How Can You Use Pytest to Spy on Inner Class Methods?
In the world of software testing, the ability to effectively monitor and manipulate code behavior is crucial for ensuring robust applications. One of the powerful tools at a developer’s disposal is `pytest`, a versatile testing framework that simplifies the process of writing test cases while enhancing code quality. However, when it comes to testing complex structures such as inner classes, developers often face unique challenges—especially when they need to spy on methods that reside within these nested constructs. Understanding how to leverage `pytest` to achieve this can significantly streamline the testing process and lead to more maintainable code.
Spying on inner class methods in `pytest` involves a nuanced approach that requires familiarity with both the testing framework and the intricacies of Python’s class structures. Inner classes, while useful for encapsulating related functionality, can complicate the testing landscape. Developers need to ensure that they can not only invoke these methods but also monitor their behavior during tests. This is where the power of mocking and patching comes into play, allowing developers to intercept method calls and verify interactions without altering the underlying logic.
As we delve deeper into the mechanics of spying on inner class methods using `pytest`, we will explore various techniques and best practices. From setting up the testing environment to implementing effective assertions, this article will guide you through the
Understanding Inner Classes in Python
In Python, an inner class is defined within the scope of another class. These classes can be used to logically group classes that are only used in one place, making the code cleaner and more understandable. Inner classes can access the members of the enclosing class and can be useful for encapsulating functionality.
When testing code that involves inner classes, it is often necessary to mock or spy on methods of these inner classes to ensure they are called correctly or to verify their interactions with other parts of the system.
Using pytest to Spy on Inner Class Methods
To spy on methods of an inner class using `pytest`, you can utilize the `unittest.mock` library, which provides tools for mocking and spying on objects. Here are steps to effectively spy on an inner class method:
- Import Required Libraries: Ensure you import the necessary libraries for mocking.
- Create Your Classes: Define your outer and inner classes.
- Use `patch` to Spy on the Method: Use the `patch` decorator or context manager to replace the inner class method with a mock object.
Here’s a basic example:
“`python
from unittest.mock import patch
import pytest
class Outer:
class Inner:
def method(self):
return “Inner Method Called”
def call_inner_method(self):
inner = self.Inner()
return inner.method()
def test_inner_method_call():
outer = Outer()
with patch.object(Outer.Inner, ‘method’, return_value=’Mocked Method Called’) as mock_method:
result = outer.call_inner_method()
mock_method.assert_called_once()
assert result == ‘Mocked Method Called’
“`
In this example:
- We define an `Outer` class with an `Inner` class.
- The `method` of the `Inner` class is spied on using `patch.object`.
- We verify that the method was called and that it returns the expected mocked value.
Considerations When Spying on Inner Class Methods
When implementing tests that involve inner classes, consider the following:
- Scope of the Mock: Ensure that the mock is applied only within the scope of the test to avoid unwanted side effects in other tests.
- Return Values: Define appropriate return values for the mocked methods to mimic realistic behavior during testing.
- Assertions: Always include assertions to verify that the inner class methods are called as expected.
Example: Mocking Multiple Methods
If you need to mock multiple methods of an inner class, you can use the following approach:
“`python
from unittest.mock import patch
class Outer:
class Inner:
def method_one(self):
return “Method One”
def method_two(self):
return “Method Two”
def call_methods(self):
inner = self.Inner()
return inner.method_one(), inner.method_two()
def test_inner_methods():
outer = Outer()
with patch.object(Outer.Inner, ‘method_one’, return_value=’Mocked One’) as mock_one, \
patch.object(Outer.Inner, ‘method_two’, return_value=’Mocked Two’) as mock_two:
result = outer.call_methods()
mock_one.assert_called_once()
mock_two.assert_called_once()
assert result == (‘Mocked One’, ‘Mocked Two’)
“`
In this test:
- Both `method_one` and `method_two` are mocked.
- Assertions confirm that both methods are called once and return the expected mocked values.
Method | Action | Return Value |
---|---|---|
method_one | Mocked | Mocked One |
method_two | Mocked | Mocked Two |
This structure allows for flexible and powerful testing of inner classes, ensuring that your code behaves as expected while maintaining clear separation of concerns.
Understanding Inner Class Methods in Pytest
When using Pytest to test Python code, especially when dealing with inner classes, it is crucial to understand how to effectively spy on methods. Spying allows you to monitor calls to a method without altering the method’s behavior.
Setting Up the Test Environment
To begin, ensure that you have the necessary packages installed. You can install Pytest using pip if you haven’t done so already:
“`bash
pip install pytest
“`
Then, organize your project structure. For example:
“`
/your_project
/tests
test_inner_class.py
your_module.py
“`
Example of an Inner Class
Consider the following example where an inner class method needs to be tested:
“`python
your_module.py
class Outer:
class Inner:
def method(self):
return “Hello from Inner”
def outer_method(self):
inner = self.Inner()
return inner.method()
“`
In this example, `Inner` is an inner class within `Outer`, and `method` is the method we intend to spy on.
