How Can You Use the Proper Case Function in ANSI SQL?
In the world of SQL, where data manipulation and retrieval reign supreme, the presentation of that data can be just as crucial as the data itself. One of the most common formatting requirements is transforming text into proper case—a style where the first letter of each word is capitalized, while the rest remain in lowercase. Whether you’re preparing reports, cleaning up user input, or ensuring consistency across your database, mastering the proper case function in ANSI SQL can elevate your data presentation and enhance readability.
While ANSI SQL does not provide a built-in function specifically for converting text to proper case, there are various approaches and techniques that can achieve this effect. Understanding how to manipulate string functions and leverage existing SQL capabilities will empower you to format your text effectively. By combining functions like `UPPER()`, `LOWER()`, and `SUBSTRING()`, you can create a custom solution that meets your specific needs.
In this article, we will explore the intricacies of implementing a proper case function in ANSI SQL. We will delve into the challenges posed by different database systems and the creative workarounds that can be employed. Whether you’re a seasoned SQL developer or a newcomer eager to enhance your skills, this guide will provide you with the insights needed to transform your text data into a polished, professional format
Understanding the Proper Case Function
In ANSI SQL, there is no built-in function explicitly designed to convert strings to proper case (where the first letter of each word is capitalized). However, this can be achieved through a combination of string manipulation functions. The approach typically involves using functions like `UPPER()`, `LOWER()`, and `SUBSTRING()`, along with custom logic to handle each word in the string.
To illustrate the concept, let’s define proper case as capitalizing the first letter of each word while ensuring that the rest of the letters are in lowercase. The following method can be employed:
- Split the string into words: This often requires a function or a recursive Common Table Expression (CTE) since ANSI SQL does not have direct support for splitting strings.
- Capitalize the first letter of each word: Use the `UPPER()` function on the first letter of each word.
- Lowercase the remaining letters: Use the `LOWER()` function for the rest of the letters in each word.
- Reassemble the words into a single string.
Here’s an example SQL query demonstrating how you might implement proper case logic using a recursive CTE:
“`sql
WITH RECURSIVE ProperCaseCTE AS (
SELECT
SUBSTRING(column_name, 1, CHARINDEX(‘ ‘, column_name + ‘ ‘) – 1) AS word,
SUBSTRING(column_name, CHARINDEX(‘ ‘, column_name + ‘ ‘) + 1, LEN(column_name)) AS rest
FROM your_table
WHERE column_name IS NOT NULL
UNION ALL
SELECT
SUBSTRING(rest, 1, CHARINDEX(‘ ‘, rest + ‘ ‘) – 1),
SUBSTRING(rest, CHARINDEX(‘ ‘, rest + ‘ ‘) + 1, LEN(rest))
FROM ProperCaseCTE
WHERE rest <> ”
)
SELECT
STRING_AGG(UPPER(SUBSTRING(word, 1, 1)) + LOWER(SUBSTRING(word, 2, LEN(word))), ‘ ‘) AS ProperCasedString
FROM ProperCaseCTE;
“`
This query defines a recursive CTE that splits the input string into words, processes each word to capitalize the first letter, and then reassembles them.
Alternative Approaches
If using a specific database system, there may be built-in functions or extensions that simplify this process. Some alternatives include:
- PostgreSQL: Can use `initcap()` to achieve proper case directly.
- SQL Server: Requires a custom function or script, as shown above.
- Oracle: Provides the `INITCAP()` function.
Sample Table of Database Functions
Database System | Function for Proper Case |
---|---|
PostgreSQL | initcap() |
MySQL | No built-in function; use custom logic |
SQL Server | No built-in function; requires custom implementation |
Oracle | INITCAP() |
In summary, while ANSI SQL lacks a direct proper case function, creative use of existing string functions allows for achieving the desired formatting across various database systems.
Understanding Proper Case in ANSI SQL
Proper case refers to the formatting of text where the first letter of each word is capitalized, and all other letters are in lowercase. ANSI SQL does not provide a built-in function specifically for converting strings to proper case. However, there are ways to achieve this using a combination of string functions.
Creating a Proper Case Function
To emulate a proper case function in ANSI SQL, you can create a user-defined function (UDF) that processes each word in a string. Below is a basic example of how to implement this in SQL Server:
“`sql
CREATE FUNCTION dbo.ProperCase(@inputString VARCHAR(255))
RETURNS VARCHAR(255)
AS
BEGIN
DECLARE @resultString VARCHAR(255);
SET @inputString = LOWER(@inputString); — Convert to lowercase
SET @resultString = ”;
DECLARE @i INT = 1;
WHILE @i <= LEN(@inputString)
BEGIN
DECLARE @currentChar CHAR(1) = SUBSTRING(@inputString, @i, 1);
IF @i = 1 OR SUBSTRING(@inputString, @i - 1, 1) = ' '
SET @resultString = @resultString + UPPER(@currentChar); -- Capitalize first letter of each word
ELSE
SET @resultString = @resultString + @currentChar; -- Append the rest as is
SET @i = @i + 1;
END
RETURN @resultString;
END;
```
Alternative Methods for Proper Case
If creating a function is not an option, you can use a series of SQL string functions to format text in proper case. This method can be cumbersome and may require additional handling for punctuation and special characters.
