How Can I Use PowerShell to Check if a File Exists?
In the realm of system administration and automation, PowerShell stands out as a powerful tool that enables users to manage and manipulate their Windows environments with ease. One of the most fundamental tasks that administrators often encounter is checking for the existence of files. Whether you’re ensuring that a critical configuration file is in place, verifying the presence of logs for troubleshooting, or simply managing file-based operations, knowing how to efficiently check if a file exists can save time and prevent errors in your scripts.
This article delves into the various methods available in PowerShell to determine if a file exists on your system. From straightforward commands that provide instant feedback to more complex scripts that can handle multiple conditions, we will explore the capabilities of PowerShell in this essential task. Understanding these techniques not only enhances your scripting skills but also empowers you to create more robust and error-resistant automation processes.
As we navigate through the different approaches to file existence checks in PowerShell, we will highlight best practices and common pitfalls to avoid. Whether you are a seasoned IT professional or a newcomer to the world of scripting, the insights provided here will equip you with the knowledge you need to streamline your workflows and improve your operational efficiency. Get ready to unlock the potential of PowerShell as we embark on this journey to master file existence checks!
Using PowerShell to Check File Existence
In PowerShell, checking for the existence of a file is a common task that can be accomplished with the `Test-Path` cmdlet. This cmdlet returns a Boolean value indicating whether the specified path points to an existing file or directory. The syntax is straightforward and can be adapted for various use cases, such as scripts that require verification of file presence before proceeding with operations.
To check if a specific file exists, use the following command:
“`powershell
Test-Path “C:\path\to\your\file.txt”
“`
This command will return `True` if the file exists and “ if it does not.
Checking Multiple Files
You may also want to check multiple files at once. This can be achieved by passing an array of file paths to the `Test-Path` cmdlet. Here’s how you can do it:
“`powershell
$files = “C:\path\to\file1.txt”, “C:\path\to\file2.txt”, “C:\path\to\file3.txt”
$results = $files | ForEach-Object { Test-Path $_ }
“`
The `$results` variable will contain an array of Boolean values corresponding to the existence of each file.
Using Conditional Statements
In practical scenarios, you often want to take action based on whether a file exists. This can be done using conditional statements. Here’s an example:
“`powershell
if (Test-Path “C:\path\to\your\file.txt”) {
Write-Host “File exists.”
} else {
Write-Host “File does not exist.”
}
“`
This code block checks if the specified file exists and outputs a message accordingly.
Handling Errors and Exceptions
While checking for file existence is generally straightforward, it’s good practice to handle potential errors. For instance, if the path is malformed or inaccessible, you should consider wrapping your commands in a try-catch block:
“`powershell
try {
if (Test-Path “C:\path\to\your\file.txt”) {
Write-Host “File exists.”
} else {
Write-Host “File does not exist.”
}
} catch {
Write-Host “An error occurred: $_”
}
“`
This ensures that your script remains robust and can handle unexpected situations gracefully.
Example Scenarios
Here is a summary table of various scenarios where you might check for file existence using PowerShell:
Scenario | PowerShell Command |
---|---|
Check a single file | Test-Path “C:\path\to\file.txt” |
Check multiple files | $files | ForEach-Object { Test-Path $_ } |
Conditional action based on existence |
if (Test-Path "C:\path\to\file.txt") { ... }
|
Error handling |
try { Test-Path "C:\path\to\file.txt" } catch { ... }
|
Using these commands and techniques, you can efficiently manage file existence checks within your PowerShell scripts, ensuring that your operations are both safe and reliable.
Using PowerShell to Check for File Existence
In PowerShell, determining whether a file exists can be efficiently accomplished using the `Test-Path` cmdlet. This cmdlet evaluates the specified path and returns a Boolean value indicating whether the file or directory exists.
Basic Syntax
The basic syntax for checking if a file exists is as follows:
“`powershell
Test-Path -Path “C:\Path\To\Your\File.txt”
“`
This command returns `True` if the file exists and “ if it does not.
Examples of File Existence Checks
Here are a few practical examples of how to use `Test-Path`:
- Check for a specific file:
“`powershell
$fileExists = Test-Path -Path “C:\Documents\Report.docx”
if ($fileExists) {
Write-Host “The file exists.”
} else {
Write-Host “The file does not exist.”
}
“`
- Check for a file using a variable:
“`powershell
$filePath = “C:\Users\Username\Desktop\example.txt”
if (Test-Path -Path $filePath) {
Write-Host “The file exists.”
} else {
Write-Host “The file does not exist.”
