How Can You Place the Legend Outside the Plot in Matplotlib?
When it comes to creating visually appealing and informative plots in Python using Matplotlib, the placement of the legend can significantly impact the clarity and aesthetics of your data visualization. While legends are essential for interpreting the various elements of a graph, their default positioning can sometimes obscure important data points or clutter the overall design. If you’ve ever found yourself wishing to enhance your plot by moving the legend outside the main plotting area, you’re not alone. This article delves into the techniques and best practices for positioning legends outside the plot area in Matplotlib, ensuring that your visualizations are both clear and compelling.
Understanding how to manipulate the legend’s placement is crucial for effective data presentation. By moving the legend outside the plot, you can create more space for your data, allowing viewers to focus on the trends and patterns without distraction. This adjustment not only improves readability but also enhances the overall aesthetic of your plot. In this discussion, we will explore various methods to achieve this, from simple adjustments to more advanced configurations, catering to users of all experience levels.
Whether you’re a data scientist looking to present your findings or a student aiming to impress with your visual storytelling, mastering the art of legend placement can elevate your work. As we proceed, you will discover practical tips and code snippets that will empower you to customize
Positioning the Legend
In Matplotlib, legends can be positioned outside of the plot area to enhance clarity and visual appeal. This is particularly useful when multiple plots or data series are presented, and the legend might obstruct important data points. The positioning can be adjusted using the `bbox_to_anchor` parameter along with the `loc` parameter within the `legend()` method.
The `loc` parameter specifies which part of the legend box to position, while `bbox_to_anchor` determines the exact coordinates of the legend’s anchor point. The coordinates are normalized between 0 and 1, where (0,0) is the bottom-left corner of the plot and (1,1) is the top-right.
Here are some common configurations for placing the legend outside the plot area:
- Top Right Outside: `loc=’upper left’, bbox_to_anchor=(1, 1)`
- Bottom Right Outside: `loc=’lower left’, bbox_to_anchor=(1, 0)`
- Center Left Outside: `loc=’center left’, bbox_to_anchor=(1, 0.5)`
Example Code for Legend Positioning
Here’s an example demonstrating how to place a legend outside the plot area:
“`python
import matplotlib.pyplot as plt
Sample Data
x = [1, 2, 3, 4]
y1 = [1, 4, 9, 16]
y2 = [2, 3, 5, 7]
Creating plots
plt.plot(x, y1, label=’Quadratic’)
plt.plot(x, y2, label=’Linear’)
Adding the legend outside the plot
plt.legend(loc=’upper left’, bbox_to_anchor=(1, 1))
Display the plot
plt.show()
“`
This code creates a simple line plot with two datasets and places the legend in the upper left corner outside the plot area.
Customizing Legend Appearance
Further customization of the legend can enhance its presentation. Common customizations include:
- Font size and style: Adjusting the `fontsize` parameter within the `legend()` method.
- Frame visibility: Using `frameon` to toggle the legend border.
- Background color: Specifying the `facecolor` parameter for the legend background.
Here’s a table summarizing key parameters for legend customization:
Parameter | Description |
---|---|
loc | Location of the legend (e.g., ‘upper right’, ‘lower left’) |
bbox_to_anchor | Coordinates to position the legend (normalized from 0 to 1) |
fontsize | Size of the legend text |
frameon | Boolean to toggle the legend frame |
facecolor | Background color of the legend |
By utilizing these parameters, users can effectively manage the appearance and location of legends in their Matplotlib visualizations, ensuring that they remain informative without obstructing the data being presented.
Placing the Legend Outside the Plot
To position the legend outside the plot area in Matplotlib, you can utilize the `bbox_to_anchor` and `loc` parameters of the `legend()` function. This allows for greater flexibility in defining the legend’s position relative to the axes.
Example Code Snippet
The following example demonstrates how to place the legend outside the plot:
“`python
import matplotlib.pyplot as plt
Sample data
x = [1, 2, 3, 4]
y1 = [10, 20, 25, 30]
y2 = [15, 18, 22, 27]
Creating the plot
plt.plot(x, y1, label=’Line 1′, color=’blue’)
plt.plot(x, y2, label=’Line 2′, color=’orange’)
Customizing the legend
plt.legend(loc=’upper left’, bbox_to_anchor=(1, 1))
Displaying the plot
plt.show()
“`
Explanation of Parameters
- `loc`: Specifies the anchor point of the legend. Common options include:
- `’upper left’`
- `’upper right’`
- `’lower left’`
- `’lower right’`
- `’center left’`
- `’center right’`
- `’upper center’`
- `’lower center’`
- `’center’`
- `bbox_to_anchor`: Defines the position of the legend box. This is a tuple that specifies the x and y coordinates in relative terms:
- The first value is the horizontal position (0 to 1 for left to right).
