Why Am I Seeing ‘Require of ES Module Not Supported’ Errors in My JavaScript Project?
In the ever-evolving landscape of web development, the transition from CommonJS to ES Modules has sparked a wave of excitement and confusion among developers. As JavaScript continues to mature, the of ES Modules (ECMAScript Modules) has brought with it a new paradigm for organizing and managing code. However, with this shift comes a set of challenges, particularly for those accustomed to the traditional `require` syntax. If you’ve ever stumbled upon the error message “require of ES module not supported,” you know just how frustrating it can be. This article delves into the intricacies of this issue, shedding light on what it means for your projects and how to navigate the complexities of module imports in modern JavaScript.
At the heart of the problem lies the fundamental difference between CommonJS and ES Modules. While CommonJS relies on the `require` function to load modules synchronously, ES Modules embrace a more flexible, asynchronous approach with the `import` statement. This shift not only enhances performance but also aligns JavaScript with the module systems of other programming languages. However, this transition can lead to compatibility issues, especially when older codebases or third-party libraries that still use CommonJS are involved. Understanding these nuances is crucial for developers aiming to harness the full power of ES Modules without
Understanding ES Modules and CommonJS
In the JavaScript ecosystem, two primary module systems are widely used: CommonJS and ES Modules (ESM). CommonJS, which is the module system traditionally used in Node.js, relies on `require()` for importing modules. In contrast, ES Modules utilize the `import` and `export` syntax, which was standardized in ECMAScript 2015 (ES6).
The crux of the issue arises when developers attempt to use `require()` to load ES Modules. This is unsupported because the two systems are inherently different in how they handle module loading and execution.
Why `require` of ES Module is Not Supported
The incompatibility of `require()` with ES Modules stems from several foundational differences:
- Synchronous vs. Asynchronous Loading: CommonJS modules are loaded synchronously, which means that the code execution pauses until the module is loaded. ES Modules, however, support asynchronous loading, allowing for better performance and optimization in web environments.
- Hoisting of Imports: In ES Modules, imports are hoisted, meaning they are available at the top of the module scope regardless of where they are declared. CommonJS does not hoist `require()` calls, which can lead to different execution behaviors.
- Dynamic vs. Static Structure: CommonJS allows dynamic module loading, while ES Modules require static import paths, which enables tree-shaking capabilities in modern build tools to remove unused code.
Given these differences, attempting to use `require()` on an ES Module results in a runtime error.
How to Work with ES Modules in Node.js
To effectively work with ES Modules in Node.js, developers should follow these practices:
- Use the `import` statement for importing ES Modules.
- Ensure that the file has a `.mjs` extension or set the `”type”: “module”` in the `package.json` file.
- Use tools like Babel or Webpack if needing to transpile or bundle CommonJS and ES Modules together.
Here’s a comparison of CommonJS and ES Module syntax:
Feature | CommonJS | ES Modules |
---|---|---|
Module Declaration | `module.exports = value;` | `export default value;` |
Importing Modules | `const module = require(‘module’);` | `import module from ‘module’;` |
Dynamic Imports | Not supported | `const module = await import(‘module’);` |
Resolving Errors
To resolve issues related to importing ES Modules, consider the following approaches:
- Check File Extensions: Ensure that the module files are using the correct `.mjs` extension or that the `”type”: “module”` is specified in `package.json`.
- Update Node.js Version: Ensure that you are using a Node.js version that supports ES Modules natively (v12 and above).
- Use Babel: If compatibility with older environments is necessary, consider using Babel to transpile your code.
By adhering to these guidelines, developers can effectively navigate the challenges posed by the differences in module systems and leverage the benefits of ES Modules in their applications.
Understanding ES Modules
ES Modules (ECMAScript Modules) represent a standardized module system in JavaScript, enabling the import and export of code between different files. This module system is designed to work seamlessly with modern JavaScript features. However, issues arise when trying to utilize CommonJS-style `require` calls in an ES Module context.
Common Reasons for `require` Issues
When encountering the error message indicating that the `require` of ES Module is not supported, several common issues may be at play:
- Module Type Declaration: The module type must be explicitly declared in the `package.json` file:
- `”type”: “module”` for ES Modules
- `”type”: “commonjs”` for CommonJS
- File Extensions: Ensure that ES Module files use the `.mjs` extension or that the package.json specifies the type as module.
- Import Syntax: The correct syntax for importing modules must be used:
- Use `import` instead of `require` to load modules.
- Top-Level Await: Ensure that any asynchronous code is handled properly since top-level await is only available in ES Modules.
