How Can You Simplify If-Else Statements in Python to One Line?
In the world of programming, efficiency and readability are paramount, especially when it comes to writing conditional statements. Python, known for its clean and straightforward syntax, offers a powerful feature that allows developers to condense their code into a single line using the `if-else` statement. This not only streamlines the code but also enhances its clarity, making it easier to understand at a glance. Whether you’re a seasoned programmer or just starting your coding journey, mastering the art of one-liners in Python can significantly boost your productivity and coding style.
When it comes to implementing conditional logic in Python, the traditional multi-line `if-else` structure can sometimes feel cumbersome. However, Python’s concise syntax allows for a more elegant solution: the ternary conditional operator. This operator enables you to evaluate conditions and return values in a single, compact line. By leveraging this feature, you can write cleaner code that performs the same functionality as its multi-line counterpart, all while maintaining readability.
Moreover, the ability to write `if-else` statements in one line is not just a matter of aesthetics; it can also lead to more efficient code execution. In scenarios where simplicity and speed are crucial, such as in data processing or algorithm development, knowing how to implement these one-l
Python One-Line If-Else Statements
In Python, one-line if-else statements provide a succinct way to write conditional expressions. This syntax is often referred to as a “ternary operator” and can be particularly useful for simple conditions where you want to assign a value based on a condition without using multiple lines of code.
The basic syntax for a one-line if-else statement is as follows:
“`python
value_if_true if condition else value_if_
“`
This structure allows you to evaluate the condition, returning `value_if_true` if the condition is true and `value_if_` if it is not.
Examples of One-Line If-Else Statements
Here are a few practical examples illustrating how to use this syntax effectively:
- Assigning a variable based on a condition:
“`python
x = 10
result = “Even” if x % 2 == 0 else “Odd”
“`
In this example, `result` will be “Even” since `x` is indeed even.
- Choosing a message based on a score:
“`python
score = 85
message = “Pass” if score >= 60 else “Fail”
“`
Here, `message` will be “Pass” because the score meets the threshold.
Advantages of One-Line If-Else Statements
- Conciseness: Reduces the number of lines in your code, making it cleaner.
- Readability: For simple conditions, it can enhance readability by summarizing logic in a single line.
- Efficiency: Minimizes the overhead of multiple lines of code, which can be beneficial in tight loops.
Limitations to Consider
Despite their benefits, one-line if-else statements come with certain limitations:
- Complexity: They should be avoided for complex conditions that require multiple actions or nested conditions, as they can reduce readability.
- Debugging: Debugging can be more challenging when logic is condensed into one line.
Summary Table of Syntax and Use Cases
Use Case | One-Line Syntax | Example |
---|---|---|
Assigning a value | value_if_true if condition else value_if_ | result = “Yes” if a > b else “No” |
Returning a message | message = “Adult” if age >= 18 else “Minor” | message = “Adult” if age >= 18 else “Minor” |
Simple calculations | output = x * 2 if x > 0 else x | output = x * 2 if x > 0 else x |
Using one-line if-else statements can streamline your Python code when applied appropriately, allowing for more elegant solutions to simple decision-making tasks.
Using Ternary Operator for Conditional Expressions
In Python, a common approach to implement simple conditional logic in a single line is through the ternary operator, also known as the conditional expression. The syntax is structured as follows:
“`python
value_if_true if condition else value_if_
“`
This allows you to succinctly assign a value based on a condition without the need for a multi-line if-else statement. Here are some practical examples:
- Assigning a variable based on a condition:
“`python
x = 10
result = “Even” if x % 2 == 0 else “Odd”
“`
- Using it in function calls:
“`python
def get_status(age):
return “Adult” if age >= 18 else “Minor”
status = get_status(15) Returns “Minor”
“`
Nesting Ternary Operators
Ternary operators can be nested to handle multiple conditions, although readability may suffer. The syntax for nested conditions looks like this:
“`python
result = value_if_true if condition else (value_if_true2 if condition2 else value_if_)
“`
Example of nesting:
“`python
x = 5
result = “Positive” if x > 0 else “Zero” if x == 0 else “Negative”
“`
While nesting is possible, it’s advisable to use it judiciously to maintain code clarity.
