How Can I Redirect Users in React When Their Token Has Expired?
In the dynamic world of web development, managing user authentication and session validity is crucial for ensuring a seamless user experience. One common challenge developers face is handling expired tokens in applications built with React. When a user’s authentication token expires, it can lead to frustrating scenarios where users are left stranded on a page, unable to access vital features or content. Understanding how to effectively redirect users in such situations not only enhances usability but also fortifies the security of your application.
As you navigate through the intricacies of React, you’ll discover that managing token expiration involves a combination of state management, lifecycle methods, and routing strategies. The key lies in detecting when a token has become invalid and implementing a smooth redirect process that guides users back to the login page or a designated area of your application. This ensures that users are always aware of their session status and can take necessary actions without confusion.
In this article, we will delve into the best practices for handling expired tokens in React applications. We’ll explore various approaches to check token validity, manage user state, and implement effective redirection strategies. Whether you’re building a simple application or a complex system, mastering these techniques will empower you to create a more robust and user-friendly experience. Get ready to enhance your React skills as we uncover the essential steps to manage token
Understanding Token Expiration
When working with authentication tokens, especially JSON Web Tokens (JWT), it is essential to handle token expiration effectively. An expired token can lead to unauthorized access attempts or users being left in a state of uncertainty about their session’s validity. Typically, tokens have a defined lifespan, after which they are considered invalid.
To manage token expiration, the following strategies can be employed:
- Set a reasonable expiration time: Define how long the token should be valid based on the application requirements. Common practices include 15 minutes for access tokens and longer for refresh tokens.
- Implement token refreshing: Use refresh tokens to obtain new access tokens without requiring the user to log in again, thereby enhancing the user experience.
- Grace period: Allow a brief grace period after expiration during which the token can still be used to minimize disruptions.
Redirecting on Expired Tokens
To redirect users when their authentication token has expired, a systematic approach is needed. This often involves middleware or hooks in your React application. The general flow includes checking the token’s validity before allowing access to protected routes.
Here’s how to implement this:
- **Check Token Validity**: Before rendering a component or accessing a route, validate the token. This can be done through a utility function that decodes the JWT and checks the expiration time.
- **Use React Router for Redirection**: Leverage React Router’s `useNavigate` hook to redirect users when their token is found to be expired.
- **Centralized Authentication Logic**: Encapsulate the logic for checking token validity in a higher-order component (HOC) or a custom hook to ensure consistency throughout the application.
Here’s an example of how this can be structured:
“`javascript
import { useNavigate } from ‘react-router-dom’;
import { useEffect } from ‘react’;
import jwt_decode from ‘jwt-decode’;
const useAuthRedirect = (token) => {
const navigate = useNavigate();
useEffect(() => {
if (token) {
const decodedToken = jwt_decode(token);
const isExpired = decodedToken.exp * 1000 < Date.now();
if (isExpired) {
// Redirect to login page or any desired route
navigate('/login');
}
}
}, [token, navigate]);
};
```
Token Expiration Handling Table
Here is a table summarizing the key points regarding token expiration handling:
Strategy | Description |
---|---|
Set Expiration Time | Define how long tokens are valid based on application needs. |
Refresh Tokens | Allow obtaining new access tokens without user re-login. |
Grace Period | A brief time after expiration during which tokens may still be valid. |
Redirect Logic | Implement checks and navigation to login on token expiration. |
By implementing these strategies and utilizing the provided code snippet, React applications can efficiently manage token expiration and enhance user experience through seamless redirection when necessary.
Checking Token Expiration
To redirect users based on token expiration, you first need a method to check the validity of the token. This typically involves decoding the token and inspecting its expiration claim. Here’s how you can implement this in a React application:
- **Decode the Token**: Use a library such as `jwt-decode` to decode the JWT and check the expiration date.
- **Compare Expiration Time**: Compare the current time with the expiration time obtained from the token.
“`javascript
import jwtDecode from ‘jwt-decode’;
const isTokenExpired = (token) => {
if (!token) return true;
const decoded = jwtDecode(token);
return decoded.exp * 1000 < Date.now(); // Convert exp to milliseconds
};
```
Redirecting Users
Once you have a function to determine if the token is expired, you can implement redirection in your React components. This can be done within a `useEffect` hook or a higher-order component (HOC) to manage the redirection logic.
– **Using `useEffect`**: This is effective for component-level checks.
“`javascript
import { useEffect } from ‘react’;
import { useHistory } from ‘react-router-dom’;
const ProtectedRoute = ({ children }) => {
const history = useHistory();
const token = localStorage.getItem(‘token’);
useEffect(() => {
if (isTokenExpired(token)) {
history.push(‘/login’); // Redirect to login if the token is expired
}
}, [token, history]);
return children; // Render children if token is valid
};
“`
– **Using Higher-Order Components (HOC)**: This can be applied to any component that requires token validation.
