `Convert` class, specifically `Convert.ToInt32()`, which provides additional functionality, such as handling null values and exceptions more gracefully. This can be particularly useful in scripts where input data may not always be formatted correctly or may contain unexpected characters. Utilizing this method can enhance the robustness of your PowerShell scripts, ensuring that they handle errors effectively.
It is also important to note that when converting strings to integers, PowerShell will throw an error if the string cannot be converted due to invalid characters. Therefore, implementing error handling mechanisms, such as try-catch blocks, can help manage these situations and prevent script failures. Overall, understanding the different methods for string-to-integer conversion in PowerShell enables users to write more effective and error-resistant scripts.
Author Profile
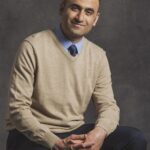
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?