How Can I Establish a JDBC Connection Using Python Code?
In the world of data management and application development, the ability to connect to databases is paramount. For Python developers, establishing a JDBC (Java Database Connectivity) connection may seem like a daunting task, especially when navigating the intricacies of integrating Java-based technologies with Python’s dynamic ecosystem. However, with the right approach and tools, this process can be both straightforward and efficient. This article will guide you through the essentials of creating a JDBC connection using Python, unlocking the potential for seamless data manipulation and interaction.
At its core, JDBC is a powerful API that allows Java applications to interact with a wide range of databases. While Python has its own set of database connectors, the need to leverage existing Java-based systems often arises. Understanding how to bridge these two worlds can enhance your application’s capabilities and provide access to robust data sources. By utilizing libraries such as JayDeBeApi or JPype, Python developers can tap into JDBC’s versatility, allowing for efficient data retrieval and management.
As we delve deeper into the mechanics of establishing a JDBC connection in Python, we will explore the necessary prerequisites, configuration steps, and best practices. Whether you’re looking to integrate legacy systems or simply expand your database interaction options, mastering JDBC connections in Python can significantly enhance your development toolkit. Get ready to unlock new possibilities and
Establishing a JDBC Connection with Python
To work with JDBC in Python, the commonly used library is `jaydebeapi`. This library allows you to connect to databases using JDBC drivers, which is particularly useful for Java-based databases. Below are the steps required to set up and establish a JDBC connection.
Prerequisites
Before establishing a connection, ensure you have the following:
- Python installed (preferably Python 3.x)
- The `jaydebeapi` library installed. You can install it via pip:
“`
pip install jaydebeapi
“`
- The corresponding JDBC driver for your database, which must be accessible in your environment.
Sample Code for JDBC Connection
The following is a basic example of Python code to establish a JDBC connection using the `jaydebeapi` library:
“`python
import jaydebeapi
Database connection parameters
jdbc_driver = ‘com.mysql.cj.jdbc.Driver’
jdbc_url = ‘jdbc:mysql://localhost:3306/your_database’
username = ‘your_username’
password = ‘your_password’
driver_path = ‘/path/to/mysql-connector-java-x.x.x.jar’ Path to your JDBC driver
Establishing connection
try:
conn = jaydebeapi.connect(jdbc_driver, jdbc_url, [username, password], driver_path)
curs = conn.cursor()
Execute a sample query
curs.execute(“SELECT * FROM your_table”)
results = curs.fetchall()
for row in results:
print(row)
except Exception as e:
print(“An error occurred:”, e)
finally:
curs.close()
conn.close()
“`
Connection Parameters Explained
Parameter | Description |
---|---|
`jdbc_driver` | The fully qualified name of the JDBC driver class. |
`jdbc_url` | The JDBC URL to connect to the database. |
`username` | The username to authenticate with the database. |
`password` | The password for the username provided. |
`driver_path` | The file path to the JDBC driver JAR file. |
Common JDBC Drivers
When working with various databases, you will need specific JDBC drivers. Below is a list of common databases and their corresponding JDBC driver class names:
- MySQL: `com.mysql.cj.jdbc.Driver`
- PostgreSQL: `org.postgresql.Driver`
- Oracle: `oracle.jdbc.driver.OracleDriver`
- SQL Server: `com.microsoft.sqlserver.jdbc.SQLServerDriver`
Ensure you have the correct driver installed and referenced in your code for successful connections.
Error Handling
Implementing error handling is crucial when establishing a JDBC connection. Utilize try-except blocks to catch and manage exceptions effectively. The following are common errors you might encounter:
- Driver Not Found: Ensure that the JDBC driver is correctly specified and the path is valid.
- Connection Timeout: Verify network connectivity and database server status.
- Authentication Failed: Check username and password for correctness.
By following these guidelines and utilizing the provided code, you can efficiently establish a JDBC connection using Python, facilitating interactions with your database of choice.
Establishing a JDBC Connection in Python
To establish a JDBC connection in Python, you typically use the `jaydebeapi` library, which allows you to connect to databases using JDBC drivers. Below is a detailed guide on how to set this up effectively.
Prerequisites
Before you start, ensure that you have the following:
- Python: Make sure Python is installed on your system.
- JayDeBeApi: This library needs to be installed. You can do this via pip:
“`bash
pip install JayDeBeApi
“`
- JPype1: This is a dependency for JayDeBeApi, also installable via pip:
“`bash
pip install JPype1
“`
- JDBC Driver: Download the appropriate JDBC driver for your database and note its path.