Using Logical Operators for Multiple Conditions
For scenarios where you need to evaluate multiple conditions succinctly, logical operators can be combined with the ternary operator. This provides a more expressive way to handle complex conditions.
Example:
“`python
x = 20
result = “High” if x > 15 else “Medium” if x > 5 else “Low”
“`
This example checks if `x` is greater than 15 first, then checks if it is greater than 5, allowing for a clear, single-line decision-making process.
Limitations and Best Practices
While using one-liners for if-else statements can be elegant, there are some limitations and best practices to consider:
- Readability: Avoid overly complex nested ternary operators. If a condition requires multiple branches, consider using traditional if-else blocks.
- Debugging: One-liners can complicate debugging. If you encounter an error, it may be harder to pinpoint the exact cause in a single line.
- Complexity: Limit the use of one-liners to simple conditions. For intricate logic, structure your code for clarity.
Examples in Practice
Here’s a table summarizing some common uses of one-liners in Python:
Scenario | One-liner Syntax |
---|---|
Check if even or odd | `result = “Even” if x % 2 == 0 else “Odd”` |
Determine sign | `result = “Positive” if x > 0 else “Negative”` |
Get status of a person | `status = “Adult” if age >= 18 else “Minor”` |
Assign maximum value | `max_value = a if a > b else b` |
Utilizing these one-liner constructs can enhance the conciseness of your code while maintaining its functionality.
Expert Insights on One-Liner If-Else Statements in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Using one-liner if-else statements in Python, often referred to as the ternary operator, can significantly enhance code readability and conciseness. However, it is essential to use this feature judiciously to avoid sacrificing clarity for brevity.”
Michael Chen (Software Engineer, CodeCraft Solutions). “The one-liner if-else syntax in Python is a powerful tool for simplifying conditional assignments. It allows developers to express conditions succinctly, but it is crucial to ensure that the logic remains clear to anyone reading the code.”
Sarah Patel (Lead Data Scientist, Data Insights Group). “In data-driven applications, using one-liner if-else statements can streamline data processing tasks. However, I recommend maintaining a balance between succinctness and readability, especially in complex conditions.”
Frequently Asked Questions (FAQs)
What is a one-liner if-else statement in Python?
A one-liner if-else statement in Python is a concise way to perform conditional assignments or evaluations using the syntax: `value_if_true if condition else value_if_`.
How do you use a one-liner if-else in a function?
You can use a one-liner if-else within a function to return values based on conditions. For example: `def check_value(x): return “Positive” if x > 0 else “Negative or Zero”`.
Can you nest one-liner if-else statements in Python?
Yes, you can nest one-liner if-else statements. For example: `result = “Positive” if x > 0 else “Negative” if x < 0 else "Zero"`.
What are the advantages of using one-liner if-else statements?
One-liner if-else statements improve code readability and reduce the number of lines, making the code more concise for simple conditions.
Are there any limitations to using one-liner if-else statements?
One-liner if-else statements can reduce clarity when conditions are complex or when multiple statements are involved, making it harder to understand at a glance.
Is it possible to use one-liner if-else statements in list comprehensions?
Yes, one-liner if-else statements can be used in list comprehensions to create lists based on conditions, such as: `[x if x > 0 else 0 for x in my_list]`.
In Python, the ability to write conditional statements in a single line is a powerful feature that enhances code readability and conciseness. The ternary operator, often referred to as the conditional expression, allows developers to evaluate a condition and return one of two values based on the outcome. This functionality is particularly useful in scenarios where a simple decision-making process is required, enabling programmers to streamline their code without sacrificing clarity.
One of the primary advantages of using one-liner if-else statements is the reduction of code clutter. By consolidating logic into a single line, developers can create cleaner and more maintainable scripts. This approach is especially beneficial in list comprehensions and lambda functions, where brevity is essential. However, it is crucial to use this feature judiciously, as overly complex one-liners can lead to decreased readability, making the code harder to understand for others or even for the original author at a later date.
In summary, utilizing one-liner if-else statements in Python can significantly improve code efficiency and clarity when applied appropriately. Developers should strive to balance brevity with readability, ensuring that their code remains accessible and easy to follow. By mastering this technique, programmers can enhance their coding practices and contribute to the development
Author Profile
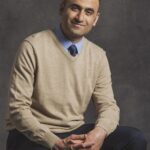
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?