How Can I Use PowerShell to Create a Folder If It Doesn’t Exist?
In the world of system administration and automation, PowerShell stands out as a powerful tool that streamlines tasks and enhances productivity. One common yet essential operation is managing file systems, particularly the creation of folders. Whether you’re organizing project files, setting up directories for applications, or simply tidying up your workspace, the ability to create a folder only if it doesn’t already exist can save time and prevent errors. In this article, we will explore how to leverage PowerShell to efficiently manage folder structures, ensuring that your file system remains organized without redundant operations.
Creating a folder in PowerShell is a straightforward task, but doing so conditionally—only when it doesn’t already exist—adds a layer of sophistication to your scripting. This approach not only prevents unnecessary errors but also enhances the robustness of your scripts, making them more reliable in various environments. By mastering this technique, you’ll be able to automate file management tasks seamlessly, allowing you to focus on more critical aspects of your projects.
Throughout this article, we will delve into the syntax and commands necessary for implementing this functionality in PowerShell. We’ll also discuss best practices and scenarios where this method can be particularly beneficial, ensuring that you have the tools at your disposal to manage your file system effectively. Whether you’re a seasoned PowerShell user or just starting,
Creating a Folder in PowerShell
In PowerShell, creating a folder if it does not already exist can be efficiently accomplished using the `New-Item` cmdlet along with a conditional check. This approach ensures that no errors occur if the folder already exists, making the script robust and user-friendly.
To create a folder conditionally, you can utilize the following syntax:
“`powershell
$folderPath = “C:\Path\To\Your\Folder”
if (-not (Test-Path -Path $folderPath)) {
New-Item -Path $folderPath -ItemType Directory
}
“`
In this script:
- `$folderPath` is a variable that stores the path of the folder you want to create.
- `Test-Path` checks if the specified path exists.
- If the path does not exist (`-not`), `New-Item` creates the new directory.
Using the `-Force` Parameter
For more advanced scenarios, the `-Force` parameter can be used with `New-Item`. This parameter allows you to overwrite existing folders or create nested directories in one command.
Here’s how you can use it:
“`powershell
$folderPath = “C:\Path\To\Your\Folder”
New-Item -Path $folderPath -ItemType Directory -Force
“`
This command will create the specified folder and any necessary parent folders, while suppressing errors if the folder already exists.
Table of Common PowerShell Cmdlets for Folder Management
Cmdlet | Description |
---|---|
New-Item |
Creates a new item, such as a file or folder. |
Test-Path |
Checks if a path exists. |
Remove-Item |
Deletes files or folders. |
Get-ChildItem |
Lists files and folders in a specified location. |
Example of Creating Nested Folders
When you need to create multiple nested folders, PowerShell can handle this as well. The following example demonstrates how to create nested directories in a single command:
“`powershell
$nestedFolderPath = “C:\Path\To\Your\Nested\Folder”
New-Item -Path $nestedFolderPath -ItemType Directory -Force
“`
If “C:\Path\To\Your\Nested” does not exist, it will be created automatically.
By utilizing these methods in PowerShell, you can effectively manage folder creation, ensuring that your scripts run smoothly without unnecessary interruptions due to existing directories.
PowerShell Command to Create a Folder if It Does Not Exist
To create a folder using PowerShell only if it does not already exist, the `Test-Path` cmdlet can be utilized in conjunction with the `New-Item` cmdlet. This ensures that you do not attempt to recreate a folder that is already present, thereby avoiding errors and unnecessary operations.
Basic Syntax
The fundamental syntax for this operation is as follows:
“`powershell
if (-not (Test-Path “C:\Path\To\Your\Folder”)) {
New-Item -ItemType Directory -Path “C:\Path\To\Your\Folder”
}
“`
In this command:
- `Test-Path` checks the existence of the specified path.
- `-not` negates the result, meaning the command inside the block will only run if the folder does not exist.
- `New-Item -ItemType Directory` creates a new directory at the specified path.
Example Usage
To illustrate this process, consider the following example:
“`powershell
$folderPath = “C:\Example\NewFolder”
if (-not (Test-Path $folderPath)) {
New-Item -ItemType Directory -Path $folderPath
Write-Host “Folder created: $folderPath”
} else {
Write-Host “Folder already exists: $folderPath”
}
“`
In this example:
- A variable `$folderPath` is defined for clarity and reusability.
- The `Write-Host` command provides feedback on whether the folder was created or if it already existed.
Using a Function for Reusability
To improve efficiency, encapsulating the logic within a function can be beneficial, especially if you need to create multiple folders throughout your scripts.
