Why Am I Seeing ‘ResizeObserver Loop Completed with Undelivered Notifications’ and How Can I Fix It?
In the ever-evolving landscape of web development, performance and responsiveness are paramount. As developers strive to create seamless user experiences, they often encounter a perplexing warning: “ResizeObserver loop completed with undelivered notifications.” This seemingly cryptic message can be a source of frustration, hinting at underlying issues in how elements on a webpage are monitored for size changes. Understanding this phenomenon is crucial for anyone looking to optimize their applications and ensure they run smoothly across all devices.
The ResizeObserver API is a powerful tool that allows developers to react to changes in the dimensions of an element. However, when the observer’s callback function triggers additional layout changes, it can lead to a loop that the browser struggles to resolve. This results in the aforementioned warning, indicating that some notifications about size changes were not delivered as expected. Such scenarios can impact performance, causing unnecessary reflows and jankiness in user interfaces.
Navigating the intricacies of the ResizeObserver can be daunting, especially for those new to modern web development practices. By delving into the causes and implications of this warning, developers can gain valuable insights into how to manage element resizing more effectively. Armed with this knowledge, they can enhance their applications’ responsiveness and provide a smoother experience for users, ultimately leading to better
Understanding ResizeObserver
ResizeObserver is a JavaScript API that allows developers to monitor changes to the dimensions of an element. It is particularly useful for responsive designs and dynamic layouts where the size of an element may change based on user interactions or changes in the viewport size. The API provides a way to react to these changes without the need for continuous polling.
When an element being observed changes size, the ResizeObserver notifies the developer through a callback function. However, there are scenarios where these notifications can become problematic, leading to a warning message in the console: “ResizeObserver loop completed with undelivered notifications.”
What Causes the Warning?
The warning occurs when a ResizeObserver callback results in additional changes to the size of the observed elements, creating a loop of notifications. This situation arises when the callback modifies the DOM or CSS properties that affect the observed element’s size. The loop is detected and subsequently interrupted, leading to undelivered notifications.
Key factors that contribute to this issue include:
- Multiple ResizeObservers observing the same element.
- Changes in the layout triggered by the ResizeObserver callback itself.
- Recursive resize events that depend on other elements or styles.
Best Practices to Avoid the Warning
To prevent the “ResizeObserver loop completed with undelivered notifications” warning, developers can adopt several best practices:
– **Debouncing Resize Events**: Implement a debouncing mechanism to limit the frequency of callbacks. This can help prevent rapid successive calls that may lead to a loop.
– **Avoid DOM Changes**: Ensure that the ResizeObserver callback does not alter the size of the observed element or any other elements that depend on it.
– **Use Conditional Logic**: Implement checks within the callback to determine if a resize event necessitates a layout change.
Here’s an example of a simple debouncing function:
“`javascript
function debounce(func, wait) {
let timeout;
return function executedFunction(…args) {
const later = () => {
clearTimeout(timeout);
func(…args);
};
clearTimeout(timeout);
timeout = setTimeout(later, wait);
};
}
“`
Handling ResizeObserver Notifications
In scenarios where the warning is unavoidable, it is essential to manage the notifications effectively. Developers can use a combination of try-catch blocks and logic to prevent application crashes or performance bottlenecks.
Approach | Description |
---|---|
Throttling | Limit the number of times the resize event is processed within a specific timeframe. |
State Management | Track the current state of the elements to avoid unnecessary updates during resize events. |
Use IntersectionObserver | Combine with IntersectionObserver for more efficient handling of layout changes that do not directly involve size. |
By implementing these practices, developers can enhance the performance and reliability of applications that utilize the ResizeObserver API, minimizing the risk of loops and undelivered notifications.
Understanding ResizeObserver
The `ResizeObserver` API provides a mechanism for observing changes to the dimensions of an element. It is particularly useful for responsive design, allowing developers to react to size changes dynamically. However, when using this API, developers may encounter the warning: “ResizeObserver loop completed with undelivered notifications.” This warning indicates that the observation loop has been interrupted before all notifications could be processed, which can lead to performance issues.
Causes of the Warning
Several factors can contribute to the appearance of this warning:
- Frequent Resizing Events: If the observed element or its ancestors are resized in a way that triggers the `ResizeObserver` repeatedly, it can create an infinite loop of notifications.
- Layout Thrashing: Manipulating the DOM in a way that affects the layout during the notification process can lead to this warning. For example, changing styles or dimensions of an element in response to a resize event can cause further resize events.
- Circular Dependencies: If an observer is set up in a way that it causes a cascade of resize events, leading back to the original observer, this can produce the loop warning.
Best Practices to Avoid the Warning
To mitigate the potential for this warning, consider the following best practices:
– **Debounce Resize Events**: Implement a debounce mechanism to limit how often resize notifications are processed. This can prevent rapid consecutive updates that may lead to an overflow of notifications.