Example Code for JDBC Connection
Here is a sample code snippet demonstrating how to connect to a JDBC-compliant database using Python:
“`python
import jaydebeapi
Database connection details
jdbc_driver = ‘com.mysql.cj.jdbc.Driver’ Example driver for MySQL
jdbc_url = ‘jdbc:mysql://localhost:3306/mydatabase’
username = ‘your_username’
password = ‘your_password’
driver_path = ‘/path/to/mysql-connector-java-8.0.25.jar’ Update this path
try:
Establishing the connection
conn = jaydebeapi.connect(jdbc_driver, jdbc_url, [username, password], driver_path)
Creating a cursor object using the connection
curs = conn.cursor()
Example query execution
curs.execute(“SELECT * FROM your_table”)
results = curs.fetchall()
Processing results
for row in results:
print(row)
except Exception as e:
print(f”An error occurred: {e}”)
finally:
Closing the cursor and connection
if curs:
curs.close()
if conn:
conn.close()
“`
Connection Parameters
The connection parameters include:
Parameter | Description |
---|---|
`jdbc_driver` | Fully qualified name of the JDBC driver. |
`jdbc_url` | URL to connect to the database. |
`username` | Username for database authentication. |
`password` | Password for database authentication. |
`driver_path` | Path to the JDBC driver JAR file. |
Handling Exceptions
When working with database connections, it’s essential to handle exceptions properly. Common exceptions include:
- Database Connection Errors: Issues related to network or authentication.
- SQL Errors: Errors that arise during query execution.
Utilize `try-except` blocks to manage these exceptions gracefully.
Best Practices
- Always close your connections and cursors to prevent resource leaks.
- Use parameterized queries to protect against SQL injection.
- Log errors and exceptions for troubleshooting purposes.
By following this structure, you can create a robust Python application capable of connecting to and interacting with various databases via JDBC.
Expert Insights on Python JDBC Connection Implementation
Dr. Emily Chen (Senior Data Engineer, Tech Innovations Inc.). “Establishing a JDBC connection in Python typically involves using a library such as JayDeBeApi. This library allows seamless integration between Python and Java databases, enabling developers to execute SQL queries effectively.”
Mark Thompson (Lead Software Architect, Data Solutions Group). “When implementing JDBC connections in Python, it is crucial to manage your dependencies carefully. Utilizing virtual environments can prevent conflicts and ensure that the correct versions of libraries like JPype and JayDeBeApi are used.”
Sarah Patel (Database Administrator, CloudTech Systems). “Security should always be a priority when establishing JDBC connections. Ensure that sensitive information such as database credentials is stored securely and consider using environment variables to keep them out of your source code.”
Frequently Asked Questions (FAQs)
What is JDBC in the context of Python?
JDBC, or Java Database Connectivity, is a Java-based API that enables Java applications to interact with databases. While Python does not use JDBC directly, similar functionality can be achieved through libraries like `jaydebeapi` or `JPype` to connect to Java-based databases.
How can I establish a JDBC connection in Python?
To establish a JDBC connection in Python, use the `jaydebeapi` library. First, install it using pip, then import the library, load the JDBC driver, and create a connection string with the appropriate database credentials.
What libraries are required for JDBC connections in Python?
The primary libraries required are `jaydebeapi` for the JDBC connection and `JPype` to interface with Java. Additionally, you will need the JDBC driver for your specific database.
Can you provide a sample Python code for JDBC connection?
Certainly. Here is a sample code snippet:
“`python
import jaydebeapi
JDBC connection details
jdbc_driver = ‘com.mysql.cj.jdbc.Driver’
jdbc_url = ‘jdbc:mysql://localhost:3306/mydatabase’
username = ‘myusername’
password = ‘mypassword’
Establishing the connection
conn = jaydebeapi.connect(jdbc_driver, jdbc_url, [username, password], ‘path/to/mysql-connector-java.jar’)
“`
What are common issues when using JDBC with Python?
Common issues include incorrect JDBC driver paths, mismatched database credentials, and compatibility problems between the JDBC driver and the database version. Ensure that all configurations are correctly set up.
Is JDBC the best option for database connections in Python?
JDBC is not typically the best option for Python. Native libraries such as `psycopg2` for PostgreSQL or `mysql-connector-python` for MySQL are generally preferred as they provide better performance and integration with Python’s ecosystem.
In summary, establishing a JDBC connection using Python involves utilizing libraries that facilitate interaction with Java Database Connectivity (JDBC) drivers. While Python does not natively support JDBC, tools such as JayDeBeApi and JPype enable Python applications to connect to databases that are typically accessed through JDBC. These libraries allow developers to leverage the power of JDBC while working within the Python ecosystem, making it possible to execute SQL queries and retrieve results seamlessly.
Key insights from the discussion highlight the importance of ensuring that the appropriate JDBC driver is available and correctly configured within the Python environment. Additionally, understanding the connection string format and the parameters required for successful database connectivity is crucial. This knowledge empowers developers to troubleshoot any issues that may arise during the connection process and enhances their ability to work with various database systems.
Furthermore, the integration of Python with JDBC opens up a range of possibilities for data manipulation and analysis. By combining Python’s extensive libraries for data science and machine learning with JDBC’s robust database access capabilities, developers can create powerful applications that leverage the strengths of both technologies. This synergy ultimately leads to more efficient data processing workflows and improved application performance.
Author Profile
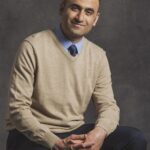
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?