How Can Pyro4 Communicate with a Daemon Using a Name Server?
In the world of distributed computing, seamless communication between components is essential for building robust applications. Pyro4, a powerful Python library, simplifies this process by enabling remote method calls, allowing developers to interact with objects across different machines as if they were local. One of the standout features of Pyro4 is its integration with a name server, which acts as a directory service to facilitate communication between clients and daemons. This article delves into how Pyro4 leverages a name server to streamline interactions, making it an invaluable tool for developers looking to harness the power of remote procedure calls in their projects.
At its core, Pyro4 provides a straightforward way to create and manage remote objects, but the of a name server elevates its capabilities significantly. The name server acts as a broker, maintaining a registry of available remote objects and their corresponding endpoints. This means that clients do not need to know the exact location of the daemon hosting the desired object; they can simply query the name server to retrieve the necessary information. This abstraction not only simplifies the connection process but also enhances the scalability of applications by allowing developers to easily add or remove services without disrupting the overall system.
Furthermore, the combination of Pyro4 and a name server fosters a more dynamic and flexible
Understanding Pyro4 and Name Servers
Pyro4 (Python Remote Objects) is a powerful library that enables remote procedure calls in Python, allowing objects to communicate over the network transparently. A name server in Pyro4 acts as a directory service that provides a means to locate remote objects registered within the network. By using a name server, clients can easily find and communicate with daemons (servers) hosting these objects without needing to know their network addresses.
Setting Up the Pyro4 Name Server
To utilize a name server in Pyro4, follow these steps:
- Install Pyro4: Ensure that Pyro4 is installed in your Python environment. This can typically be done via pip:
“`bash
pip install Pyro4
“`
- Start the Name Server: Launch the Pyro4 name server from the command line. This can be done using:
“`bash
python -m Pyro4.naming
“`
This command starts the name server, which will listen for incoming requests.
- Registering Objects: A daemon must register its objects with the name server. This is done using the `Pyro4.Daemon` class and the `register` method.
Communicating with the Daemon
Once the name server is running and the daemon has registered its objects, clients can communicate with the daemon by resolving the object’s name.
- Client Code Example:
“`python
import Pyro4
Locate the name server
uri = “PYRONAME:my.remote.object”
remote_object = Pyro4.Proxy(uri)
Call a method on the remote object
result = remote_object.some_method()
print(result)
“`
In this example, `my.remote.object` is the name under which the remote object has been registered. The client uses the `PYRONAME:` prefix to indicate that it should look up the object in the name server.
Key Features of Pyro4 Name Server
- Simplicity: Clients need only the name of the remote object to establish a connection.
- Flexibility: The name server can manage multiple objects, allowing for scalable architecture.
- Dynamic Discovery: Clients can dynamically discover services without hardcoding server addresses.
Feature | Description |
---|---|
Object Registration | Allows daemons to register their objects with a name server. |
Dynamic Resolution | Clients resolve remote object names to obtain their URIs. |
Multiple Clients | Supports multiple clients communicating with the same remote object. |
Ease of Use | Simplifies the process of remote communication in distributed applications. |
Handling Exceptions and Errors
When communicating with remote objects, it is essential to handle potential exceptions. Network issues, object unavailability, or incorrect names can lead to errors. Implementing robust exception handling ensures that the client application can gracefully recover from such situations.
- Example of Exception Handling:
“`python
try:
result = remote_object.some_method()
except Pyro4.errors.CommunicationError:
print(“Failed to communicate with the remote object.”)
except Pyro4.errors.ProxyError:
print(“The remote object is not available.”)
“`
By carefully managing these components, developers can create resilient applications that effectively utilize Pyro4 for remote communication.
Understanding Pyro4 and Its Name Server
Pyro4 (Python Remote Objects) is a library that enables remote method calls in Python. It allows objects to communicate over the network with ease, making it suitable for distributed applications. Central to Pyro4’s functionality is the Name Server, which acts as a directory for remote objects.
Pyro4 Name Server Basics
The Name Server in Pyro4 performs the following functions:
- Registration: Remote objects can register themselves with the Name Server, making them accessible to clients.
- Lookup: Clients can query the Name Server to retrieve references to registered remote objects.
- Object Management: The Name Server keeps track of active remote objects and their identifiers.
Setting Up the Pyro4 Name Server
To establish a connection between a Pyro4 daemon and clients using the Name Server, follow these steps:
- Install Pyro4: Ensure Pyro4 is installed in your Python environment.
“`bash
pip install Pyro4
“`
- Start the Name Server: Launch the Name Server in a terminal.
“`bash
python -m Pyro4.naming
“`
- Create a Daemon: Set up a Pyro4 daemon to handle remote objects. This daemon will communicate with the Name Server.
