How Can You Change the Order of Widgets in PyQt6?
In the world of GUI development, PyQt6 stands out as a powerful toolkit for creating stunning and responsive applications. One of the most crucial aspects of designing an intuitive user interface is the arrangement of widgets. The order in which these elements are displayed can significantly impact user experience, making it essential for developers to have a solid grasp of how to manipulate widget order effectively. Whether you’re building a simple form or a complex application, understanding how to change the order of widgets in PyQt6 can elevate your design and functionality to new heights.
When working with PyQt6, developers often encounter scenarios where the visual hierarchy of widgets needs adjustment. This could be to enhance usability, improve aesthetics, or respond to dynamic user interactions. Fortunately, PyQt6 offers a variety of methods for rearranging widgets, allowing for flexibility in layout management. From utilizing layout managers to programmatically altering the widget order, the toolkit provides the necessary tools to create a seamless user experience.
Moreover, mastering the techniques for changing widget order not only helps in achieving the desired layout but also fosters a deeper understanding of PyQt6’s architecture. As you delve into the intricacies of widget management, you’ll discover best practices that can streamline your development process and lead to more maintainable code. Join us as we
Changing the Order of Widgets in PyQt6
To change the order of widgets in a PyQt6 application, you typically manipulate the layout that contains the widgets. The layout manager is responsible for arranging child widgets, and you can adjust their order through various methods depending on the layout type you are using.
Using QVBoxLayout and QHBoxLayout
When using vertical (`QVBoxLayout`) or horizontal (`QHBoxLayout`) layouts, you can easily change the order of widgets by removing and re-adding them in the desired sequence. Below is a step-by-step process on how to do this.
- Remove the widget from the layout.
- Insert the widget back at the desired position.
For example:
“`python
from PyQt6.QtWidgets import QApplication, QWidget, QVBoxLayout, QPushButton
app = QApplication([])
window = QWidget()
layout = QVBoxLayout(window)
Create buttons
button1 = QPushButton(‘Button 1’)
button2 = QPushButton(‘Button 2’)
button3 = QPushButton(‘Button 3’)
Add buttons to layout
layout.addWidget(button1)
layout.addWidget(button2)
layout.addWidget(button3)
Change order: Move button3 to the top
layout.removeWidget(button3)
layout.insertWidget(0, button3)
window.show()
app.exec()
“`
Using QGridLayout
In a `QGridLayout`, changing the order of widgets involves modifying their row and column positions. This can be done by removing the widget from its current position and adding it back at the new coordinates.
Example:
“`python
from PyQt6.QtWidgets import QApplication, QWidget, QGridLayout, QPushButton
app = QApplication([])
window = QWidget()
layout = QGridLayout(window)
Create buttons
button1 = QPushButton(‘Button 1’)
button2 = QPushButton(‘Button 2’)
button3 = QPushButton(‘Button 3’)
Add buttons to layout
layout.addWidget(button1, 0, 0) Row 0, Column 0
layout.addWidget(button2, 0, 1) Row 0, Column 1
layout.addWidget(button3, 1, 0) Row 1, Column 0
Change order: Move button3 to the top right
layout.removeWidget(button3)
layout.addWidget(button3, 0, 1) Move to Row 0, Column 1
window.show()
app.exec()
“`
Dynamic Reordering with Buttons
For a more dynamic approach, you can implement buttons that allow users to change the order of widgets interactively. Here’s how you could set this up:
- Create buttons for reordering.
- Connect the buttons to functions that handle the reordering logic.
Example code snippet:
“`python
def move_up():
Logic to move the selected widget up in the layout
pass
def move_down():
Logic to move the selected widget down in the layout
pass
Add move buttons
move_up_button = QPushButton(‘Move Up’)
move_down_button = QPushButton(‘Move Down’)
move_up_button.clicked.connect(move_up)
move_down_button.clicked.connect(move_down)
layout.addWidget(move_up_button)
layout.addWidget(move_down_button)
“`
Summary of Widget Arrangement Methods
The following table summarizes the methods for changing the order of widgets in different layouts:
Layout Type | Method | Example Function |
---|---|---|
QVBoxLayout | Remove and Insert | layout.removeWidget(widget) |
QHBoxLayout | Remove and Insert | layout.insertWidget(index, widget) |
QGridLayout | Remove and Add at New Position | layout.addWidget(widget, row, column) |
By utilizing these methods, you can effectively manage the order of widgets within your PyQt6 applications, enhancing the user interface and user experience.
Methods to Change the Order of Widgets in PyQt6
In PyQt6, the order of widgets can be modified using various layout managers and methods. The choice of method often depends on the specific requirements of the application and the desired layout behavior.
Using Layout Managers
Layout managers in PyQt6 help in organizing widgets within a container. Common layout managers include `QVBoxLayout`, `QHBoxLayout`, and `QGridLayout`. Each layout manager allows for reordering widgets in distinct ways.
- QVBoxLayout: Stacks widgets vertically.
- QHBoxLayout: Stacks widgets horizontally.
- QGridLayout: Places widgets in a grid format, allowing for more flexible positioning.
To change the order of widgets using a layout manager:
- Remove Widgets: First, remove the widgets from the layout.
- Re-add Widgets: Add the widgets back in the desired order.
Example code snippet for reordering widgets in a `QVBoxLayout`:
“`python
from PyQt6.QtWidgets import QApplication, QWidget, QVBoxLayout, QPushButton
app = QApplication([])
window = QWidget()
layout = QVBoxLayout()
button1 = QPushButton(“Button 1”)
button2 = QPushButton(“Button 2”)
button3 = QPushButton(“Button 3”)
layout.addWidget(button1)
layout.addWidget(button2)
layout.addWidget(button3)
Change the order: Move Button 3 to the top
layout.removeWidget(button3)
layout.insertWidget(0, button3) Insert Button 3 at the top
window.setLayout(layout)
window.show()
app.exec()
“`
Dynamic Widget Reordering
For dynamic situations where the order of widgets may change based on user interactions, signals and slots can be utilized. This allows for a more interactive user experience.