“`powershell
function Create-FolderIfNotExists {
param (
[string]$Path
)
if (-not (Test-Path $Path)) {
New-Item -ItemType Directory -Path $Path
Write-Host “Folder created: $Path”
} else {
Write-Host “Folder already exists: $Path”
}
}
Example of using the function
Create-FolderIfNotExists “C:\Example\AnotherFolder”
“`
This function:
- Accepts a parameter for the folder path.
- Contains the same conditional logic to check for existence and create the folder if needed.
Considerations
When implementing folder creation scripts, keep the following in mind:
- Permissions: Ensure that the script runs with adequate permissions to create directories in the target location.
- Path Validity: Verify that the path provided is valid and does not contain invalid characters or formats.
- Error Handling: Incorporate error handling to manage unexpected issues, using `try-catch` blocks as necessary.
“`powershell
try {
Create-FolderIfNotExists “C:\Example\NewFolder”
} catch {
Write-Host “An error occurred: $_”
}
“`
This approach enhances the robustness of your scripts by gracefully managing exceptions.
Expert Insights on Creating Folders with PowerShell
Dr. Emily Johnson (Senior Systems Administrator, Tech Solutions Inc.). “Using PowerShell to create a folder only if it does not exist is a best practice that enhances script efficiency. The command ‘New-Item -Path “C:\YourPath” -ItemType Directory -Force’ ensures that the script does not fail if the folder is already present.”
Mark Thompson (PowerShell Automation Specialist, IT Pro Magazine). “Incorporating conditional checks in PowerShell scripts is crucial for robust automation. I recommend using ‘if (!(Test-Path “C:\YourPath”)) { New-Item -Path “C:\YourPath” -ItemType Directory }’ to prevent unnecessary errors and maintain clean script execution.”
Linda Martinez (Cloud Infrastructure Engineer, CloudTech Innovations). “Efficient folder management in PowerShell not only saves time but also reduces clutter. By implementing ‘New-Item’ with a preceding ‘Test-Path’ check, you ensure that your scripts are both effective and user-friendly, which is essential in cloud environments.”
Frequently Asked Questions (FAQs)
How can I create a folder in PowerShell if it does not exist?
You can use the `New-Item` cmdlet with the `-ItemType Directory` parameter. Combine it with the `-Force` parameter to avoid errors if the folder already exists. For example: `New-Item -Path “C:\Your\Path\FolderName” -ItemType Directory -Force`.
What command checks if a folder exists in PowerShell?
You can use the `Test-Path` cmdlet to check if a folder exists. For example: `Test-Path “C:\Your\Path\FolderName”` returns `True` if the folder exists and “ if it does not.
Can I create multiple folders at once using PowerShell?
Yes, you can create multiple folders by specifying an array of folder paths with a loop. For example:
“`powershell
$folders = “C:\Path\Folder1”, “C:\Path\Folder2”
foreach ($folder in $folders) {
New-Item -Path $folder -ItemType Directory -Force
}
“`
Is there a one-liner command to create a folder if it does not exist?
Yes, you can use the following one-liner:
“`powershell
if (-not (Test-Path “C:\Your\Path\FolderName”)) { New-Item -Path “C:\Your\Path\FolderName” -ItemType Directory }
“`
What happens if I try to create a folder that already exists in PowerShell?
If you use `New-Item` without the `-Force` parameter, PowerShell will throw an error indicating that the folder already exists. Using `-Force` prevents this error.
Can I create a folder with a timestamp in its name using PowerShell?
Yes, you can create a folder with a timestamp by appending the current date and time to the folder name. For example:
“`powershell
$timestamp = Get-Date -Format “yyyyMMdd_HHmmss”
New-Item -Path “C:\Your\Path\Folder_$timestamp” -ItemType Directory -Force
“`
In summary, creating a folder in PowerShell only if it does not already exist is a straightforward process that can be accomplished using the `Test-Path` cmdlet in conjunction with the `New-Item` cmdlet. This approach allows users to check for the existence of a directory before attempting to create it, thereby preventing unnecessary errors and ensuring efficient script execution. The use of conditional statements to handle folder creation reflects best practices in scripting and automation.
Key takeaways from this discussion include the importance of error handling and validation in scripting environments. By incorporating checks for existing directories, users can enhance the robustness of their scripts. Additionally, leveraging PowerShell’s built-in cmdlets not only simplifies the process but also aligns with the principles of clean and maintainable code.
Overall, mastering the ability to create folders conditionally in PowerShell is a valuable skill for system administrators and developers alike. It facilitates better resource management and contributes to the overall efficiency of automation tasks. Familiarity with these concepts can lead to more effective PowerShell scripting practices.
Author Profile
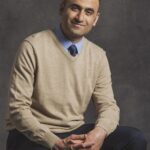
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?