- Using `UPPER()` and `LOWER()`:
- First, convert the entire string to lowercase.
- Then, capitalize the first letter of each word by leveraging space delimiters.
Example using basic SQL operations:
“`sql
SELECT
UPPER(SUBSTRING(column_name, 1, 1)) + LOWER(SUBSTRING(column_name, 2, LEN(column_name)))
FROM
your_table;
“`
However, this approach would need to be repeated for each word within a string.
Considerations and Limitations
When implementing a proper case function or logic, consider the following:
- Handling Special Characters: Ensure that special characters and punctuation do not disrupt the proper case format.
- Performance: User-defined functions may incur performance overhead, especially on large datasets.
- Database Compatibility: The implementation may vary depending on the SQL dialect you are using (e.g., SQL Server, MySQL, PostgreSQL).
Example Usage
Suppose you have a table named `employees` with a column `full_name`. You can retrieve names in proper case using the created function:
“`sql
SELECT dbo.ProperCase(full_name) AS ProperFullName
FROM employees;
“`
This query will return each employee’s name formatted in proper case.
Implementing proper case functionality in ANSI SQL requires creativity and a good understanding of string manipulation. While there is no direct function available, user-defined functions and careful use of existing string functions can achieve the desired results effectively.
Understanding the Proper Case Function in ANSI SQL
Dr. Emily Carter (Database Systems Analyst, SQL Innovations). The proper case function in ANSI SQL is not natively supported as a single built-in function. However, it can be achieved through a combination of string manipulation functions such as UPPER, LOWER, and SUBSTRING. This approach allows developers to format strings to a proper case by capitalizing the first letter of each word while ensuring the rest remain in lowercase.
Michael Tran (Senior SQL Developer, Data Solutions Corp). Implementing a proper case function in ANSI SQL often requires custom user-defined functions (UDFs). This is particularly useful in scenarios where consistent data formatting is critical for reporting and analysis. By creating a UDF, developers can encapsulate the logic for transforming text into proper case, making it reusable across different queries and applications.
Jessica Liu (Data Integrity Specialist, Analytics Group). It’s essential to consider the implications of using a proper case function in ANSI SQL, especially in terms of performance. While formatting text can improve readability, excessive use of string functions in large datasets may lead to slower query performance. Therefore, it is advisable to apply such transformations judiciously, ideally during data entry or preprocessing stages.
Frequently Asked Questions (FAQs)
What is the proper case function in ANSI SQL?
The proper case function in ANSI SQL does not exist as a built-in function. However, it can be simulated using string manipulation functions available in SQL.
How can I convert a string to proper case in ANSI SQL?
To convert a string to proper case, you can use a combination of string functions such as `UPPER()`, `LOWER()`, and `SUBSTRING()`, along with a user-defined function or a recursive common table expression (CTE) to handle each word in the string.
Are there any database systems that provide a proper case function?
Yes, some database systems like PostgreSQL and SQL Server offer specific functions like `INITCAP()` and `PROPER()` respectively, which can convert strings to proper case directly.
Can I create a custom proper case function in ANSI SQL?
Yes, you can create a custom function using procedural SQL to iterate through each character of the string, capitalizing the first letter of each word while converting the rest to lowercase.
What challenges might I face when implementing a proper case function?
Challenges include handling special characters, punctuation, and ensuring that acronyms or specific words retain their intended casing. Additionally, performance may be affected with large datasets.
Is there a performance impact when using string manipulation for proper case conversion?
Yes, using string manipulation for proper case conversion can impact performance, especially with large datasets, as it requires additional processing for each string transformation.
The proper case function in ANSI SQL refers to the ability to format strings such that the first letter of each word is capitalized while the remaining letters are in lowercase. This functionality is not natively supported in ANSI SQL, as there is no built-in function specifically designated for converting text to proper case. However, various database management systems (DBMS) provide their own methods or functions to achieve this effect, often through a combination of string manipulation functions.
For instance, in SQL Server, one can utilize a user-defined function to iterate through each character of a string and capitalize the first letter of each word. Similarly, PostgreSQL offers the `initcap` function, which directly converts a string to proper case. In contrast, MySQL does not have a built-in proper case function, but users can create a custom function to replicate this behavior. Understanding these variations is crucial for developers working across different SQL environments.
Key takeaways from the discussion include the recognition that while ANSI SQL lacks a standardized proper case function, many DBMSs offer alternative solutions. Users must familiarize themselves with the specific functions available in their respective SQL environments to effectively implement proper case formatting. Additionally, the implementation of user-defined functions can provide a tailored approach to meet specific
Author Profile
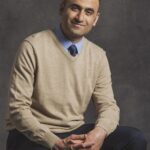
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?