}
“`
Checking Multiple File Paths
You can also check multiple file paths simultaneously. This is particularly useful when validating the existence of several files:
“`powershell
$files = “C:\File1.txt”, “C:\File2.txt”, “C:\File3.txt”
foreach ($file in $files) {
if (Test-Path -Path $file) {
Write-Host “$file exists.”
} else {
Write-Host “$file does not exist.”
}
}
“`
Using Wildcards with Test-Path
`Test-Path` also supports wildcard characters, allowing for pattern matching in file names:
“`powershell
$directoryPath = “C:\Reports\*.pdf”
if (Test-Path -Path $directoryPath) {
Write-Host “PDF files exist in the Reports directory.”
} else {
Write-Host “No PDF files found in the Reports directory.”
}
“`
Return Values and Error Handling
The `Test-Path` cmdlet returns a Boolean value (`True` or “). You can handle errors or unexpected results by implementing try-catch blocks:
“`powershell
try {
if (Test-Path -Path “C:\Path\To\File.txt”) {
Write-Host “File exists.”
} else {
Write-Host “File does not exist.”
}
} catch {
Write-Host “An error occurred: $_”
}
“`
Utilizing `Test-Path` in PowerShell provides a simple yet effective way to check for file existence. By mastering this cmdlet, you can enhance your scripting capabilities and ensure your scripts run as expected without encountering file-not-found errors.
Expert Insights on Using PowerShell to Check File Existence
Emily Carter (Senior Systems Administrator, Tech Solutions Inc.). “Utilizing PowerShell to check if a file exists is a fundamental skill for any systems administrator. The command ‘Test-Path’ is efficient and straightforward, allowing for quick verification of file presence without the need for complex scripting.”
Michael Chen (DevOps Engineer, Cloud Innovators). “Incorporating PowerShell scripts into your workflow for file existence checks can significantly streamline processes. By leveraging ‘Test-Path’, you can automate tasks and ensure that your scripts execute only when necessary files are present, thus enhancing overall efficiency.”
Sarah Patel (Cybersecurity Analyst, SecureTech Labs). “When checking for file existence with PowerShell, it is crucial to consider the security implications. Using ‘Test-Path’ not only verifies file presence but can also be integrated with security protocols to ensure that sensitive files are accessed appropriately, maintaining data integrity.”
Frequently Asked Questions (FAQs)
How can I check if a file exists using PowerShell?
You can use the `Test-Path` cmdlet in PowerShell. For example, `Test-Path “C:\path\to\your\file.txt”` will return `True` if the file exists and “ if it does not.
What is the syntax for checking a file’s existence in PowerShell?
The syntax is straightforward: `Test-Path “full\path\to\file”`. Replace `”full\path\to\file”` with the actual path of the file you want to check.
Can I check for the existence of a directory using PowerShell?
Yes, `Test-Path` can be used to check for both files and directories. Simply provide the path to the directory, and it will return `True` if it exists.
What will happen if I check for a file that is on a network drive?
`Test-Path` works with network drives as long as the drive is accessible. Ensure you have the correct permissions and the network path is valid.
Is there a way to check for multiple files at once in PowerShell?
Yes, you can use a loop or the `ForEach-Object` cmdlet to check multiple files. For example: `@(“file1.txt”, “file2.txt”) | ForEach-Object { Test-Path $_ }`.
What should I do if `Test-Path` returns an error?
If `Test-Path` returns an error, verify the file path for accuracy, check your permissions, and ensure that the file or directory is not on a disconnected drive.
In summary, utilizing PowerShell to check if a file exists is a straightforward yet powerful task that can significantly enhance automation and scripting capabilities. The most common method involves using the `Test-Path` cmdlet, which provides a simple and effective way to verify the presence of a file or directory. This cmdlet returns a Boolean value, indicating whether the specified path exists, which can be particularly useful in conditional statements within scripts.
Additionally, PowerShell offers flexibility in specifying paths, allowing users to check for files on local drives, network locations, or even within cloud storage solutions. By employing this functionality, users can streamline their workflows, automate file management tasks, and implement error handling to manage scenarios where files may be missing. This enhances the reliability of scripts and reduces the likelihood of runtime errors.
mastering the use of PowerShell for file existence checks is a fundamental skill for IT professionals and system administrators. It not only aids in maintaining efficient operations but also empowers users to create more robust and error-resistant scripts. By leveraging the capabilities of PowerShell, users can ensure that their automation processes are both effective and reliable.
Author Profile
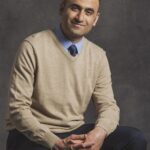
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?