How Can I Use a PowerShell Script to Run Another PowerShell Script?
In the realm of system administration and automation, PowerShell stands out as a powerful tool that empowers users to execute complex tasks with ease. Whether you’re managing servers, automating repetitive tasks, or orchestrating workflows, the ability to run one PowerShell script from another can significantly enhance your productivity and streamline your processes. This technique not only promotes modularity in your scripts but also allows for better organization and maintenance of your code.
At its core, invoking one PowerShell script from another is about leveraging the capabilities of PowerShell to create a more efficient scripting environment. This approach enables you to break down larger tasks into smaller, manageable scripts, each responsible for a specific function. By doing so, you can easily debug, test, and reuse code, ultimately leading to a more robust automation framework. Moreover, this method allows for the passing of parameters and the sharing of variables between scripts, which can be crucial for complex operations.
As we delve deeper into this topic, we will explore various techniques and best practices for executing PowerShell scripts within one another. From utilizing the `&` call operator to understanding the nuances of script execution policies, you’ll gain insights that will empower you to harness the full potential of PowerShell scripting. Whether you’re a seasoned administrator or just starting your journey, mastering this skill
Executing Another PowerShell Script
To run another PowerShell script from within a script, you can utilize the `&` (call operator), which allows you to execute a command, script, or function. This approach is straightforward and effective for chaining scripts together.
Here’s how you can do it:
“`powershell
& “C:\Path\To\Your\Script.ps1”
“`
This command will execute the specified PowerShell script located at `C:\Path\To\Your\Script.ps1`.
Passing Parameters
If the script you are calling requires parameters, you can pass them directly in the call. For example, if your script accepts two parameters, you can execute it as follows:
“`powershell
& “C:\Path\To\Your\Script.ps1” -Parameter1 “Value1” -Parameter2 “Value2”
“`
It’s essential to ensure that the parameters you are passing match the definitions within the called script.
Using the Invoke-Expression Cmdlet
Another method to execute a script is to use the `Invoke-Expression` cmdlet. This is particularly useful if you have the script path stored in a variable.
Example:
“`powershell
$scriptPath = “C:\Path\To\Your\Script.ps1”
Invoke-Expression $scriptPath
“`
This method evaluates the string and runs it as a command, effectively executing the script.
Script Execution Policy Considerations
Before executing scripts, it’s important to be aware of the PowerShell script execution policy, which might restrict script running. The policies include:
- Restricted: No scripts can be run.
- AllSigned: Only scripts signed by a trusted publisher can be run.
- RemoteSigned: Scripts downloaded from the internet must be signed.
- Unrestricted: All scripts can be run.
You can check the current execution policy with:
“`powershell
Get-ExecutionPolicy
“`
To change the execution policy, you can use:
“`powershell
Set-ExecutionPolicy RemoteSigned
“`
Make sure to run PowerShell as an administrator when changing the execution policy.
Example Table of Script Execution Methods
Method | Syntax | Usage |
---|---|---|
Call Operator | & “C:\Path\To\Your\Script.ps1” | Directly executes a script |
Invoke-Expression | Invoke-Expression $scriptPath | Executes a script path stored in a variable |
With Parameters | & “C:\Path\To\Your\Script.ps1” -Param “Value” | Executes a script with parameters |
Utilizing these methods effectively can streamline your PowerShell scripting tasks, allowing for modular and organized code.
Executing a PowerShell Script from Another PowerShell Script
To run one PowerShell script from another, you can use several methods depending on your specific requirements. Below are some common approaches along with examples.
Using the Call Operator
The simplest way to invoke another script is by using the call operator (`&`). This allows you to execute the script in the current scope.
“`powershell
& “C:\Path\To\Your\Script.ps1”
“`
Advantages:
- Maintains the current scope and environment.
- Variables and functions defined in the calling script remain accessible.
Using the Dot-Sourcing Method
Dot-sourcing is another way to run a script that also keeps its variables and functions in the current scope.
“`powershell
. “C:\Path\To\Your\Script.ps1”
“`
Use Case:
- When you need to load functions or variables defined in the called script.
Using Start-Process for Independent Execution
If you prefer to run a script independently, use the `Start-Process` cmdlet. This method launches the script in a new PowerShell process.
“`powershell
Start-Process powershell.exe -ArgumentList “-File C:\Path\To\Your\Script.ps1”
“`
Benefits:
- The calling script will continue running without waiting for the called script to finish.
