How Can You Use Python’s If-Else Statement in a Single Line?
In the world of programming, efficiency and clarity are paramount, especially when it comes to writing code that is both easy to read and maintain. Python, renowned for its simplicity and elegance, offers a powerful way to streamline conditional statements through the use of single-line `if-else` expressions. This feature not only enhances the aesthetic of your code but also reduces the number of lines required to express complex logic, making your scripts more concise and readable. Whether you’re a seasoned developer or just starting your coding journey, mastering this technique can significantly improve your coding style and efficiency.
The single-line `if-else` statement in Python, often referred to as a conditional expression, allows programmers to evaluate conditions and assign values in a compact format. This powerful feature can be particularly useful in scenarios where you want to make quick decisions without the overhead of traditional multi-line structures. By leveraging this syntax, you can create cleaner and more efficient code, which is especially beneficial in data manipulation, functional programming, and when working with lists or other collections.
As we delve deeper into the mechanics of single-line `if-else` statements in Python, you’ll discover how to implement this feature effectively, explore practical examples, and understand the nuances that come with using it. Whether you’re looking to simplify your code or enhance
Using Ternary Operators in Python
In Python, a common way to implement conditional logic in a single line is through the use of the ternary operator, also known as a conditional expression. This allows you to concisely express an if-else statement without using multiple lines. The syntax for the ternary operator is as follows:
“`python
value_if_true if condition else value_if_
“`
This structure evaluates the `condition`; if it is `True`, it returns `value_if_true`, otherwise, it returns `value_if_`. This makes it particularly useful for simple conditions that can be expressed briefly.
For example:
“`python
result = “Even” if number % 2 == 0 else “Odd”
“`
In this example, the variable `result` will hold the string “Even” if `number` is even, and “Odd” if it is not.
Use Cases for One-Liner Conditionals
The ternary operator is particularly effective in scenarios where you want to assign values based on conditions without cluttering your code with multiple lines. Here are some common use cases:
- Variable Assignments: Quickly set a variable based on a condition.
- Function Return Values: Return values directly from functions based on conditions.
- List Comprehensions: Integrate conditional logic within list comprehensions for filtering or modifying lists.
Examples of Ternary Operators
Below are some practical examples demonstrating the use of ternary operators in Python:
“`python
Example 1: Basic usage
age = 18
status = “Adult” if age >= 18 else “Minor”
Example 2: In a function
def check_number(num):
return “Positive” if num > 0 else “Negative or Zero”
Example 3: List comprehension with a ternary operator
numbers = [1, -2, 3, 0]
status_list = [“Positive” if n > 0 else “Non-positive” for n in numbers]
“`
Table of Ternary Operator Syntax
The following table summarizes the syntax and usage of the ternary operator in Python:
Condition | True Result | Result | Example |
---|---|---|---|
condition | value_if_true | value_if_ | result = “Adult” if age >= 18 else “Minor” |
Limitations and Best Practices
While the ternary operator can enhance code readability when used appropriately, there are some limitations and best practices to consider:
- Readability: Avoid overly complex conditions that make the code difficult to read. If the logic is intricate, prefer traditional if-else statements.
- Nesting: Avoid nesting ternary operators, as this can lead to confusion. Instead, use if-else statements to maintain clarity.
- Performance: For performance-critical applications, be mindful that unnecessary complexity can affect maintainability more than execution speed.
By employing the ternary operator judiciously, you can write cleaner, more efficient Python code that captures the essence of conditional logic in a succinct manner.
Using Ternary Operator in Python
In Python, the ternary operator provides a concise way to write an `if-else` statement in a single line. The syntax for the ternary operator is:
“`
value_if_true if condition else value_if_
“`
This structure enables you to evaluate a condition and return a value based on that condition, allowing for clear and succinct code.
Examples of Single-Line If-Else Statements
Here are practical examples demonstrating the use of the ternary operator:
– **Basic Example**:
“`python
result = “Even” if number % 2 == 0 else “Odd”
“`
In this example, the variable `result` will hold “Even” if `number` is even, otherwise it will hold “Odd”.
– **Nested Example**:
“`python
status = “Adult” if age >= 18 else “Minor”
“`
This checks the `age` and assigns “Adult” or “Minor” based on the condition.
