How Can I Use PowerShell to Ensure a Command Completes Before Moving On?
In the realm of system administration and automation, PowerShell stands out as a powerful tool that enables users to manage and configure systems with remarkable efficiency. However, as with any scripting language, understanding how to control the flow of commands is crucial for effective execution. One common challenge that many PowerShell users encounter is the need to ensure that a command has completed its execution before proceeding to the next one. This is where the concept of waiting for a command to finish becomes essential, allowing for smoother workflows and preventing potential errors that could arise from running commands in an unintended sequence.
When executing scripts or commands in PowerShell, it’s not uncommon to have tasks that depend on the successful completion of previous commands. Without proper control, you might find yourself facing race conditions, where subsequent commands are executed before the prior ones have finished processing. This can lead to unexpected results, especially in complex scripts that involve multiple steps or external processes. Understanding how to implement waiting mechanisms is key to building robust and reliable PowerShell scripts.
In this article, we will explore various methods to ensure that commands in PowerShell complete their execution before moving forward. From leveraging built-in cmdlets to employing asynchronous techniques, we will delve into the tools and strategies that can help you manage command execution effectively. Whether you’re a seasoned administrator or
Using Start-Process with Wait
One of the most straightforward methods to wait for a command or process to finish in PowerShell is by using the `Start-Process` cmdlet with the `-Wait` parameter. This method is particularly useful for executing external applications and ensuring that the script execution pauses until the external command completes.
Here’s how you can implement it:
“`powershell
Start-Process -FilePath “notepad.exe” -Wait
“`
In this example, the script will launch Notepad and will not proceed until Notepad is closed. This technique is beneficial in scenarios where subsequent commands depend on the completion of the initial command.
Using the Call Operator
Another method to wait for a command to finish is by using the call operator `&`. When you invoke a script or command using the call operator, PowerShell will wait for that command to complete before proceeding.
Example usage:
“`powershell
& “C:\Path\To\YourScript.ps1”
“`
In this scenario, the script located at the specified path will be executed, and PowerShell will wait for it to finish before executing any subsequent commands.
Job Management with Start-Job
If you want to run a command in the background but still need to wait for its completion, you can utilize `Start-Job` and `Receive-Job`. This approach is particularly useful for running long-running processes without blocking the console.
“`powershell
$job = Start-Job -ScriptBlock { Start-Sleep -Seconds 10 }
Wait-Job -Job $job
Receive-Job -Job $job
“`
In this example, a job is started that sleeps for 10 seconds, and the main script waits for the job to complete. After completion, it retrieves the output of the job.
Using Invoke-Expression
For executing commands stored in strings, `Invoke-Expression` can be used. This command will evaluate the string as a command and wait for it to finish.
Example:
“`powershell
$command = “Get-Process”
Invoke-Expression $command
“`
In this case, the command stored in the variable `$command` is executed, and PowerShell will wait until it is completed.
Comparison of Methods
The following table summarizes the various methods for waiting for commands to finish in PowerShell:
Method | Use Case | Example |
---|---|---|
Start-Process | Launching external applications | Start-Process -FilePath “notepad.exe” -Wait |
Call Operator (&) | Running scripts or commands directly | & “C:\Path\To\YourScript.ps1” |
Start-Job | Running commands in the background | $job = Start-Job -ScriptBlock { Start-Sleep -Seconds 10 } |
Invoke-Expression | Executing string commands | Invoke-Expression $command |
Each method serves a distinct purpose, and the choice depends on the specific requirements of your PowerShell script and how you want to manage command execution flow.
Understanding PowerShell’s Execution Flow
PowerShell scripts and commands run sequentially by default. This means that when you execute a command, PowerShell waits for that command to finish before proceeding to the next one. However, in some cases, you may want to ensure that a command has completed before executing subsequent commands, especially when dealing with background jobs or asynchronous operations.
Using the Start-Process Cmdlet
The `Start-Process` cmdlet allows you to start a process and optionally wait for it to complete. By default, this cmdlet does not wait for the process to finish, but you can use the `-Wait` parameter to achieve this.
Example:
“`powershell
Start-Process “notepad.exe” -Wait
“`
In this example, PowerShell will launch Notepad and wait until it is closed before moving on to the next command.
Handling Background Jobs
When using background jobs in PowerShell, the `Start-Job` cmdlet runs the job asynchronously. To ensure that your script waits for the job to finish, use the `Wait-Job` cmdlet.
Example:
“`powershell
$job = Start-Job -ScriptBlock { Get-Process }
Wait-Job -Job $job
Receive-Job -Job $job
“`
In this example:
- `Start-Job` initiates a job that retrieves the list of processes.
- `Wait-Job` pauses the script until the job is complete.
- `Receive-Job` retrieves the output of the completed job.