- Signal: Triggered by user actions (e.g., button clicks).
- Slot: A function that executes in response to a signal.
Example of reordering widgets dynamically:
“`python
def move_button_up():
layout.removeWidget(button_to_move)
new_index = layout.indexOf(button_to_move) – 1
layout.insertWidget(new_index, button_to_move)
Connect the signal of a button to the move function
move_button_button.clicked.connect(move_button_up)
“`
Custom Widget Order with `QStackedWidget`
`QStackedWidget` allows for stacking widgets on top of each other while only displaying one at a time. This is useful for applications with multiple views.
- To change the order of widgets, use `insertWidget` or `removeWidget` methods.
- You can programmatically switch between widgets using `setCurrentIndex`.
Example:
“`python
from PyQt6.QtWidgets import QStackedWidget
stacked_widget = QStackedWidget()
Adding widgets
stacked_widget.addWidget(widget1)
stacked_widget.addWidget(widget2)
stacked_widget.addWidget(widget3)
Changing the order
stacked_widget.removeWidget(widget2)
stacked_widget.insertWidget(0, widget2) Move widget2 to the top
Display the second widget
stacked_widget.setCurrentIndex(1)
“`
Using `QBoxLayout` with Spacer Items
In addition to direct widget manipulation, spacer items can help control the spacing and order of widgets. By adding spacer items, you can effectively push widgets into desired positions.
- Use `addStretch()` to create flexible space in `QBoxLayout`.
- This can indirectly influence the order and positioning of widgets.
Example:
“`python
layout.addWidget(button1)
layout.addStretch() Adds flexible space
layout.addWidget(button2)
“`
Conclusion on Widget Ordering
Reordering widgets in PyQt6 can be achieved through various techniques, from using layout managers to dynamic signal-slot interactions. The approach selected should align with the application’s requirements for user interaction and visual design.
Expert Insights on Changing the Order of Widgets in PyQt6
Dr. Emily Chen (Senior Software Engineer, Qt Development Company). “In PyQt6, changing the order of widgets can be achieved efficiently by utilizing layout managers. By manipulating the layout’s item order, developers can dynamically rearrange widgets without needing to recreate them, which enhances both performance and user experience.”
Michael Thompson (UI/UX Designer, Interactive Interfaces Inc.). “When altering the order of widgets in PyQt6, it is crucial to consider the overall user interface flow. A well-structured layout not only improves usability but also ensures that changes in widget order remain intuitive to users, thereby maintaining a seamless interaction.”
Sarah Patel (Software Architect, Tech Innovations Group). “To effectively change the order of widgets in PyQt6, developers should familiarize themselves with the QBoxLayout and QGridLayout classes. These classes provide powerful methods for rearranging widgets programmatically, allowing for responsive designs that adapt to varying screen sizes.”
Frequently Asked Questions (FAQs)
How can I change the order of widgets in a PyQt6 layout?
To change the order of widgets in a PyQt6 layout, you can use the `QBoxLayout`’s `insertWidget()` method or `addWidget()` method after removing the widget from its current position. This allows you to specify the new index for the widget.
Is it possible to reorder widgets dynamically at runtime?
Yes, you can dynamically reorder widgets at runtime by modifying the layout. You can remove the widget from its current position and then reinsert it at the desired index using the layout’s methods.
What layout types support changing the order of widgets in PyQt6?
Layouts such as `QVBoxLayout`, `QHBoxLayout`, and `QGridLayout` support changing the order of widgets. Each layout provides methods to manipulate the position of widgets within them.
Can I use a custom signal to trigger the reordering of widgets?
Yes, you can create a custom signal that, when emitted, triggers a slot function to reorder the widgets in the layout. This allows for a flexible and interactive user experience.
Are there any limitations to changing the order of widgets in PyQt6?
While changing the order of widgets is generally straightforward, limitations may arise if the layout is not updated properly or if the widget’s visibility state affects its position. Always ensure to call `update()` on the layout after making changes.
How do I ensure the layout updates visually after changing widget order?
To ensure the layout updates visually, call the `update()` method on the layout after modifying the widget order. Additionally, you may want to call `adjustSize()` on the parent widget to ensure it resizes correctly to accommodate the new widget arrangement.
In PyQt6, changing the order of widgets within a layout is a common requirement for developers seeking to enhance user interface design. The framework provides several methods to manipulate widget order, primarily through the use of layout managers such as QVBoxLayout, QHBoxLayout, and QGridLayout. By utilizing methods like `addWidget()` and `insertWidget()`, developers can easily rearrange the sequence in which widgets are displayed, allowing for a more intuitive user experience.
Moreover, the flexibility of PyQt6 layouts enables the dynamic adjustment of widget positions at runtime. This capability is particularly useful in applications where user interactions dictate the arrangement of elements. Developers can programmatically remove and re-add widgets or use the `setStretch()` method to control the space allocation among widgets, further refining the layout to meet specific design requirements.
mastering the manipulation of widget order in PyQt6 is essential for creating responsive and user-friendly applications. Understanding the various layout managers and their associated methods provides developers with the tools necessary to customize their interfaces effectively. By leveraging these techniques, one can ensure that applications not only function well but also provide an engaging and organized user experience.
Author Profile
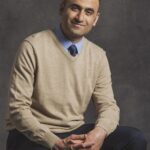
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?