How to Effectively Implement Nullable Fields in R Data Transfer Objects for Database Integration?
In the world of data management and software development, the ability to handle nullable fields in data transfer objects (DTOs) is a crucial skill. As applications grow in complexity and the need for seamless data interchange increases, developers must navigate the intricacies of nullable fields within their databases. This article delves into the nuances of writing nullable fields in R, exploring best practices, potential pitfalls, and the implications for database design. Whether you’re a seasoned developer or just starting your journey, understanding how to effectively manage nullable fields can enhance your application’s robustness and flexibility.
Nullable fields represent a significant aspect of database design, allowing for the representation of missing or data. In R, working with these fields requires a thoughtful approach to ensure that your data models accurately reflect the underlying database schema. This involves not only understanding how to define nullable fields within your DTOs but also how to effectively interact with them during data manipulation and retrieval. The interplay between R’s data structures and database constraints can lead to both opportunities and challenges, making it essential for developers to grasp the underlying principles.
As we explore the best practices for writing nullable fields in R DTOs, we will also examine the implications of these fields on data integrity and application performance. The ability to handle null values gracefully can significantly impact how your application behaves
Understanding Nullable Fields in R
In R, handling nullable fields when writing Data Transfer Objects (DTOs) for databases is crucial, especially when dealing with databases that support null values. Nullable fields allow for the representation of missing or data without causing errors during database operations.
When defining a nullable field in a DTO, it is essential to consider the data type and how it interacts with the database schema. R does not have a native concept of null in the same way some other programming languages do, but it provides mechanisms to represent missing values.
- Use `NA` to denote missing values in R.
- When defining a nullable field in a DTO, you can use the `as.nullable()` function to explicitly specify that a field can accept null values.
For example, in a DTO structure, a nullable field can be defined as follows:
“`r
my_dto <- list(
id = 1,
name = "Sample Name",
age = NA Nullable field
)
```
Mapping Nullable Fields to Database Columns
When mapping DTO fields to database columns, ensure that the database schema is designed to accept null values. This typically involves defining columns as nullable in the SQL table definition.
Here’s a comparison of how nullable fields can be represented in R and SQL:
R Data Type | Nullable Representation | SQL Data Type |
---|---|---|
Character | NA | VARCHAR NULL |
Numeric | NA | FLOAT NULL |
Logical | NA | BOOLEAN NULL |
In this table, we can see how different R data types align with their SQL counterparts. It is essential to match the nullable representation correctly, as this ensures that when data is written to the database, it conforms to the expected schema.
Writing DTOs with Nullable Fields to a Database
To write DTOs with nullable fields to a database, utilize an ORM (Object-Relational Mapping) package in R, such as `DBI` or `dplyr`. These packages provide functions that help manage the insertion of data while respecting nullable constraints.
Example of inserting a DTO into a database:
“`r
library(DBI)
con <- dbConnect(RSQLite::SQLite(), dbname = "my_database.sqlite") Create a sample table with a nullable field dbExecute(con, "CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, name TEXT, age INTEGER NULL)") Insert a DTO with a nullable field dbExecute(con, "INSERT INTO users (name, age) VALUES (?, ?)", params = list(my_dto$name, my_dto$age)) ``` In this example, the `dbExecute` function allows for parameterized queries, ensuring that `NA` values are correctly interpreted as NULL in the SQL command. This is crucial for maintaining data integrity and avoiding errors when inserting records with nullable fields.
Understanding Nullable Fields in R
In R, a nullable field indicates that a variable can hold a value or be absent (null). This is particularly useful when dealing with databases where certain fields may not always have data. In R, you can represent nullable fields using the `NA` value.
Creating a Data Transfer Object (DTO)
A Data Transfer Object (DTO) is a design pattern used to transfer data between software application subsystems or layers. When creating a DTO in R that accommodates nullable fields, you should define your data structure accordingly.
- Use a list or data frame to create the DTO.
- Specify fields that can hold `NA` values.
Example of a simple DTO in R:
“`r
create_dto <- function(name, age, email = NA) {
dto <- list(
Name = name,
Age = age,
Email = email
)
return(dto)
}
```
In the above example, the `Email` field is nullable.
Writing Nullable Fields to a Database
When writing DTOs with nullable fields to a database, you need to ensure that the database schema accommodates null values. Most databases support nullable fields, but the way you insert them may vary.
- Database Connection: Use libraries such as `DBI` and `RMySQL`, or `RSQLite` to connect to your database.
- Insert Statement: Prepare your SQL insert statement to include conditions for nullable fields.
