How Can You Rename a Column by Number in R?
Renaming columns in R can be a straightforward yet essential task, especially when working with datasets that require clarity and precision. Whether you’re cleaning up data for analysis or preparing it for presentation, having the right column names can make all the difference. However, sometimes you may find yourself needing to rename columns not by their names but by their positions in the dataset. This is where the flexibility of R shines, allowing you to manipulate your data efficiently and effectively.
In R, renaming columns by their numeric position is a powerful technique that can save time and reduce errors, especially when dealing with large datasets. Instead of sifting through potentially lengthy column names, you can directly reference the column numbers to make quick adjustments. This approach can be particularly useful in scenarios where datasets are imported from external sources and the column names are either missing or not descriptive enough for your analytical needs.
As we delve deeper into the methods and functions available in R for renaming columns by number, you’ll discover practical tips and tricks that can enhance your data manipulation skills. From utilizing built-in functions to leveraging packages designed for data wrangling, this article will equip you with the knowledge needed to streamline your workflow and enhance the clarity of your datasets. So, let’s explore the various ways to efficiently rename columns in R by
Using Base R to Rename Columns by Number
In Base R, renaming columns by their position can be achieved easily using the `colnames()` function. This method allows you to specify the column index directly, making it straightforward to manipulate datasets when the column names are not known or are irrelevant for the task at hand.
To rename a column by its position, follow these steps:
- Identify the index of the column you want to rename.
- Assign a new name to that specific index using `colnames()`.
Here is an example:
“`R
Sample data frame
df <- data.frame(A = 1:3, B = 4:6, C = 7:9)
Rename the second column
colnames(df)[2] <- "NewName"
print(df)
```
This will change the second column name from "B" to "NewName".
Using dplyr for Renaming Columns by Number
The `dplyr` package offers a more streamlined approach to renaming columns, especially useful when working with larger datasets. The `rename()` function allows you to rename columns using their positions in a more readable manner.
To rename a column by its index using `dplyr`, you can use the following syntax:
“`R
library(dplyr)
Sample data frame
df <- data.frame(A = 1:3, B = 4:6, C = 7:9)
Rename the second column
df <- df %>%
rename(NewName = .data[[2]])
print(df)
“`
This approach provides clarity and flexibility in renaming multiple columns if needed.
Renaming Multiple Columns by Number
When needing to rename multiple columns by their positions, you can extend the functionality of either Base R or `dplyr`. The following example demonstrates how to rename several columns at once.
Using Base R:
“`R
Sample data frame
df <- data.frame(A = 1:3, B = 4:6, C = 7:9)
Rename first and third columns
colnames(df)[c(1, 3)] <- c("FirstName", "ThirdName")
print(df)
```
Using `dplyr`:
```R
library(dplyr)
Sample data frame
df <- data.frame(A = 1:3, B = 4:6, C = 7:9)
Rename multiple columns
df <- df %>%
rename(
FirstName = .data[[1]],
ThirdName = .data[[3]]
)
print(df)
“`
Comparison of Methods
Here’s a brief comparison of using Base R and `dplyr` for renaming columns by number:
Method | Advantages | Disadvantages |
---|---|---|
Base R |
|
|
dplyr |
|
|
Both methods are effective, and the choice between them often depends on personal preference or the specific context of data manipulation tasks.
Renaming Columns by Number in R
Renaming columns by their numeric index in R can be accomplished using various methods, primarily through the use of data frames. Below are the most common approaches.
Using Base R
In base R, you can directly manipulate the `names()` function or the column indexing to rename columns. Here’s how you can do it:
“`R
Create a sample data frame
df <- data.frame(A = 1:5, B = 6:10, C = 11:15)
Display original column names
print(names(df))
Rename the second column
names(df)[2] <- "NewName"
```
After executing the code, the column names of the data frame will be:
Old Name | New Name |
---|---|
A | NewName |
C | C |
Using dplyr Package
The `dplyr` package provides a user-friendly way to rename columns using the `rename()` function. When renaming by index, you can utilize `dplyr::rename_with()` alongside `names()`. Here’s an example:
“`R
library(dplyr)
Sample data frame
df <- data.frame(A = 1:5, B = 6:10, C = 11:15)
Rename the second column
df <- df %>% rename_with(~ c(“A”, “NewName”, “C”), .cols = 2)
“`
This will yield the same result as in the base R method, where the second column is now named “NewName”.
