Is Printing Finally Supported in Slurm Python?
In the ever-evolving landscape of high-performance computing, the integration of user-friendly features into complex systems is a welcome development. One such advancement is the recent of printing capabilities in SLURM (Simple Linux Utility for Resource Management) through Python. This long-awaited feature not only enhances the usability of SLURM for researchers and developers but also streamlines the process of job management and output handling in computational environments. As the demand for efficient resource allocation and job monitoring grows, understanding how to leverage these new capabilities can significantly improve workflow and productivity.
The addition of printing functionality in SLURM via Python marks a pivotal moment for users who rely on this powerful job scheduler. Traditionally, SLURM’s command-line interface posed challenges for those seeking to capture and manage output effectively. With the new Python integration, users can now easily implement print statements within their scripts, allowing for real-time feedback and debugging during job execution. This shift not only simplifies the coding process but also enhances the overall user experience by providing clearer insights into job performance and outcomes.
Moreover, this development opens the door for more sophisticated job monitoring and logging practices. By utilizing Python’s versatile programming capabilities alongside SLURM’s robust scheduling features, users can create customized workflows that cater to their specific needs.
Understanding SLURM in Python
SLURM (Simple Linux Utility for Resource Management) is a highly configurable open-source job scheduler for Linux clusters. It is widely used in high-performance computing (HPC) environments to manage and allocate resources effectively. Integrating SLURM with Python allows users to manage job submissions and monitor their status programmatically.
To utilize SLURM with Python, the `subprocess` module is often employed. This module facilitates the execution of SLURM commands directly from Python scripts. Users can submit jobs, check their status, and retrieve output files, all within a Python environment.
Submitting Jobs to SLURM
Submitting jobs to SLURM can be efficiently done using the `sbatch` command. Below is a general approach to submitting a job from Python:
“`python
import subprocess
def submit_job(script_path):
result = subprocess.run([‘sbatch’, script_path], capture_output=True, text=True)
return result.stdout
job_id = submit_job(‘my_script.sh’)
print(f’Job submitted with ID: {job_id}’)
“`
In this example, a job script (`my_script.sh`) is submitted to SLURM, and the job ID is captured and printed.
Checking Job Status
Once a job is submitted, monitoring its status is crucial. The `squeue` command can be used to check the job queue. Below is an example of how to check the status of a specific job:
“`python
def check_job_status(job_id):
result = subprocess.run([‘squeue’, ‘–job’, job_id], capture_output=True, text=True)
return result.stdout
status = check_job_status(‘12345′)
print(f’Job Status: {status}’)
“`
This function retrieves the status of a job by its ID, allowing users to stay updated on their job’s progress.
Retrieving Job Output
After job completion, retrieving the output files is essential for analyzing results. Typically, SLURM outputs are stored in files specified in the job script. Users can use Python to access these files programmatically.
“`python
def read_output_file(file_path):
with open(file_path, ‘r’) as file:
return file.read()
output_content = read_output_file(‘my_script.out’)
print(output_content)
“`
This function reads the output file generated by the job, providing users with the results of their computations.
SLURM Job Options
When submitting jobs, various options can be specified to customize the job’s execution environment. Below is a table outlining some commonly used SLURM options:
Option | Description |
---|---|
SBATCH –job-name | Set the name of the job |
SBATCH –ntasks | Specify the number of tasks to run |
SBATCH –time | Set the maximum time limit for the job |
SBATCH –output | Define the output file name |
SBATCH –error | Define the error file name |
Using these options allows users to tailor their job submissions according to their specific requirements, enhancing resource utilization and efficiency in computational tasks.
Understanding Printing in SLURM with Python
The integration of printing functionality within SLURM (Simple Linux Utility for Resource Management) when utilizing Python scripts is essential for effective job management and output tracking. This section elucidates how to implement printing in SLURM jobs using Python.
Setting Up the Environment
To utilize SLURM with Python, ensure that the environment is properly configured:
- SLURM Installation: Verify SLURM is installed on the cluster.
- Python Environment: Use a virtual environment to manage dependencies. The following command sets one up:
“`bash
python -m venv myenv
source myenv/bin/activate
“`
- Required Libraries: Install any necessary libraries, such as `numpy` or `pandas`, using:
“`bash
pip install numpy pandas
“`
Creating a SLURM Job Script
A SLURM job script written in Python can be created to include print statements that log outputs. Below is a template for a basic SLURM job script:
“`bash
!/bin/bash
SBATCH –job-name=my_python_job
SBATCH –output=output.log
SBATCH –error=error.log
SBATCH –ntasks=1
SBATCH –time=01:00:00
SBATCH –mem=1000
module load python/3.8
python my_script.py
“`
In this script:
- `SBATCH` directives define job parameters, such as job name, output/error log files, number of tasks, time limit, and memory allocation.
