What is the Python Equivalent of PHP’s isset() Function?
In the world of web development, the ability to check for the existence of variables is a fundamental skill that can significantly impact the robustness of your code. For PHP developers, the `isset()` function serves as a reliable tool for determining whether a variable is set and is not `NULL`. However, as the programming landscape evolves, many developers are exploring Python as an alternative for its simplicity and versatility. This raises an important question: what is the Python equivalent of PHP’s `isset()`?
In this article, we will delve into the nuances of variable existence checks in Python, drawing comparisons to PHP’s approach. Understanding how to verify variable presence is crucial for preventing errors and ensuring smooth execution of your code. We’ll explore the mechanisms that Python offers, including its unique handling of variables and data types, which can differ significantly from PHP’s methodology.
Join us as we navigate through the various techniques and best practices in Python for checking variable existence, equipping you with the knowledge to write cleaner, more efficient code. Whether you’re transitioning from PHP to Python or simply looking to enhance your programming toolkit, this exploration will provide valuable insights into effective variable management in Python.
Understanding the `isset` Function in PHP
The `isset` function in PHP is used to determine if a variable is set and is not `NULL`. It is a commonly used function for checking if a variable exists before performing operations on it. The syntax for `isset` is straightforward:
“`php
isset($variable);
“`
This function returns `true` if the variable exists and is not `NULL`, and “ otherwise. It is particularly useful for handling form data and checking for the existence of optional variables.
Key Characteristics of `isset`
- Returns `true` for any variable that has been declared and is not `NULL`.
- Can accept multiple variables as arguments; it will return `true` only if all variables are set.
- Does not raise a notice if the variable is not defined.
Python Equivalent of PHP’s `isset`
In Python, the equivalent functionality can be achieved using the `in` keyword or by employing the `get` method for dictionaries. The following methods can be used to check if a variable exists or has a value:
- Using the `in` operator: This is particularly applicable to dictionaries.
“`python
if ‘key’ in dictionary:
key exists in the dictionary
“`
- Using `get` method: This method returns `None` if the key does not exist, allowing you to check for both existence and value.
“`python
value = dictionary.get(‘key’)
if value is not None:
key exists and is not None
“`
Comparison of PHP and Python Approaches
The following table outlines the differences between PHP’s `isset` and Python’s approaches:
Feature | PHP (isset) | Python (in / get) |
---|---|---|
Check for existence | isset($variable) | ‘key’ in dictionary |
Check for None | isset($variable) and $variable !== NULL | dictionary.get(‘key’) is not None |
Multiple variables | isset($var1, $var2) | No direct equivalent; check individually |
Practical Examples
Here are practical examples demonstrating both PHP and Python usage:
- PHP Example:
“`php
if (isset($user)) {
echo “User is set.”;
} else {
echo “User is not set.”;
}
“`
- Python Example:
“`python
if ‘user’ in locals():
print(“User is set.”)
else:
print(“User is not set.”)
“`
In these examples, both languages effectively check for the existence of a variable, showcasing their respective syntactical differences while achieving similar outcomes.
Understanding `isset()` in PHP
The `isset()` function in PHP is commonly used to check if a variable is set and is not `NULL`. It returns `true` if the variable exists and is not `NULL`, and “ otherwise. Here are key characteristics of `isset()`:
- It can check multiple variables at once.
- It returns “ if the variable has been set to `NULL`.
- It does not generate a warning if the variable does not exist.
Example in PHP:
“`php
$var = “Hello”;
if (isset($var)) {
echo “Variable is set!”;
}
“`
Python Equivalent: Checking Variable Existence
In Python, there is no direct equivalent to `isset()`. However, you can achieve similar functionality using various methods. Here are some common approaches:
- Using `in` Keyword: This checks for the existence of a key in a dictionary.
- Using `try` and `except`: This captures `NameError` if a variable is not defined.
- Using `getattr()`: This checks for attributes in objects, useful for class instances.
Examples of Python Equivalents
Here are examples demonstrating how to check variable existence in Python:
Using `in` with a Dictionary:
“`python
my_dict = {‘key’: ‘value’}
if ‘key’ in my_dict:
print(“Key exists!”)
