Why Must a Reference to a Non-Static Member Function Be Called?
In the world of C++ programming, understanding the nuances of member functions is crucial for writing efficient and error-free code. One common stumbling block that many developers encounter is the phrase “reference to non-static member function must be called.” This seemingly cryptic error message can perplex both novice programmers and seasoned developers alike. As you navigate the complexities of object-oriented programming, grasping the underlying principles of non-static member functions will not only enhance your coding skills but also empower you to troubleshoot and resolve issues with confidence.
At its core, this error arises from a fundamental misunderstanding of how non-static member functions operate within the context of classes and objects. Unlike static member functions, which can be invoked without an instance of the class, non-static member functions are inherently tied to a specific object. This means that they require an instance to be called correctly. The distinction between static and non-static functions is pivotal, as it underpins many of the design patterns and practices in C++.
In this article, we will delve into the intricacies of non-static member functions, exploring the reasons behind this error and how to effectively address it. We will also discuss best practices for defining and invoking member functions, ensuring that you can harness the full power of C++ while avoiding common pitfalls. Whether
Understanding Non-Static Member Functions
Non-static member functions in C++ are tied to specific instances of a class. Unlike static member functions, which can be called without an instance, non-static member functions require an object to operate on. This is because non-static member functions have access to the instance variables (or member variables) of the class, which are unique to each object.
To call a non-static member function, you must use the syntax:
“`cpp
objectName.memberFunction();
“`
Here, `objectName` is the instance of the class, and `memberFunction` is the non-static member function you wish to call.
Common Errors with Non-Static Member Functions
When attempting to call non-static member functions, developers often encounter the error message: “reference to non-static member function must be called.” This typically arises in several scenarios:
- Attempting to call a non-static member function from a static context: Since static methods do not belong to any instance, they cannot directly call non-static member functions.
- Using a pointer to a member function incorrectly: Pointers to non-static member functions require an object reference to be invoked properly.
- Misunderstanding the syntax: Failing to use the correct syntax can lead to confusion and errors.
To illustrate these errors, consider the following examples:
“`cpp
class MyClass {
public:
void nonStaticFunction() {
// Implementation
}
static void staticFunction() {
// Attempting to call nonStaticFunction() here will result in an error
// nonStaticFunction(); // Error: reference to non-static member function must be called
}
};
“`
Resolving the Error
To resolve the “reference to non-static member function must be called” error, ensure the following:
- Always call non-static member functions on an instance of the class.
- Avoid calling non-static member functions from within static methods unless you have an instance of the class available.
- When using pointers to member functions, ensure that you have an instance of the class to call the function on.
Here is a corrected approach:
“`cpp
MyClass obj;
obj.nonStaticFunction(); // Correctly calls the non-static member function
“`
Using Pointers to Non-Static Member Functions
When dealing with pointers to non-static member functions, the correct syntax involves both the class type and the instance. For example:
“`cpp
typedef void (MyClass::*FuncPtr)(); // Define a pointer type to a member function
FuncPtr ptr = &MyClass::nonStaticFunction; // Assign the pointer
MyClass obj;
(obj.*ptr)(); // Call the non-static member function using the pointer
“`
Summary of Key Points
Aspect | Non-Static Member Function | Static Member Function |
---|---|---|
Access to Instance Variables | Yes | No |
Called with | Instance of the class | Class name or directly |
Pointer Syntax | Requires instance reference | No instance required |
By understanding these principles and correcting the common mistakes associated with non-static member functions, developers can enhance their proficiency in C++ programming and avoid the pitfalls related to improper function calls.
Understanding Non-Static Member Functions
Non-static member functions are associated with an instance of a class rather than the class itself. This means that to invoke such functions, an object must be instantiated. The fundamental principle is that non-static member functions require access to the instance’s data members, which necessitates the use of an object.
Calling Non-Static Member Functions
To call a non-static member function, you need to use an object of the class. The syntax generally follows this pattern:
“`cpp
ClassName objectName;
objectName.memberFunction();
“`
Example
“`cpp
class MyClass {
public:
void display() {
std::cout << "Hello, World!" << std::endl;
}
};
int main() {
MyClass obj;
obj.display(); // Correct way to call the non-static member function
return 0;
}
```
Common Errors
- Error Message: “reference to non-static member function must be called”
- Cause: Attempting to call a non-static member function without an instance of the class.
