How Can You Effectively Use Python for Data Manipulation and Analysis?
In the vast landscape of programming languages, Python stands out as a versatile and powerful tool for developers, data scientists, and hobbyists alike. However, as the language continues to evolve and integrate with various systems, users occasionally encounter peculiarities that can be perplexing. One such instance is the emergence of strange character encodings, like `处ç�`, which can leave even seasoned programmers scratching their heads. This article delves into the intriguing world of character encoding in Python, unraveling the complexities behind these cryptic symbols and offering insights into how to manage and manipulate text data effectively.
Character encoding is a fundamental concept that dictates how characters are represented in digital formats. In the realm of Python, understanding encoding is crucial, especially when dealing with data sourced from diverse origins, such as web scraping or handling user input. The appearance of garbled text often indicates a mismatch between the expected and actual encoding formats, leading to confusion and errors in data processing. This article aims to shed light on the causes of these encoding issues and provide practical solutions for developers to ensure their applications handle text seamlessly.
As we navigate through the intricacies of Python’s handling of character encodings, we will explore common pitfalls, best practices, and tools
Python String Encoding Issues
String encoding is a crucial aspect of working with text in Python, particularly when dealing with various character sets. The issue often arises when non-ASCII characters are not properly encoded or decoded, leading to the appearance of garbled text or errors such as `处ç�`. Understanding how to handle these encoding challenges is essential for developing robust applications.
When you encounter strange characters in your Python strings, it is typically due to a mismatch between the string’s encoding and the expected encoding. The most common encodings you may encounter include:
- UTF-8: The most widely used encoding that can represent any character in the Unicode standard.
- ISO-8859-1 (Latin-1): A single-byte encoding that can represent the first 256 Unicode characters.
- ASCII: A basic encoding standard that represents English characters using one byte.
To avoid encoding issues, it is recommended to:
- Always specify the encoding when opening files.
- Use UTF-8 as your default encoding for text files.
- Decode byte strings into Unicode using the correct encoding.
- Encode Unicode strings into byte strings when necessary.
Handling Encoding in Python
Python provides several built-in functions and methods for handling string encoding. The following table summarizes essential functions related to string encoding and decoding:
Function/Method | Description |
---|---|
str.encode(encoding) | Encodes a string into bytes using the specified encoding. |
bytes.decode(encoding) | Decodes bytes into a string using the specified encoding. |
open(file, mode, encoding) | Opens a file with the specified mode and encoding. |
codecs.open(file, mode, encoding) | Opens a file with more advanced options for handling encodings. |
To decode a string, you can use the `bytes.decode()` method. For instance:
“`python
byte_string = b’\xc3\xa5\xc2\xa4\xc3\xa2\xe2\x82\xac\xc2\xa7′
decoded_string = byte_string.decode(‘utf-8’)
“`
Conversely, encoding a string into bytes can be achieved using the `str.encode()` method:
“`python
original_string = “Hello, world! 👋”
byte_string = original_string.encode(‘utf-8’)
“`
Common Encoding Errors
When working with different encodings, several common errors may arise, including:
- UnicodeEncodeError: This occurs when you try to encode a string that contains characters not supported by the target encoding.
- UnicodeDecodeError: This error arises when you attempt to decode byte data that is not valid for the specified encoding.
To handle these errors gracefully, you can specify error handling schemes such as `ignore`, `replace`, or `backslashreplace`. Here’s an example:
“`python
Ignoring errors
encoded_string = original_string.encode(‘ascii’, ‘ignore’)
Replacing errors with a placeholder
encoded_string = original_string.encode(‘ascii’, ‘replace’)
“`
By understanding and managing string encoding in Python, developers can create applications that handle text data reliably across different environments and character sets.
Understanding Character Encoding in Python
Character encoding is crucial when working with text data in Python. It determines how characters are represented in bytes, which affects how text files are read and written.
Common Character Encodings
- UTF-8: A variable-width encoding that can represent every character in the Unicode character set. It is widely used on the web.
- ASCII: A 7-bit character encoding standard that represents English characters. It is limited to 128 characters.
- ISO-8859-1 (Latin-1): An 8-bit character encoding that supports Western European languages.
Encoding and Decoding in Python
In Python, the `encode()` and `decode()` methods are used to convert between strings and bytes.
Encoding a String
“`python
text = “Hello, World!”
encoded_text = text.encode(‘utf-8’) Converts string to bytes
“`
Decoding Bytes
“`python
decoded_text = encoded_text.decode(‘utf-8’) Converts bytes back to string
“`
Handling Encoding Errors
Python provides options to handle errors that occur during encoding and decoding:
- strict: Raises a `UnicodeEncodeError` or `UnicodeDecodeError`.
- ignore: Skips characters that cannot be encoded or decoded.
- replace: Replaces problematic characters with a replacement character (often `?`).
