How Do I Import a Module from the Same Directory in Python?
Importing Modules from the Same Directory
When working on Python projects, importing modules from the same directory is a common practice. This can be done using the standard import statement, which allows you to access functions, classes, and variables defined in other files. Here are the key steps and considerations for successfully importing modules:
Basic Import Syntax
To import a module from the same directory, use the following syntax:
“`python
import module_name
“`
Alternatively, if you want to import specific functions or classes from a module, you can use:
“`python
from module_name import function_name
“`
Example Structure
Consider the following directory structure:
“`
/project_directory
├── main.py
└── helper.py
“`
In this example, if you want to use a function defined in `helper.py` within `main.py`, follow these steps:
In `helper.py`:
“`python
def greet():
return “Hello, World!”
“`
In `main.py`:
“`python
import helper
print(helper.greet())
“`
This will output: `Hello, World!`
Handling Circular Imports
Circular imports occur when two modules attempt to import each other. This can lead to issues such as `ImportError`. Here are strategies to avoid circular imports:
- Reorganize Code: Combine related functions into a single module.
- Use Local Imports: Import modules inside functions to delay the import until necessary.
Example of a local import:
“`python
def some_function():
from helper import greet
print(greet())
“`
Using `__init__.py` for Package Structure
If your project is structured as a package, include an `__init__.py` file in your directory. This file can be empty, but its presence allows Python to treat the directory as a package. You can then use relative imports.
Directory Structure:
“`
/project_package
├── __init__.py
├── main.py
└── helper.py
“`
In this case, you can use:
“`python
from .helper import greet
“`
This notation explicitly indicates that you are importing from the same package.
Common Pitfalls
- Module Name Conflicts: Avoid naming your files with the same name as standard libraries (e.g., `math.py`).
- File Execution Context: Ensure you are running the script from the correct directory; otherwise, the imports may fail.
- PYTHONPATH: Modify the `PYTHONPATH` if you are dealing with complex directory structures to ensure Python can locate your modules.
Debugging Import Errors
If you encounter issues when importing, consider the following steps:
- Check Syntax: Verify that your import statements are correctly spelled and formatted.
- Inspect the Directory: Ensure that the target module exists in the same directory as the script attempting to import it.
- Use `print(sys.path)`: This will help you understand where Python is searching for modules.
By following these guidelines, you can effectively manage imports within the same directory, streamline your code organization, and avoid common pitfalls associated with module imports in Python.
Understanding Module Imports in Python: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When importing modules from the same directory in Python, it is crucial to ensure that the directory is recognized as a package. This can be accomplished by including an `__init__.py` file, even if it is empty, which signals to Python that the directory should be treated as a module.”
James Liu (Lead Python Developer, CodeCraft Solutions). “Utilizing relative imports can simplify the process of importing modules from the same directory. It is advisable to use the dot notation to reference sibling modules, as this enhances code readability and maintains a clear structure.”
Maria Gonzalez (Python Educator, Open Source Academy). “Understanding the Python import system is essential for effective coding practices. When working within the same directory, developers should be cautious of circular imports, which can lead to unexpected behavior and errors in the application.”
Frequently Asked Questions (FAQs)
How do I import a module from the same directory in Python?
You can import a module from the same directory by using the standard import statement. For example, if you have a file named `module.py`, you can import it using `import module`.
What if the module I want to import has a different name than the file?
You must use the exact name of the module as defined in the file, excluding the `.py` extension. For instance, if your file is named `my_module.py`, you would import it using `import my_module`.
Can I import specific functions or classes from a module in the same directory?
Yes, you can import specific functions or classes using the `from` keyword. For example, `from module import function_name` allows you to directly use `function_name` without prefixing it with the module name.
What should I do if I encounter an ImportError when importing from the same directory?
Ensure that the module name is correct and that the file exists in the same directory. Additionally, check for any circular imports or syntax errors in the module.
Is it necessary to have an `__init__.py` file in the directory for imports to work?
In Python 3.3 and later, an `__init__.py` file is not strictly required for imports to work in the same directory. However, including it can help define a package structure and avoid potential import issues in more complex scenarios.
Can I use relative imports to import modules from the same directory?
Yes, you can use relative imports by using a dot (`.`) to refer to the current package. For example, `from . import module` will import `module` from the same directory. However, this is typically used within packages.
In Python, importing modules from the same directory is a straightforward process that allows developers to organize their code effectively. When working on a project, it is common to create multiple Python files that need to interact with each other. By using the standard import statement, developers can access functions, classes, and variables defined in other files within the same directory. This promotes modularity and code reusability, which are essential principles in software development.
To import a module from the same directory, one simply uses the module name without any additional path specifications. For example, if there are two files, `module_a.py` and `module_b.py`, located in the same directory, one can import `module_a` into `module_b` using the statement `import module_a`. This eliminates the need for complex directory structures and enhances the readability of the code by keeping related modules together.
It is also important to consider the implications of the `__init__.py` file in a directory. While it is not strictly necessary for Python 3.3 and above, having an `__init__.py` file can help in defining a package, which can be beneficial for larger projects. Additionally, developers should be cautious of circular imports, which
Author Profile
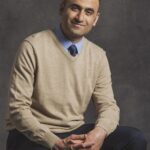
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?