Key Differences Between CommonJS and ES Modules
Understanding the differences between the two module systems is crucial:
Feature | CommonJS | ES Modules |
---|---|---|
Syntax | `require()` for imports | `import` for imports |
Exporting | `module.exports` | `export` and `export default` |
Loading Mechanism | Synchronous | Asynchronous |
Scope | Module scope | Block scope |
Circular Dependencies | Handled automatically | Can lead to issues without care |
Converting CommonJS to ES Modules
To transition code from CommonJS to ES Modules, follow these steps:
- Change File Extensions: Rename files from `.js` to `.mjs` or update the `package.json` to include `”type”: “module”`.
- Modify Import Statements:
- Replace `const module = require(‘module’)` with `import module from ‘module’`.
- Adjust Export Statements:
- Change `module.exports = value` to `export default value` or `export const name = value`.
- Handle Dependencies: Review any third-party libraries to ensure they support ES Modules.
Debugging Tips for ES Modules
If issues persist, consider the following debugging strategies:
- Check Node Version: Ensure you are using a Node.js version that supports ES Modules (Node.js 12.x and above).
- Inspect the Error Message: The error message often provides clues about what specifically is causing the issue.
- Review Documentation: Consult the official Node.js documentation for ES Modules, as it contains valuable insights and updates.
- Use Babel or Transpilers: If immediate compatibility is necessary, consider using Babel to transpile ES Module code to CommonJS.
- Test in Isolation: Create a minimal reproduction of the problem to isolate the issue and simplify debugging.
Best Practices for Using ES Modules
Adhering to best practices can prevent many common pitfalls:
- Consistency: Stick to either CommonJS or ES Modules throughout your codebase to avoid confusion.
- Use Descriptive Names: When exporting multiple entities, use clear and descriptive names to enhance readability.
- Keep Imports Organized: Group and order imports logically to improve maintainability.
- Avoid Side Effects: Ensure that your modules do not have unintended side effects when imported.
Understanding ES Module Compatibility Issues
Dr. Emily Chen (Software Architect, Tech Innovations Inc.). “The error message ‘require of ES module not supported’ typically arises when attempting to use CommonJS syntax in an environment that expects ES module syntax. Developers must ensure they are using the correct module system for their JavaScript files to avoid such compatibility issues.”
Michael Thompson (JavaScript Specialist, CodeMaster Academy). “This error is a clear indication of the transition from CommonJS to ES modules. It’s crucial for developers to familiarize themselves with the differences in import/export syntax and to leverage tools like Babel or Webpack to bridge the gap when necessary.”
Sarah Patel (Senior Frontend Developer, NextGen Solutions). “When encountering the ‘require of ES module not supported’ error, I recommend checking your Node.js version and ensuring that your project configuration aligns with ES module standards. This is essential for maintaining compatibility and maximizing the benefits of modern JavaScript features.”
Frequently Asked Questions (FAQs)
What does “require of es module not supported” mean?
This error indicates that the CommonJS `require` function is being used to import an ES module, which is not supported in environments that strictly adhere to ES module syntax.
How can I resolve the “require of es module not supported” error?
To resolve this error, you can either convert your code to use ES module syntax (using `import` statements) or configure your environment to support both module types, if applicable.
What are the differences between CommonJS and ES modules?
CommonJS modules use `require` and `module.exports` for importing and exporting, while ES modules use `import` and `export` syntax. ES modules are designed to work natively in the browser and support asynchronous loading.
Can I use `require` in an ES module?
No, using `require` in an ES module will result in an error. ES modules must use the `import` statement for importing dependencies.
What environments typically support ES modules?
Modern browsers and Node.js (version 12 and above with appropriate settings) support ES modules. Ensure that your environment is configured correctly to utilize ES module features.
Is there a way to mix CommonJS and ES modules?
Yes, it is possible to mix CommonJS and ES modules, but it requires careful handling. You can use dynamic `import()` to load ES modules from CommonJS files, or vice versa, but be aware of potential compatibility issues.
The issue of “require of ES module not supported” primarily arises from the differences between CommonJS and ES Module systems in JavaScript. CommonJS, which uses the `require` function, has been the traditional module system in Node.js. In contrast, ES Modules (ESM) utilize the `import` and `export` syntax, which is part of the ECMAScript standard. As JavaScript evolves, the adoption of ES Modules has become more prevalent, leading to compatibility challenges for developers who attempt to use `require` with ES Modules.
One of the key takeaways from this discussion is the importance of understanding the module system being used in a project. Developers must ensure that they are using the correct syntax and methods for importing and exporting modules based on whether they are working with CommonJS or ES Modules. This understanding is crucial for avoiding runtime errors and ensuring smooth interoperability between different modules.
Additionally, the transition to ES Modules is encouraged due to their benefits, such as improved performance, better support for asynchronous loading, and a more standardized approach to module management. As the JavaScript ecosystem continues to evolve, developers should consider adopting ES Modules to future-proof their applications and take advantage of the latest features and improvements in the language.
Author Profile
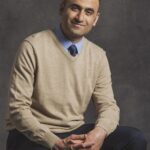
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?