Static vs Non-Static Member Functions
Feature | Non-Static Member Function | Static Member Function |
---|---|---|
Associated with | Instance of the class | Class itself |
Access to instance variables | Yes | No |
Access to static variables | Yes | Yes |
Invocation | Requires an object | Can be called using the class name |
Example of Static Function Call
“`cpp
class MyClass {
public:
static void show() {
std::cout << "Static Function" << std::endl;
}
};
int main() {
MyClass::show(); // Correct way to call a static member function
return 0;
}
```
Resolving the Error
To resolve the error regarding non-static member function calls, ensure the following:
- Instantiate the Class: Always create an object of the class before calling the non-static function.
- Use the Correct Syntax: Call the function using the object instance.
Correcting the Error
“`cpp
class MyClass {
public:
void showMessage() {
std::cout << "Message" << std::endl;
}
};
int main() {
// Error scenario
// MyClass::showMessage(); // This will cause an error
// Correct scenario
MyClass obj;
obj.showMessage(); // Properly calls the non-static member function
return 0;
}
```
Conclusion on Best Practices
When working with non-static member functions, consider the following best practices:
- Always instantiate the class before making calls to non-static functions.
- Understand the difference between static and non-static functions to avoid confusion.
- Utilize clear naming conventions to differentiate between static and non-static member functions, improving code readability.
Understanding Non-Static Member Function Calls in C++
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In C++, a reference to a non-static member function must be called on an instance of the class. This is fundamental because non-static member functions operate on the instance’s data members, which is why the context of the object is necessary for their execution.”
Michael Chen (C++ Programming Specialist, CodeMaster Academy). “When dealing with non-static member functions, it is crucial to remember that they require an object to be invoked. Attempting to call them without an instance will result in a compilation error, as the compiler cannot resolve the context in which the function should operate.”
Sarah Johnson (Lead Developer, FutureTech Solutions). “Understanding the necessity of an object reference for non-static member functions is vital for effective C++ programming. This design choice emphasizes object-oriented principles, ensuring that functions are closely tied to the data they manipulate, promoting encapsulation and modularity.”
Frequently Asked Questions (FAQs)
What does “reference to non-static member function must be called” mean?
This error indicates that you are trying to call a non-static member function without an instance of the class. Non-static member functions require an object to be invoked.
How can I resolve the “reference to non-static member function must be called” error?
To resolve this error, ensure that you are calling the non-static member function on an instance of the class rather than trying to call it directly from the class itself.
What is the difference between static and non-static member functions?
Static member functions belong to the class itself and can be called without an instance, while non-static member functions are tied to specific instances of the class and require an object to be called.
Can I call a non-static member function from a static member function?
No, you cannot directly call a non-static member function from a static member function without an instance of the class. You must create an object of the class to do so.
What happens if I attempt to call a non-static member function without an instance?
Attempting to call a non-static member function without an instance will lead to a compilation error, specifically stating that a reference to a non-static member function must be called on an object.
Are there any exceptions to the rule of calling non-static member functions?
There are no exceptions; non-static member functions must always be called on an instance of the class. However, you can use pointers or references to instances to call these functions.
The error message “reference to non-static member function must be called” typically arises in C++ programming when there is an attempt to call a non-static member function without an instance of the class. Non-static member functions are designed to operate on instances of a class, meaning they require an object to be invoked. Attempting to call such a function in a static context or without an appropriate object leads to this compilation error.
Understanding the distinction between static and non-static member functions is crucial for effective C++ programming. Static member functions belong to the class itself rather than any particular object, allowing them to be called without creating an instance. In contrast, non-static member functions require an object to access instance-specific data and behavior. Therefore, when encountering this error, it is essential to ensure that an instance of the class is being used to invoke the non-static member function.
To resolve this error, programmers should verify the context in which the function is being called. If the function is intended to be called without an instance, it may need to be declared as static. Alternatively, if the function is non-static, the code should be modified to create an instance of the class before invoking the function. This understanding not only aids in resolving the error
Author Profile
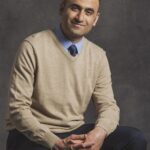
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?