How Can You Split a Line into an Array Using PowerShell?
In the world of scripting and automation, PowerShell stands out as a powerful tool for system administrators and developers alike. One of the common tasks you might encounter is the need to manipulate strings, particularly when you want to break down a line of text into manageable pieces. Whether you’re parsing log files, processing CSV data, or simply organizing information, knowing how to split a line into an array can streamline your workflow and enhance your scripts. In this article, we will delve into the various methods available in PowerShell to achieve this, equipping you with the skills to handle string manipulation with ease.
When working with strings in PowerShell, the ability to split a line into an array can be invaluable. This process allows you to take a single line of text and break it down into individual components based on specified delimiters. Understanding how to effectively use the `Split` method or the `-split` operator can open up a new realm of possibilities for data processing and manipulation. From handling simple text files to more complex data structures, mastering this skill can significantly improve your scripting efficiency.
As we explore the different techniques for splitting lines into arrays, we will also touch on best practices and common pitfalls to avoid. Whether you are a beginner looking to enhance your PowerShell skills or
Using the Split Method
In PowerShell, the most common way to split a string into an array is by using the `Split` method. This method can divide a string based on a specified delimiter, which can be a character, string, or regular expression.
To use the `Split` method, you can follow this syntax:
“`powershell
$array = “string1,string2,string3” -split “,”
“`
This command will create an array with the elements `string1`, `string2`, and `string3`. PowerShell automatically handles the result as an array, making it easy to work with the individual elements.
Using the Split Operator
An alternative to the `Split` method is the split operator `-split`. This operator provides flexibility and is often more straightforward in scripts.
Example:
“`powershell
$array = “apple banana cherry” -split ” ”
“`
In this example, the string is split at each space, resulting in an array containing `apple`, `banana`, and `cherry`.
Defining Delimiters
You can specify different delimiters by adjusting the parameters of the `-split` operator or the `Split` method. Here are some common examples:
- To split by a single character:
“`powershell
$array = “a;b;c” -split “;”
“`
- To split by multiple characters:
“`powershell
$array = “one,two;three|four” -split “[,;|]”
“`
- To split using a regular expression:
“`powershell
$array = “word1 word2,word3.word4” -split “\W+”
“`
Handling Empty Entries
When splitting strings, you may encounter empty entries in the resulting array. You can use the `-split` operator with an additional parameter to control how empty entries are handled.
Example:
“`powershell
$array = “apple,,banana” -split “,”, [System.StringSplitOptions]::RemoveEmptyEntries
“`
This will produce an array with only `apple` and `banana`, excluding the empty entry.
Example Table of Split Scenarios
The following table illustrates various scenarios for splitting strings using different delimiters:
Input String | Delimiter | Resulting Array |
---|---|---|
“red;green;blue” | ; | red, green, blue |
“cat, dog, mouse” | , | cat, dog, mouse |
“one|two|three” | | | one, two, three |
“apple banana cherry” | apple, banana, cherry |
This table summarizes how the input string is split based on the specified delimiter, providing clear examples of how the `Split` method and `-split` operator function in PowerShell.
Using PowerShell to Split Lines into Arrays
PowerShell provides various methods to split strings into arrays, particularly useful for processing text data. The most commonly utilized method is the `-split` operator, which allows for flexible string manipulation.
Basic Syntax of the `-split` Operator
The `-split` operator can split a string based on a specified delimiter. The basic syntax is:
“`powershell
$array = $string -split $delimiter
“`
- $string: The string you want to split.
- $delimiter: The character or regular expression that specifies where to split the string.
Examples of String Splitting
Here are some practical examples demonstrating how to use the `-split` operator effectively:
- Splitting by Space:
Splitting a string into words using space as the delimiter.
“`powershell
$text = “PowerShell is powerful”
$array = $text -split ” ”
“`
Result:
- `PowerShell`
- `is`
- `powerful`
- Splitting by Comma:
Useful for processing CSV-like data.
“`powershell
$csv = “name,age,city”
$array = $csv -split “,”
“`
Result:
- `name`
- `age`
- `city`
- Using Regular Expressions:
You can use regex for more complex splitting.
“`powershell
$text = “word1;word2,word3.word4”
$array = $text -split “[;,\.]”