“`javascript
const debounce = (fn, delay) => {
let timeoutId;
return (…args) => {
clearTimeout(timeoutId);
timeoutId = setTimeout(() => {
fn.apply(null, args);
}, delay);
};
};
const observer = new ResizeObserver(debounce(entries => {
// Handle resize events
}, 100));
“`
- Avoid Direct DOM Manipulations: Within the resize callback, avoid making immediate changes to the DOM that could trigger further resize events. Instead, use a separate function to handle those changes after the observer has completed processing.
- Limit Observations: Only observe elements that are necessary. If possible, unobserve elements that do not require ongoing observation.
Example of Proper Usage
Here is a simple example of how to use `ResizeObserver` effectively while avoiding the common pitfalls:
“`javascript
const element = document.querySelector(‘myElement’);
const observer = new ResizeObserver(entries => {
for (let entry of entries) {
// Update styles or classes based on the entry’s contentRect
const { width, height } = entry.contentRect;
if (width > 500) {
element.classList.add(‘large’);
} else {
element.classList.remove(‘large’);
}
}
});
// Start observing the target element
observer.observe(element);
“`
Handling Notifications Gracefully
In cases where the warning does occur, it is essential to ensure that your application can handle it gracefully:
- Monitor Performance: Use performance monitoring tools to track how resize events affect the application and identify areas for optimization.
- Log Warnings: Implement logging for the warning to understand when it happens and under what conditions, helping to guide further debugging efforts.
By following these practices and understanding the mechanics of `ResizeObserver`, developers can effectively manage element resizing without encountering the loop completion warning, leading to smoother and more efficient applications.
Understanding the ResizeObserver Loop Issue
Dr. Emily Chen (Web Performance Specialist, Tech Innovations Inc.). “The ‘ResizeObserver loop completed with undelivered notifications’ warning often arises due to a cycle in the observation process. This typically occurs when the observed element’s size changes as a result of the observer callback, leading to an infinite loop. Developers should ensure that their resize handling logic does not trigger further resize events.”
Mark Thompson (Front-End Development Lead, Creative Solutions). “This warning indicates that the ResizeObserver is trying to notify about changes that are not being processed. It is crucial to manage the observer’s callbacks effectively to avoid performance bottlenecks, especially in applications with complex layouts or frequent DOM updates.”
Sarah Patel (UX/UI Engineer, Future Design Agency). “To mitigate the ‘ResizeObserver loop’ issue, developers should consider debouncing resize events or using flags to prevent recursive calls. This approach can help maintain a smooth user experience while ensuring that the observer works as intended without overwhelming the browser.”
Frequently Asked Questions (FAQs)
What does the error “resizeobserver loop completed with undelivered notifications” mean?
This error indicates that the ResizeObserver has detected changes in the size of an observed element, but the notifications about these changes could not be delivered due to a loop in the observation process.
What causes the ResizeObserver loop error?
The error typically occurs when the resizing of an element triggers further changes in the layout, which then causes the ResizeObserver to fire again, creating a loop that prevents notifications from being delivered.
How can I resolve the ResizeObserver loop error?
To resolve this error, you can optimize your layout changes to avoid triggering additional ResizeObserver notifications. This may involve debouncing resize events or restructuring your code to minimize layout thrashing.
Is the ResizeObserver loop error a critical issue?
While it is not a critical issue that will crash your application, it can lead to performance problems and unintended behavior in your layout. It is advisable to address it to ensure smooth user experience.
Can I safely ignore the ResizeObserver loop error?
Ignoring the error is not recommended, as it may lead to performance degradation and unexpected behavior in your application. It is best to investigate and resolve the underlying cause.
Are there any known browser compatibility issues with ResizeObserver?
ResizeObserver is supported in most modern browsers. However, older versions of browsers may not fully support it, which could lead to inconsistencies or errors, including the ResizeObserver loop error. Always check for compatibility when implementing this feature.
The ResizeObserver loop completed with undelivered notifications is a common issue encountered by developers when working with the ResizeObserver API. This problem arises when the observer detects changes in the size of an element, leading to a loop of notifications that cannot be processed in a single frame. The browser’s rendering engine attempts to handle these notifications, but if they exceed the frame’s processing capacity, it results in the loop being interrupted, causing the error message to appear. Understanding this behavior is crucial for optimizing performance and ensuring smooth user experiences in web applications.
One of the primary causes of this issue is the manipulation of the DOM in response to resize events. When a ResizeObserver callback modifies the observed element’s size, it can trigger additional resize notifications, creating a feedback loop. Developers should be cautious about making synchronous changes to the DOM within the observer’s callback function. Instead, implementing debouncing techniques or using requestAnimationFrame can help manage these updates more effectively, preventing the loop from becoming unmanageable.
Moreover, it is essential to consider the implications of this error on user experience and application performance. Frequent resize notifications can lead to performance bottlenecks, especially in complex layouts or applications with multiple observers. By optimizing the use of ResizeObserver and ensuring that
Author Profile
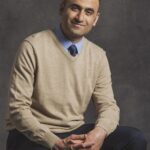
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?