Using data.table Package
The `data.table` package offers another efficient method for renaming columns by their indices. You can utilize the `setnames()` function:
“`R
library(data.table)
Create a sample data.table
dt <- data.table(A = 1:5, B = 6:10, C = 11:15)
Rename the second column
setnames(dt, 2, "NewName")
```
After executing this code, the output will reflect the updated column names in the `data.table`.
Considerations
When renaming columns by number, keep in mind:
- Ensure the index you are using corresponds to the correct column.
- Be cautious of potential data frame changes that might alter the column indices.
- If using `dplyr` or `data.table`, ensure the respective library is loaded.
This approach simplifies the process of renaming columns without needing to reference their original names, making it especially useful in data manipulation tasks where column names may not be known or are subject to change.
Expert Insights on Renaming Columns by Number in R
Dr. Emily Carter (Data Scientist, StatTech Solutions). “Renaming columns by number in R is a straightforward task, often accomplished using the `colnames()` function. This method allows users to directly assign new names to specific columns by their index, which can be particularly useful when working with large datasets where column names may not be known in advance.”
Michael Chen (R Programming Instructor, Data Academy). “Utilizing the `names()` function in conjunction with indexing provides an efficient way to rename columns by their position. This approach is not only simple but also enhances code readability, making it easier for others to understand the modifications made to the dataset.”
Lisa Patel (Senior Statistician, Insight Analytics Group). “When renaming columns by number in R, it is essential to ensure that the new names are unique and descriptive. This practice minimizes confusion and enhances data integrity, especially when performing subsequent analyses or merging datasets with similar structures.”
Frequently Asked Questions (FAQs)
How can I rename a column by its index in a data frame in R?
You can rename a column by its index using the `names()` function. For example, `names(df)[2] <- "new_name"` will rename the second column of the data frame `df` to "new_name".
Is there a function that allows renaming multiple columns by their indices in R?
Yes, you can rename multiple columns by their indices using a vector. For instance, `names(df)[c(1, 3)] <- c("new_name1", "new_name3")` will rename the first and third columns of `df`.
Can I use the `dplyr` package to rename columns by index?
Yes, the `dplyr` package provides the `rename()` function, but it typically uses column names. To rename by index, you can use `rename()` in conjunction with `select()`, such as `df <- df %>% rename(new_name = df[[2]])`.
What happens if I try to rename a column index that does not exist?
If you attempt to rename a column index that does not exist, R will return an error indicating that the index is out of bounds. Always ensure the index is valid for the data frame.
Can I rename columns in a tibble using their indices?
Yes, you can rename columns in a tibble using their indices similarly to a data frame. Use `names(tibble)[index] <- "new_name"` to rename the specified column.
Are there any alternatives to renaming columns by index in R?
Alternatives include renaming by name directly or using the `setNames()` function to create a new data frame with updated column names. This method can enhance readability and maintainability of the code.
Renaming columns by number in R is a straightforward process that can be accomplished using various methods. The most common approach involves utilizing the `colnames()` function, which allows users to directly assign new names to specific columns based on their numeric positions. This method is particularly useful when dealing with data frames where the column names may not be known in advance or when a quick renaming is required for analysis or presentation purposes.
Another effective method involves using the `dplyr` package, which provides a more intuitive syntax for renaming columns. The `rename()` function within `dplyr` allows users to specify the new names alongside the existing column indices, making it easier to manage larger datasets. This approach not only enhances code readability but also integrates seamlessly with other data manipulation tasks commonly performed in R.
It is essential to keep in mind that renaming columns by number can lead to potential issues, particularly when the structure of the data frame changes. Therefore, it is advisable to ensure that the column indices being referenced remain consistent throughout the analysis. Additionally, utilizing descriptive column names is recommended for better clarity and maintainability of the code.
Author Profile
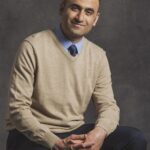
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?