How Can You Remove ‘n’ from a String in Python?
In the world of programming, string manipulation is a fundamental skill that every developer should master. Whether you’re cleaning up user input, formatting data for display, or preparing text for analysis, knowing how to efficiently modify strings is essential. One common task that arises frequently is the need to remove specific characters from a string, such as the letter ‘n’. This seemingly simple operation can be crucial in various applications, from data processing to text analysis. In this article, we will explore the different methods available in Python to remove ‘n’ from strings, empowering you with the knowledge to streamline your code and enhance your programming toolkit.
When it comes to string manipulation in Python, there are several approaches you can take to remove unwanted characters. From built-in string methods to more advanced techniques using regular expressions, Python offers a versatile set of tools that cater to different needs and preferences. Understanding these methods not only simplifies your code but also improves its readability and efficiency.
As we delve deeper into the topic, we will examine practical examples and scenarios where removing ‘n’ from strings can be particularly useful. Whether you’re working on a small script or a larger application, the ability to manipulate strings effectively will enhance your programming prowess and help you tackle various challenges with ease. Get ready to unlock the
Removing Specific Characters from a String
To remove a specific character, such as ‘n’, from a string in Python, you can employ various methods. The most common approaches include using the `replace()` method, list comprehensions, and regular expressions.
Using the replace() Method
The `replace()` method is a straightforward way to remove a character from a string. This method creates a new string where all occurrences of a specified substring are replaced with another substring. If you want to remove a character, you can replace it with an empty string.
“`python
original_string = “banana”
modified_string = original_string.replace(“n”, “”)
print(modified_string) Output: “baa”
“`
Using List Comprehensions
List comprehensions provide a concise way to filter out characters from a string. By iterating through each character and including only those that do not match the specified character, you can construct a new string.
“`python
original_string = “banana”
modified_string = ”.join([char for char in original_string if char != ‘n’])
print(modified_string) Output: “baa”
“`
Using Regular Expressions
For more complex scenarios, regular expressions can be employed using the `re` module. This is particularly useful if you need to remove characters based on patterns rather than specific characters alone.
“`python
import re
original_string = “banana”
modified_string = re.sub(r’n’, ”, original_string)
print(modified_string) Output: “baa”
“`
Performance Considerations
When choosing a method for removing characters from a string, consider the following factors:
- Simplicity: The `replace()` method is the most straightforward for removing known characters.
- Flexibility: Regular expressions offer greater flexibility for complex patterns.
- Performance: For large strings or frequent operations, list comprehensions may be more efficient.
Method | Use Case | Performance |
---|---|---|
replace() | Removing specific, known characters | Fast for small strings |
List Comprehensions | Custom filtering of characters | Efficient for moderate sizes |
Regular Expressions | Complex patterns and multiple conditions | Slower due to overhead |
By understanding these methods, you can effectively remove characters from strings in Python, choosing the approach that best fits your specific needs and constraints.
Methods to Remove ‘n’ from a String in Python
Removing a specific character, such as ‘n’, from a string in Python can be accomplished using several methods. Below are some effective techniques for achieving this:
Using the `replace()` Method
The simplest way to remove a character from a string is by using the `replace()` method. This method returns a new string with all occurrences of a specified substring replaced with another substring.
“`python
original_string = “banana”
modified_string = original_string.replace(‘n’, ”)
print(modified_string) Output: “baa”
“`
- Syntax: `string.replace(old, new[, count])`
- Parameters:
- `old`: The substring to be replaced.
- `new`: The substring to replace with (use an empty string `”` to remove).
- `count`: Optional. The number of occurrences to replace. If omitted, all occurrences are replaced.
Using List Comprehension
Another efficient method is to use list comprehension to filter out the character ‘n’. This approach constructs a new string by including only the characters that do not match ‘n’.
“`python
original_string = “banana”
modified_string = ”.join([char for char in original_string if char != ‘n’])
print(modified_string) Output: “baa”
“`
- Explanation:
- Iterate over each character in the original string.
- Use a conditional check to exclude ‘n’.
- Join the resulting list of characters back into a string.
Using the `filter()` Function
The `filter()` function can also be utilized to remove unwanted characters. This functional programming approach returns an iterator that filters elements from the original string.