Example of inserting a DTO into a database:
“`r
library(DBI)
Assuming you have a connection object ‘conn’
insert_dto <- function(conn, dto) {
query <- sprintf("INSERT INTO users (name, age, email) VALUES ('%s', %d, %s)",
dto$Name,
dto$Age,
ifelse(is.na(dto$Email), "NULL", sprintf("'%s'", dto$Email)))
dbExecute(conn, query)
}
```
In this example, if the `Email` field is `NA`, the SQL query inserts `NULL`.
Best Practices for Handling Nullable Fields
When working with nullable fields in R DTOs and databases, consider the following best practices:
- Validation: Always validate the data before insertion to avoid errors.
- Clear Schema Design: Ensure your database schema clearly defines which fields can be null.
- Use Consistent Naming: Maintain consistent naming conventions for nullable fields across your application.
- Error Handling: Implement error handling to manage potential database insert failures.
Best Practice | Description |
---|---|
Validation | Check data integrity before database operations. |
Clear Schema Design | Define which fields are nullable in your schema. |
Consistent Naming | Use uniform naming for nullable fields. |
Error Handling | Manage errors gracefully during database interactions. |
By following these practices, you can effectively manage nullable fields in R DTOs and ensure smooth interactions with your database.
Expert Insights on Implementing Nullable Fields in R Data Transfer Objects for Databases
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “Incorporating nullable fields in R data transfer objects (DTOs) is crucial for accurately representing optional data in a database. It allows for greater flexibility in data modeling, ensuring that applications can handle incomplete datasets without compromising integrity.”
Michael Thompson (Senior Database Architect, Cloud Solutions Group). “When designing DTOs in R, it is essential to consider how nullable fields interact with the underlying database schema. Properly defining these fields can prevent runtime errors and facilitate smoother data migrations.”
Lisa Patel (Software Engineer, Data Systems Corp.). “Utilizing nullable fields in R DTOs not only enhances data representation but also improves the performance of database queries. By allowing fields to be null, we can optimize storage and retrieval processes, ultimately leading to more efficient applications.”
Frequently Asked Questions (FAQs)
What is a nullable field in a database?
A nullable field in a database is a column that can store a NULL value, indicating the absence of data. This allows for more flexible data entry, accommodating situations where information may not be applicable or available.
How do I define a nullable field in an R data transfer object (DTO)?
To define a nullable field in an R DTO, you can use the `NA` value for numeric or character types. For example, you can declare a field as `field_name = NA` to signify that it can accept NULL values.
What are the benefits of using nullable fields in a database?
Nullable fields provide the advantage of representing optional data without enforcing a value. This can lead to more accurate data modeling and storage efficiency, reducing unnecessary placeholder values.
Can I perform operations on nullable fields in R?
Yes, you can perform operations on nullable fields in R. However, you must handle potential NA values appropriately to avoid errors. Functions like `is.na()` can help check for NULL values before proceeding with calculations.
How does a nullable field affect data integrity in a database?
Nullable fields can impact data integrity by allowing for incomplete records. It is essential to implement validation rules and constraints to ensure that the presence of NULL values does not compromise the overall quality of the data.
What considerations should I keep in mind when using nullable fields in a DTO?
When using nullable fields in a DTO, consider the implications for data validation, serialization, and deserialization processes. Ensure that your application logic can appropriately handle NULL values to maintain data consistency and integrity.
In the context of R programming, the concept of writing nullable fields in a Data Transfer Object (DTO) for database interactions is essential for ensuring flexibility and robustness in data handling. Nullable fields allow for the representation of optional data, which is particularly useful in scenarios where certain attributes may not always have a value. This capability is crucial for maintaining data integrity and accommodating various data states when interfacing with databases.
When implementing nullable fields in DTOs, it is important to consider the data types and how they are represented in both R and the target database. R provides several ways to handle missing or null values, such as using NA for numeric and character types. Additionally, the choice of database schema should align with the DTO design to ensure seamless data mapping and retrieval. Properly managing these nullable fields can significantly enhance the efficiency of data operations and reduce the likelihood of errors during data processing.
Furthermore, leveraging nullable fields in DTOs can lead to improved application performance by minimizing unnecessary data storage and optimizing query execution. It is also beneficial for API design, where optional parameters can streamline communication between systems. Developers should adopt best practices in defining and using nullable fields to ensure that their applications remain maintainable and scalable in the long run.
Author Profile
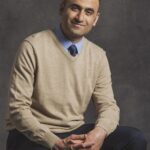
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?