Why Does My Python For Loop Only Execute the First Time?
In the world of programming, loops are a fundamental concept that allows developers to execute a block of code multiple times efficiently. Among the various types of loops available in Python, the `for` loop stands out for its simplicity and versatility. However, there are scenarios where you might want your loop to execute a specific block of code only during its first iteration. This requirement can arise in numerous applications, from initializing variables to setting up conditions that should only be established once. Understanding how to control the execution flow in a `for` loop can significantly enhance the functionality and performance of your Python programs.
When working with Python’s `for` loop, the ability to execute code conditionally based on the iteration count can be a game-changer. This technique allows developers to streamline their code and avoid unnecessary computations during subsequent iterations. By leveraging flags or counters, you can create a robust structure that ensures certain actions are taken only at the beginning of the loop’s execution. This approach not only improves readability but also optimizes performance, especially in cases where the loop iterates over large datasets or complex operations.
As we delve deeper into this topic, we will explore various methods to implement this functionality in Python. From using boolean flags to more advanced techniques, you’ll discover how to tailor
Understanding the For Loop in Python
In Python, a `for` loop is primarily used to iterate over a sequence (like a list, tuple, or string) or other iterable objects. However, if you want to ensure that a particular block of code within the loop executes only during the first iteration, you can implement a simple control mechanism.
Implementing First-Time Execution
To execute code only during the first iteration of a loop, you can use a conditional statement that checks the iteration index. Below is a straightforward approach using a counter.
“`python
items = [‘apple’, ‘banana’, ‘cherry’]
for index, item in enumerate(items):
if index == 0:
Code to run only during the first iteration
print(f’First item: {item}’)
Code to run on every iteration
print(f’Processing: {item}’)
“`
In this example, the `enumerate` function is used to keep track of the index of each item, allowing you to identify the first iteration easily.
Alternative Methods
Besides using `enumerate`, there are other methods to achieve the same goal:
- Using a Boolean Flag: You can set a flag variable that indicates whether the first iteration has occurred.
“`python
items = [‘apple’, ‘banana’, ‘cherry’]
first_iteration = True
for item in items:
if first_iteration:
print(f’First item: {item}’)
first_iteration =
print(f’Processing: {item}’)
“`
- Using a `break` Statement: If you want to execute a block only once and then break the loop, this method can be employed.
“`python
items = [‘apple’, ‘banana’, ‘cherry’]
for item in items:
print(f’First item: {item}’)
break
“`
This method is less common for iterating over all items, as it will terminate the loop after the first execution.
Comparison of Methods
The following table outlines the pros and cons of the different methods discussed:
Method | Pros | Cons |
---|---|---|
Enumerate | Simple and effective, easy to read | Requires managing index |
Boolean Flag | Clear control flow, versatile | Extra variable needed |
Break Statement | Direct and straightforward | Terminates loop, not suitable for full iteration |
By evaluating these options, you can choose the method that best fits your coding style and the specific requirements of your task. Each of these techniques allows you to control the flow of your loop effectively, ensuring that certain actions are performed only during the first iteration.
Understanding the Behavior of Python For Loops
In Python, the `for` loop iterates over a sequence (like a list, tuple, or string) and executes a block of code for each item in that sequence. However, if you want to perform an action only during the first iteration, there are several techniques you can implement.
Using a Flag Variable
One straightforward method is to use a flag variable that indicates whether the loop is executing its first iteration.
“`python
first_time = True
for item in iterable:
if first_time:
Action to execute only the first time
print(f”First item: {item}”)
first_time =
Action to execute on subsequent iterations
print(f”Current item: {item}”)
“`
This approach allows you to control when specific code executes based on the value of the `first_time` variable.
Using `enumerate` for Index Tracking
Another effective method is to use the `enumerate()` function, which provides both the index and the value from the iterable. You can then check if the index is zero to determine the first iteration.
“`python
for index, item in enumerate(iterable):
if index == 0:
Action to execute only the first time
print(f”First item: {item}”)
Action to execute on subsequent iterations
print(f”Current item: {item}”)
“`
This method eliminates the need for a separate flag variable, allowing for cleaner and more concise code.
