How Can You Use PowerShell to Replace a String in a File?
In the world of system administration and automation, PowerShell stands out as a powerful tool that can streamline a multitude of tasks. One common scenario that many IT professionals encounter is the need to modify text within files, whether it be updating configuration settings, correcting typos, or replacing outdated information. If you’ve ever found yourself wrestling with manual edits or cumbersome text editors, you’re in for a treat. This article will explore the art of replacing strings in files using PowerShell, empowering you to enhance your efficiency and accuracy.
PowerShell offers a range of cmdlets and techniques that simplify the process of string manipulation within files. With just a few lines of code, you can search for specific text patterns and replace them seamlessly, saving you valuable time and reducing the risk of human error. Whether you’re working with simple text files or more complex scripts, understanding how to leverage PowerShell for string replacement can significantly enhance your workflow.
As we delve deeper into this topic, we’ll uncover the various methods available for string replacement, highlighting best practices and potential pitfalls along the way. From using built-in cmdlets to crafting custom scripts, you’ll gain insights that can be applied in real-world scenarios, making your PowerShell experience even more rewarding. Get ready to transform the way you handle text files and elevate
Using PowerShell to Replace a String in a File
PowerShell provides a powerful set of cmdlets that enable users to manipulate files and strings efficiently. One of the most common tasks is replacing a specific string within a file. This can be accomplished using the `Get-Content` and `Set-Content` cmdlets, along with the `-replace` operator.
To replace a string in a file, follow these steps:
- Read the Content: Use `Get-Content` to read the contents of the file.
- Replace the String: Apply the `-replace` operator to substitute the desired string.
- Write the Changes: Use `Set-Content` to save the modified content back to the file.
Here’s a simple example:
“`powershell
(Get-Content “C:\path\to\your\file.txt”) -replace “oldString”, “newString” | Set-Content “C:\path\to\your\file.txt”
“`
In this command:
- `”C:\path\to\your\file.txt”` is the path to the file.
- `”oldString”` is the string you want to replace.
- `”newString”` is the new string that will replace the old one.
Considerations When Replacing Strings
When performing string replacements, there are several important considerations to keep in mind:
- Case Sensitivity: The `-replace` operator is case-insensitive by default. If you need case sensitivity, you can use the `-creplace` operator.
- Regular Expressions: The `-replace` operator uses regular expressions. This allows for complex patterns to be matched and replaced, but also means that special characters need to be escaped.
- Backup Files: It is a good practice to create a backup of the original file before performing replacements, especially if the changes are significant.
Example of Replacing Multiple Strings
To replace multiple strings in a file, you can chain the `-replace` operations or use a loop. Here’s an example of replacing multiple strings using a hashtable:
“`powershell
$replacements = @{
“firstString” = “replacement1”
“secondString” = “replacement2”
}
$content = Get-Content “C:\path\to\your\file.txt”
foreach ($key in $replacements.Keys) {
$content = $content -replace $key, $replacements[$key]
}
Set-Content “C:\path\to\your\file.txt” $content
“`
In this example, the `$replacements` hashtable maps old strings to their new values, allowing for easy modifications.
Performance Considerations
For larger files, performance can become an issue. Here are some strategies to improve efficiency:
- Use `-Raw`: When reading large files, use `Get-Content -Raw` to read the entire file as a single string, which can be more efficient.
- Stream Output: If you need to process very large files, consider using `StreamReader` and `StreamWriter` for line-by-line processing without loading the entire file into memory.
Method | Description | Performance |
---|---|---|
Get-Content | Reads file line by line | Good for small to medium files |
Get-Content -Raw | Reads the whole file as a single string | Better for larger files |
StreamReader | Reads file line by line with lower memory usage | Best for very large files |
Using PowerShell to Replace Strings in a File
To perform string replacement in files using PowerShell, the `Get-Content` cmdlet is often combined with `Set-Content` or `Out-File`. This allows you to read the contents of a file, modify the text, and then write the changes back to the file.
Basic Syntax for String Replacement
The fundamental syntax for replacing a string in a file is as follows:
“`powershell
(Get-Content ‘path\to\your\file.txt’) -replace ‘oldString’, ‘newString’ | Set-Content ‘path\to\your\file.txt’
“`
Explanation of Components:
- `Get-Content`: Reads the content of the specified file.
- `-replace`: A PowerShell operator that allows you to specify the old string and the new string.
- `Set-Content`: Writes the modified content back to the original file.
Example: Simple String Replacement
Suppose you have a text file named `example.txt` containing the phrase “Hello World”. To replace “World” with “PowerShell”, you would execute the following command:
“`powershell
(Get-Content ‘example.txt’) -replace ‘World’, ‘PowerShell’ | Set-Content ‘example.txt’
“`
Result:
The content of `example.txt` will now read “Hello PowerShell”.
Using Regular Expressions for Advanced Replacements
PowerShell’s `-replace` operator supports regular expressions, enabling more complex string manipulations. For instance, if you want to replace all occurrences of “cat” or “dog” with “pet”, you can use:
“`powershell
(Get-Content ‘example.txt’) -replace ‘cat|dog’, ‘pet’ | Set-Content ‘example.txt’
“`
Regular Expression Breakdown:
- `cat|dog`: Matches either “cat” or “dog”.
- `pet`: The string that will replace the matched values.