- Useful for running scripts asynchronously.
Passing Parameters to the Called Script
You can pass parameters to the called script in both the call operator and the dot-sourcing methods.
“`powershell
Example of parameters
param (
[string]$Name,
[int]$Age
)
& “C:\Path\To\Your\Script.ps1” -Name “John” -Age 30
“`
Parameter Handling:
- Ensure the called script is set up to accept the parameters using the `param` block.
Example of a Calling Script
Below is an example of a calling script that utilizes the call operator to execute another script.
“`powershell
MainScript.ps1
$scriptPath = “C:\Path\To\Your\SecondScript.ps1”
& $scriptPath -Name “Alice” -Age 28
“`
Using Try-Catch for Error Handling
Incorporating error handling can help manage issues that may arise during script execution.
“`powershell
try {
& “C:\Path\To\Your\Script.ps1”
} catch {
Write-Host “An error occurred: $_”
}
“`
Error Management:
- The `try-catch` block allows you to handle exceptions gracefully and provide feedback.
Each method for running a PowerShell script from another has its unique features and use cases. Depending on your needs—whether it’s variable scope, asynchronous execution, or error handling—you can select the most appropriate method to achieve your objectives effectively.
Expert Insights on Running PowerShell Scripts
Dr. Emily Carter (Senior Systems Engineer, Tech Innovations Inc.). “To run another PowerShell script from within a script, you can simply use the `&` operator followed by the path to the script. This method allows for seamless execution and is widely used in automation tasks.”
Michael Thompson (PowerShell Specialist, Cloud Solutions Group). “Utilizing the `Invoke-Expression` cmdlet is another effective way to execute a PowerShell script from another script. This approach can be particularly useful when the script path is stored in a variable, providing flexibility in script management.”
Sarah Jenkins (IT Automation Consultant, Future Tech Advisors). “For more complex scenarios, consider using the `Start-Process` cmdlet. This allows for running scripts in a separate process, which can be beneficial for performance and isolation, especially when dealing with long-running tasks.”
Frequently Asked Questions (FAQs)
How can I run another PowerShell script from within a PowerShell script?
You can run another PowerShell script by using the `&` (call) operator followed by the path to the script. For example: `& “C:\Path\To\YourScript.ps1″`.
Can I pass parameters to the script being called?
Yes, you can pass parameters to the script by including them after the script path. For example: `& “C:\Path\To\YourScript.ps1” -Param1 Value1 -Param2 Value2`.
What happens if the called script has execution policy restrictions?
If the execution policy restricts running scripts, you will encounter an error. You can change the execution policy using `Set-ExecutionPolicy` or run the script with the `-ExecutionPolicy Bypass` flag.
Is there a way to check if the script executed successfully?
Yes, you can check the `$LASTEXITCODE` variable immediately after calling the script. A value of `0` indicates success, while any other value indicates an error.
Can I run multiple scripts sequentially in a single PowerShell script?
Yes, you can run multiple scripts sequentially by calling each script one after the other using the `&` operator. Ensure that each script path is correctly specified.
How can I handle errors when running another PowerShell script?
You can use `try` and `catch` blocks to handle errors. Wrap the script call in a `try` block, and use the `catch` block to manage any exceptions that occur during execution.
utilizing a PowerShell script to execute another PowerShell script is a straightforward yet powerful technique that enhances automation and efficiency in system administration tasks. By leveraging the `&` call operator or the `Start-Process` cmdlet, users can easily run scripts from within other scripts, allowing for modular scripting practices. This approach not only promotes code reusability but also simplifies complex workflows by breaking them down into manageable components.
Moreover, understanding the execution policies and ensuring that scripts are signed or executed in a safe environment is crucial for maintaining security. Users should be aware of the implications of script execution policies and configure them appropriately to prevent unauthorized script execution. Additionally, incorporating error handling and logging mechanisms within the scripts can significantly improve troubleshooting and monitoring capabilities.
Overall, the ability to run one PowerShell script from another is an essential skill for IT professionals and system administrators. It empowers them to create more dynamic and flexible automation solutions, ultimately leading to improved productivity and streamlined operations. By mastering this technique, users can take full advantage of PowerShell’s capabilities to manage and automate their environments effectively.
Author Profile
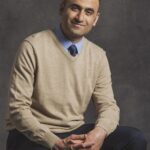
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?