Why Am I Getting ‘Property [id] Does Not Exist on the Eloquent Builder Instance’ Error?
In the world of web development, particularly when working with the Laravel framework, encountering errors can be a frustrating yet illuminating experience. One such error that developers often face is the message: “property [id] does not exist on the eloquent builder instance.” This seemingly cryptic notification can halt progress and leave even seasoned programmers scratching their heads. However, understanding the nuances behind this error is essential for anyone looking to harness the full power of Eloquent ORM in Laravel.
This article delves into the intricacies of Eloquent builders and the common pitfalls that lead to this error. By exploring the underlying reasons why Laravel might throw this message, we can illuminate best practices for querying data and manipulating model instances. Whether you’re a novice developer or a seasoned pro, grasping these concepts will enhance your coding skills and ensure smoother development processes.
As we navigate through the various scenarios that can trigger this error, we will also highlight effective troubleshooting strategies and coding techniques that can help you avoid similar issues in the future. By the end of this article, you’ll have a clearer understanding of Eloquent’s behavior and how to work with it more effectively, empowering you to build robust applications with confidence.
Understanding the Error Message
The error message “property [id] does not exist on the eloquent builder instance” typically occurs in Laravel when attempting to access a property or method that is not available on an Eloquent builder instance. This often indicates that the code is trying to retrieve a property from a query builder rather than an actual model instance.
In Eloquent, the builder is returned when using methods that do not immediately retrieve results, such as `where()`, `orderBy()`, or `get()`. It is crucial to understand the distinction between a builder instance and a model instance, as they serve different purposes.
Common Causes of the Error
Several scenarios can lead to this error. Recognizing these can help prevent it in future code:
– **Accessing Properties Prematurely**: Attempting to access properties like `$model->id` on the builder instead of after retrieving the actual model.
- Incorrect Method Chaining: Misusing method chains that do not return a model instance.
- Expecting Single Results: Using methods like `first()` or `find()` improperly, which return single instances, while still trying to access properties on the builder.
Examples of Incorrect Usage
Here are a few code snippets that illustrate common mistakes leading to the error:
“`php
// Incorrect: Accessing property on the builder
$user = User::where(’email’, ‘[email protected]’);
echo $user->id; // This will throw the error
“`
“`php
// Incorrect: Method chaining without retrieving the model
$user = User::where(‘active’, true)->get();
echo $user->id; // This will throw the error because $user is a collection
“`
Correcting the Error
To resolve this error, you must ensure that you are working with an actual model instance. Here are some methods to correct your code:
– **Retrieve the Model Instance**: Always call a method that returns a model instance before accessing its properties.
“`php
// Correct: Retrieve the model instance
$user = User::where(’email’, ‘[email protected]’)->first();
echo $user->id; // This will work correctly
“`
– **Accessing First Item from Collection**: If you are working with a collection, use the `first()` method to get the first instance.
“`php
// Correct: Access first item from a collection
$user = User::where(‘active’, true)->get()->first();
echo $user->id; // This will work correctly
“`
Best Practices
To minimize the occurrence of this error, follow these best practices:
- Use Eloquent Methods Appropriately: Familiarize yourself with Eloquent methods and their return types.
- Check Instance Types: Use `instanceof` to verify the type of returned objects when uncertain.
- Debugging: Use debugging tools or `dd()` to inspect the returned value when encountering issues.
Method | Returns | Usage Example |
---|---|---|
all() | Collection | User::all() |
first() | Model Instance or null | User::where(’email’, ‘[email protected]’)->first() |
get() | Collection | User::where(‘active’, true)->get() |
find() | Model Instance or null | User::find(1) |
By adhering to these guidelines and understanding how Eloquent builders and models interact, you can effectively avoid the “property [id] does not exist on the eloquent builder instance” error and write more efficient Laravel code.
Understanding the Eloquent Builder Instance
The Eloquent ORM in Laravel provides a fluent interface for working with database records. The Eloquent Builder instance is a core component that allows developers to construct database queries using an expressive syntax. However, certain errors can arise when interacting with this instance, notably the message: `property [id] does not exist on the eloquent builder instance`.
Common Causes of the Error
This error typically occurs due to a misunderstanding of how Eloquent models and the Builder instance work. Below are common scenarios that lead to this error:
- Incorrect Access: Attempting to access a property directly on the Builder instance instead of on a model instance.
- Misusing Query Methods: Using a method that returns a Builder instance, such as `where()`, and trying to access the result as if it were a single model.
- Expecting a Single Result: Assuming a method like `all()` or `get()` returns a single model instead of a collection.
