How Can You Use Python to Loop Through a List in Reverse?
In the world of programming, the ability to manipulate data structures efficiently is a crucial skill, and Python, with its elegant syntax and powerful features, makes this task both accessible and enjoyable. One common scenario that often arises is the need to traverse a sequence in reverse order. Whether you’re processing a list of items, iterating through a string, or working with any iterable, knowing how to implement a reverse loop can save time and enhance your code’s readability. In this article, we’ll explore the various techniques available in Python for looping in reverse, empowering you to write cleaner and more efficient code.
When it comes to reversing a sequence in Python, there are several methods to choose from, each with its own advantages and use cases. From leveraging built-in functions to employing slicing techniques, Python offers a rich toolkit that caters to both novice and experienced programmers. Understanding these methods not only enhances your coding repertoire but also deepens your appreciation for Python’s versatility.
Moreover, looping in reverse can be particularly useful in scenarios where the order of processing is critical, such as when dealing with stacks, undo operations, or even when implementing certain algorithms. By mastering reverse loops, you will be better equipped to tackle a variety of programming challenges, making your code more efficient and effective. Join
Using the `reversed()` Function
In Python, the simplest way to iterate through a sequence in reverse is by utilizing the built-in `reversed()` function. This function returns an iterator that accesses the given sequence in the reverse order. It is particularly useful when you want to avoid modifying the original sequence.
Here’s an example of using `reversed()` in a for loop:
“`python
my_list = [1, 2, 3, 4, 5]
for item in reversed(my_list):
print(item)
“`
This will output:
“`
5
4
3
2
1
“`
The `reversed()` function can be applied to various sequence types including lists, tuples, and strings.
Slicing for Reverse Iteration
Another method to achieve reverse iteration is through slicing. By specifying a step of `-1`, you can create a reversed copy of the sequence. This approach works only with sequences that support slicing, such as lists and strings.
Example of slicing a list in reverse:
“`python
my_list = [1, 2, 3, 4, 5]
for item in my_list[::-1]:
print(item)
“`
This will produce the same output as the previous example:
“`
5
4
3
2
1
“`
Using a While Loop for Manual Reverse Iteration
In scenarios where more control is needed over the iteration process, a while loop can be employed to manually iterate in reverse. This can be useful if you need to perform additional operations during the loop.
Here’s an example:
“`python
my_list = [1, 2, 3, 4, 5]
index = len(my_list) – 1
while index >= 0:
print(my_list[index])
index -= 1
“`
Output:
“`
5
4
3
2
1
“`
Comparative Overview of Reverse Iteration Methods
The following table summarizes the different methods available for reverse iteration in Python:
Method | Description | Sequence Types | Performance |
---|---|---|---|
reversed() | Returns an iterator for reverse access. | Lists, Tuples, Strings | Efficient, no additional memory overhead. |
Slicing | Creates a new reversed list or string. | Lists, Strings | Memory-intensive for large sequences. |
While Loop | Manual control over iteration. | All sequences | Flexible but less concise. |
By understanding these methods, you can choose the most appropriate approach for reverse iteration based on your specific requirements and the type of sequence you are working with.
Reversing a For Loop in Python
Reversing a for loop in Python can be accomplished in several ways, depending on the data structure you are working with. Below are the most common methods to achieve this.
Using the `reversed()` Function
The `reversed()` function returns an iterator that accesses the given sequence in the reverse order. This method is particularly useful for lists and other iterable objects.
“`python
my_list = [1, 2, 3, 4, 5]
for item in reversed(my_list):
print(item)
“`
This code will output:
“`
5
4
3
2
1
“`
Using Slicing
Python allows slicing of lists, which can be utilized to reverse a list efficiently. The syntax for slicing is `list[start:end:step]`.
“`python
my_list = [1, 2, 3, 4, 5]
for item in my_list[::-1]:
print(item)
“`
This will yield the same output as using `reversed()`.
Using a Range with Negative Step
When working with a range of numbers, you can create a reverse loop by specifying a negative step in the `range()` function.
“`python
for i in range(5, 0, -1):
print(i)
“`
Output:
“`
5
4
3
2
1
“`
Iterating Over a Dictionary in Reverse
Dictionaries maintain insertion order as of Python 3.7. You can reverse a dictionary’s keys or items using the same techniques mentioned above.
