How Can You Use PowerShell to Test If a File Exists?
In the world of automation and system administration, PowerShell stands out as a powerful tool for managing and manipulating files and systems. One of the most fundamental tasks that IT professionals and developers often encounter is the need to check whether a file exists before performing operations on it. This seemingly simple action can have significant implications for error handling, script efficiency, and overall system performance. As we delve into the intricacies of PowerShell, understanding how to effectively test for file existence will empower you to write more robust and reliable scripts.
PowerShell provides a variety of methods to determine if a file is present on the system, catering to different user needs and scenarios. Whether you’re working with scripts that require conditional logic based on file availability or simply looking to validate user inputs, knowing how to check for file existence is a crucial skill. The commands and techniques available in PowerShell not only streamline these processes but also enhance the functionality of your scripts, allowing for more dynamic and responsive automation solutions.
As we explore this topic further, we will uncover the various approaches to checking file existence in PowerShell, including built-in cmdlets and best practices for error handling. By mastering these techniques, you can ensure that your scripts run smoothly, avoid unnecessary errors, and ultimately save time and resources in your workflows. Join us
Using PowerShell to Check for File Existence
To determine if a file exists in PowerShell, you can utilize the `Test-Path` cmdlet. This cmdlet returns a Boolean value, indicating whether the specified path exists. The path can be either a file or a directory.
The basic syntax for using `Test-Path` is as follows:
“`powershell
Test-Path -Path “C:\path\to\your\file.txt”
“`
If the file exists, the command will return `True`; if it does not, it will return “.
Examples of File Existence Checks
Here are some practical examples of how to use `Test-Path` to check for file existence:
- Check for a specific file:
“`powershell
$filePath = “C:\Users\Public\Documents\example.txt”
if (Test-Path -Path $filePath) {
“The file exists.”
} else {
“The file does not exist.”
}
“`
- Check for multiple files:
You can also check for multiple files in a loop:
“`powershell
$filePaths = @(“C:\file1.txt”, “C:\file2.txt”, “C:\file3.txt”)
foreach ($file in $filePaths) {
if (Test-Path -Path $file) {
“$file exists.”
} else {
“$file does not exist.”
}
}
“`
Handling File Paths
When working with file paths, it’s important to consider both absolute and relative paths. Here’s how they differ:
Path Type | Description |
---|---|
Absolute Path | A complete path that specifies the location from the root directory (e.g., `C:\Users\Public\Documents\example.txt`). |
Relative Path | A path that is relative to the current directory (e.g., `.\example.txt` if the file is in the current directory). |
Using Wildcards with Test-Path
PowerShell also supports wildcards with `Test-Path`. This allows you to check for the existence of files matching specific patterns. For example:
“`powershell
$directoryPath = “C:\Users\Public\Documents\*.txt”
if (Test-Path -Path $directoryPath) {
“There are .txt files in the directory.”
} else {
“No .txt files found.”
}
“`
This command checks if there are any `.txt` files in the specified directory.
Using PowerShell to test if a file exists is straightforward with the `Test-Path` cmdlet. By utilizing this cmdlet effectively, you can enhance your scripts and automate tasks that depend on file presence.
Using PowerShell to Check File Existence
In PowerShell, checking if a file exists can be accomplished with a few straightforward methods. The most common approach is to utilize the `Test-Path` cmdlet. This cmdlet is efficient and easy to use.
Basic Syntax of Test-Path
The basic syntax for the `Test-Path` cmdlet is as follows:
“`powershell
Test-Path -Path “C:\path\to\your\file.txt”
“`
This command returns a boolean value: `True` if the file exists and “ if it does not.
Examples of Test-Path in Use
Here are several examples demonstrating the usage of `Test-Path`:
- Check for a specific file:
“`powershell
if (Test-Path -Path “C:\example\file.txt”) {
Write-Host “File exists.”
} else {
Write-Host “File does not exist.”
}
“`
- Check for a directory:
“`powershell
if (Test-Path -Path “C:\example\directory”) {
Write-Host “Directory exists.”
} else {
Write-Host “Directory does not exist.”
}
“`
- Using a variable for the path:
“`powershell
$filePath = “C:\example\file.txt”
if (Test-Path -Path $filePath) {
Write-Host “File exists.”
} else {
Write-Host “File does not exist.”
}
“`
Checking Multiple Files
To check the existence of multiple files, you can use a loop:
“`powershell
$files = @(“C:\example\file1.txt”, “C:\example\file2.txt”, “C:\example\file3.txt”)
foreach ($file in $files) {
if (Test-Path -Path $file) {
Write-Host “$file exists.”
