How Can I Use PowerShell to Save a List to a File?
In the world of system administration and automation, PowerShell stands out as a powerful tool that empowers users to manage and manipulate data with ease. Whether you’re a seasoned IT professional or a curious beginner, the ability to save a list to a file using PowerShell can streamline your workflows and enhance your productivity. Imagine effortlessly capturing the results of your scripts or commands into a neatly organized file, ready for analysis or reporting. This capability not only simplifies data management but also opens up a realm of possibilities for further automation and data processing.
When working with PowerShell, the process of saving a list to a file is both straightforward and versatile. From exporting data generated by complex queries to logging essential information for future reference, PowerShell provides a variety of methods to achieve this. Understanding the foundational commands and techniques can significantly enhance your scripting skills and enable you to handle data more efficiently.
As you delve deeper into the intricacies of PowerShell, you’ll discover various ways to format and export your data, ensuring that it meets your specific needs. Whether you prefer working with CSV files, text files, or other formats, PowerShell offers the flexibility to cater to your requirements. Join us as we explore the essential commands and best practices for saving lists to files, unlocking the full potential of PowerShell in your
Saving a List to a File in PowerShell
To save a list to a file in PowerShell, you can utilize various cmdlets that streamline the process. The most common cmdlets for this purpose are `Out-File`, `Set-Content`, and `Add-Content`. Each cmdlet serves a specific function depending on your requirements for data handling.
Using Out-File Cmdlet
The `Out-File` cmdlet is often the go-to method for exporting data to a file. It allows you to create a new file or overwrite an existing one. The syntax is straightforward:
“`powershell
Your-Command | Out-File -FilePath “C:\Path\To\Your\File.txt”
“`
- Example: To save a list of running processes to a text file, you can run:
“`powershell
Get-Process | Out-File -FilePath “C:\Processes.txt”
“`
Using Set-Content Cmdlet
The `Set-Content` cmdlet is another powerful tool for saving text data to a file. It writes content to a file, replacing the existing content if the file already exists. Here’s how you can use it:
“`powershell
Set-Content -Path “C:\Path\To\Your\File.txt” -Value “Your List Here”
“`
- Example: To save a simple list of items:
“`powershell
$items = “Item1”, “Item2”, “Item3”
Set-Content -Path “C:\Items.txt” -Value $items
“`
Using Add-Content Cmdlet
If you need to append data to an existing file rather than overwrite it, use the `Add-Content` cmdlet. This is particularly useful for logging or gradually building a file. The syntax is:
“`powershell
Add-Content -Path “C:\Path\To\Your\File.txt” -Value “Your New Item”
“`
- Example: To add more items to the previously created list:
“`powershell
Add-Content -Path “C:\Items.txt” -Value “Item4”
“`
Considerations for File Encoding
When saving a list, it’s essential to consider the encoding format. PowerShell allows you to specify the encoding type to ensure compatibility, especially when working with text that includes special characters. The `-Encoding` parameter can be used with the above cmdlets. Common encoding types include:
Encoding Type | Description |
---|---|
ASCII | Standard ASCII encoding |
UTF8 | UTF-8 encoding (with BOM) |
UTF7 | UTF-7 encoding |
Unicode | UTF-16 encoding |
Example of specifying encoding with `Set-Content`:
“`powershell
Set-Content -Path “C:\Items.txt” -Value $items -Encoding UTF8
“`
By employing these cmdlets, you can effectively save lists to files in PowerShell, catering to various needs such as overwriting, appending, and specifying encoding formats. Each cmdlet provides a unique method to handle file operations seamlessly within scripts and command-line tasks.
Using PowerShell to Save a List to a File
PowerShell provides a variety of methods to save data to files, making it a powerful tool for automation and data management. Below are some common approaches to achieve this.
Saving Arrays to a Text File
You can easily save arrays to text files using the `Out-File` cmdlet or the `Set-Content` cmdlet. Here’s how to do it:
Example: Using Out-File
“`powershell
$array = “Item1”, “Item2”, “Item3”
$array | Out-File -FilePath “C:\path\to\your\file.txt”
“`
Example: Using Set-Content
“`powershell
$array = “Item1”, “Item2”, “Item3”
Set-Content -Path “C:\path\to\your\file.txt” -Value $array
“`
These commands will create a text file with each item on a new line.
Saving Objects to a CSV File
When dealing with structured data, such as objects, saving them to a CSV file is often more appropriate. This can be accomplished using the `Export-Csv` cmdlet.
Example: Exporting Objects to CSV
“`powershell
$objects = @(
[PSCustomObject]@{ Name = “John”; Age = 30 },
[PSCustomObject]@{ Name = “Jane”; Age = 25 }
)
$objects | Export-Csv -Path “C:\path\to\your\file.csv” -NoTypeInformation
“`
The `-NoTypeInformation` parameter suppresses the type information header, making the CSV cleaner.