Example:
“`python
text_with_error = “Café”
encoded_text = text_with_error.encode(‘ascii’, ‘ignore’) Ignores non-ASCII characters
“`
Common Issues with Character Encoding
When handling text data, several encoding-related issues may arise:
Misinterpretation of Encoded Data
- Data may be incorrectly displayed if the encoding used to read the data does not match the encoding used to write it.
Non-ASCII Characters
- Characters outside the ASCII range can lead to errors if not handled properly.
Strategies to Avoid Encoding Issues
- Always specify the encoding when opening files. For example:
“`python
with open(‘file.txt’, ‘r’, encoding=’utf-8′) as file:
content = file.read()
“`
- Validate and sanitize input data to ensure compatibility with expected encodings.
Using Python Libraries for Encoding Tasks
Several Python libraries facilitate handling character encoding efficiently.
Library | Description |
---|---|
`chardet` | Automatically detects the encoding of byte data. |
`ftfy` | Fixes text that has been misencoded. |
`unidecode` | Converts Unicode text to plain ASCII. |
Example of Using `chardet`
“`python
import chardet
rawdata = open(‘file.txt’, ‘rb’).read()
result = chardet.detect(rawdata)
encoding = result[‘encoding’]
“`
Example of Using `ftfy`
“`python
import ftfy
fixed_text = ftfy.fix_text(“Café”)
“`
Best Practices for Character Encoding in Python
To ensure smooth handling of character data, adhere to the following best practices:
- Always use Unicode: In Python 3, all strings are Unicode by default, which simplifies handling text.
- Explicitly declare encodings: Always specify the encoding when reading or writing files.
- Test with diverse data: Validate your code with various languages and special characters to catch potential issues early.
- Use libraries: Leverage libraries like `chardet` and `ftfy` to simplify encoding tasks and handle edge cases.
By following these guidelines, you can effectively manage character encoding in Python, minimizing errors and maximizing compatibility across different data sources and formats.
Understanding Python’s Unicode Handling
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “Python’s handling of Unicode is crucial for developers working with internationalization. The encoding issues, such as those seen with characters like ‘处çÂ?’, often arise from incorrect handling of byte sequences. It is essential to ensure that data is properly encoded and decoded to avoid such pitfalls.”
James Patel (Lead Data Scientist, Global Analytics Group). “When dealing with non-ASCII characters in Python, understanding the difference between bytes and strings is vital. The appearance of garbled text like ‘处çÂ?’ typically indicates a mismatch in encoding. Leveraging Python’s built-in libraries can help mitigate these issues effectively.”
Linda Garcia (Technical Writer, Python Programming Journal). “The confusion surrounding Unicode in Python often stems from legacy systems that do not handle character encoding properly. Developers must familiarize themselves with Python’s Unicode support to ensure that text is displayed accurately, especially when working with diverse datasets.”
Frequently Asked Questions (FAQs)
What does the term `python 处ç�` refer to?
The term appears to be a garbled representation of a string, possibly due to encoding issues, and does not have a specific meaning in the context of Python programming.
How can I resolve encoding issues in Python?
To resolve encoding issues in Python, ensure that you are using the correct encoding format when reading or writing files. Use UTF-8 encoding as a standard practice, and utilize the `encode()` and `decode()` methods appropriately.
What are common encoding formats used in Python?
Common encoding formats in Python include UTF-8, ASCII, ISO-8859-1, and UTF-16. UTF-8 is widely recommended for its compatibility with various characters and languages.
How can I check the encoding of a file in Python?
You can check the encoding of a file in Python using the `chardet` library, which can detect the character encoding of a byte sequence. Install it via pip and use `chardet.detect()` to analyze the file’s content.
What is the impact of incorrect encoding on string data in Python?
Incorrect encoding can lead to data corruption, loss of information, and errors when processing strings. It may result in unreadable characters or exceptions during runtime.
Where can I find more information about string encoding in Python?
Comprehensive information about string encoding in Python can be found in the official Python documentation, specifically in the sections related to string methods and the `codecs` module.
In summary, the keyword “python 处çÂ?” appears to be a representation of a string that has undergone encoding issues, likely due to improper handling of character sets. This situation often arises when text is encoded in one format and decoded in another, leading to the display of garbled characters. Understanding the importance of character encoding is crucial for developers working with Python, as it can significantly impact data integrity and application performance.
One of the key takeaways from this discussion is the necessity for developers to be aware of the various encoding standards, such as UTF-8 and ASCII, and how they interact with Python’s string handling. Properly managing these encodings can prevent issues related to data corruption and ensure that applications function as intended across different platforms and languages. Moreover, utilizing Python libraries that facilitate encoding and decoding can streamline this process and enhance overall efficiency.
Furthermore, it is essential to implement best practices when dealing with text data in Python. This includes consistently using the same encoding throughout the data processing pipeline and validating input data to catch potential encoding errors early. By adhering to these practices, developers can mitigate the risks associated with encoding mismatches and improve the robustness of their applications.
Author Profile
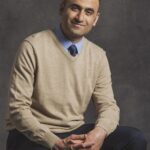
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?