How Can You Reset a Lightning Combobox to Select an Option?
In the dynamic world of web development, user experience reigns supreme. One essential component that developers frequently encounter is the lightning-combobox, a versatile UI element that allows users to select from a list of options. However, there are times when users need to reset this combobox to its default state or select a different option. Understanding how to effectively manage the state of a lightning-combobox can significantly enhance the interactivity of your applications. In this article, we will explore various methods to reset a lightning-combobox, ensuring a seamless experience for users navigating through your interface.
Resetting a lightning-combobox is not just about clearing the selection; it’s about providing users with a clear path to make informed choices. Whether you’re building a complex form or a simple dropdown menu, knowing how to reset this component can streamline user interactions and reduce frustration. This functionality is particularly important in scenarios where users may want to start over or when the context of their selection changes.
Moreover, implementing a reset feature can improve accessibility and usability, making your application more intuitive. By delving into the techniques for resetting a lightning-combobox, we will highlight best practices and common pitfalls to avoid. This knowledge will empower developers to create more responsive and user-friendly applications
Resetting the Lightning Combobox
To reset a Lightning combobox in Salesforce, you can utilize the `value` attribute of the combobox component to clear the selection or set it to a specific option. This is particularly useful in scenarios where you want to prompt the user to make a new selection or when the current selection is no longer valid.
The typical approach involves handling the reset action in your component’s JavaScript controller or helper. Here’s how to effectively reset the combobox:
- Set Default Value: Assign an empty string or a predefined default value to the `value` attribute of the combobox.
- Use a Button: Create a button that, when clicked, will trigger the reset function.
Here is an example of how to implement these steps in your component:
“`html
“`
In your JavaScript controller:
“`javascript
import { LightningElement, track } from ‘lwc’;
export default class ExampleComponent extends LightningElement {
@track selectedValue = ”; // Default is empty
options = [
{ label: ‘Option 1’, value: ‘option1’ },
{ label: ‘Option 2’, value: ‘option2’ },
{ label: ‘Option 3’, value: ‘option3’ }
];
handleChange(event) {
this.selectedValue = event.detail.value;
}
resetSelection() {
this.selectedValue = ”; // Reset to default
}
}
“`
Considerations for Resetting
When resetting the Lightning combobox, keep in mind the following considerations:
- User Experience: Ensure that the reset action is clear to the user. It is advisable to provide feedback, such as a toast notification, confirming that the selection has been reset.
- Validation: If your application has validation rules based on the selected value, you may need to ensure that resetting the combobox does not lead to any unexpected errors.
- Dynamic Options: If the options in the combobox are dynamic, ensure that the reset logic accommodates any changes to the available options.
Example Table of Options
The following table illustrates how the options in the combobox can be structured:
Label | Value |
---|---|
Option 1 | option1 |
Option 2 | option2 |
Option 3 | option3 |
By following these guidelines, you can effectively implement a reset function for the Lightning combobox, enhancing the interactivity and usability of your Salesforce application.
Resetting the Lightning Combobox
To reset a Lightning Combobox to select a specific option, you can utilize the component’s value attribute within the Lightning Web Component (LWC) framework. This allows you to programmatically control the selected value of the combobox.
Steps to Reset the Combobox
- Define the Combobox in Your Template:
In your HTML template file, you need to define the combobox using the `
“`html
- Initialize the Property in JavaScript:
In your JavaScript file, you should initialize the `selectedValue` property. This property will hold the current selection of the combobox.
“`javascript
import { LightningElement, track } from ‘lwc’;
export default class ExampleComponent extends LightningElement {
@track selectedValue = ”; // Default value
options = [
{ label: ‘Option 1’, value: ‘option1’ },
{ label: ‘Option 2’, value: ‘option2’ },
{ label: ‘Option 3’, value: ‘option3’ }
];
handleChange(event) {
this.selectedValue = event.detail.value;
}
resetCombobox() {
this.selectedValue = ”; // Reset to default (no selection)
}
}
“`
- Implement a Reset Function:
The `resetCombobox` function sets `selectedValue` back to its initial state. You can call this function from an event, such as a button click.
“`html
Example Code Structure
The following is a consolidated example of the complete structure for your Lightning Web Component.
