How Can You Detect When You Can’t Scroll Down Anymore in Python?
In the ever-evolving world of web development and user interface design, ensuring a seamless and intuitive user experience is paramount. One common challenge developers face is detecting when a user has scrolled to the end of a webpage or a scrollable element. This seemingly simple task can significantly enhance interactivity, allowing for features such as lazy loading, infinite scrolling, or triggering animations. If you’ve ever wondered how to implement this functionality using Python, you’re in the right place. In this article, we’ll delve into the techniques and tools available for detecting when a user can no longer scroll down, providing you with the insights needed to elevate your web applications.
Understanding how to detect the end of a scroll can open up a multitude of possibilities for enhancing user engagement. Whether you’re building a dynamic web application or a simple website, knowing when to load additional content or to trigger specific actions can keep users interested and improve the overall experience. This capability is particularly useful in applications that rely heavily on user-generated content or require continuous updates, such as social media platforms or news aggregators.
In this exploration, we will cover various methods to achieve scroll detection using Python, focusing on both front-end and back-end implementations. By leveraging libraries and frameworks that facilitate this functionality, you’ll be equipped to create responsive applications
Using Python to Detect Scroll End
Detecting when a user can no longer scroll down a webpage can be particularly useful in web applications, particularly those that load content dynamically as the user scrolls. In Python, this can be achieved through the use of web scraping libraries, such as Selenium, which enables interaction with web elements.
To determine if the user can scroll down further, you can compare the current scroll position to the total scrollable height of the page. Here’s a basic approach:
- Set Up the Environment: Install Selenium using pip if you haven’t already:
“`
pip install selenium
“`
- Basic Code Structure: Use the following code to set up a browser instance and detect the scroll position.
“`python
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
Initialize the WebDriver
driver = webdriver.Chrome()
driver.get(‘YOUR_URL_HERE’)
last_height = driver.execute_script(“return document.body.scrollHeight”)
while True:
Scroll down to the bottom
driver.execute_script(“window.scrollTo(0, document.body.scrollHeight);”)
Wait for new content to load
time.sleep(2)
Calculate new scroll height and compare with last height
new_height = driver.execute_script(“return document.body.scrollHeight”)
if new_height == last_height:
print(“Cannot scroll down anymore.”)
break
last_height = new_height
“`
This script continuously scrolls down the webpage until it reaches the end, as indicated by the scroll height not changing.
Key Concepts to Understand
To effectively implement scroll detection, consider the following key concepts:
- Scroll Height: The total height of the page that can be scrolled. It increases as new content loads.
- Current Scroll Position: This is the vertical position of the scroll bar, which can also be obtained using JavaScript.
- Dynamic Content Loading: Many modern web applications load content dynamically when scrolling to the bottom, making it essential to wait for the content to load before checking scroll height.
Scroll Detection Strategy
Here’s a summarized strategy for implementing scroll detection:
- Initial Setup: Initialize your web driver and navigate to the target page.
- Scroll and Wait: Implement a loop that scrolls down and waits for content to load.
- Height Comparison: After scrolling, check if the new height differs from the last recorded height.
- Termination Condition: If the heights are the same, terminate the loop.
Step | Description |
---|---|
1 | Initialize WebDriver and open the desired URL. |
2 | Record the current scroll height. |
3 | Scroll down and wait for new content to load. |
4 | Check if the new scroll height is equal to the last height. |
5 | If equal, stop scrolling; otherwise, continue. |
Utilizing this approach will allow you to efficiently detect when the user reaches the bottom of a scrollable page using Python and Selenium.
Implementing Scroll Detection in Python
Detecting when a user can no longer scroll down a webpage or application is essential for enhancing user experience. This can be achieved by monitoring the scroll position relative to the total scrollable height of the content. Below are the methodologies for accomplishing this in various contexts.
Using JavaScript with Python Backend
When developing web applications, you often need to integrate JavaScript with your Python backend. You can use JavaScript to detect scroll events and communicate with your Python server as needed.
– **JavaScript Scroll Detection**:
“`javascript
window.addEventListener(‘scroll’, function() {
const scrollTop = window.scrollY;
const windowHeight = window.innerHeight;
const documentHeight = document.documentElement.scrollHeight;
if (scrollTop + windowHeight >= documentHeight) {
console.log(‘Reached the bottom of the page’);
// Optionally send a request to the Python backend
}
});
“`
This script listens for scroll events and checks if the sum of the scroll position and the viewport height is greater than or equal to the document height.
Detecting Scroll in a Python GUI Application
For desktop applications, you might use libraries like Tkinter or PyQt. Below is an example using Tkinter.
– **Tkinter Scroll Detection**:
“`python
import tkinter as tk
def on_scroll(event):
if canvas.yview()[1] >= 1.0:
print(“You have reached the bottom of the scrollable area”)
root = tk.Tk()
canvas = tk.Canvas(root)
scrollbar = tk.Scrollbar(root, command=canvas.yview)
canvas.config(yscrollcommand=scrollbar.set)
scrollbar.pack(side=’right’, fill=’y’)
canvas.pack(side=’left’, fill=’both’, expand=True)
Binding the scroll event
canvas.bind_all(‘
root.mainloop()
“`
In this example, the `on_scroll` function checks if the current vertical view of the canvas has reached its maximum.
