How Can You Get the Current Route in React Router?
In the dynamic world of web development, React has emerged as a powerful library for building user interfaces, particularly for single-page applications (SPAs). One of the key features that enhances the user experience in these applications is routing. With React Router, developers can create seamless navigation between different components and views, allowing users to explore content without the need for full-page reloads. However, as applications grow in complexity, understanding how to effectively manage and retrieve the current route becomes crucial. This article delves into the intricacies of React Router and provides insights on how to get the current route, ensuring that your application remains intuitive and user-friendly.
Navigating through a React application often involves multiple routes, each corresponding to different components or pages. React Router simplifies this process, but it can also introduce challenges when it comes to tracking the active route. Knowing how to access the current route is essential for various functionalities, such as displaying breadcrumbs, managing active links, or implementing conditional rendering based on the route.
In this exploration, we will uncover the methods and tools available within React Router that allow developers to efficiently retrieve the current route. From leveraging hooks to utilizing higher-order components, we will provide a comprehensive overview of the techniques that can enhance your routing strategy. Whether you are a seasoned developer or
Accessing the Current Route in React Router
To get the current route in React Router, developers can utilize the `useLocation` hook provided by the library. This hook returns the current location object, which includes properties such as `pathname`, `search`, and `hash`.
Here’s how you can implement it:
“`javascript
import React from ‘react’;
import { useLocation } from ‘react-router-dom’;
const CurrentRoute = () => {
const location = useLocation();
return (
Current Route Information
Pathname: {location.pathname}
Search Query: {location.search}
Hash: {location.hash}
);
};
“`
This component displays the current route’s pathname, search parameters, and hash fragment, enabling developers to access all relevant information about the current route.
Using the `useRoutes` Hook
The `useRoutes` hook is another powerful feature of React Router that allows developers to define and manage routes declaratively. This hook can be combined with `useLocation` to dynamically render components based on the current route.
Here’s an example:
“`javascript
import { useRoutes, useLocation } from ‘react-router-dom’;
const AppRoutes = () => {
const location = useLocation();
const routes = [
{ path: ‘/’, element:
{ path: ‘/about’, element:
{ path: ‘/contact’, element:
];
const element = useRoutes(routes);
return (
Current Path: {location.pathname}
);
};
“`
In this example, the current path is displayed alongside the rendered route component, providing a seamless integration of routing and location tracking.
Accessing Route Parameters
When working with dynamic routes, it may be necessary to access route parameters. This can be done using the `useParams` hook. This hook returns an object containing key-value pairs of the parameters defined in the route.
For example, consider a route defined as `/user/:id`:
“`javascript
import { useParams } from ‘react-router-dom’;
const UserProfile = () => {
const { id } = useParams();
return
User ID: {id}
;
};
“`
This component will display the user ID extracted from the route, allowing for dynamic content rendering based on the route parameters.
Comparison of Hooks for Route Management
A summary of the primary hooks used for route management in React Router is provided in the table below:
Hook | Purpose | Returns |
---|---|---|
useLocation | Access current location object | Location object (pathname, search, hash) |
useParams | Access URL parameters | Object of route parameters |
useRoutes | Render routes declaratively | Element representing the matched route |
Utilizing these hooks effectively allows for comprehensive route management and dynamic content rendering based on the application’s navigation state.
Accessing the Current Route in React Router
To retrieve the current route in React Router, developers can utilize the `useLocation` and `useParams` hooks. These hooks provide access to the current route’s location and parameters, facilitating dynamic rendering based on the route.
Using the useLocation Hook
The `useLocation` hook is essential for obtaining the current location object, which includes properties such as pathname, search, and hash. Here’s how to implement it:
“`javascript
import { useLocation } from ‘react-router-dom’;
function CurrentRoute() {
const location = useLocation();
return (
Current Route:
Pathname: {location.pathname}
Search: {location.search}
Hash: {location.hash}
);
}
“`
Key properties of the `location` object:
- pathname: The path of the current URL.
- search: The query string, including the leading `?`.
- hash: A string representing the anchor part of the URL.
Using the useParams Hook
For routes with dynamic segments, the `useParams` hook is invaluable. It allows developers to extract parameters from the current route. For example:
“`javascript
import { useParams } from ‘react-router-dom’;
function User() {
const { userId } = useParams();
return (
User ID:
{userId}
);
}
“`
In this case, if the route is defined as `/user/:userId`, the `userId` parameter will be available through `useParams`.