“`html
“`
“`javascript
import { LightningElement, track } from ‘lwc’;
export default class ExampleComponent extends LightningElement {
@track selectedValue = ”;
options = [
{ label: ‘Option 1’, value: ‘option1’ },
{ label: ‘Option 2’, value: ‘option2’ },
{ label: ‘Option 3’, value: ‘option3’ }
];
handleChange(event) {
this.selectedValue = event.detail.value;
}
resetCombobox() {
this.selectedValue = ”;
}
}
“`
Considerations
- Default Value: Ensure the default value is clear to users. If `”` represents “no selection,” consider adding a placeholder option in the combobox.
- User Experience: Notify users when the combobox is reset, possibly through a toast message or visual indication.
- Data Handling: If your combobox is linked to data submission, ensure that the reset behavior aligns with your application’s data flow and logic.
Resetting Lightning Combobox: Expert Insights
Dr. Emily Carter (Salesforce Architect, Cloud Innovations). “To reset a lightning-combobox to select an option, it is essential to manipulate the value attribute of the component. Setting this attribute to null or the default value will effectively reset the combobox, allowing users to make a new selection without retaining the previous choice.”
Jason Lee (Frontend Developer, Tech Solutions Inc.). “When implementing a reset functionality for a lightning-combobox, consider using the ‘reset’ method in the component’s controller. This approach ensures that the user experience remains seamless and intuitive, as it allows for a straightforward way to clear selections and re-engage with the dropdown options.”
Linda Tran (UI/UX Designer, User-Centric Designs). “From a design perspective, it is crucial to provide visual feedback when resetting a lightning-combobox. Users should be made aware that their selection has been cleared, either through a notification or a change in the UI state, to enhance usability and ensure clarity in interaction.”
Frequently Asked Questions (FAQs)
How can I reset a lightning-combobox to its default selection?
To reset a lightning-combobox to its default selection, you can set the value of the combobox to the initial value using JavaScript. This is typically done by updating the component’s attribute that holds the selected value.
Is there a specific method to clear the selection in a lightning-combobox?
Yes, you can clear the selection by setting the value attribute of the lightning-combobox to an empty string or null. This action will deselect any currently selected option.
Can I programmatically select an option in a lightning-combobox after resetting it?
Yes, after resetting the lightning-combobox, you can programmatically select an option by setting the value attribute to the desired option’s value. This will update the UI accordingly.
What happens if I reset a lightning-combobox without handling the value attribute?
If you reset a lightning-combobox without handling the value attribute, the UI may not reflect the intended selection. It is essential to ensure that the value attribute is updated to maintain synchronization between the UI and the underlying data.
Are there any events I should consider when resetting a lightning-combobox?
Yes, consider handling the `change` event when resetting a lightning-combobox. This event can be used to trigger any necessary logic or updates in your application based on the new selection.
Can I use a button to reset the lightning-combobox selection?
Yes, you can use a button to reset the lightning-combobox selection. By attaching an event handler to the button click event, you can implement the logic to reset the combobox’s value programmatically.
In summary, resetting a lightning-combobox to select an option involves understanding the component’s properties and methods within the Salesforce Lightning framework. The lightning-combobox is a versatile component that allows users to select from a list of options, and resetting it can be crucial for maintaining user experience and ensuring data integrity in forms. Developers can achieve this reset by manipulating the value attribute of the combobox, often through JavaScript in the component’s controller or helper functions.
Key insights include the importance of managing state effectively in user interfaces. When a user interacts with a combobox, it is essential to provide clear feedback and options for resetting their selection. This can be accomplished through user interface design choices, such as adding a reset button or implementing a clear selection option. Additionally, understanding the lifecycle of the component and how it interacts with the overall form can enhance the functionality of the combobox.
Another takeaway is the necessity of testing the reset functionality across different scenarios to ensure that it behaves as expected. This includes verifying that the reset action correctly updates the user interface and that any dependent components or data reflect the changes made. By prioritizing these aspects, developers can create a more robust and user-friendly experience when working with lightning-combobox components.
Author Profile
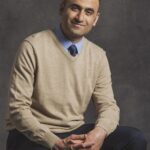
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?