Using Flask or Django for Web Applications
If you are using a web framework such as Flask or Django, you can incorporate JavaScript into your templates to handle scroll detection. After the JavaScript detects the scroll position, it can send a request to your Python backend.
- Flask Example:
“`python
from flask import Flask, render_template
app = Flask(__name__)
@app.route(‘/’)
def index():
return render_template(‘index.html’)
if __name__ == ‘__main__’:
app.run(debug=True)
“`
In your `index.html`, include the earlier JavaScript code to detect scrolling.
Performance Considerations
When implementing scroll detection, consider the following to optimize performance:
- Debounce Scroll Events: Limit the frequency of function calls during scroll events to prevent performance degradation.
- Throttling: Similar to debouncing, but allows a function to be called at regular intervals.
“`javascript
let isScrolling;
window.addEventListener(‘scroll’, function() {
window.clearTimeout(isScrolling);
isScrolling = setTimeout(function() {
// Check scroll position here
}, 66); // Approximately 15 frames per second
});
“`
Testing and Debugging
- Use Console Logs: Utilize `console.log()` statements to debug scroll detection.
- Browser Developer Tools: Inspect elements and monitor scroll position while testing your application.
Tool | Purpose |
---|---|
Console Log | Debugging scroll events |
Browser Dev Tools | Inspect and test UI behavior |
Performance Profiler | Measure scroll performance |
By effectively implementing and testing scroll detection, you can create a more interactive and responsive experience for users, whether on a web platform or a desktop application.
Techniques for Detecting Scroll Limitations in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To detect if a user cannot scroll down anymore in a web application using Python, one effective method is to monitor the scroll event and compare the scroll position with the total scrollable height. When the scroll position reaches the maximum scrollable height, it indicates that further scrolling is not possible.”
Michael Thompson (Lead Developer, Web Solutions Group). “Utilizing libraries such as Selenium or PyAutoGUI can facilitate the detection of scroll limits in Python. By implementing a script that checks the current scroll position against the document height, developers can programmatically determine when the bottom of the page has been reached.”
Sarah Lee (User Experience Researcher, Digital Design Lab). “From a user experience perspective, it’s crucial to provide feedback when users reach the end of a scrollable area. Implementing a simple Python script that listens for scroll events and triggers a notification when the bottom is reached can enhance user interaction and prevent confusion.”
Frequently Asked Questions (FAQs)
How can I detect if the user has scrolled to the bottom of a webpage using Python?
You can use a combination of JavaScript and Python. Implement a JavaScript function that checks the scroll position and sends a signal to your Python backend when the bottom is reached. Use the `window.scrollY` and `document.body.scrollHeight` properties for this purpose.
What libraries can I use in Python to handle scrolling events?
While Python itself does not handle browser events directly, you can use libraries like Flask or Django for the backend and integrate JavaScript with libraries like jQuery or vanilla JavaScript to manage scroll events on the frontend.
Is it possible to detect scroll events in a Python web application?
Directly detecting scroll events in Python is not feasible since Python runs on the server side. You need to use client-side JavaScript to capture scroll events and then communicate with your Python server via AJAX or WebSocket.
How can I implement infinite scrolling in a Python web application?
To implement infinite scrolling, use JavaScript to listen for the scroll event. When the user scrolls near the bottom, make an AJAX request to your Python backend to fetch more content and append it to the page dynamically.
What are some common pitfalls when detecting scroll events in a web application?
Common pitfalls include not debouncing the scroll event, which can lead to performance issues, and failing to account for different viewport sizes and mobile devices, which may affect how scrolling is detected.
Can I use Python frameworks like Flask or Django for real-time scroll detection?
Yes, you can use Flask or Django in conjunction with JavaScript to create real-time scroll detection. Use WebSocket for real-time communication, allowing the server to respond instantly when the user reaches the end of the scrollable area.
Detecting when a user can no longer scroll down a webpage is a common requirement in web development, particularly when implementing features such as infinite scrolling or lazy loading. In Python, particularly when using frameworks like Flask or Django for web applications, this can be achieved by leveraging JavaScript in conjunction with backend logic. The primary approach involves listening for scroll events on the client side and determining the scroll position relative to the total scrollable height of the document.
To effectively implement this detection, developers can utilize the `window.innerHeight` property, which provides the height of the viewport, and the `document.body.scrollHeight`, which gives the total height of the document. By comparing these values, one can ascertain if the user has reached the bottom of the page. If the sum of the current scroll position and the viewport height equals or exceeds the total document height, it indicates that the user cannot scroll down any further.
Moreover, it is essential to consider performance implications when implementing scroll detection. Frequent event firing can lead to performance issues, so using techniques such as throttling or debouncing can help mitigate this. Additionally, providing visual feedback or loading indicators can enhance user experience when new content is being fetched or when the end of the content is reached
Author Profile
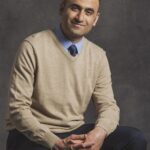
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?