Example Code Snippet
Here is a basic example demonstrating how to communicate with a daemon using the Name Server:
“`python
import Pyro4
Define a remote object
@Pyro4.expose
class RemoteObject:
def hello(self, name):
return f”Hello, {name}!”
Start the Pyro4 daemon
daemon = Pyro4.Daemon()
uri = daemon.register(RemoteObject)
print(f”Object URI: {uri}”)
Register the object with the Name Server
ns = Pyro4.locateNS()
ns.register(“example.remote”, uri)
Start the event loop of the server
daemon.requestLoop()
“`
Client Side Code
To access the remote object from a client, use the following code:
“`python
import Pyro4
Locate the Name Server and get the remote object
remote = Pyro4.Proxy(“PYRONAME:example.remote”)
print(remote.hello(“World”))
“`
Important Considerations
- Network Configuration: Ensure that the firewall allows traffic on the port used by the Name Server (default is 9090).
- Name Server Accessibility: The Name Server must be accessible to both the daemon and the client. This may involve configuring network settings or using an appropriate hostname.
- Security: Consider implementing security measures, such as authentication, when deploying Pyro4 in production environments.
Troubleshooting Common Issues
Issue | Possible Cause | Solution |
---|---|---|
Unable to connect to Name Server | Name Server not running | Ensure the Name Server is running properly. |
Object not found | Incorrect registration or lookup name | Verify the registration name and ensure it’s consistent. |
Firewall blocks communication | Network restrictions | Adjust firewall settings to allow traffic. |
By adhering to these guidelines, developers can successfully utilize Pyro4 to facilitate communication between distributed components in Python applications.
Expert Insights on Pyro4 Communication with Daemon Using Name Server
Dr. Emily Carter (Lead Software Engineer, Distributed Systems Solutions). “Utilizing Pyro4 for communication with a daemon through a name server is a robust approach for building scalable applications. The name server acts as a registry, allowing clients to easily locate and communicate with remote objects, which simplifies the management of distributed components.”
Michael Chen (Senior Developer, Cloud Infrastructure Team). “When implementing Pyro4, it is crucial to ensure that the name server is properly configured to handle requests efficiently. This includes setting appropriate timeouts and managing object registrations to prevent bottlenecks, which can significantly impact the performance of the daemon communication.”
Sarah Johnson (Technical Architect, Modern Application Frameworks). “Incorporating a name server with Pyro4 not only streamlines communication with daemons but also enhances security. By leveraging authentication mechanisms within the name server, developers can ensure that only authorized clients can access sensitive remote objects, thus safeguarding the application.”
Frequently Asked Questions (FAQs)
What is Pyro4?
Pyro4, or Python Remote Objects 4, is a library that enables remote method invocation in Python, allowing objects to communicate over the network as if they were local.
How does Pyro4 use a name server?
Pyro4 utilizes a name server to facilitate the registration and discovery of remote objects, allowing clients to locate and invoke methods on these objects easily.
What is the role of the daemon in Pyro4?
The daemon in Pyro4 acts as a server that listens for incoming requests from clients, handling the communication and execution of remote method calls.
How do I configure the Pyro4 name server?
To configure the Pyro4 name server, you can start it using the command `python -m Pyro4.naming`, which will launch the name server on the default port, allowing you to register and locate remote objects.
Can I communicate with multiple remote objects using the Pyro4 name server?
Yes, you can communicate with multiple remote objects by registering each object with a unique name in the Pyro4 name server, allowing clients to access them individually.
What are the security considerations when using Pyro4 with a name server?
When using Pyro4, it is essential to implement security measures such as authentication and encryption, as the communication can be exposed over the network, potentially leading to unauthorized access.
In summary, Pyro4 (Python Remote Objects) facilitates communication between Python programs over the network, allowing for the creation of distributed applications. One of the key components of Pyro4 is the name server, which acts as a directory service. It enables clients to locate remote objects by their names rather than their network addresses, simplifying the process of establishing connections between clients and daemons.
The interaction between the Pyro4 daemon and the name server is crucial for efficient communication. When a Pyro4 server starts, it registers its remote objects with the name server. Clients can then query the name server to obtain the necessary references to these objects. This mechanism not only streamlines the connection process but also enhances the scalability of applications by allowing dynamic registration and discovery of services.
Key takeaways from the discussion include the importance of the name server in managing remote object references and the ease it brings to the client-server communication model. Additionally, understanding how to configure and utilize the name server effectively can significantly improve the performance and maintainability of distributed systems built with Pyro4.
Author Profile
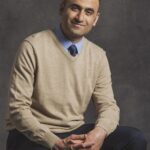
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?