“`
Using `try` and `except`:
“`python
try:
print(my_var)
except NameError:
print(“Variable is not defined.”)
“`
Using `getattr()`:
“`python
class MyClass:
def __init__(self):
self.attribute = “I exist”
obj = MyClass()
if hasattr(obj, ‘attribute’):
print(“Attribute exists!”)
“`
Comparing PHP `isset()` and Python Methods
The following table summarizes the differences between PHP’s `isset()` and Python’s methods to check variable existence:
Feature | PHP `isset()` | Python Method |
---|---|---|
Checks existence | Yes | Yes (using `in` or `try`) |
Returns boolean | Yes | Yes |
Handles `NULL` | Yes (returns ) | N/A (Python uses `None`) |
Multiple checks | Yes | Yes (using `all()` or loops) |
Error handling | No | Yes (with `try`/`except`) |
Conclusion on Usage
While PHP provides a straightforward function for checking variable existence, Python requires a more flexible approach that may involve different data structures or error handling techniques. Understanding these methods allows developers to write robust code that effectively handles variable existence checks.
Comparative Insights on PHP’s isset() and Python Alternatives
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, the equivalent of PHP’s isset() function can be achieved using the ‘in’ keyword or the ‘get()’ method for dictionaries. This allows developers to check for the existence of a key without raising an error, promoting safer code practices.”
Michael Chen (Lead Developer, CodeCraft Solutions). “While PHP’s isset() is a straightforward way to check for variable existence, Python offers a more flexible approach with the ‘try-except’ block or the ‘get()’ method on dictionaries. This not only checks for key existence but also allows for default values, enhancing code readability and functionality.”
Laura Patel (Technical Writer, Programming Insights). “Understanding the transition from PHP’s isset() to Python’s alternatives is crucial for developers. In Python, using ‘if key in dictionary’ or ‘dictionary.get(key, default_value)’ provides a robust way to handle variable checks, aligning with Python’s emphasis on readability and simplicity.”
Frequently Asked Questions (FAQs)
What is the Python equivalent of PHP’s isset() function?
In Python, the equivalent of PHP’s isset() function is using the `in` keyword to check if a variable exists in the local or global scope, or using `try` and `except` blocks to handle potential `NameError`.
How do I check if a variable is defined in Python?
You can check if a variable is defined in Python by using a `try` block to access the variable and catch a `NameError`, or by checking if the variable is in `locals()` or `globals()`.
Can I use the `get()` method for dictionaries in Python to check if a key exists?
Yes, the `get()` method can be used to check for the existence of a key in a dictionary. If the key exists, it returns the associated value; if not, it returns `None` or a specified default value.
What happens if I try to access an variable in Python?
If you attempt to access an variable in Python, it raises a `NameError`, indicating that the variable is not defined in the current scope.
Is there a built-in function in Python similar to isset()?
Python does not have a direct built-in function like `isset()`, but you can achieve similar functionality using `locals()`, `globals()`, or exception handling with `try` and `except`.
How can I check for multiple variables at once in Python?
You can check for multiple variables using a combination of `all()` with `in` checks against `locals()` or `globals()`, or by using a `try` block with multiple variable accesses to catch any `NameError`.
In the context of programming, the PHP function `isset()` is used to determine if a variable is set and is not null. In Python, there is no direct equivalent to `isset()`, but similar functionality can be achieved using various approaches. The most common method is to use the `in` keyword to check for the presence of a variable in the local or global scope, or to use exception handling to manage cases where a variable may not be defined. Additionally, Python’s `getattr()` function can be utilized to safely access attributes of an object, providing a way to check for their existence without raising an error.
Another important distinction is that Python treats variables differently than PHP. In Python, attempting to access an variable raises a `NameError`, while in PHP, `isset()` will simply return if the variable is not set. This difference in behavior necessitates a more cautious approach in Python when checking for variable existence, often involving the use of try-except blocks or default values with the `get()` method for dictionaries.
In summary, while there is no direct equivalent of PHP’s `isset()` in Python, developers can effectively check for variable existence through various techniques. Understanding these differences is crucial for transitioning
Author Profile
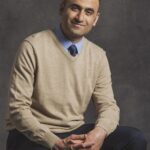
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?