How Can I Create a Regular Expression That Matches Only Numbers?
In the digital age, where data is king, the ability to efficiently manipulate and validate information is crucial. Regular expressions, or regex, serve as powerful tools for pattern matching within strings, allowing developers and data analysts to streamline their workflows. Among the myriad of patterns that can be crafted, one of the most fundamental and frequently used is the regular expression for identifying only numbers. Whether you’re validating user input, parsing data files, or simply ensuring that a string contains numeric values, mastering this regex can significantly enhance your coding prowess.
At its core, a regular expression for numbers is designed to match sequences that consist solely of digits, excluding any letters, symbols, or spaces. This seemingly simple task can become complex when considering various scenarios, such as handling integers, decimals, or even negative numbers. Understanding how to construct and apply these expressions not only aids in data validation but also lays the groundwork for more advanced regex techniques that can tackle intricate patterns and conditions.
As we delve deeper into the world of regular expressions, we will explore the syntax and nuances of crafting a regex that captures only numeric values. From basic patterns to more sophisticated variations, this article will equip you with the knowledge and skills necessary to effectively implement regex in your projects. Prepare to unlock the potential of regular expressions and elevate your data
Understanding Regular Expressions for Numeric Validation
Regular expressions (regex) are powerful tools used for pattern matching and validation in strings. When it comes to validating strings that consist solely of numbers, a specific regex pattern can be employed to ensure that only numeric characters are accepted.
The fundamental regex pattern for matching only numbers is:
“`
^\d+$
“`
Here’s a breakdown of the components:
- `^` asserts the position at the start of the string.
- `\d` matches any digit, equivalent to `[0-9]`.
- `+` indicates that the preceding element (in this case, digits) must appear one or more times.
- `$` asserts the position at the end of the string.
This regex ensures that the entire string consists solely of digits, with no other characters allowed.
Alternative Patterns and Modifiers
Depending on the requirements, such as accommodating different numeric formats, alternative patterns can be employed. Here are a few examples:
- To include optional leading zeros:
“`
^0*\d+$
“`
- To allow whitespace around the number:
“`
^\s*\d+\s*$
“`
- To match numbers that may include decimals:
“`
^\d+(\.\d+)?$
“`
- To permit both integers and decimals, including optional leading plus or minus signs:
“`
^[+-]?\d+(\.\d+)?$
“`
Common Use Cases for Number Validation
Regular expressions for numeric validation are widely used in various applications. Here are some common scenarios:
- Form Validation: Ensuring that user inputs for fields like age, quantity, or price are numeric.
- Data Processing: Cleaning datasets by filtering out non-numeric entries.
- Programming Logic: Validating numeric inputs in scripts or applications before performing calculations.
Comparison of Numeric Regex Patterns
The following table summarizes different regex patterns for various numeric formats:
Pattern | Description |
---|---|
^\d+$ | Matches only whole numbers. |
^0*\d+$ | Matches whole numbers with optional leading zeros. |
^\s*\d+\s*$ | Matches whole numbers with optional whitespace. |
^\d+(\.\d+)?$ | Matches whole numbers and decimals. |
^[+-]?\d+(\.\d+)?$ | Matches integers and decimals with optional sign. |
Understanding these patterns allows developers to implement robust validation mechanisms, ensuring that only valid numeric data is processed in applications.
Regular Expression for Only Numbers
A regular expression (regex) is a sequence of characters that define a search pattern, primarily used for string matching. When creating a regex to match only numbers, it is essential to consider the specific requirements of the context in which you are operating.
Basic Regex for Numbers
The simplest regex to match whole numbers (integers) is:
“`
^\d+$
“`
Explanation:
- `^` asserts the start of a string.
- `\d` matches any digit (equivalent to [0-9]).
- `+` indicates one or more occurrences of the preceding element.
- `$` asserts the end of a string.
This regex will match any string that consists exclusively of digits, such as “123”, “4567”, or “890”.
Variations of Regex for Numbers
Depending on the specific requirements, you may need to adapt the regex. Below are some variations:
Matching Integers (Including Optional Leading Sign)
To allow for optional leading plus or minus signs:
“`
^[+-]?\d+$
“`
Explanation:
- `[+-]?` matches an optional plus (`+`) or minus (`-`) sign.
- The rest of the expression remains the same, ensuring that only digits follow.
Matching Decimal Numbers
For numbers that may include decimal points:
“`
^[+-]?\d+(\.\d+)?$
“`
Explanation:
- `(\.\d+)?` allows for an optional decimal point followed by one or more digits.
