How Can I Write a List to a CSV in a Specific Column Order Using PowerShell?
In the world of data management and automation, PowerShell stands out as a powerful tool for system administrators and developers alike. One common task that many professionals encounter is the need to export data in a structured format, particularly when dealing with lists and collections. Writing lists to CSV files in a specific column order can be crucial for ensuring that the data is not only organized but also easily interpretable by other applications or users. Whether you’re compiling reports, creating logs, or preparing data for analysis, mastering this skill can significantly enhance your workflow.
Understanding how to manipulate and export data in PowerShell is essential for anyone looking to streamline their processes. The ability to write lists to CSV files while maintaining a desired column order allows you to present information clearly and effectively. This process involves not only selecting the right data but also formatting it in a way that meets your specific needs. By leveraging PowerShell’s capabilities, you can automate repetitive tasks, saving time and reducing the likelihood of errors.
As we delve deeper into this topic, we will explore the various methods and best practices for writing lists to CSV files in PowerShell. You’ll learn how to customize the output to match your requirements, ensuring that your data is both accessible and organized. Whether you’re a novice or an experienced user, this guide will equip you
Using PowerShell to Write a List to CSV with Column Order
When working with PowerShell to export data to a CSV file, controlling the order of columns is essential for clarity and usability. By default, PowerShell may not maintain the order of properties in objects, which can lead to confusion when the CSV is opened in applications like Excel. To ensure your data is output in a specific column order, follow these steps.
Defining the Object Properties
Before exporting your list, you should explicitly define the properties you want to include and their order. This can be achieved by creating a custom object that specifies the property order. Here’s an example of how to do this:
“`powershell
Create an array of custom objects
$data = @(
[PSCustomObject]@{ Name = “Alice”; Age = 30; City = “New York” },
[PSCustomObject]@{ Name = “Bob”; Age = 25; City = “Los Angeles” },
[PSCustomObject]@{ Name = “Charlie”; Age = 35; City = “Chicago” }
)
Define the order of properties
$orderedData = $data | Select-Object Name, Age, City
“`
In this example, the `Select-Object` cmdlet is used to specify the desired property order: Name, Age, and City.
Exporting to CSV
Once the object properties are defined in the required order, you can export the data to a CSV file using the `Export-Csv` cmdlet. Here’s how to do it:
“`powershell
Export the ordered data to a CSV file
$orderedData | Export-Csv -Path “output.csv” -NoTypeInformation
“`
The `-NoTypeInformation` parameter suppresses the type information header, making the CSV cleaner and easier to read.
Example Table of Exported Data
When you export the data, the resulting CSV will appear in the following format:
Name | Age | City |
---|---|---|
Alice | 30 | New York |
Bob | 25 | Los Angeles |
Charlie | 35 | Chicago |
This table demonstrates the output structure of the CSV file, ensuring that the columns are in the desired order.
Additional Considerations
When exporting data to CSV using PowerShell, consider the following:
- Data Types: Ensure that data types are consistent across the properties to avoid misinterpretation by applications.
- Special Characters: Handle any special characters in your data appropriately to prevent formatting issues in the CSV.
- Encoding: If you’re dealing with international characters, specify the encoding using the `-Encoding` parameter in the `Export-Csv` cmdlet. For example, `-Encoding UTF8` for UTF-8 encoding.
By following these guidelines, you can effectively manage the order of columns when writing lists to CSV files in PowerShell, enhancing the clarity and usability of your exported data.
Writing a List to CSV in Specific Column Order
When using PowerShell to write a list to a CSV file, you may need to specify the order of columns in the output file. This can be achieved using `Select-Object` to rearrange properties before exporting them.
Example Scenario
Suppose you have a list of objects, each representing a user, with properties such as `Name`, `Age`, and `Email`. You want to export this data to a CSV file but in the order of `Email`, `Name`, `Age`.
PowerShell Command Structure
The following command demonstrates how to write a list to a CSV file in a specified column order:
“`powershell
Sample list of user objects
$users = @(
[PSCustomObject]@{ Name = “John Doe”; Age = 30; Email = “[email protected]” },
[PSCustomObject]@{ Name = “Jane Smith”; Age = 25; Email = “[email protected]” }
)
Exporting to CSV with specified column order
$users | Select-Object Email, Name, Age | Export-Csv -Path “C:\path\to\output.csv” -NoTypeInformation
“`
Explanation of Command Components
- `$users`: An array of custom objects representing user data.
- `Select-Object Email, Name, Age`: This command rearranges the properties of the objects in the desired order.
- `Export-Csv -Path “C:\path\to\output.csv”`: This exports the selected properties to a CSV file, specifying the output file path.
- `-NoTypeInformation`: This flag omits type information from the CSV, resulting in cleaner output.