“`python
my_dict = {‘a’: 1, ‘b’: 2, ‘c’: 3}
for key in reversed(my_dict):
print(key, my_dict[key])
“`
Output:
“`
c 3
b 2
a 1
“`
Using List Comprehensions
List comprehensions also support reverse iteration. Here’s how to create a new list in reverse order using a list comprehension.
“`python
my_list = [1, 2, 3, 4, 5]
reversed_list = [item for item in my_list[::-1]]
for item in reversed_list:
print(item)
“`
Performance Considerations
- `reversed()`: More memory efficient than slicing as it does not create a new list.
- Slicing: Simple and intuitive but creates a new list, consuming more memory.
- Negative Step in Range: Best suited for integer sequences when the range is known ahead of time.
Method | Memory Efficiency | Ease of Use | Performance |
---|---|---|---|
`reversed()` | High | Moderate | Fast |
Slicing | Low | High | Moderate |
Negative Step in Range | High | Moderate | Fast |
Using these techniques, you can effectively reverse a for loop in Python to suit the requirements of your application or script.
Reversing Iteration: Expert Insights on Python For Loops
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). In Python, reversing a for loop can be efficiently achieved using the `reversed()` function or by utilizing slicing. This approach not only enhances readability but also maintains the performance integrity of the code, especially when dealing with large datasets.
Michael Chen (Lead Python Developer, CodeCraft Solutions). When iterating in reverse with a for loop, it’s essential to consider the data structure in use. For lists, using `range(len(my_list) – 1, -1, -1)` is a common pattern. However, leveraging `reversed()` provides a more Pythonic and cleaner solution that aligns with best practices in coding.
Sarah Thompson (Data Scientist, Analytics Hub). In data manipulation tasks, reversing a for loop can be particularly useful. Utilizing constructs like `for i in range(len(data)-1, -1, -1)` allows for backward iteration, which can be advantageous in scenarios where the order of processing matters, such as when handling time series data.
Frequently Asked Questions (FAQs)
How can I iterate over a list in reverse using a for loop in Python?
You can iterate over a list in reverse by using the `reversed()` function. For example:
“`python
my_list = [1, 2, 3, 4]
for item in reversed(my_list):
print(item)
“`
Is there a way to reverse a for loop using slicing?
Yes, you can use slicing to reverse a list. For instance:
“`python
my_list = [1, 2, 3, 4]
for item in my_list[::-1]:
print(item)
“`
Can I reverse a for loop with a range in Python?
Absolutely. You can use the `range()` function with a negative step to iterate in reverse. For example:
“`python
for i in range(4, 0, -1):
print(i)
“`
What is the difference between using `reversed()` and slicing to reverse a list?
`reversed()` returns an iterator, which is more memory efficient for large lists, while slicing creates a new list in memory. Choose based on performance needs.
Are there any built-in functions to reverse a string in a for loop?
You can reverse a string using slicing within a for loop. For example:
“`python
my_string = “hello”
for char in my_string[::-1]:
print(char)
“`
Can I use a for loop in reverse with a dictionary in Python?
Yes, you can reverse the keys of a dictionary using `reversed()` on `dict.keys()`. For example:
“`python
my_dict = {1: ‘a’, 2: ‘b’, 3: ‘c’}
for key in reversed(my_dict.keys()):
print(key, my_dict[key])
“`
In Python, reversing a for loop can be achieved through various methods, allowing developers to iterate over sequences in reverse order. The most common approach is using the built-in `reversed()` function, which returns an iterator that accesses the given sequence in the reverse order. This method is efficient and straightforward, making it a preferred choice for many programmers. Additionally, using slicing with negative indices can also reverse a list or string, providing an alternative way to achieve similar results.
Another effective technique involves utilizing the `range()` function in conjunction with a specified start and end point. By setting the step parameter to a negative value, developers can create a loop that counts downwards, thereby allowing for reverse iteration. This approach is particularly useful when the range of numbers is known and can be defined explicitly. Each of these methods offers flexibility depending on the context and requirements of the task at hand.
In summary, Python provides multiple efficient ways to implement reverse for loops, including the use of `reversed()`, slicing, and the `range()` function. Understanding these methods not only enhances coding efficiency but also broadens a programmer’s toolkit for handling various scenarios. By mastering reverse iteration, developers can write cleaner, more effective code that meets their specific needs
Author Profile
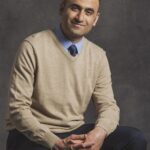
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?