How Can You Retrieve the Name of the Calling Function in Python 3?
In the world of programming, understanding the flow of execution is crucial for debugging, logging, and enhancing code readability. Python, with its dynamic nature and rich introspection capabilities, provides developers with powerful tools to navigate through the intricacies of their code. One intriguing aspect of this is the ability to retrieve the name of the calling function—a feature that can significantly aid in creating more informative logs or debugging outputs. Whether you’re building a complex application or simply refining a script, knowing how to access the calling function’s name can elevate your coding practices and provide deeper insights into your program’s execution.
When a function calls another function in Python, it creates a chain of execution that can sometimes be difficult to trace, especially in larger applications. Being able to identify the calling function can help developers understand context and flow, making it easier to diagnose issues or optimize performance. Python’s introspection capabilities allow you to tap into the call stack, providing a way to access not just the current function but also its caller. This functionality can be particularly useful in scenarios like debugging, where knowing the origin of a function call can lead to quicker resolutions.
In this article, we will explore the methods available in Python to retrieve the name of the calling function. We will delve into the built-in modules
Accessing the Calling Function’s Name
In Python, obtaining the name of the calling function can be accomplished through the `inspect` module, which provides several useful functions to get information about live objects. The `inspect.stack()` function is particularly useful for retrieving the stack frames, from which you can extract the name of the calling function.
Here’s a step-by-step breakdown of how to achieve this:
- Import the Inspect Module: First, you need to import the `inspect` module in your script.
- Use inspect.stack(): Call this function to get a list of `FrameInfo` objects, which contain information about the current call stack.
- Extract the Function Name: The calling function’s name can be found in the second frame of the stack (index 1).
Here is a code example demonstrating this approach:
“`python
import inspect
def get_caller_name():
return inspect.stack()[1].function
def caller_function():
print(“The calling function is:”, get_caller_name())
caller_function()
“`
When you run the above code, it will output:
`The calling function is: caller_function`
Understanding the Inspect Module
The `inspect` module offers various functions to interact with live object information. Below are some key features of the `inspect` module relevant to this topic:
- inspect.stack(): Returns a list of `FrameInfo` objects, representing the call stack.
- inspect.currentframe(): Returns the current stack frame.
- inspect.getframeinfo(frame): Retrieves information about a frame, such as the filename, line number, and function name.
This module can be particularly useful for debugging and logging purposes, allowing developers to trace the execution flow of their code.
Example Use Cases
There are several scenarios where knowing the calling function’s name can be beneficial:
- Debugging: Easily identify which function called another function, aiding in tracking down errors.
- Logging: Enhance log messages by including the calling function, providing better context.
- Decorators: When creating decorators, you may want to log or manipulate the behavior based on the calling function.
Here’s a simple table summarizing these use cases:
Use Case | Description |
---|---|
Debugging | Helps trace back errors to the function that initiated a call. |
Logging | Provides context in logs for better understanding of the execution flow. |
Decorators | Allows modification of function behavior based on the caller. |
Using the `inspect` module effectively can enhance code maintainability and debugging capabilities, empowering developers to create more robust Python applications.
Accessing the Calling Function’s Name in Python
To retrieve the name of the calling function in Python, the `inspect` module provides a robust solution. This module allows you to introspect live objects, including functions, and can be particularly useful for debugging or logging purposes.
Using the `inspect` Module
The `inspect` module includes the `stack()` function, which returns a list of `FrameInfo` objects representing the call stack. Each `FrameInfo` object contains information about a frame in the stack, including the function name.
“`python
import inspect
def get_calling_function_name():
return inspect.stack()[1].function
def example_function():
print(“Calling function name:”, get_calling_function_name())
example_function()
“`
In the above code:
- `inspect.stack()[1].function` retrieves the name of the function that called `get_calling_function_name()`.
- The index `[1]` indicates that we want the caller’s frame (the current function’s frame is at index `[0]`).
Example Implementation
Here’s a more detailed example demonstrating how to log the names of calling functions.
“`python
import inspect
def log_calling_function():
caller_name = inspect.stack()[1].function
print(f”Function called: {caller_name}”)
def my_function():
log_calling_function()
def another_function():
log_calling_function()
my_function() Output: Function called: my_function
another_function() Output: Function called: another_function
“`
In this example:
- The `log_calling_function()` logs the name of the function that invoked it.