Customizing Output for Complex Objects
For objects with nested properties or arrays, you can flatten them or create calculated properties. Here’s an example of how to handle a more complex object structure:
“`powershell
Complex object with nested properties
$users = @(
[PSCustomObject]@{ Name = “John Doe”; Age = 30; Email = “[email protected]”; Address = @{ City = “New York”; State = “NY” } },
[PSCustomObject]@{ Name = “Jane Smith”; Age = 25; Email = “[email protected]”; Address = @{ City = “Los Angeles”; State = “CA” } }
)
Exporting with nested properties flattened
$users | Select-Object Email, Name, Age, @{Name=’City’;Expression={$_.Address.City}} | Export-Csv -Path “C:\path\to\output.csv” -NoTypeInformation
“`
In this example, the `Select-Object` command uses a calculated property to extract the city from a nested `Address` object.
Best Practices for CSV Exports
- Always specify the output path: This helps in avoiding confusion about where the file is saved.
- Use `-NoTypeInformation`: This keeps the CSV file clean and focused on the data.
- Validate your data: Ensure that the data structure is consistent before exporting to prevent runtime errors.
- Test with sample data: Run the commands with a small dataset to confirm the output before using larger datasets.
By following these guidelines, you can effectively manage how lists are written to CSV files in PowerShell, ensuring the data is structured in a way that meets your requirements.
Expert Insights on Writing Lists to CSV in PowerShell
Jessica Lin (Senior Software Engineer, Tech Innovations Inc.). “When writing lists to a CSV in PowerShell, it is crucial to define the order of the columns explicitly. This can be achieved by using the `Select-Object` cmdlet to specify the properties in the desired sequence before exporting the data.”
Michael Carter (Data Analyst, Insights Analytics Group). “Utilizing the `Export-Csv` cmdlet in PowerShell allows for seamless data exportation. However, to maintain the column order, ensure that the objects being exported are structured correctly, as the order of properties in the object will dictate the CSV column arrangement.”
Linda Patel (PowerShell Specialist, Scripting Solutions). “For optimal results when writing lists to a CSV file, I recommend creating a custom object that explicitly defines the properties in the order you want them. This approach guarantees that the output matches your expectations, regardless of the input data structure.”
Frequently Asked Questions (FAQs)
How can I write a list to a CSV file using PowerShell?
You can use the `Export-Csv` cmdlet in PowerShell to write a list to a CSV file. For example, you can pipe your list to `Export-Csv -Path “output.csv” -NoTypeInformation`.
What is the default column order when exporting to CSV in PowerShell?
The default column order in PowerShell when exporting to CSV is based on the order of properties in the object. If you want to specify a different order, you need to create a custom object.
How do I specify the order of columns when writing to a CSV file?
To specify the order of columns, create a custom object with the desired property order. For example:
“`powershell
$customObject = [PSCustomObject]@{ Column1 = ‘Value1’; Column2 = ‘Value2’ }
$customObject | Export-Csv -Path “output.csv” -NoTypeInformation
“`
Can I append data to an existing CSV file in PowerShell?
Yes, you can append data to an existing CSV file by using the `-Append` parameter with the `Export-Csv` cmdlet. For example:
“`powershell
$myData | Export-Csv -Path “output.csv” -NoTypeInformation -Append
“`
What happens if the CSV file already exists when I export data?
If the CSV file already exists, using `Export-Csv` without the `-Append` parameter will overwrite the existing file. To prevent data loss, ensure you use the `-Append` parameter if you intend to add to the file.
Is it possible to export a list of objects with specific properties to a CSV file?
Yes, you can select specific properties to export by using the `Select-Object` cmdlet before piping to `Export-Csv`. For example:
“`powershell
$myList | Select-Object Property1, Property2 | Export-Csv -Path “output.csv” -NoTypeInformation
“`
In summary, utilizing PowerShell to write lists to a CSV file in a specific column order is a straightforward yet powerful task. PowerShell provides various cmdlets, such as `Export-Csv`, which enable users to easily export data to CSV format. By carefully structuring the data into objects and defining the desired column order, users can ensure that the resulting CSV file meets their specific requirements. This capability is particularly useful for data reporting and analysis, where the arrangement of information can significantly impact clarity and usability.
One of the key takeaways is the importance of creating custom objects in PowerShell. By defining properties in the desired order before exporting to CSV, users can control how the data appears in the final output. This practice not only enhances the readability of the CSV file but also ensures that the data is organized in a manner that aligns with user expectations. Additionally, understanding how to manipulate and format data within PowerShell can lead to more efficient workflows and better data management practices.
Furthermore, leveraging PowerShell’s capabilities to handle CSV files opens up a range of possibilities for automation and integration with other systems. Users can easily script repetitive tasks, such as data extraction and reporting, which can save time and reduce the likelihood of errors. Overall,
Author Profile
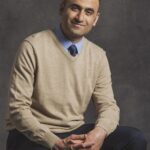
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?