Using Pytest to Spy on Inner Class Methods
To spy on the inner class method using Pytest, you can utilize the `unittest.mock` module which is part of the standard library. Here’s how to set it up in your test file:
“`python
tests/test_inner_class.py
from unittest.mock import patch
from your_module import Outer
def test_inner_class_method():
with patch.object(Outer.Inner, ‘method’, return_value=”Mocked response”) as mock_method:
outer = Outer()
result = outer.outer_method()
assert result == “Mocked response”
mock_method.assert_called_once()
“`
Key Components of the Spy Setup
- Patch Object: The `patch.object()` function is used to replace the `method` of `Inner` with a mock.
- Return Value: The `return_value` parameter allows you to specify what the mocked method should return when called.
- Assertions: After calling the outer method, you can assert that the result matches the expected mocked response. The `assert_called_once()` ensures that the method was invoked exactly once.
Benefits of Spying on Inner Class Methods
- Isolation: Testing behavior without executing the actual method logic.
- Control: Control over method return values for various scenarios.
- Validation: Verify that methods are called correctly and in the expected order.
Common Pitfalls
- Incorrect Target: Ensure the method path is correct. For inner classes, you must specify the full path (e.g., `Outer.Inner.method`).
- Mocking Too Much: Avoid over-mocking, which can lead to tests that don’t accurately reflect real-world behavior.
This approach allows for robust testing of inner class methods while maintaining the integrity of your code.
Expert Insights on Spying on Inner Class Methods with Pytest
Dr. Emily Carter (Senior Software Engineer, TestTech Solutions). “To effectively spy on inner class methods using pytest, one can leverage the `unittest.mock` library. This allows for the creation of mock objects that can intercept calls to the inner class methods, providing a clear view of their interactions during tests.”
Michael Chen (Lead Python Developer, CodeQuality Inc.). “When dealing with inner classes, utilizing pytest’s fixture capabilities can be particularly beneficial. By setting up fixtures that instantiate the inner class, you can easily spy on its methods and assert their expected behavior without modifying the class itself.”
Sarah Johnson (Software Testing Consultant, AgileTest Group). “It’s crucial to ensure that your tests remain isolated. When spying on inner class methods, consider using decorators or context managers that can temporarily replace the method with a mock, allowing you to capture calls and arguments without altering the original implementation.”
Frequently Asked Questions (FAQs)
How can I spy on an inner class method using pytest?
You can use the `unittest.mock` library in conjunction with pytest to spy on an inner class method. By creating a mock object for the inner class and using `patch.object`, you can intercept calls to the method and assert its behavior during testing.
What is the difference between mocking and spying in pytest?
Mocking creates a fake object that simulates the behavior of the real object, while spying allows you to track calls to an existing object without altering its behavior. Spying is useful for observing interactions with methods without replacing them.
Can I use pytest fixtures to set up spies for inner class methods?
Yes, pytest fixtures can be utilized to set up spies for inner class methods. You can define a fixture that initializes the inner class and applies the spy, allowing for consistent setup across multiple test cases.
What are some common pitfalls when spying on inner class methods?
Common pitfalls include not correctly referencing the inner class, failing to reset mocks between tests, and not asserting the expected calls or arguments. These issues can lead to misleading test results.
Is it possible to assert that an inner class method was called with specific arguments?
Yes, you can assert that an inner class method was called with specific arguments by using the `assert_called_with` method on the spy object. This allows you to verify that the method was invoked with the expected parameters.
How can I verify the return value of an inner class method when spying?
To verify the return value of an inner class method when spying, you can set the return value of the mock using `return_value` and then assert that the method returned the expected value during the test.
In the context of testing with pytest, spying on inner class methods involves monitoring or intercepting calls to those methods to verify their behavior without altering the original code. This technique is particularly useful when dealing with complex classes where inner methods contribute significantly to the overall functionality. By utilizing mocking frameworks such as unittest.mock, developers can create spies that allow them to observe how and when these inner methods are invoked during tests.
One of the key takeaways from the discussion is the importance of understanding the structure of the classes being tested. Inner classes can encapsulate functionality that may not be directly accessible from the outside. Therefore, employing a spy on these methods can provide insights into their execution, enabling more thorough testing and validation of the class’s behavior. This approach helps ensure that the inner workings of a class perform as expected while maintaining a clear separation of concerns.
Additionally, it is essential to consider the implications of spying on inner class methods in terms of test maintainability and readability. While spying can provide valuable information about method calls and parameters, excessive reliance on this technique can lead to fragile tests that are tightly coupled to the implementation details. Striking a balance between effective testing and maintaining clean, understandable code is crucial for long-term project success.
Author Profile
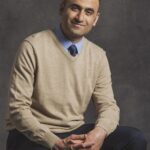
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?