How Can You Save Checkpoints Every N Epochs in PyTorch Lightning?
In the realm of deep learning, managing the training process effectively is crucial for achieving optimal model performance. One of the key strategies in this endeavor is the use of checkpoints, which allow practitioners to save the state of their models at various stages during training. This not only provides a safety net in case of interruptions but also enables the evaluation of model performance over time. For those using PyTorch Lightning, a popular high-level framework built on top of PyTorch, the ability to save checkpoints every n epochs can significantly streamline this process, ensuring that valuable training progress is not lost and that the best models can be easily retrieved.
PyTorch Lightning offers a flexible and intuitive way to implement checkpointing, allowing users to customize their training loops with minimal boilerplate code. By saving checkpoints at regular intervals, users can monitor their model’s learning curve, experiment with different hyperparameters, and revert to previous iterations if needed. This systematic approach not only enhances reproducibility but also facilitates the exploration of various training strategies without the fear of starting from scratch.
As we delve deeper into the mechanics of saving checkpoints in PyTorch Lightning, we’ll explore how to configure the checkpointing mechanism to save your model every n epochs, the benefits of this practice, and tips for managing your saved models effectively. Whether you are a
Saving Checkpoints in PyTorch Lightning
In PyTorch Lightning, managing checkpoints is crucial for model training, particularly when you want to save the model’s state at regular intervals. This allows for easy recovery from interruptions or for analyzing model performance at different training stages. PyTorch Lightning provides a straightforward mechanism for saving checkpoints every `n` epochs through its `ModelCheckpoint` callback.
ModelCheckpoint Callback Configuration
To enable checkpoint saving every `n` epochs, you need to configure the `ModelCheckpoint` callback in your Lightning model. Here’s how you can do it:
“`python
from pytorch_lightning.callbacks import ModelCheckpoint
checkpoint_callback = ModelCheckpoint(
monitor=’val_loss’, Metric to monitor
save_top_k=-1, Save all models
save_weights_only=True, Save only the weights
every_n_epochs=5 Save every 5 epochs
)
“`
Key Parameters:
- monitor: Specifies the metric you want to monitor (e.g., validation loss).
- save_top_k: Controls how many models to save based on the monitored metric. Use `-1` to save all models.
- save_weights_only: When set to `True`, only the weights are saved, which reduces the size of the checkpoint files.
- every_n_epochs: Defines how often (in epochs) you want to save the checkpoint.
Integrating Callbacks in Trainer
After defining the `ModelCheckpoint`, you need to integrate it into the `Trainer` object when you initiate training. Here’s an example:
“`python
from pytorch_lightning import Trainer
trainer = Trainer(callbacks=[checkpoint_callback], max_epochs=50)
trainer.fit(model)
“`
This setup will ensure that your model’s checkpoints are saved every 5 epochs during the training process.
Example of Checkpointing Table
The following table illustrates how the checkpointing process works based on the configuration:
Epoch | Validation Loss | Checkpoint Saved |
---|---|---|
1 | 0.75 | Yes |
2 | 0.70 | No |
3 | 0.68 | No |
4 | 0.65 | No |
5 | 0.60 | Yes |
In this example, checkpoints are saved at epochs 1 and 5, demonstrating the functionality of saving at specified intervals.
Conclusion on Checkpoint Management
Using the `ModelCheckpoint` callback in PyTorch Lightning allows for efficient and manageable model training, ensuring that you can recover your work and analyze model performance at critical intervals. Configuring the checkpointing system is a straightforward process that can significantly enhance the training workflow.
Implementing Checkpoint Saving in PyTorch Lightning
In PyTorch Lightning, saving model checkpoints at specified intervals can be efficiently managed using the `ModelCheckpoint` callback. This callback allows users to define criteria for saving model states, such as saving every n epochs.
Configuration of the ModelCheckpoint Callback
To save checkpoints every n epochs, you can configure the `ModelCheckpoint` callback during your model training setup. Here’s how to do it:
“`python
from pytorch_lightning.callbacks import ModelCheckpoint
checkpoint_callback = ModelCheckpoint(
monitor=’val_loss’, Metric to monitor
save_top_k=-1, Save all checkpoints
save_weights_only=True, Only save model weights
every_n_epochs=5, Save a checkpoint every 5 epochs
)
“`
Key Parameters
- monitor: Specifies which metric to monitor for improvements.
- save_top_k: Controls how many of the best models to save. Use `-1` to save all.
- save_weights_only: If set to `True`, only the model weights will be saved, reducing file size.
- every_n_epochs: Defines the frequency of saving checkpoints.
Integration with Trainer
Once the callback is configured, it needs to be passed to the `Trainer` object during training. Here’s an example:
“`python
from pytorch_lightning import Trainer
trainer = Trainer(
callbacks=[checkpoint_callback],
max_epochs=50, Total number of epochs for training
)
trainer.fit(model)
“`
Explanation of the Training Setup
- callbacks: This parameter accepts a list of callback objects, including the `ModelCheckpoint`.