- Each call to `log_calling_function()` outputs the respective calling function name.
Considerations and Best Practices
When using the `inspect` module to get the calling function’s name, consider the following:
- Performance Impact: Inspecting the call stack can be slower than direct function calls. Use it judiciously in performance-sensitive areas.
- Readability: Excessive use of stack inspection can make code harder to read and maintain.
- Debugging: This technique is particularly useful in debugging or logging scenarios where function context is crucial.
Alternative Approaches
While the `inspect` module is the most straightforward method, there are alternative approaches:
- Passing Function Names Explicitly: For better performance and clarity, consider passing function names as arguments when required.
“`python
def log(message, function_name):
print(f”{function_name}: {message}”)
def my_function():
log(“This is a log message”, “my_function”)
“`
- Decorators: You can create a decorator that wraps functions and automatically logs their names.
“`python
def log_function_call(func):
def wrapper(*args, **kwargs):
print(f”Calling function: {func.__name__}”)
return func(*args, **kwargs)
return wrapper
@log_function_call
def my_function():
pass
my_function() Output: Calling function: my_function
“`
By employing these strategies, you can effectively manage and utilize function names in your Python applications.
Understanding Function Call Context in Python 3
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). In Python 3, retrieving the name of the calling function can be effectively achieved using the `inspect` module. By utilizing `inspect.stack()`, developers can access the stack frames and identify the caller’s function name, which is crucial for debugging and logging purposes.
Michael Chen (Lead Python Developer, CodeCraft Solutions). When implementing functionality to get the name of the calling function, it is important to consider performance implications. Using `inspect` can introduce overhead, so for performance-critical applications, alternative strategies such as decorators or context managers may be more suitable.
Sarah Thompson (Python Programming Instructor, Code Academy). Teaching the concept of function call context in Python 3 is essential for novice programmers. Understanding how to retrieve the calling function’s name not only enhances their debugging skills but also deepens their comprehension of Python’s execution model and stack management.
Frequently Asked Questions (FAQs)
How can I get the name of the calling function in Python 3?
You can use the `inspect` module, specifically `inspect.stack()`, to retrieve the name of the calling function. This method provides access to the call stack, allowing you to identify the function that invoked the current function.
What is the purpose of using `inspect.stack()`?
`inspect.stack()` returns a list of `FrameInfo` objects for the current call stack. Each object contains information about the frame, including the function name, which can be used to determine the caller’s identity.
Can I retrieve the calling function’s name without using `inspect`?
While `inspect` is the most straightforward method, you can also pass the caller’s name as an argument to the function. However, this approach requires manual tracking and is less dynamic than using `inspect`.
Are there any performance implications when using `inspect.stack()`?
Yes, using `inspect.stack()` can introduce performance overhead, especially in performance-critical applications. It is advisable to use it judiciously and only when necessary.
Is it possible to get the full call stack in Python 3?
Yes, you can obtain the full call stack using `inspect.stack()` and iterate through the list of `FrameInfo` objects. This allows you to see not just the immediate caller but the entire chain of function calls.
What Python version introduced the `inspect` module?
The `inspect` module has been available since Python 2.1 and continues to be a part of Python 3.x, providing tools for introspection and stack inspection.
In Python, obtaining the name of the calling function can be achieved using the `inspect` module, which provides several useful functions to retrieve information about live objects. Specifically, the `inspect.stack()` function allows developers to access the call stack and extract details about the calling function, including its name. This capability is particularly useful for debugging, logging, or creating decorators that need to know the context in which they are invoked.
Another method to retrieve the name of the calling function is through the use of the `traceback` module. This module can also provide insights into the call stack, allowing developers to identify the function that called the current function. Using these techniques, developers can enhance the traceability of their code, making it easier to diagnose issues or understand the flow of execution.
In summary, Python offers robust mechanisms to retrieve the name of the calling function through the `inspect` and `traceback` modules. These tools empower developers to create more maintainable and debuggable code by providing context about function calls. Understanding how to leverage these modules effectively can significantly enhance the quality of Python applications.
Author Profile
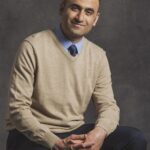
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?