Proper Usage of Eloquent Queries
To avoid this error, it’s crucial to understand the difference between a Builder instance and a model instance. Here are examples of correct usage:
“`php
// Correct Usage
$user = User::find(1); // This returns a User model instance
echo $user->id; // Accessing the id property correctly
// Incorrect Usage
$userBuilder = User::where(‘active’, true); // This returns a Builder instance
echo $userBuilder->id; // This will throw the error
“`
Methods and Their Returns
Familiarizing yourself with the return types of various Eloquent methods can prevent this error. The following table summarizes common methods and their expected return types:
Method | Returns |
---|---|
`all()` | Collection of model instances |
`find($id)` | Single model instance |
`first()` | Single model instance or null |
`get()` | Collection of model instances |
`where(…)` | Builder instance |
`firstOrFail()` | Single model instance or throws |
Resolving the Error
If you encounter the `property [id] does not exist on the eloquent builder instance` error, consider the following steps to resolve it:
- Check Method Returns: Ensure you are calling a method that returns a model instance when trying to access properties.
- Use `first()` or `find()`: If you’re expecting a single result, use methods like `first()` or `find()` to retrieve a model instance.
- Accessing Collections: If a method returns a collection, iterate through the results to access individual model instances.
Example Fix
Here’s an example of how to modify code that may generate this error:
“`php
// Before: Incorrectly trying to access id on the Builder instance
$activeUsers = User::where(‘active’, true);
foreach ($activeUsers as $user) {
echo $user->id; // Error: property [id] does not exist
}
// After: Correctly fetching the collection and accessing model properties
$activeUsers = User::where(‘active’, true)->get();
foreach ($activeUsers as $user) {
echo $user->id; // Accessing the id property correctly
}
“`
By adhering to these guidelines and understanding the underlying mechanics of Eloquent, developers can effectively avoid common pitfalls related to the Builder instance.
Understanding Eloquent Builder Errors in Laravel
Dr. Emily Carter (Senior Software Engineer, Laravel Insights). “The error ‘property [id] does not exist on the eloquent builder instance’ typically arises when attempting to access a property on an Eloquent query builder instead of an Eloquent model. Developers must ensure they are working with the correct object type, as the builder does not have direct access to model properties.”
Michael Chen (Lead Developer, CodeReview Weekly). “This error often indicates a misunderstanding of the Eloquent ORM’s chainable methods. It’s crucial to remember that methods like ‘get()’ or ‘first()’ must be called to retrieve the actual model instance before accessing its properties.”
Sarah Thompson (Technical Writer, PHP Development Journal). “To resolve the ‘property [id] does not exist’ error, developers should verify their queries. Utilizing ‘find()’ or ‘findOrFail()’ can help directly retrieve a model by its ID, thus avoiding this common pitfall.”
Frequently Asked Questions (FAQs)
What does the error “property [id] does not exist on the eloquent builder instance” mean?
This error indicates that you are attempting to access a property on an Eloquent builder instance that does not exist. Typically, this occurs when you try to access a property directly on the query builder rather than on a model instance.
How can I resolve the “property [id] does not exist on the eloquent builder instance” error?
To resolve this error, ensure that you are calling the `first()`, `get()`, or `find()` method on the Eloquent query builder to retrieve a model instance before accessing its properties.
What is the difference between Eloquent builder and Eloquent model in Laravel?
The Eloquent builder is used to construct database queries, while the Eloquent model represents a single record in the database. Accessing properties directly on the builder will lead to errors since it does not represent a specific record.
Can I access properties of a model after using the `get()` method?
Yes, after using the `get()` method, you will receive a collection of model instances. You can then iterate over this collection to access properties of each individual model.
What should I check if I encounter this error in a Laravel application?
Check if you are mistakenly trying to access a property on the query builder instead of a retrieved model instance. Review your code to ensure you are using the appropriate methods to fetch data from the database.
Is this error specific to Laravel, or can it occur in other frameworks?
While the error message is specific to Laravel’s Eloquent ORM, similar issues can occur in other frameworks that utilize an object-relational mapping (ORM) system when trying to access properties on a query builder instead of a model instance.
The error message “property [id] does not exist on the eloquent builder instance” typically arises in Laravel when attempting to access a property that is not available on the Eloquent query builder object. This situation often occurs when developers mistakenly try to retrieve a property directly from the query builder instead of from the model instance that the query returns. Understanding the distinction between the query builder and the model is crucial for effective Eloquent usage.
To resolve this issue, developers should ensure that they are calling the appropriate methods to retrieve model instances. For example, using methods like `first()`, `get()`, or `find()` will return actual model instances rather than the query builder itself. By doing so, developers can access the properties of the model, including the `id` property, without encountering this error.
Additionally, it is essential to familiarize oneself with the Eloquent ORM’s structure and behavior. Properly managing the flow of data between the database and the application can prevent similar errors. Leveraging Laravel’s extensive documentation and community resources can further enhance understanding and troubleshooting capabilities when working with Eloquent.
Author Profile
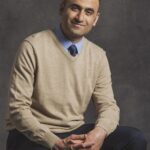
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?