“`
Result:
- `word1`
- `word2`
- `word3`
- `word4`
Advanced Splitting Options
PowerShell allows for additional parameters to control the split behavior:
- Count: Limits the number of splits.
“`powershell
$text = “one,two,three,four”
$array = $text -split “,”, 2
“`
Result:
- `one`
- `two,three,four`
- Remove Empty Entries: To avoid empty entries in the resulting array, you can utilize regex.
“`powershell
$text = “a,,b,,c”
$array = $text -split “,+” This removes empty entries
“`
Result:
- `a`
- `b`
- `c`
Handling New Lines
To split a string by new lines, use the newline character (`”`n”`”) as the delimiter:
“`powershell
$text = “Line1`nLine2`nLine3”
$array = $text -split “`n”
“`
Result:
- `Line1`
- `Line2`
- `Line3`
Converting the Array to a String
If you need to convert the array back into a single string, you can use the `-join` operator:
“`powershell
$array = @(“PowerShell”, “is”, “great”)
$string = $array -join ” ”
“`
Result:
- `PowerShell is great`
PowerShell’s `-split` operator, combined with its support for regular expressions, provides powerful tools for dividing strings into manageable arrays. Whether working with simple text or complex data formats, mastering these techniques can significantly enhance scripting efficiency and data manipulation capabilities.
Expert Insights on Splitting Lines into Arrays in PowerShell
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In PowerShell, the `-split` operator is a powerful tool for dividing a string into an array based on specified delimiters. Understanding how to effectively utilize this operator can significantly enhance data manipulation capabilities in scripts.”
Michael Thompson (PowerShell Specialist, Scripting Solutions LLC). “When splitting lines into arrays, it is crucial to consider the context of the data. For instance, using `-split` with regex patterns allows for more complex splitting scenarios, which can be particularly useful when dealing with structured text files.”
Linda Garcia (IT Consultant, CloudTech Advisors). “Properly handling the output of a split operation is essential. After splitting a line into an array, leveraging methods such as `ForEach-Object` can streamline further processing, enabling efficient data handling in automation tasks.”
Frequently Asked Questions (FAQs)
How can I split a string into an array in PowerShell?
You can use the `-split` operator to divide a string into an array based on a specified delimiter. For example, `$array = “apple,banana,cherry” -split “,”` will create an array with three elements: “apple”, “banana”, and “cherry”.
What is the default delimiter for the `-split` operator?
The default delimiter for the `-split` operator is whitespace. If no delimiter is specified, it will split the string at spaces, tabs, and line breaks.
Can I use regular expressions with the `-split` operator?
Yes, the `-split` operator supports regular expressions, allowing for more complex splitting criteria. For instance, `$array = “one1two2three” -split “\d”` will split the string at each digit.
How do I split a multiline string into an array in PowerShell?
You can split a multiline string using the `-split` operator with the newline character as the delimiter. For example, `$array = “line1`nline2`nline3” -split “`n”` will result in an array with three elements.
What happens if the string contains consecutive delimiters?
If the string contains consecutive delimiters, the `-split` operator will create empty elements in the resulting array. For example, `”a,,b” -split “,”` will produce an array with elements: “a”, “”, “b”.
How can I remove empty elements from the resulting array?
You can use the `Where-Object` cmdlet to filter out empty elements. For example, `$array = “a,,b” -split “,” | Where-Object { $_ -ne “” }` will yield an array containing only “a” and “b”.
PowerShell provides a robust set of tools for string manipulation, making it straightforward to split lines into arrays. The primary method for achieving this is by utilizing the `-split` operator, which allows users to define a delimiter that separates the elements of the string. This operator is versatile and can handle various data types, including strings with spaces, commas, or other specified characters.
Another useful approach is to employ the `Split()` method of the string class. This method offers additional parameters, such as the ability to limit the number of substrings returned or to specify whether to remove empty entries from the resulting array. Both methods are efficient and can be easily integrated into scripts for data processing or automation tasks.
understanding how to split lines into arrays in PowerShell is essential for effective data manipulation and analysis. By leveraging the `-split` operator or the `Split()` method, users can enhance their scripting capabilities, streamline workflows, and improve the overall efficiency of their PowerShell scripts. Mastery of these techniques is invaluable for anyone looking to work with text data in a PowerShell environment.
Author Profile
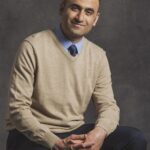
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?