Using the Wait-Process Cmdlet
The `Wait-Process` cmdlet is particularly useful when you want to wait for a specific process to finish. It can be used to pause execution until a specified process ends.
Example:
“`powershell
Start-Process “notepad.exe”
Wait-Process -Name “notepad”
“`
In this example, PowerShell starts Notepad and then waits until the Notepad process is closed before executing further commands.
Waiting for External Commands
When executing external commands or scripts, you can use the `&` operator, which runs the command and waits for it to complete.
Example:
“`powershell
& “C:\Path\To\YourScript.ps1”
“`
This command executes the specified PowerShell script and waits for it to finish before moving on.
Best Practices for Waiting on Commands
- Use `-Wait` with `Start-Process`: This is the most straightforward approach for GUI applications.
- Employ `Wait-Job`: Ideal for handling background jobs where you need to ensure completion.
- Utilize `Wait-Process`: Use this for waiting on specific processes, especially useful in scripts that interact with multiple applications.
- Monitor Job States: If using jobs, periodically check the job state using `Get-Job` for more complex workflows.
Cmdlet | Purpose |
---|---|
`Start-Process` | Starts a process, optionally waits for it. |
`Wait-Job` | Pauses execution until a background job is complete. |
`Wait-Process` | Waits for a specific running process to terminate. |
By utilizing these methods, you can effectively manage execution flow in PowerShell scripts, ensuring that commands complete as intended before proceeding.
Understanding Command Execution in PowerShell
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In PowerShell, the `Start-Process` cmdlet allows for asynchronous execution, but to wait for a command to finish, one must use the `-Wait` parameter. This ensures that the script pauses until the initiated process completes, which is critical for managing dependencies in automated workflows.”
Michael Tran (DevOps Specialist, Cloud Solutions Ltd.). “Utilizing the `&` operator in PowerShell can lead to concurrent execution of commands. However, if you need to ensure that one command finishes before another begins, employing the `Wait-Process` cmdlet is essential. This approach is particularly useful in CI/CD pipelines where the order of operations can affect deployment success.”
Lisa Chen (PowerShell Expert and Author, Scripting Mastery). “For scripts that require precise timing and resource management, the `Start-Sleep` cmdlet can be used in conjunction with command execution to create intentional pauses. However, for scenarios where command completion is the priority, leveraging the `-Wait` parameter or `Wait-Process` is the most effective strategy to ensure that subsequent commands execute only after the previous ones have successfully completed.”
Frequently Asked Questions (FAQs)
How can I make PowerShell wait for a command to finish executing?
You can use the `Start-Process` cmdlet with the `-Wait` parameter. This ensures that PowerShell waits for the specified process to complete before moving on to the next command.
What is the purpose of the `Wait-Process` cmdlet in PowerShell?
The `Wait-Process` cmdlet is used to pause the execution of a script or command until a specified process has finished running. This is useful for synchronizing tasks that depend on the completion of another process.
Can I use `&&` to wait for a command to finish in PowerShell?
No, the `&&` operator is not used in PowerShell for command execution. Instead, you can simply place commands sequentially, as PowerShell will wait for the first command to finish before executing the next one.
Is there a way to check if a command is still running in PowerShell?
Yes, you can use the `Get-Process` cmdlet to check for a specific process by name or ID. If the process is still listed, it means it is still running.
What happens if I run a command without waiting in PowerShell?
If you run a command without waiting, PowerShell will immediately proceed to the next command, which may lead to unexpected results if the subsequent commands depend on the completion of the first command.
How can I handle errors when waiting for a command to finish in PowerShell?
You can use `Try-Catch` blocks to handle errors effectively. This allows you to catch exceptions that may occur while waiting for a command to finish and take appropriate action based on the error.
In PowerShell, managing the execution of commands is crucial for ensuring that scripts run smoothly and efficiently. One of the key aspects of this management is the ability to wait for a command to finish executing before proceeding to the next command. This can be achieved using various methods, such as the `Start-Process` cmdlet with the `-Wait` parameter, or by using the `Wait-Job` cmdlet when working with background jobs. Understanding these techniques is essential for scripting best practices and for avoiding potential issues that may arise from concurrent execution.
Moreover, the ability to wait for a command to complete can significantly enhance the reliability of scripts. It allows for better resource management and ensures that dependent commands do not execute prematurely. This is particularly important in scenarios where the outcome of one command directly influences the execution of subsequent commands. By implementing appropriate waiting mechanisms, users can create more robust and predictable PowerShell scripts.
mastering the concept of waiting for commands in PowerShell is vital for anyone looking to leverage the full power of the scripting environment. By utilizing the available tools and methods effectively, users can enhance their scripting capabilities, leading to improved performance and reduced errors. As PowerShell continues to evolve, staying informed about these functionalities
Author Profile
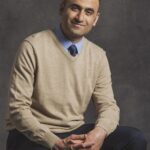
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?