- The second value is the vertical position (0 to 1 for bottom to top).
Example Scenarios
Here are a few different configurations for placing the legend outside the plot:
Legend Position | `loc` | `bbox_to_anchor` |
---|---|---|
Top Right | `’upper right’` | `(1, 1)` |
Bottom Right | `’lower right’` | `(1, 0)` |
Center Left | `’center left’` | `(0, 0.5)` |
Custom Position | `’upper center’` | `(0.5, 1.1)` |
Additional Customizations
- Adjusting the Legend Box: You can modify the legend box’s appearance using parameters like `frameon`, `shadow`, and `fontsize` to enhance visibility.
- Multiple Legends: If needed, you can create multiple legends by calling `legend()` multiple times with different handles and labels.
Tips for Effective Visualization
- Ensure the legend does not overlap with important data points.
- Use contrasting colors for legend text and background for clarity.
- Consider the overall layout of the plot to maintain an organized appearance.
Expert Insights on Positioning Legends in Matplotlib Plots
Dr. Emily Carter (Data Visualization Specialist, Visual Insights Inc.). “Positioning the legend outside of a plot in Matplotlib can greatly enhance the clarity of the data presented. By doing so, you allow the viewer to focus on the data itself without the distraction of overlapping elements, which is particularly beneficial in complex visualizations.”
Michael Chen (Senior Software Engineer, Data Science Solutions). “Utilizing the `bbox_to_anchor` parameter in Matplotlib is an effective way to place legends outside the plot area. This method provides flexibility in positioning and can be adjusted to fit various layouts, ensuring that the legend complements rather than obstructs the data.”
Sarah Thompson (Academic Researcher, Department of Statistics). “Incorporating legends outside the plot not only improves aesthetics but also enhances interpretability. It is essential to maintain a balance between the plot’s visual appeal and its functional clarity, especially when presenting to diverse audiences.”
Frequently Asked Questions (FAQs)
How can I position the legend outside the plot in Matplotlib?
To position the legend outside the plot, use the `bbox_to_anchor` parameter in the `legend()` function. For example, `plt.legend(loc=’upper left’, bbox_to_anchor=(1, 1))` places the legend outside to the right of the plot.
What does the `loc` parameter in the legend function do?
The `loc` parameter specifies the location of the legend. It accepts string values like ‘upper left’, ‘upper right’, ‘lower left’, etc., which determine the anchor point of the legend box.
Can I customize the size of the legend when placing it outside the plot?
Yes, you can customize the size of the legend by adjusting the `fontsize` parameter in the `legend()` function. Additionally, you can modify the `frameon` parameter to control the legend’s border appearance.
Is it possible to place the legend in a specific position using coordinates?
Yes, you can use `bbox_to_anchor` with specific coordinates to place the legend at an exact position. For example, `bbox_to_anchor=(x, y)` allows you to define the legend’s position in relation to the axes.
What should I do if the legend overlaps with the plot elements?
If the legend overlaps with plot elements, consider adjusting the `bbox_to_anchor` coordinates or changing the `loc` parameter to reposition the legend. Alternatively, you can increase the figure size using `plt.figure(figsize=(width, height))`.
Are there any limitations to placing the legend outside the plot?
While placing the legend outside the plot is flexible, ensure that the legend does not exceed the figure boundaries. If it does, adjust the figure size or reposition the legend accordingly to maintain visibility.
In summary, placing the legend outside of a plot in Matplotlib can significantly enhance the clarity and aesthetics of visualizations. By default, legends are positioned within the axes, which can sometimes obscure important data points or trends. Adjusting the legend’s position to the outside of the plot area allows for a cleaner presentation, enabling viewers to focus on the data without distraction.
To achieve this, users can utilize the `bbox_to_anchor` parameter in the `legend()` function. This parameter allows for precise control over the legend’s location, enabling it to be placed at various positions outside the plot, such as to the right, left, or even above the axes. Additionally, adjusting the `loc` parameter helps in aligning the legend correctly relative to the specified anchor point.
It is essential to consider the overall layout of the plot when placing the legend externally. Proper spacing and alignment can prevent the legend from overlapping with other elements of the figure. Moreover, using the `tight_layout()` function can help in managing the layout dynamically, ensuring that all components of the plot are well-distributed and visually appealing.
positioning the legend outside the plot in Matplotlib is a straightforward yet effective technique that enhances the interpretability
Author Profile
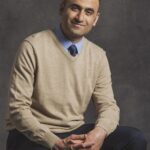
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?