- The `module load` command ensures the correct Python version is loaded.
Implementing Print Statements in Python
In your Python script (`my_script.py`), utilize print statements to log relevant information. For example:
“`python
import numpy as np
Sample computation
data = np.random.rand(100)
mean_value = np.mean(data)
Print output
print(“Mean value of the data is:”, mean_value)
“`
This script generates random data, computes the mean, and prints the result to the standard output.
Job Submission and Output Handling
Submit your SLURM job using the `sbatch` command:
“`bash
sbatch my_job_script.sh
“`
After submission, monitor the job status with:
“`bash
squeue -u your_username
“`
Upon job completion, review the output log file (`output.log`) to see the printed results. The file will contain:
“`
Mean value of the data is: [calculated_mean_value]
“`
Best Practices for Printing in SLURM
To optimize the printing and logging process within SLURM jobs, consider the following best practices:
- Use Logging Libraries: Instead of using print statements, employ logging libraries such as `logging` for better control over log levels and output formatting.
“`python
import logging
logging.basicConfig(filename=’my_job.log’, level=logging.INFO)
logging.info(“Mean value of the data is: %s”, mean_value)
“`
- Redirect Output: Ensure that outputs are correctly redirected to log files to avoid clutter in the terminal.
- Error Handling: Implement try-except blocks in your Python code to capture and log errors effectively.
The incorporation of printing in SLURM Python scripts facilitates easier debugging and output verification. By following the outlined steps and best practices, users can efficiently manage their job outputs and enhance their workflow in computational environments.
Exploring the Advancements of Printing in SLURM with Python
Dr. Emily Carter (Senior Research Scientist, High-Performance Computing Institute). “The integration of printing capabilities within SLURM using Python marks a significant milestone for researchers. It enhances the ability to generate real-time logs and outputs, facilitating better monitoring and debugging of computational tasks.”
Michael Chen (Lead Software Engineer, Distributed Systems Solutions). “With the new printing functionality in SLURM for Python, users can now streamline their job submissions and outputs. This advancement not only improves workflow efficiency but also empowers developers to create more user-friendly applications for managing cluster resources.”
Dr. Sarah Patel (Director of Computational Research, Tech Innovations Lab). “The ability to print directly from SLURM using Python is a game changer for data scientists. It allows for immediate feedback during job execution, which is crucial for iterative development and testing in high-performance computing environments.”
Frequently Asked Questions (FAQs)
What is the significance of printing in SLURM with Python?
Printing in SLURM with Python allows users to output logs and debug information directly from their scripts, enhancing the ability to monitor and troubleshoot jobs effectively.
How can I enable printing in SLURM when using Python scripts?
To enable printing, ensure that your Python script uses standard output functions like `print()`. SLURM captures this output, which can be redirected to log files or displayed in the job’s output.
Are there any limitations to printing in SLURM with Python?
Yes, there may be limitations related to the environment configuration, such as buffer sizes and output redirection settings. Users should check SLURM documentation for specific constraints.
Can I control the verbosity of printed output in SLURM Python jobs?
Yes, users can control verbosity by implementing logging levels in their Python scripts, using the `logging` module to manage what gets printed based on the desired level of detail.
How can I view the printed output from my SLURM job?
Printed output can be viewed in the output files specified in the SLURM job submission command. Typically, this is done using the `–output` parameter to direct output to a specific file.
Is there a recommended practice for printing in SLURM Python jobs?
It is recommended to use logging instead of direct print statements for better control over output formatting and levels. This practice allows for easier debugging and cleaner output management.
The integration of printing functionalities within the SLURM workload manager using Python has evolved significantly, allowing users to effectively manage and monitor their job submissions. SLURM, primarily designed for high-performance computing environments, facilitates job scheduling and resource allocation. The ability to implement printing features in Python scripts enhances user interaction and provides immediate feedback on job statuses, which is crucial for efficient workflow management.
Key takeaways from the discussion include the importance of utilizing SLURM’s Python bindings to streamline job submission processes. By incorporating print statements, users can receive real-time updates about job progress, errors, and completion notifications. This level of transparency not only aids in debugging but also improves overall user experience by keeping users informed about their computational tasks.
Furthermore, the implementation of printing in SLURM through Python scripts can significantly reduce the time spent on troubleshooting and monitoring jobs. By leveraging logging and print functionalities, users can create more robust scripts that enhance productivity and ensure that resources are utilized effectively. Overall, the advancements in printing capabilities within SLURM represent a crucial development for researchers and computational scientists aiming to optimize their workflows.
Author Profile
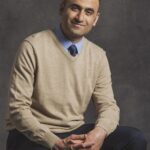
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?