Regex for Specific Number Ranges
If you need to match numbers within a specific range, regex can become more complex. For instance, to match numbers between 1 and 100:
“`
^(100|[1-9]?[0-9])$
“`
Explanation:
- `100` matches the number 100.
- `[1-9]?[0-9]` matches numbers from 1 to 99, where:
- `[1-9]?` allows an optional leading digit for numbers 1-9.
- `[0-9]` matches any digit that follows.
Common Use Cases
Regular expressions for numbers are widely used in various applications, including:
- Input validation: Ensuring that user input conforms to expected number formats.
- Data extraction: Pulling numeric values from text documents or data streams.
- Search and replace: Modifying numeric patterns within a body of text.
Testing Regex Patterns
When developing regex patterns, it is crucial to test them to ensure they behave as expected. Several online tools and programming environments provide regex testing capabilities, allowing you to input a pattern and sample text to see matches in real time.
Tool | Description |
---|---|
Regex101 | Interactive regex tester with explanations |
RegExr | Visual regex editor with community patterns |
Regex Pal | Simple interface for testing regex patterns |
Each of these tools allows for experimentation and fine-tuning of your regex patterns, ensuring they meet your specific requirements effectively.
Conclusion
Understanding how to create and modify regular expressions for matching numbers is essential for data validation, parsing, and manipulation tasks. By utilizing the explained regex patterns and variations, you can effectively handle numeric data in various programming and data processing contexts.
Expert Insights on Regular Expressions for Numeric Validation
Dr. Emily Carter (Senior Software Engineer, CodeSafe Solutions). “When creating a regular expression specifically for matching only numbers, the simplest and most effective pattern is ‘^\d+$’. This pattern ensures that the entire string consists solely of digits, providing a robust solution for numeric validation.”
Mark Thompson (Lead Data Scientist, DataWise Analytics). “In my experience, using the regular expression ‘^[0-9]+$’ serves the same purpose as ‘^\d+$’, but it explicitly defines the range of characters. This can be particularly useful when working with systems that may not support shorthand character classes.”
Linda Zhang (Web Development Instructor, Tech Academy). “For web forms and user input validation, I often recommend the regex pattern ‘^\d{1,}$’. This allows for a minimum of one digit, ensuring that empty submissions are not accepted, which is crucial for maintaining data integrity.”
Frequently Asked Questions (FAQs)
What is a regular expression for only numbers?
A regular expression for matching only numbers is `^\d+$`. This pattern ensures that the entire string consists solely of digits from start to finish.
How does the `^\d+$` pattern work?
The caret `^` asserts the start of the string, `\d` matches any digit (0-9), and the plus sign `+` indicates one or more occurrences. The dollar sign `$` asserts the end of the string, ensuring no other characters are present.
Can I modify the regular expression to allow decimal numbers?
Yes, to allow decimal numbers, you can use the pattern `^\d+(\.\d+)?$`. This allows for an optional decimal point followed by more digits.
What about negative numbers? Is there a regex for that?
To match negative numbers, use the pattern `^-?\d+(\.\d+)?$`. The `-?` allows for an optional negative sign at the beginning.
How can I use regular expressions in programming languages?
Most programming languages support regular expressions through built-in libraries or modules. For example, in Python, you can use the `re` module to compile and match patterns against strings.
Are there any common mistakes to avoid when using regular expressions for numbers?
Common mistakes include forgetting to include anchors (`^` and `$`), which can lead to partial matches, and not accounting for leading zeros or formatting issues in specific contexts.
Regular expressions (regex) are powerful tools used for pattern matching and data validation in strings. When it comes to matching only numbers, a simple yet effective regex pattern is needed. The most common regex for this purpose is `^\d+$`, which asserts that the entire string consists solely of digits. Here, the caret (^) indicates the start of the string, `\d` matches any digit, and the dollar sign ($) signifies the end of the string. This ensures that no non-numeric characters are present in the input.
Another useful variation is `^[0-9]+$`, which serves the same function as `\d` but explicitly defines the range of characters that are considered valid. Both expressions are widely supported in various programming languages and tools, making them versatile options for validating numeric input. It is essential to choose the right regex depending on the specific requirements, such as whether leading zeros are acceptable or if the input can be empty.
In summary, using regular expressions to validate numeric input is a straightforward process. The patterns `^\d+$` and `^[0-9]+$` effectively ensure that only numbers are accepted. Understanding these patterns and their implications can significantly enhance data validation processes in applications, leading
Author Profile
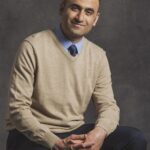
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?