- max_epochs: Sets the maximum number of epochs for training.
Example of Saving Checkpoints
When the training process is initiated, checkpoints will be saved according to the defined `every_n_epochs` parameter. For instance, if `every_n_epochs=5`, checkpoints will be saved at the end of the 5th, 10th, 15th epochs, and so forth.
Checkpoint File Naming
The saved checkpoint files will be automatically named based on the monitored metric and the epoch number, making it easy to identify the best performing model versions.
Loading Checkpoints
To load a specific checkpoint for further training or evaluation, you can use the following command:
“`python
model = MyModel.load_from_checkpoint(‘path/to/checkpoint.ckpt’)
“`
Notes on Loading
- Ensure that the model class definition matches the architecture used during training.
- Loading a checkpoint allows you to resume training or perform inference without needing to retrain the model from scratch.
Conclusion on Checkpoint Management
Using the `ModelCheckpoint` callback in PyTorch Lightning provides a systematic approach to managing model checkpoints during training. With configurable parameters, users can easily customize their training workflow to ensure that important model states are preserved based on specific training criteria.
Strategies for Saving Checkpoints in PyTorch Lightning
Dr. Emily Carter (Machine Learning Researcher, AI Innovations Lab). “In PyTorch Lightning, saving checkpoints every n epochs is crucial for long training sessions. It allows for recovery from interruptions and helps in evaluating model performance at various stages. Utilizing the `ModelCheckpoint` callback with the `every_n_epochs` parameter is an effective way to automate this process.”
Michael Chen (Senior Data Scientist, Tech Solutions Inc.). “Implementing checkpointing every n epochs can significantly enhance the training workflow. It not only provides a safety net against data loss but also facilitates hyperparameter tuning by allowing quick access to models trained at different intervals. I recommend setting the `save_top_k` parameter alongside to retain only the best-performing models.”
Sarah Thompson (AI Engineer, FutureTech Labs). “For practitioners using PyTorch Lightning, it’s essential to understand the balance between resource usage and checkpoint frequency. Saving checkpoints too often can lead to excessive storage use, while saving too infrequently might risk losing valuable training progress. The `ModelCheckpoint` callback is highly customizable, enabling users to define their own logic for checkpointing based on their specific needs.”
Frequently Asked Questions (FAQs)
How do I save a checkpoint every n epochs in PyTorch Lightning?
You can save a checkpoint every n epochs by using the `ModelCheckpoint` callback and setting the `period` parameter to your desired value. For example, `ModelCheckpoint(save_top_k=-1, period=n)` will save a checkpoint every n epochs.
Can I customize the checkpoint filename in PyTorch Lightning?
Yes, you can customize the checkpoint filename by using the `filename` parameter in the `ModelCheckpoint` callback. You can include variables such as epoch number and metrics in the filename format.
What happens if I set the save frequency to a very low number?
Setting the save frequency to a very low number may lead to excessive disk usage and slower training times due to frequent I/O operations. It is advisable to balance the frequency with your storage capabilities.
Is it possible to save checkpoints based on validation metrics instead of epochs?
Yes, you can save checkpoints based on validation metrics by using the `monitor` parameter in the `ModelCheckpoint` callback. This allows you to save the model only when a specified metric improves.
How can I load a specific checkpoint in PyTorch Lightning?
To load a specific checkpoint, use the `load_from_checkpoint` method of your model class, providing the path to the checkpoint file. This will restore the model’s state along with the optimizer and scheduler states.
Can I resume training from a specific checkpoint in PyTorch Lightning?
Yes, you can resume training from a specific checkpoint by passing the checkpoint file path to the `Trainer` instance using the `resume_from_checkpoint` parameter. This allows you to continue training from the last saved state.
In PyTorch Lightning, managing model checkpoints is a crucial aspect of training deep learning models efficiently. The framework offers built-in support for saving checkpoints at regular intervals, which can be configured to save every ‘n’ epochs. This feature is particularly beneficial for long training processes, allowing users to preserve the model’s state at specific milestones, thus facilitating recovery from interruptions and enabling experimentation with different training strategies.
To implement this functionality, users can leverage the `ModelCheckpoint` callback provided by PyTorch Lightning. By specifying the `every_n_epochs` parameter, one can easily control the frequency of checkpoint saving. This not only helps in tracking the model’s performance over time but also aids in selecting the best-performing model based on validation metrics. Additionally, users can customize the checkpointing behavior to save only the best models or to save all models at specified intervals, depending on their needs.
In summary, the ability to save checkpoints every ‘n’ epochs in PyTorch Lightning enhances the training workflow by ensuring that valuable model states are preserved. This capability not only aids in resuming training after interruptions but also provides flexibility in model evaluation and selection. Overall, utilizing this feature effectively can lead to more robust and efficient model development processes.
Author Profile
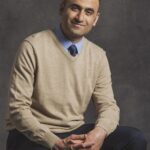
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?