} else {
Write-Host “$file does not exist.”
}
}
“`
Using Try-Catch for Error Handling
In some scenarios, you may want to include error handling, especially when dealing with paths that may lead to exceptions:
“`powershell
try {
if (Test-Path -Path “C:\example\file.txt”) {
Write-Host “File exists.”
} else {
Write-Host “File does not exist.”
}
} catch {
Write-Host “An error occurred: $_”
}
“`
Performance Considerations
When using `Test-Path`, consider the following:
- Efficiency: `Test-Path` is optimized for performance and can check file or directory existence quickly.
- Network Paths: When checking network paths, the response time may vary depending on network speed.
- Wildcard Support: `Test-Path` supports wildcards. For example:
“`powershell
Test-Path “C:\example\*.txt”
“`
This checks if any `.txt` files exist in the specified directory.
Utilizing `Test-Path` in PowerShell provides a reliable way to check for file and directory existence. With its straightforward syntax and flexible usage, it can easily be integrated into scripts for various administrative tasks.
Expert Insights on Using PowerShell to Check File Existence
Jessica Thompson (Senior Systems Administrator, Tech Solutions Inc.). “In PowerShell, checking if a file exists is a fundamental task that can be accomplished using the Test-Path cmdlet. This cmdlet returns a Boolean value, allowing scripts to make decisions based on the presence of files, which is crucial for automation and error handling.”
Michael Chen (DevOps Engineer, Cloud Innovations). “Utilizing PowerShell to verify file existence not only streamlines workflows but also enhances reliability in deployment scripts. By incorporating Test-Path, developers can avoid unnecessary errors and ensure that their scripts behave as expected under various conditions.”
Linda Garcia (IT Security Consultant, SecureTech). “From a security perspective, using PowerShell to check for file existence is essential for validating the integrity of critical files. By implementing checks at the start of scripts, organizations can prevent unauthorized access and modifications to sensitive data.”
Frequently Asked Questions (FAQs)
How can I test if a file exists in PowerShell?
You can test if a file exists in PowerShell using the `Test-Path` cmdlet. For example, `Test-Path “C:\path\to\your\file.txt”` will return `True` if the file exists and “ if it does not.
What is the syntax for using Test-Path in a script?
The syntax is straightforward: `if (Test-Path “C:\path\to\your\file.txt”) { Write-Host “File exists” } else { Write-Host “File does not exist” }`. This checks the file’s existence and executes the corresponding block of code.
Can I check for the existence of a directory using Test-Path?
Yes, `Test-Path` can also be used to check for the existence of directories. For instance, `Test-Path “C:\path\to\your\directory”` will return `True` if the directory exists.
Is there a way to check for multiple files at once?
You can check for multiple files by using a loop. For example:
“`powershell
$files = @(“C:\file1.txt”, “C:\file2.txt”)
foreach ($file in $files) {
if (Test-Path $file) {
Write-Host “$file exists”
} else {
Write-Host “$file does not exist”
}
}
“`
What happens if I provide an invalid path to Test-Path?
If you provide an invalid path to `Test-Path`, it will return “. No error will be thrown; it simply indicates that the specified path does not exist.
Can I use wildcards with Test-Path?
No, `Test-Path` does not support wildcards directly. It checks for the existence of a specific path. To check for files matching a pattern, consider using `Get-ChildItem` with the `-Filter` parameter instead.
testing if a file exists in PowerShell is a fundamental task that can be accomplished using various methods. The most common approach is utilizing the `Test-Path` cmdlet, which provides a straightforward and efficient way to check for the presence of a file or directory. This cmdlet returns a Boolean value, indicating whether the specified path exists, making it easy to incorporate into conditional statements for further processing.
Another method involves using the .NET framework, specifically the `System.IO.File` class, which offers additional functionalities for file handling. By invoking the `Exists` method, users can achieve similar results while also gaining access to other file-related operations. This approach may be preferable in scenarios where more complex file manipulations are required alongside existence checks.
Key takeaways from this discussion include the importance of selecting the appropriate method based on the specific requirements of the task at hand. While `Test-Path` is generally sufficient for basic existence checks, leveraging .NET methods can provide enhanced capabilities for advanced scripting needs. Furthermore, understanding these techniques can significantly improve the efficiency and reliability of PowerShell scripts in various automation tasks.
Author Profile
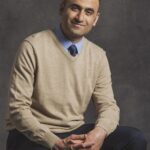
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?