Using a `break` Statement
In scenarios where you want to perform an action only once and then stop further processing, you can use a `break` statement after executing the code for the first item.
“`python
for item in iterable:
Action to execute only the first time
print(f”First item: {item}”)
break Exit the loop after the first iteration
“`
This method is useful when you only need to process the first item and no further iterations are necessary.
Example Scenarios
Consider the following scenarios where these techniques could apply:
Scenario | Suggested Method |
---|---|
Processing only the first item | Use a flag variable or `break` |
Logging the first item differently | Use `enumerate` |
Conditional processing based on item type | Use a flag variable |
Each method serves different needs based on how you structure your loop and what you intend to accomplish in your program.
Best Practices
When implementing a `for` loop that executes a block of code only on the first iteration, consider the following best practices:
- Clarity: Choose a method that makes your code easy to read and understand.
- Performance: Evaluate performance implications, especially with large datasets. Using `enumerate()` may introduce overhead if not needed.
- Maintainability: Ensure that your chosen method aligns with future changes, such as adding more conditions or altering loop logic.
By applying these techniques, you can efficiently control the execution of code within a Python `for` loop, ensuring that specific actions occur only during the first iteration.
Understanding Python For Loops and Their Behavior
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, if you want a for loop to execute only the first time, you can utilize a flag variable or a conditional statement that checks if the loop has already run. This approach allows for more control over the execution flow, ensuring that certain actions are performed only once.”
Michael Chen (Python Developer, CodeCraft Solutions). “The concept of executing a for loop only once can be achieved by breaking out of the loop after the first iteration. This can be done using the ‘break’ statement, which is a straightforward method to limit the loop’s execution to a single cycle.”
Lisa Patel (Data Scientist, Analytics Hub). “When designing a loop in Python that should only perform an action the first time, consider using a generator or an iterator that yields values conditionally. This not only optimizes performance but also adheres to Pythonic principles of clarity and simplicity in code.”
Frequently Asked Questions (FAQs)
How can I execute a block of code only during the first iteration of a for loop in Python?
You can use a conditional statement to check if the current iteration is the first. For example, you can utilize an index variable or a boolean flag to determine if it is the first iteration.
Is there a way to skip the first iteration of a for loop in Python?
Yes, you can use the `enumerate()` function to start the loop from the second item by checking the index. Alternatively, you can use the `next()` function to skip the first item directly.
What is the purpose of using a flag variable in a for loop?
A flag variable can help track whether a specific condition has been met, such as whether it is the first iteration. This allows you to execute certain code only when the flag indicates the first iteration.
Can I use the `break` statement to control the first iteration in a for loop?
While the `break` statement can terminate a loop, it is not typically used to control the first iteration. Instead, use it to exit the loop based on a condition after the first iteration has been executed.
How do I implement a for loop that behaves differently on the first iteration in a function?
You can define a function that includes a for loop with a counter or a flag. Check the counter or flag at the beginning of the loop to determine if it’s the first iteration, and execute the specific code accordingly.
What are some common mistakes when trying to execute code only on the first iteration of a for loop?
Common mistakes include failing to properly initialize a counter or flag, not updating the counter or flag within the loop, or using incorrect loop constructs that do not allow for easy iteration tracking.
In Python, a for loop is designed to iterate over a sequence, executing a block of code for each item in that sequence. However, there are scenarios where it may seem that the loop only executes its body the first time. This behavior typically arises from issues related to the structure of the loop, the data being iterated over, or the conditions set within the loop. Understanding these factors is crucial for effective loop implementation and debugging.
One common reason for a for loop appearing to execute only once is the use of mutable objects, such as lists, which may be modified during the iteration. If the loop alters the sequence being iterated over, it can lead to unexpected behavior. Additionally, if the loop contains a break statement or conditions that prevent further iterations, it will terminate prematurely, resulting in the impression that it only ran once.
To ensure that a for loop operates as intended, it is essential to review the loop’s logic and the data structure involved. Debugging techniques, such as printing the values at each iteration, can help clarify the loop’s behavior. By being aware of these potential pitfalls, developers can write more robust and predictable for loops in their Python code.
Author Profile
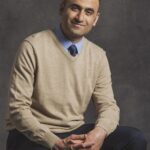
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?