Use Cases for Single-Line If-Else Statements
The single-line `if-else` statement can be particularly useful in various scenarios:
- Conditional Assignments: Reduces the verbosity of code when assigning variables based on conditions.
- Returning Values: Can be utilized in return statements within functions to streamline logic.
- List Comprehensions: Enhances readability when filtering or transforming lists.
Combining Ternary Operator with List Comprehensions
The ternary operator can be effectively used within list comprehensions to create new lists based on conditions. For instance:
“`python
squared = [x**2 if x > 0 else 0 for x in numbers]
“`
This statement produces a list of squared values for positive numbers, while replacing negative numbers with zero.
Limitations of Single-Line If-Else Statements
While the ternary operator is useful, it has certain limitations:
- Readability: Overusing nested ternary operators can make code difficult to read.
- Complex Conditions: If the condition is complex, it may be better to use standard `if-else` blocks for clarity.
Best Practices for Using Ternary Operators
To maximize the effectiveness of single-line `if-else` statements, consider the following best practices:
- Keep it Simple: Use ternary operators for straightforward conditions.
- Avoid Nesting: Limit the use of nested ternary operators to maintain readability.
- Comment When Necessary: If the logic is not immediately clear, add a comment for context.
By adhering to these guidelines, you can ensure that your use of single-line `if-else` statements enhances the clarity and maintainability of your code.
Efficient Conditional Statements in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Using single-line if-else statements in Python, often referred to as ternary operators, can significantly enhance code readability and conciseness. This approach allows developers to express simple conditional logic without cluttering the codebase.”
Michael Chen (Python Instructor, Code Academy). “The syntax for a single-line if-else in Python is straightforward: `value_if_true if condition else value_if_`. This not only saves space but also encourages cleaner coding practices, especially in list comprehensions and lambda functions.”
Sarah Thompson (Data Scientist, Analytics Group). “While single-line if-else statements can simplify code, it is crucial to use them judiciously. Overusing this syntax can lead to reduced readability, especially for complex conditions. Always prioritize clarity in your code.”
Frequently Asked Questions (FAQs)
What is a single-line if-else statement in Python?
A single-line if-else statement in Python, also known as a ternary operator, allows for a concise way to evaluate a condition and return one of two values based on that condition. The syntax is `value_if_true if condition else value_if_`.
How do you write a single-line if-else statement in Python?
To write a single-line if-else statement, use the format: `result = value_if_true if condition else value_if_`. This assigns `value_if_true` to `result` if the condition is true, otherwise it assigns `value_if_`.
Can you provide an example of a single-line if-else statement?
Certainly. For instance: `status = “Adult” if age >= 18 else “Minor”` assigns “Adult” to `status` if `age` is 18 or older; otherwise, it assigns “Minor”.
Are there any limitations to using single-line if-else statements?
Yes, single-line if-else statements can reduce code readability, especially when the expressions are complex. They are best used for simple conditions and assignments.
Is it possible to nest single-line if-else statements in Python?
Yes, you can nest single-line if-else statements. For example: `result = “High” if score > 90 else “Medium” if score > 50 else “Low”` evaluates multiple conditions in a single line.
When should you avoid using single-line if-else statements?
Avoid using single-line if-else statements when the logic is complex or involves multiple conditions. In such cases, traditional multi-line if-else statements improve clarity and maintainability.
In Python, the if-else statement can be condensed into a single line using the ternary conditional operator. This operator provides a concise way to evaluate a condition and return one of two values based on whether the condition is true or . The syntax follows the format: `value_if_true if condition else value_if_`. This approach not only enhances code readability but also allows for more compact expressions in scenarios where a full if-else block would be unnecessarily verbose.
Utilizing single-line if-else statements can significantly streamline code, particularly in situations where simple decisions are made. It is especially useful in list comprehensions or when assigning values to variables based on conditions. However, developers should exercise caution; excessive use of this syntax can lead to reduced clarity, especially when the conditions or expressions become complex. Therefore, while single-line if-else statements can improve brevity, they should be used judiciously to maintain code readability.
In summary, the single-line if-else statement in Python offers an efficient way to implement conditional logic. By leveraging this feature, programmers can write cleaner and more concise code. Nevertheless, it is crucial to balance brevity with clarity to ensure that the code remains understandable to others who may read or maintain
Author Profile
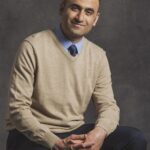
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?