Saving Data in JSON Format
For scenarios requiring data interchange or configuration storage, JSON format can be beneficial. Use the `ConvertTo-Json` cmdlet followed by `Set-Content`.
Example: Saving Data as JSON
“`powershell
$data = @(
[PSCustomObject]@{ Name = “John”; Age = 30 },
[PSCustomObject]@{ Name = “Jane”; Age = 25 }
)
$data | ConvertTo-Json | Set-Content -Path “C:\path\to\your\file.json”
“`
This command will create a JSON file that can easily be read by other programs or systems.
Appending to Existing Files
If you need to append data to an existing file instead of overwriting it, you can use the `Add-Content` cmdlet.
Example: Appending Data
“`powershell
$array = “Item4”, “Item5”
$array | Add-Content -Path “C:\path\to\your\file.txt”
“`
This will add “Item4” and “Item5” to the end of the specified text file.
Writing Data with Formatting
For more complex data structures where formatting is important, consider using `Format-Table` or `Format-List` before saving.
Example: Saving Formatted Output
“`powershell
$processes = Get-Process
$processes | Format-Table -Property Name, Id | Out-File -FilePath “C:\path\to\your\processes.txt”
“`
This command saves a formatted table of processes to a text file, enhancing readability.
File Path Considerations
When specifying file paths, it is essential to ensure:
- The directory exists; otherwise, PowerShell will return an error.
- You have the necessary permissions to write to the specified location.
Best Practices for File Management:
- Use full paths to avoid confusion.
- Use `Join-Path` for constructing file paths dynamically.
- Verify file existence or handle exceptions gracefully when saving files.
Expert Insights on Saving Lists to Files in PowerShell
Jessica Tran (Senior PowerShell Developer, Tech Solutions Inc.). “When saving a list to a file in PowerShell, utilizing the `Out-File` cmdlet is essential. It allows for straightforward redirection of output, ensuring that your data is stored in a specified format, such as TXT or CSV, which can be easily managed later.”
Michael Chen (IT Systems Administrator, Cloud Innovations). “For efficiency, I often recommend using the `Export-Csv` cmdlet when dealing with structured data. This method not only saves the list to a file but also preserves the integrity of the data, making it easier to import back into PowerShell or other applications.”
Linda Patel (PowerShell Automation Specialist, Automation Gurus). “It is crucial to consider the encoding format when saving a list to a file. Using the `-Encoding` parameter with `Out-File` can prevent issues with special characters, ensuring that the saved file is universally readable across different systems.”
Frequently Asked Questions (FAQs)
How can I save a list to a file in PowerShell?
You can save a list to a file in PowerShell by using the `Out-File` cmdlet or the `Set-Content` cmdlet. For example, to save a list of items to a text file, use the command: `Get-Content ‘source.txt’ | Out-File ‘destination.txt’`.
What file formats can I use when saving a list in PowerShell?
PowerShell allows you to save lists in various formats, including plain text (.txt), CSV (.csv), and JSON (.json), depending on the cmdlet you use. For structured data, CSV and JSON are preferred.
Can I append data to an existing file using PowerShell?
Yes, you can append data to an existing file by using the `-Append` parameter with the `Out-File` cmdlet. For example: `Get-Content ‘source.txt’ | Out-File ‘destination.txt’ -Append`.
Is it possible to save a list in a specific encoding format?
Yes, you can specify the encoding format when saving a file in PowerShell. Use the `-Encoding` parameter with cmdlets like `Out-File` or `Set-Content`. For example: `Out-File ‘file.txt’ -Encoding UTF8`.
How do I save an array to a file in PowerShell?
To save an array to a file, you can use the `Set-Content` cmdlet. For example: `$array = @(‘Item1’, ‘Item2’, ‘Item3’); $array | Set-Content ‘output.txt’` will save the array items to a text file.
Can I use PowerShell to export data to Excel?
Yes, you can export data to Excel using the `Export-Excel` cmdlet from the ImportExcel module. This allows you to create Excel files directly from PowerShell, enabling more complex data manipulations.
In summary, saving a list to a file using PowerShell is a straightforward process that can be accomplished through various methods. PowerShell provides several cmdlets, such as `Out-File`, `Set-Content`, and `Export-Csv`, which allow users to easily write data to text files or CSV formats. Each method serves different purposes, depending on the structure of the data and the desired output format.
Key takeaways include the importance of selecting the appropriate cmdlet based on the data type and the desired file format. For instance, `Out-File` is ideal for plain text, while `Export-Csv` is specifically designed for structured data in tabular form. Additionally, users should be mindful of file encoding and overwrite options when saving files to ensure data integrity and avoid unintentional data loss.
Overall, mastering these techniques not only enhances productivity but also empowers users to manage and manipulate data efficiently within PowerShell. By understanding the nuances of each cmdlet, users can tailor their scripts to meet specific needs, ultimately leading to more effective automation and data handling processes.
Author Profile
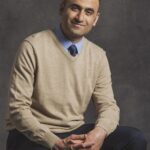
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?