Why Does ‘require’ Not Define in ES Module Scope?
In the evolving landscape of JavaScript, developers are frequently confronted with the nuances of module systems, particularly as the shift from CommonJS to ES Modules becomes more prevalent. One common error that many encounter during this transition is the message: “require is not defined in ES module scope.” This seemingly cryptic notification can halt progress and leave even seasoned developers scratching their heads. Understanding the underlying reasons for this error is crucial for anyone looking to harness the full power of modern JavaScript.
As JavaScript continues to advance, the way we manage dependencies and organize code has undergone a significant transformation. ES Modules (ECMAScript Modules) offer a more standardized and cleaner approach to modular programming compared to their predecessor, CommonJS. However, this shift brings with it a set of challenges, particularly when it comes to compatibility and the use of certain features. The error message in question serves as a reminder of the differences between these two module systems and highlights the importance of adapting to the new paradigms.
In this article, we will delve into the intricacies of module systems in JavaScript, exploring the reasons behind the “require is not defined” error and how to effectively navigate the transition from CommonJS to ES Modules. By understanding the core concepts and best practices, developers can not only
Understanding the Error
The error message “require is not defined in ES module scope” typically arises when attempting to use Node.js’s `require` function in an environment that expects ECMAScript (ES) modules. In ES modules, the `require` function, which is a part of CommonJS, is not available. Instead, ES modules use the `import` statement for module loading.
This distinction is crucial, particularly in environments like Node.js, where both module systems coexist. Understanding the differences between CommonJS and ES modules is essential for resolving this error.
CommonJS vs. ES Modules
The main differences between CommonJS and ES modules include syntax, loading behavior, and scope management. Below is a comparison of these two module systems:
Feature | CommonJS | ES Modules |
---|---|---|
Syntax | Uses `require()` for importing and `module.exports` for exporting. | Uses `import` for importing and `export` for exporting. |
Loading | Modules are loaded synchronously. | Modules are loaded asynchronously. |
Scope | Modules have their own scope; global variables are not shared. | Modules are executed in strict mode by default, affecting variable scoping. |
Solutions to the Error
To resolve the “require is not defined in ES module scope” error, consider the following approaches:
- Use `import` Instead of `require`: If you are working in an ES module context, replace `require` with `import`. For example:
“`javascript
// Instead of this
const module = require(‘module-name’);
// Use this
import module from ‘module-name’;
“`
- Change the Module Type: If you need to use `require`, you can change the module type in your `package.json`:
“`json
{
“type”: “commonjs”
}
“`
- Use Dynamic Imports: If you need to conditionally load modules, consider using dynamic imports:
“`javascript
const module = await import(‘module-name’);
“`
- Transpile Code: Use a tool like Babel to transpile ES module syntax into CommonJS, allowing for broader compatibility.
Best Practices
When working with modules in JavaScript, consider the following best practices to avoid issues:
- Always define the module type in your `package.json` to clarify whether you are using CommonJS or ES modules.
- Stick to one module system within a project to maintain consistency and avoid confusion.
- Test your modules in the environment they are intended for, ensuring compatibility with the module system in use.
- Keep dependencies updated to utilize the latest features and fixes related to module handling.
Understanding the Error
The error message “require is not defined in ES module scope” typically arises when attempting to use CommonJS `require` syntax within an ES module context. In JavaScript, ES modules and CommonJS modules have different syntax and loading mechanisms, leading to compatibility issues.
- CommonJS: Utilizes `require()` for importing modules and `module.exports` for exporting.
- ES Modules: Uses `import` and `export` statements, promoting a more standardized approach for modular code.
When a file is marked as an ES module (typically by using the `.mjs` extension or including `”type”: “module”` in `package.json`), the CommonJS `require` function is not available, resulting in this error.
Common Causes
Several scenarios can lead to encountering this error:
- File Extensions: Using a `.js` extension without setting the module type in `package.json`.
- Mixing Module Types: Importing a CommonJS module in an ES module without proper interop.
- Global Environment: Attempting to use `require` in environments where only ES module syntax is supported, such as the browser.
Solutions
To resolve this error, consider the following approaches:
- Convert to ES Module Syntax:
- Replace `require()` with `import`.
- Example:
“`javascript
// CommonJS
const module = require(‘./module’);
// ES Module
import module from ‘./module.js’;
“`
- Use Dynamic Imports:
- If you must use CommonJS, use dynamic imports as a workaround.
- Example:
“`javascript
const module = await import(‘./module.js’);
“`
- Adjust Package Configuration:
- Ensure your `package.json` specifies the correct module type.