“`python
original_string = “banana”
modified_string = ”.join(filter(lambda char: char != ‘n’, original_string))
print(modified_string) Output: “baa”
“`
- How it works:
- The `filter()` function takes a function and an iterable.
- The lambda function checks if the character is not ‘n’.
- The result is then joined into a single string.
Using Regular Expressions
For more complex string manipulations, the `re` module offers powerful pattern matching capabilities, including the ability to remove characters.
“`python
import re
original_string = “banana”
modified_string = re.sub(‘n’, ”, original_string)
print(modified_string) Output: “baa”
“`
- Components:
- `re.sub(pattern, repl, string)` replaces occurrences of the pattern with `repl`.
- In this case, `pattern` is ‘n’ and `repl` is an empty string.
Performance Considerations
When selecting a method to remove characters from strings, consider the following:
Method | Time Complexity | Description |
---|---|---|
`replace()` | O(n) | Simple and effective for direct replacements. |
List Comprehension | O(n) | Provides flexibility and is easy to read. |
`filter()` | O(n) | Useful for functional programming style. |
Regular Expressions | O(n) | More powerful for complex patterns but can be slower. |
Choosing the appropriate method depends on the specific requirements of your project, such as readability, performance, and complexity of the operation.
Expert Insights on Removing Characters from Strings in Python
Dr. Emily Carter (Senior Software Engineer, CodeCraft Inc.). “To effectively remove a specific character, such as ‘n’, from a string in Python, the most straightforward method is to use the `replace()` function. This allows for clear and concise code, enhancing readability and maintainability.”
Michael Chen (Python Developer, Tech Innovations). “Utilizing list comprehensions provides a powerful and flexible way to remove unwanted characters from strings. This method not only improves performance but also allows for more complex filtering conditions if needed.”
Sarah Thompson (Data Scientist, Analytics Hub). “For those dealing with larger datasets, leveraging regular expressions through the `re` module can be particularly effective. It offers advanced pattern matching capabilities, making it easier to remove multiple instances of a character or even entire patterns.”
Frequently Asked Questions (FAQs)
How can I remove a specific character from a string in Python?
You can use the `replace()` method to remove a specific character. For example, `string.replace(‘n’, ”)` will remove all occurrences of ‘n’ from the string.
Is there a way to remove multiple characters from a string in Python?
Yes, you can use the `str.translate()` method combined with `str.maketrans()`. For example, `string.translate(str.maketrans(”, ”, ‘n’))` removes ‘n’ from the string.
Can I remove characters based on their index in Python?
Yes, you can use slicing to remove characters by index. For instance, `string[:index] + string[index+1:]` removes the character at the specified index.
What is the difference between `replace()` and `translate()` in Python?
The `replace()` method is used for replacing specific substrings with another substring, while `translate()` is more efficient for removing or replacing multiple characters at once.
How do I remove all occurrences of ‘n’ from a list of strings in Python?
You can use a list comprehension with the `replace()` method. For example, `[s.replace(‘n’, ”) for s in string_list]` removes ‘n’ from each string in the list.
Are there any performance considerations when removing characters from large strings in Python?
Yes, using `replace()` or `translate()` is generally efficient, but for very large strings, `translate()` is preferred due to its optimized performance for multiple character removals.
In Python, removing a specific character, such as ‘n’, from a string can be accomplished through various methods. The most common techniques include using the `str.replace()` method, list comprehensions, and the `str.translate()` method. Each of these approaches offers unique advantages depending on the context and requirements of the task at hand. For instance, `str.replace()` is straightforward and efficient for simple replacements, while list comprehensions provide flexibility for more complex conditions.
Another effective method is utilizing the `str.translate()` function in conjunction with the `str.maketrans()` method, which can be particularly useful when dealing with multiple characters to remove. This approach is efficient and can enhance performance when processing larger strings or when multiple characters need to be eliminated simultaneously. Understanding these various methods allows developers to choose the most appropriate solution based on their specific needs.
In summary, Python provides a range of options for removing characters from strings, with each method having its own use cases and benefits. Familiarity with these techniques not only improves code efficiency but also enhances overall programming skills. By leveraging the appropriate method, developers can streamline their string manipulation tasks effectively.
Author Profile
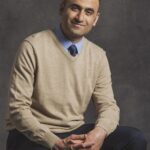
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?