“`javascript
const withAuthRedirect = (WrappedComponent) => {
return (props) => {
const token = localStorage.getItem(‘token’);
if (isTokenExpired(token)) {
return
}
return
};
};
“`
Implementing in Routes
You can use the above logic in your routing setup. For example, you can create a protected route that utilizes the `ProtectedRoute` component.
“`javascript
import { BrowserRouter as Router, Route, Switch } from ‘react-router-dom’;
const App = () => {
return (
);
};
“`
Handling Token Refresh
In cases where you want to automatically refresh tokens instead of redirecting users, consider implementing a refresh token strategy. Here’s a brief outline of steps:
– **Store Refresh Token**: Store a refresh token securely, usually in cookies.
– **Intercept Requests**: Use an Axios interceptor to check for token expiration before making API calls.
– **Refresh Token Logic**: Upon detecting an expired token, make a request to refresh it.
“`javascript
import axios from ‘axios’;
axios.interceptors.request.use(async (config) => {
const token = localStorage.getItem(‘token’);
if (isTokenExpired(token)) {
const response = await axios.post(‘/api/refresh-token’, { /* refresh token data */ });
localStorage.setItem(‘token’, response.data.token);
}
config.headers[‘Authorization’] = `Bearer ${localStorage.getItem(‘token’)}`;
return config;
});
“`
This approach ensures a smoother user experience by minimizing interruptions caused by token expiration.
Strategies for Handling Token Expiration in React Applications
Dr. Emily Carter (Senior Software Engineer, SecureTech Solutions). “Implementing a middleware to check token validity before every API call is essential. If the token is expired, redirecting the user to the login page ensures a seamless experience while maintaining security protocols.”
Michael Tran (Lead Developer, React Innovations). “Utilizing React Router’s hooks to handle redirects based on token expiration can enhance user experience. A custom hook that checks the token’s status can trigger a redirect when necessary, keeping the application responsive and user-friendly.”
Sarah Patel (Full Stack Developer, CodeGuardians). “It’s crucial to store token expiration information securely. By leveraging local storage and setting up an interval to check token validity, developers can proactively redirect users when their session is about to expire, preventing unexpected logouts.”
Frequently Asked Questions (FAQs)
How can I check if a token is expired in React?
You can check if a token is expired by decoding it and verifying the `exp` claim against the current time. Use libraries like `jwt-decode` to decode the token and compare the expiration time with the current timestamp.
What should I do if the token is expired?
If the token is expired, you should redirect the user to the login page or refresh the token if your application supports token refreshing. This ensures that users can re-authenticate and regain access to protected resources.
How do I implement a redirect in React when the token is expired?
You can implement a redirect using React Router. In your component, check the token’s validity, and if it is expired, use the `useHistory` hook or the `Navigate` component to redirect the user to the login page.
Can I use a higher-order component (HOC) for token validation?
Yes, you can create a higher-order component that wraps around protected components. This HOC can check the token’s validity and perform the redirect if the token is expired, streamlining the authentication process across your application.
What is the best practice for handling token expiration in a React app?
The best practice involves implementing a centralized authentication context or state management solution. This allows you to manage token expiration checks and redirects consistently across your application, enhancing user experience and security.
How can I notify users when their session has expired?
You can use a modal or toast notification to inform users that their session has expired. This can be triggered when the application detects an expired token, prompting users to log in again for continued access.
In the context of React applications, handling token expiration is crucial for maintaining secure user sessions. When a token expires, it is essential to implement a redirect mechanism that guides users back to the login page or prompts them to refresh their session. This process typically involves checking the validity of the token before making API calls or rendering protected routes. If the token is found to be expired, the application should trigger a redirect to ensure users cannot access restricted content without proper authentication.
Implementing a robust token expiration check can be achieved through various methods, such as using middleware in a Redux store or leveraging React Router’s route guards. By centralizing the token validation logic, developers can ensure a consistent user experience across the application. Additionally, utilizing libraries like Axios for API requests can simplify the process of intercepting responses to handle token expiration gracefully.
Key takeaways include the importance of proactively managing authentication states within a React application. Developers should not only focus on the initial login process but also consider how to handle scenarios where the token may expire during a user’s session. By establishing a clear redirect strategy, applications can enhance security and improve user experience, thereby fostering greater trust and satisfaction among users.
Author Profile
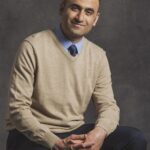
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?