Combining Hooks for Complete Route Information
Developers can combine `useLocation` and `useParams` to get a comprehensive view of the current route. Here’s an integrated example:
“`javascript
import { useLocation, useParams } from ‘react-router-dom’;
function CurrentRouteDetails() {
const location = useLocation();
const params = useParams();
return (
Current Route Details:
Pathname: {location.pathname}
Search: {location.search}
Hash: {location.hash}
Parameters: {JSON.stringify(params)}
);
}
“`
This approach provides a complete overview of the route, making it easier to manage routing logic and display dynamic content based on the user’s navigation.
Listening to Route Changes
To respond to route changes, developers can leverage the `useEffect` hook alongside `useLocation`. This allows for side effects to trigger when the route changes:
“`javascript
import { useEffect } from ‘react’;
import { useLocation } from ‘react-router-dom’;
function RouteChangeTracker() {
const location = useLocation();
useEffect(() => {
console.log(‘Route changed to:’, location.pathname);
}, [location]);
return null;
}
“`
This component logs the current route to the console whenever it changes, providing a way to track navigation for analytics or other purposes.
By utilizing the `useLocation` and `useParams` hooks, developers can efficiently access and manage the current route in React Router, facilitating responsive and dynamic user experiences.
Understanding Current Routes in React Router
Emily Carter (Senior Frontend Developer, CodeCraft Solutions). “To effectively retrieve the current route in React Router, developers should utilize the `useLocation` hook. This hook provides access to the current location object, enabling them to extract pathname and search parameters seamlessly.”
Michael Chen (React Specialist, WebDev Insights). “In React Router v6 and above, the `useMatch` hook can be particularly useful for determining if the current route matches a specific path. This allows for more dynamic rendering based on the current route, enhancing user experience.”
Sarah Thompson (Full Stack Engineer, Tech Innovators). “For applications that require route tracking, implementing a custom hook that leverages both `useLocation` and `useEffect` can provide a powerful solution. This approach allows developers to respond to route changes effectively, ensuring that the application state remains in sync with the URL.”
Frequently Asked Questions (FAQs)
How can I get the current route in React Router?
You can use the `useLocation` hook provided by React Router to access the current route. This hook returns the current location object, which contains the pathname, search, and state properties.
What is the purpose of the `useParams` hook?
The `useParams` hook allows you to access the dynamic parameters of the current route. It returns an object of key-value pairs where the keys correspond to the parameter names defined in the route path.
Can I access the current route information in a class component?
Yes, you can access the current route information in a class component by utilizing the `withRouter` higher-order component. This wraps your component and provides it with the routing props, including location, history, and match.
How do I get the current route’s query parameters?
You can retrieve the current route’s query parameters using the `useLocation` hook. The `search` property of the location object can be parsed using the `URLSearchParams` API to extract individual query parameters.
Is it possible to listen for route changes in React Router?
Yes, you can listen for route changes by using the `useEffect` hook in conjunction with the `useLocation` hook. By setting up an effect that depends on the location object, you can execute code whenever the route changes.
What is the difference between `useLocation` and `useHistory`?
`useLocation` provides access to the current location object, allowing you to know where you are in the app. In contrast, `useHistory` gives you access to the history instance, enabling you to programmatically navigate or manipulate the browser history.
In React Router, obtaining the current route is essential for managing navigation and rendering components based on the active path. The library provides several methods to access route information, including the use of hooks such as `useLocation`, `useParams`, and `useRouteMatch`. These hooks allow developers to retrieve the current URL, parameters, and match information, facilitating dynamic rendering and state management in applications.
Utilizing `useLocation` is particularly effective for tracking the current route, as it returns an object containing the pathname, search string, and hash, which can be leveraged to conditionally render components or update application state. Additionally, `useParams` is invaluable for extracting URL parameters, enabling developers to create more interactive and user-specific experiences. Understanding these tools is crucial for building robust applications that respond intuitively to user navigation.
In summary, mastering the methods provided by React Router to get the current route enhances the development process by allowing for more responsive and dynamic applications. By effectively implementing these hooks, developers can ensure a seamless user experience while maintaining clean and maintainable code. This knowledge is not only beneficial for current projects but also serves as a foundation for future enhancements and features within React applications.
Author Profile
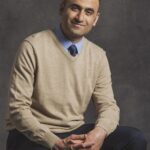
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?