Saving to a Different File
If you prefer to keep the original file intact while saving the changes to a new file, you can redirect the output to a different file:
“`powershell
(Get-Content ‘example.txt’) -replace ‘World’, ‘PowerShell’ | Set-Content ‘newfile.txt’
“`
Result:
The changes will be saved in `newfile.txt`, leaving `example.txt` unchanged.
Handling Large Files Efficiently
For large files, using `Get-Content` can be memory-intensive. To handle this more efficiently, consider using a stream approach:
“`powershell
$reader = [System.IO.StreamReader]::new(‘path\to\your\file.txt’)
$writer = [System.IO.StreamWriter]::new(‘path\to\your\newfile.txt’)
while ($null -ne ($line = $reader.ReadLine())) {
$line -replace ‘oldString’, ‘newString’ | $writer.WriteLine()
}
$reader.Close()
$writer.Close()
“`
Advantages:
- Reduces memory consumption.
- Handles larger files without loading everything into memory at once.
Using PowerShell ISE for Testing
When experimenting with string replacements, consider using PowerShell ISE or another script editor. This allows you to test commands without affecting the original files until you’re confident in your script.
Tips for Testing:
- Always back up original files.
- Use `Write-Output` to preview changes before applying them.
Common Pitfalls to Avoid
- Overwriting Files: Be cautious when using `Set-Content` on the same file you are reading from.
- Incorrect String Patterns: Ensure that the strings you are replacing are correctly specified, especially when using regular expressions.
- Permissions: Ensure you have the necessary permissions to read from and write to the specified files.
Expert Insights on Using PowerShell for String Replacement in Files
Jessica Harmon (Senior Systems Administrator, Tech Solutions Inc.). “Utilizing PowerShell for string replacement in files is an efficient way to automate text modifications across multiple documents. The `-replace` operator in PowerShell is particularly powerful, allowing for both simple and complex replacements using regular expressions.”
Michael Chen (DevOps Engineer, Cloud Innovations). “When replacing strings in files with PowerShell, it’s crucial to consider the encoding of the files. Using `Get-Content` with the `-Encoding` parameter ensures that the content is read correctly, which can prevent issues during the replacement process.”
Linda Patel (PowerShell Automation Specialist, IT Efficiency Group). “For large files, leveraging the `-replace` method in a pipeline can significantly enhance performance. Additionally, implementing error handling with try-catch blocks is essential to manage any exceptions that may arise during the string replacement operation.”
Frequently Asked Questions (FAQs)
How can I replace a string in a file using PowerShell?
You can use the `Get-Content` cmdlet to read the file, pipe it to `ForEach-Object` to replace the string, and then use `Set-Content` to write the changes back. For example:
“`powershell
(Get-Content “path\to\file.txt”) -replace “oldString”, “newString” | Set-Content “path\to\file.txt”
“`
Is it possible to replace multiple strings in a file at once with PowerShell?
Yes, you can chain multiple `-replace` operations or use a loop. For example:
“`powershell
$content = Get-Content “path\to\file.txt”
$content -replace “oldString1”, “newString1” -replace “oldString2”, “newString2” | Set-Content “path\to\file.txt”
“`
What if I want to replace a string in a large file without loading the entire file into memory?
You can use `StreamReader` and `StreamWriter` for more efficient memory usage. Here’s an example:
“`powershell
$reader = [System.IO.StreamReader]::new(“path\to\file.txt”)
$writer = [System.IO.StreamWriter]::new(“path\to\tempfile.txt”)
while (($line = $reader.ReadLine()) -ne $null) {
$line -replace “oldString”, “newString” | $writer.WriteLine()
}
$reader.Close()
$writer.Close()
Remove-Item “path\to\file.txt”
Rename-Item “path\to\tempfile.txt” “path\to\file.txt”
“`
Can I perform a case-insensitive string replacement in PowerShell?
Yes, you can perform a case-insensitive replacement by using the `-creplace` operator. For example:
“`powershell
(Get-Content “path\to\file.txt”) -creplace “oldString”, “newString” | Set-Content “path\to\file.txt”
“`
How do I backup a file before replacing strings in it using PowerShell?
You can create a copy of the file before making changes by using the `Copy-Item` cmdlet. For example:
“`
In summary, using PowerShell to replace strings in files is a powerful and efficient method for managing text data. PowerShell provides various cmdlets, such as `Get-Content`, `Set-Content`, and `-replace` operator, that allow users to read, modify, and write content back to files seamlessly. This functionality is particularly useful for system administrators and developers who need to automate tasks or update configuration files in bulk.
One of the key insights is the flexibility offered by PowerShell in handling different file types and formats. Users can perform replacements in plain text files, scripts, or even structured data formats like JSON and XML. Additionally, the ability to use regular expressions with the `-replace` operator enhances the power of string manipulation, enabling more complex search and replace operations.
Another important takeaway is the emphasis on safety and testing when performing string replacements. It is advisable to create backups of files before making changes, especially when dealing with critical system files. Using the `-WhatIf` parameter can help simulate changes without applying them, allowing users to verify the impact of their commands beforehand.
mastering string replacement in files using PowerShell not only streamlines workflows but also empowers users to maintain and
Author Profile
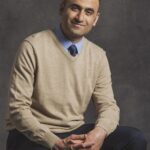
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?