- Example:
“`json
{
“type”: “module”
}
“`
Interop Between CommonJS and ES Modules
When working with both CommonJS and ES modules, it’s essential to understand how they can interoperate:
CommonJS Syntax | ES Module Equivalent |
---|---|
`const module = require(‘module’);` | `import module from ‘module’;` |
`module.exports = value;` | `export default value;` |
- Default Exports: In CommonJS, `module.exports` is used, while in ES modules, `export default` is the norm.
- Named Exports: CommonJS exports can be accessed as properties, while ES modules utilize explicit named exports.
Additional Considerations
When transitioning to ES modules, keep in mind the following:
- Top-Level Await: ES modules support top-level await, allowing asynchronous code to run at the top level.
- Loading Order: ES modules are loaded in a strict order, which can impact how dependencies are resolved.
- Browser Compatibility: Ensure that the environment where the code runs supports ES modules, especially in older browsers.
By understanding the differences between module systems and adapting your code accordingly, you can effectively address the “require is not defined in ES module scope” error and leverage the benefits of ES modules in your JavaScript projects.
Understanding the ‘require is not defined in ES Module Scope’ Issue
Dr. Emily Carter (JavaScript Framework Specialist, Tech Innovations Inc.). “The error message ‘require is not defined in ES module scope’ typically arises when attempting to use CommonJS syntax in an ES module. ES modules utilize the ‘import’ and ‘export’ syntax, which means that developers need to refactor their code to align with this modern module system.”
Mark Thompson (Senior Software Engineer, Node.js Foundation). “This issue is a common stumbling block for developers transitioning from CommonJS to ES modules. It is crucial to understand that while Node.js supports both module systems, they are not interchangeable. Using ‘require’ in an ES module context will lead to this specific error.”
Lisa Nguyen (Lead Developer Advocate, Modern Web Technologies). “To resolve the ‘require is not defined’ error, developers should consider using dynamic imports or converting their modules to ES module syntax. This shift not only resolves the error but also enhances compatibility with modern JavaScript features.”
Frequently Asked Questions (FAQs)
What does “require is not defined in es module scope” mean?
This error indicates that you are attempting to use the `require` function, which is part of CommonJS module syntax, in a JavaScript file that is being treated as an ES Module. ES Modules use `import` and `export` statements instead.
How can I resolve the “require is not defined in es module scope” error?
To resolve this error, you can either switch to using ES Module syntax by replacing `require` with `import`, or you can configure your environment to treat the file as a CommonJS module by changing the file extension to `.cjs` or modifying the `package.json` file.
Can I use both CommonJS and ES Module syntax in the same project?
Yes, you can use both syntaxes in the same project, but you must ensure that files are correctly identified as either CommonJS or ES Modules. This typically involves using the appropriate file extensions and ensuring that your environment supports both.
What are the main differences between CommonJS and ES Modules?
CommonJS uses `require` for importing modules and `module.exports` for exporting, while ES Modules use `import` and `export` statements. Additionally, CommonJS is synchronous and primarily used in Node.js, whereas ES Modules support asynchronous loading and are designed for both Node.js and browser environments.
Is it possible to convert a CommonJS module to an ES Module?
Yes, you can convert a CommonJS module to an ES Module by replacing `require` statements with `import` statements and changing `module.exports` to `export default` or named exports. Ensure to also update any dependencies that may rely on the original module format.
What should I do if I need to use a CommonJS library in an ES Module?
If you need to use a CommonJS library in an ES Module, you can import it using dynamic import syntax, like `const module = await import(‘module-name’)`, or use a bundler like Webpack or Babel that can handle both module types seamlessly.
The error message “require is not defined in ES module scope” typically arises when developers attempt to use the CommonJS `require` function within an ES module context. ES modules, introduced in ECMAScript 6, utilize a different syntax for importing and exporting modules, primarily through the `import` and `export` statements. This distinction is crucial for developers to understand, as it reflects the evolving nature of JavaScript and its module system.
One of the main points to consider is that ES modules are designed to work asynchronously and are better suited for modern JavaScript development, particularly in environments such as browsers and Node.js. The shift from CommonJS to ES modules promotes a more standardized approach to module management, enhancing interoperability and performance. Developers must adapt their code accordingly to avoid encountering this error.
Key takeaways include the importance of recognizing the environment in which the code is executed. If a project is set up to use ES modules, developers should replace `require` with `import` statements. Additionally, understanding the configuration of the JavaScript runtime, such as using the correct file extensions and module type settings in `package.json`, can prevent such issues. Overall, embracing ES modules is essential for leveraging the full capabilities of modern JavaScript
Author Profile
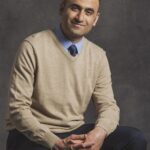
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?